Unlocking Rust's JSON Potential: Serde, The Important Parts
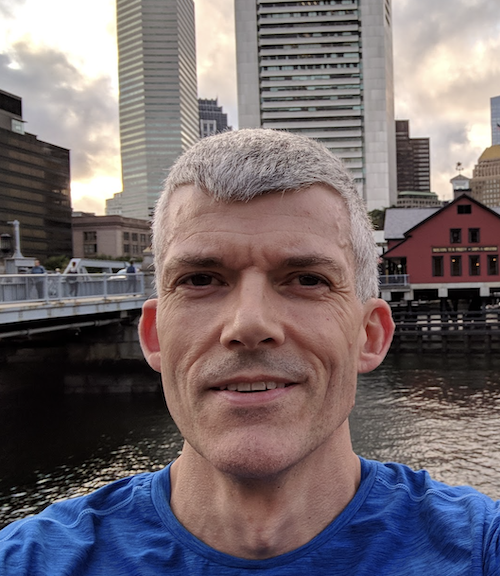
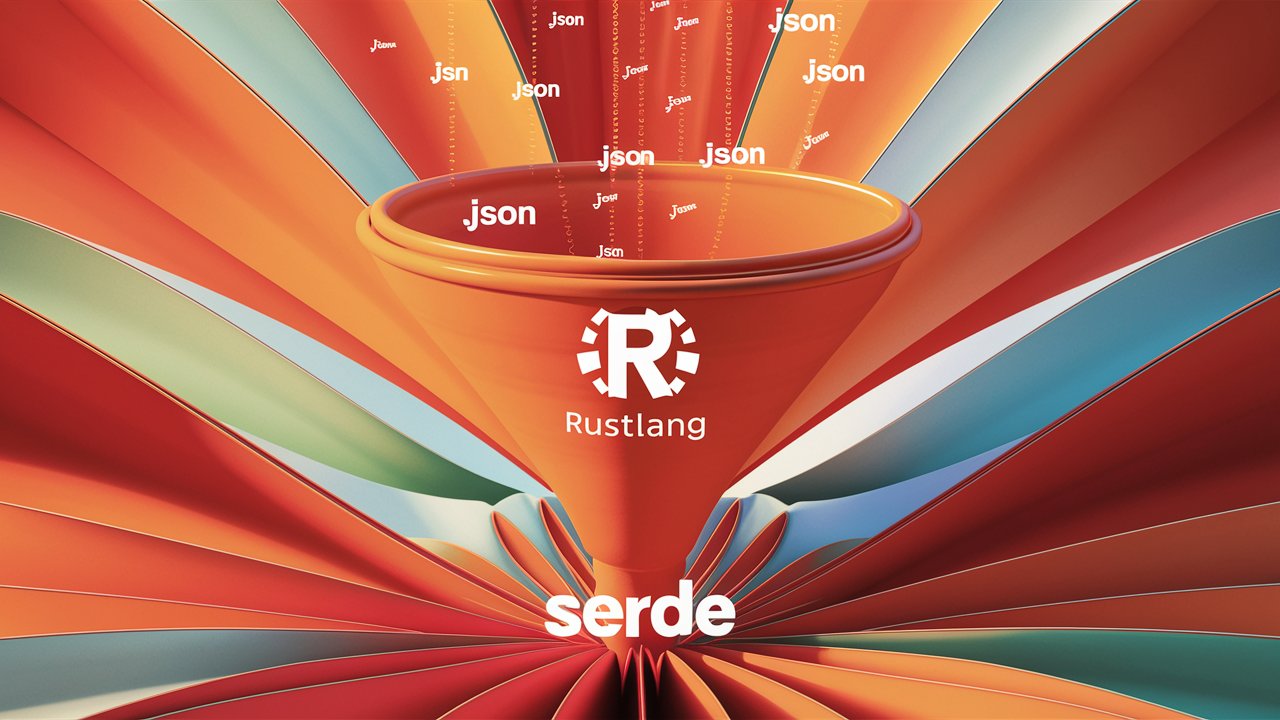
In this tutorial, we will explore the Serde (Ser*ializeDeserialize*) crate, a powerful library that simplifies working with JSON in Rust. By the end of this guide, you'll have a solid understanding of how to serialize and deserialize JSON data efficiently, enabling you to build applications that seamlessly communicate with other systems.
Getting Started with Serde
Before diving into the intricacies of JSON manipulation let's set up our Rust project and add the necessary dependencies. To begin, create a new Rust project using Cargo. Open your terminal and run the following command:
cargo new json_tutorial
cd json_tutorial
Next, we need to add the Serde crate to our project. Cargo, Rust's package manager, provides a convenient way to add dependencies using the cargo add
command. Open your terminal and run the following commands:
cargo add serde --features derive
cargo add serde_json
The cargo add
command automatically adds the specified crates to your Cargo.toml
file. By adding the serde
crate with the --features derive
flag, we enable the powerful derive feature, which we'll explore later in this post. The serde_json
crate is also added as a dependency for handling JSON serialization and deserialization.
After running these commands, your Cargo.toml
file will be updated with the following lines under the [dependencies]
section:
[dependencies]
serde = { version = "1.0.nnn", features = ["derive"] }
serde_json = "1.0.nnn"
The serde
crate provides the core functionality for serialization and deserialization, while serde_json
is a JSON-specific implementation of Serde. By specifying the derive
feature, we can use Serde's powerful derive macros to automatically generate serialization and deserialization code for our Rust structs (more on that next).
Defining Rust Structs for JSON Serialization
To work with JSON data effectively, we need to define Rust structs that mirror the structure of our JSON objects. Serde makes this process straightforward by allowing us to derive the Serialize
and Deserialize
traits for our structs.
Let's consider an example JSON object representing a person:
{
"name": "John Doe",
"age": 30,
"email": "john@example.com"
}
To represent this JSON object in Rust, we can define a struct as follows:
use serde::{Serialize, Deserialize};
#[derive(Serialize, Deserialize)]
struct Person {
name: String,
age: u32,
email: String,
}
By deriving the Serialize
and Deserialize
traits, Serde automatically generates the necessary code to convert between the Rust struct and its JSON representation.
Serializing Rust Structs to JSON
With our Rust struct defined, let's explore how to serialize it to JSON string using Serde.
To serialize a Person
instance to JSON, we can use the serde_json::to_string
function:
use serde::{Serialize, Deserialize};
use serde_json;
#[derive(Serialize, Deserialize)]
struct Person {
name: String,
age: u32,
email: String,
}
fn main() {
let person = Person {
name: "John Doe".to_string(),
age: 30,
email: "john@example.com".to_string(),
};
let json = serde_json::to_string(&person).unwrap();
println!("{}", json);
}
In this code snippet, we create an instance of the Person
struct and then use serde_json::to_string
to serialize it to a JSON string.
Running this code will output the following JSON string:
{"name":"John Doe","age":30,"email":"john@example.com"}
Serde handles the serialization process efficiently, ensuring that the resulting JSON string is valid and properly formatted.
Deserializing JSON to Rust Structs
In addition to serialization, Serde also simplifies the process of deserializing JSON strings back into Rust structs. Deserialization allows us to parse JSON data and convert it into meaningful Rust objects.
Using out Person struct example, we can have this JSON representation:
let json_person = r#"
{
"name": "Jane Smith",
"age": 28,
"email": "jane@example.com"
}
"#;
To deserialize this JSON string into a Person
struct, we can use the serde_json::from_str
function:
let person: Person = serde_json::from_str(json_person).unwrap();
println!("Name: {}", person.name);
println!("Age: {}", person.age);
println!("Email: {}", person.email);
Serde takes care of parsing the JSON string and populating the Person
struct with the corresponding values.
Running this code will output:
Name: Jane Smith
Age: 28
Email: jane@example.com
This is all brought to you via the magic of macros, in this case: #[derive(Serialize, Deserialize)]
Additional Features With Serde JSON
Serde offers several advanced features and configuration options to handle complex JSON structures and customize the serialization and deserialization process. Some notable ones include:
Renaming Fields: Serde allows you to rename struct fields to match the JSON keys using the
#[serde(rename = "...")]
attribute. This is useful when the Rust naming convention differs from the JSON key names.Optional Fields: You can mark struct fields as optional using the
Option<T>
type. Serde will automatically handle missing or null values during deserialization.Custom Serialization and Deserialization: For more complex scenarios, Serde provides the ability to implement custom serialization and deserialization logic using the
serialize_with
anddeserialize_with
attributes.Use Serde's
#[serde(default)]
attribute to provide default values for missing fields during deserialization.
Let's expand the Person
struct to incorporate these features:
#[derive(Serialize, Deserialize)]
struct Person {
#[serde(rename = "full_name")]
name: String,
age: Option<u32>,
email: String,
#[serde(default)]
is_active: bool,
}
In this updated Person
struct:
The
name
field is renamed tofull_name
using the#[serde(rename = "full_name")]
attribute to match the JSON key.The
age
field is marked as optional usingOption<u32>
, allowing it to handle missing or null values during deserialization.The
is_active
field is added with a default value offalse
using the#[serde(default)]
attribute.
Now, let's see how to use this updated Person
struct:
fn main() {
let json = r#"
{
"full_name": "John Doe",
"email": "john@example.com"
}
"#;
let person: Person = serde_json::from_str(json).unwrap();
println!("Name: {}", person.name);
println!("Age: {:?}", person.age);
println!("Email: {}", person.email);
println!("Is Active: {}", person.is_active);
}
In this example, the JSON string omits the age
field, and the is_active
field is not present. Serde handles these cases gracefully:
The
age
field is deserialized asNone
since it's missing in the JSON.The
is_active
field is assigned its default value offalse
.
Running this code will output:
Name: John Doe
Age: None
Email: john@example.com
Is Active: false
Serde: Your JSON Sidekick in Rust
In this tutorial, we explored the Serde crate and its capabilities for working with JSON in Rust. We learned how to define Rust structs that correspond to JSON objects, serialize Rust objects to JSON strings, and deserialize JSON strings back into Rust structs. Additionally, we discussed some advanced features and best practices to consider when using Serde.
It's important to note that Serde offers much more JSON functionality beyond what we've covered in this tutorial. The official Serde documentation is a great resource to explore additional features and advanced use cases.
Moreover, Serde has become the de facto serialization and deserialization library in the Rust ecosystem. If you need to work with any data format in Rust, it's often a good idea to search for "serde {{format}}" to find the relevant Serde integration. Chances are, there's already a Serde-compatible library available for your needs.
So, go forth and leverage the power of Serde in your Rust projects to handle JSON and other data formats with ease!
Subscribe to my newsletter
Read articles from Sam Keen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
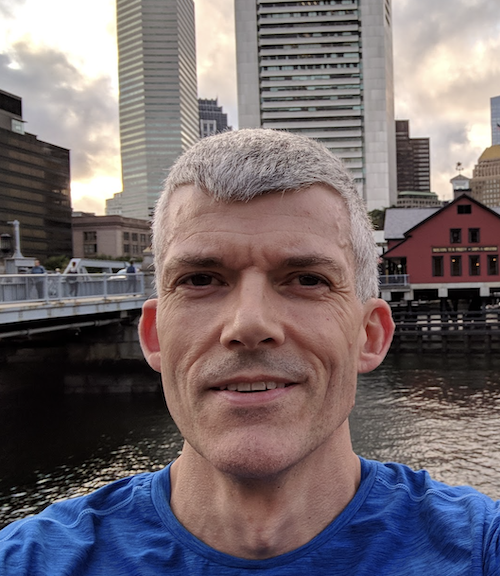
Sam Keen
Sam Keen
Experienced Software Development Leader | Expertise in Cloud Architecture | Spearheaded Projects at AWS, Lululemon, & Nike