Unlocking the Potential of C++: A Deep Dive into Closures
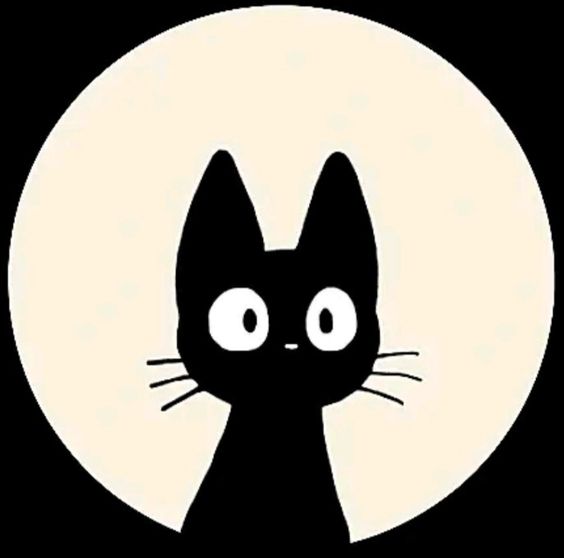
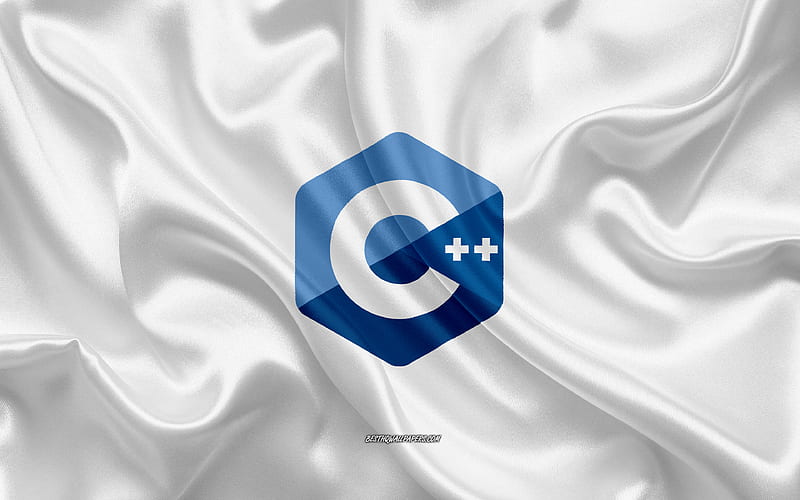
Pointers arent hard the syntax is said by someone i dont know lol. The thing about cpp is you can pretty much understand any concept but you will surely struggle to remember the syntax . why soo ?? because it is confusing . well if you are a developer like me who came from JS/TS environment you will be struck by the all the memory management concpet which are present in the C++. but fear not it is easy once you learn it .
Now coming to out today's topic , have you ever wondered what is the thing that a C++ lacks . Is that dynamic typing ? is that not having a easy syntax ? or is that not having js concept like closure ? yess you are absolutely correct . C++ does not have a concept like closure. does it affect C++ . nope!! not a tiny bit. but why do even need this , you don't!! what ? then why am i writing this blog you say ? it's something i did when i was learning about lambda and other cpp (kinda advance topics of C++). so i wanted to share this with this all.
What is closure ?
Before even i'm starting to implement the closure you guys should know about closure, right ? In simple words a closure is a function which returns another function. That's all !! in simple words i guess. what makes it pretty interesting is if you make use of variable which is present in the function which is returning function inside the returning function you get access to the variable directly. ok it didn't made much sense right . now let's look into the example given below.
function fn(){
let value=10;
return function(){
console.log("value is :",value);
}
}
let returnedFun=fn();
returnedFun(); // output: value is :10
If you are programmer coming from the other languages , let me explain you what is happening in the above code. fn is a function which is returning a anonymous function( means nameless function) which is possible in javascript cause in js functions are treated like object (in simple words like a variable) you can assign the function to any variable . it might be confusing a little bit but don't worry. just understand that fn is returning a function which is captured by returnedFun and then later gets called. The real intersting part is actaully the variable inside the returning function 'value'. If you guys know about memory management little bit especially stack , once you call a function it gets loaded to stack then after the execution everything inside the function will be destroyed . but not in closure!!
Closure concept is unique cause of this . value the variable inside the 'fn' function does not go out of scope untill the every other function which is making use of this has been executed. Its quite intersting right ? Where does these gets stored in the memory stack or heap ? . well they are not stored in stack cause of the behavior of stack so js stores it in heap (to learn more you can refer this ). The closure concept can lead to memory leaks if not handled correctly.
Implementing the closure in C++
Untill now you read about what is closure . how they work right ? now let's implement that in the C++. There are some pre requisite for going furhter cause i m making use of concept like functionl pointers, lambda and smart pointers . make sure to have basic understanding of these if not some thing might go overhead but you can still read cause who knows you might be a genius who gets this concept in one go!!
#include <iostream>
#include <functional>
#include <memory>
std::function<void()> fn()
{
std::shared_ptr<int> value = std::make_shared<int>(10);
std::cout << "outside address :" << &value << std::endl;
std::cout << "outside value :" << *value << std::endl;
return [&value]()
{
std::cout << "inside address :" << &value << std::endl;
std::cout << "inside value :" << *value << std::endl;
};
}
int main()
{
std::function<void()> fnPtr = fn();
fnPtr();
return 0;
}
//output:
//outside address :0x61fee4
//outside value :10
//inside address :0x61fee4
//inside value :10
In the above example i created a behavior like closure. The function fn have a return type of function which returns void. The returned function is lambda this is one of the way we can return function C++. we could also define the function outside and then return the reference. Now coming to the main topic where we are making use of value which is present in the parent function. I'm allocating space in heap so that it might remain even after the function execution. To manage the memory management of the pointer i m using smart pointer which deallocates memory after the value goes out of the scope (this is for simplifying) .
This is one of the way we could make the closure.Here i m passing value by reference but you can simply pass by value also . This will simplify thing even more.
#include <iostream>
#include <functional>
#include <memory>
std::function<void()> fn()
{
int value = 10;
std::cout << "outside address :" << &value << std::endl;
std::cout << "outside value :" << value << std::endl;
return [value]()
{
std::cout << "inside address :" << &value << std::endl;
std::cout << "inside value :" << value << std::endl;
};
}
int main()
{
std::function<void()> fnPtr = fn();
fnPtr();
return 0;
}
//output:
//outside address :0x61feec
//outside value :10
//inside address :0x61ff10
//inside value :10
Difference between JS and C++ closure:
As you might know this might not be the perfect closure implementation. But yeahh, what's wrong with experimenting things right ? Now looking at what is the difference between these two is JS allows automatic memory management. You don't need to manage memory but what about C++?? You have to do it manually . That is the primary reason i'm using smart pointers which manages memory automatically. As for the js syntax it is easy as hell , but C++ syntax is some what considered hard.
One of the issue with syntax ,if not handled correcty is the memory leak . This could give you one hell of nightmare .
Conclusion:
This is the two ways you can achieve the closure behavior in the C++ . well this is not might be the perfect implementation cause i did this for fun. The thing about C++ is , It is easy but not the syntax. But after learning how the C++ works you surely enjoy the coding . Enjoy the process, You will definitely get the good results.The last part might be gone off-topic but don't mind the minor error. Thank you <3
Subscribe to my newsletter
Read articles from Shainil P S directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
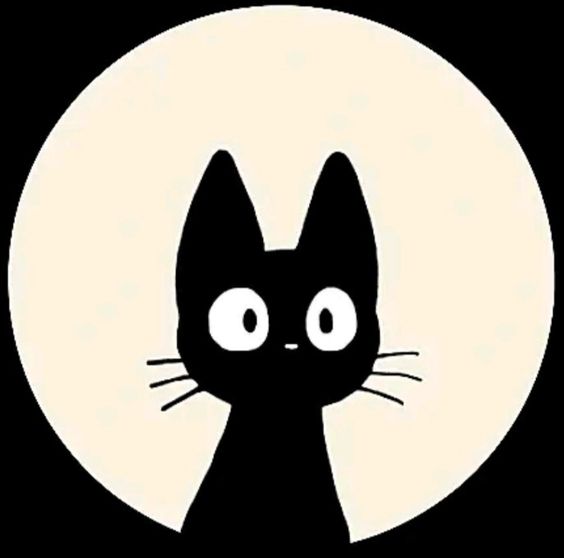
Shainil P S
Shainil P S
Hey, This is shainil. I m learning backend development along with dsa in cpp<3