Project-Based Learning: Building an Accordion Component
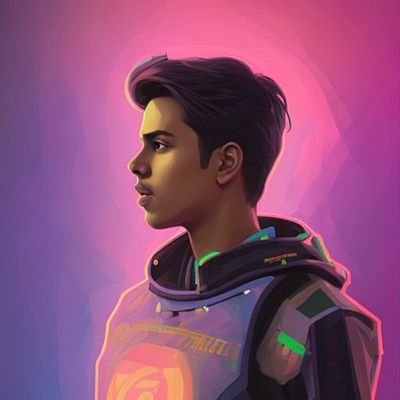
Table of contents
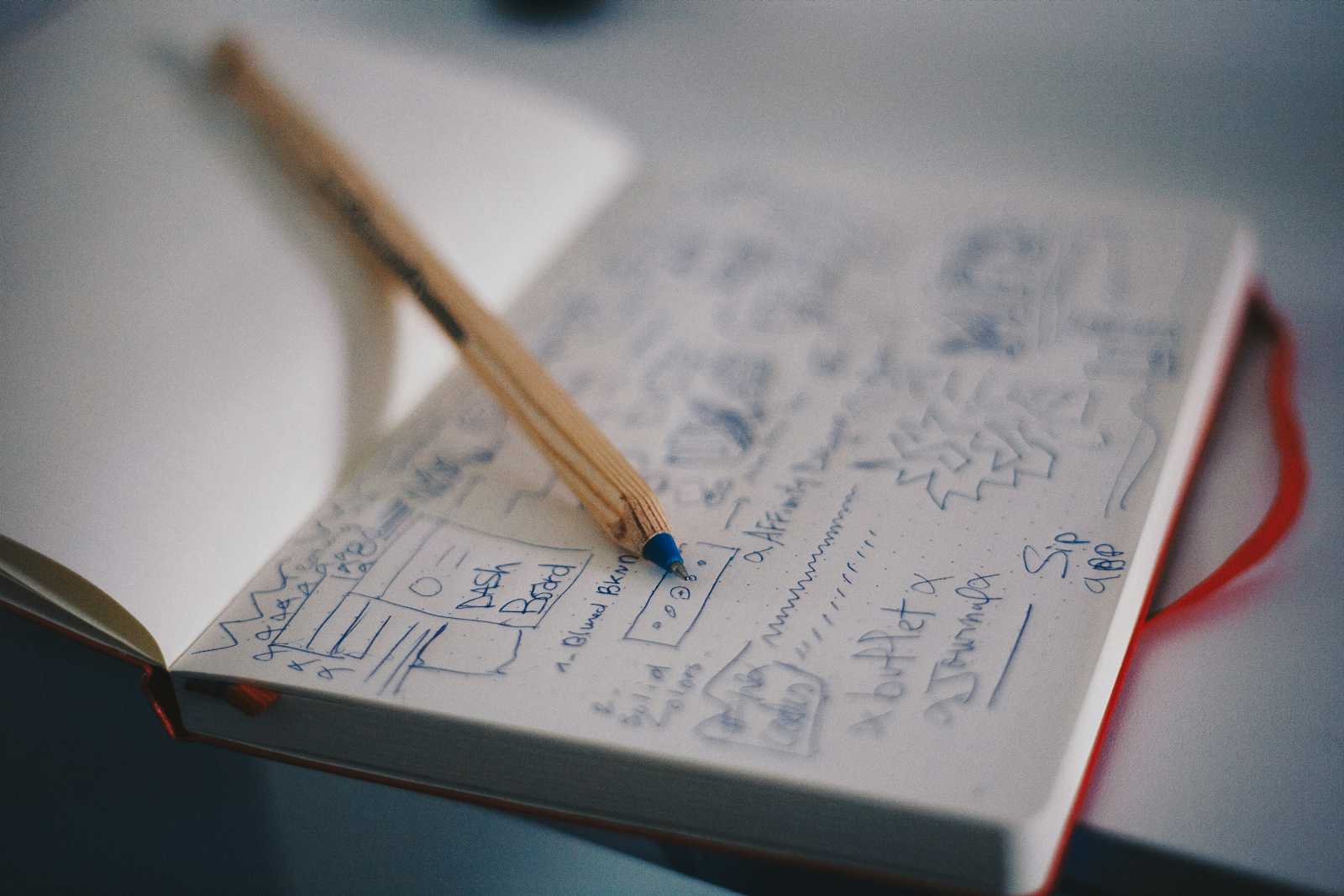
What's the best way to learn to code?
Project-based learning!
This is something we have always come across. I have encountered many blogs where devs have shared their journey of building products from scratch through blog posts and videos. In my journey, I am at the stage where I have started building projects to gain confidence in the skills that I have learned so far and what way is better than sharing the projects I build! So here I am trying my best to share my process and journey of getting better at react.
Now focusing on the main event, I will be building an accordion component. This component looks like this,
For building such a component we need some data that can be used as an example, I have used the following sample data for this practice session,
const data = [
{
id: "1",
question: "What are accordion components?",
answer:
"Accordion components are user interface elements used for organizing and presenting content in a collapsible manner. They typically consist of a header, content, and an expand/collapse action.",
},
{
id: "2",
question: "What are they used for?",
answer:
"They are commonly employed in various contexts, including FAQs, product descriptions, navigation menus, settings panels, and data tables, to save screen space and provide a structured and user-friendly interface for presenting information or options.",
},
{
id: "3",
question: "Accordion as a musical instrument",
answer:
"The accordion is a musical instrument with a keyboard and bellows. It produces sound by air passing over reeds when the player expands or compresses the bellows, used in various music genres.",
},
{
id: "4",
question: "Can I create an accordion component with a different framework?",
answer:
"Yes, of course, it is very possible to create an accordion component with another framework.",
},
];
export default data;
Here I have 3 params mainly id, question, and answer. My approach is gonna be to render an unordered list (ul) while running a loop to render the data with the help of the map method. I also used the useState hook to enable single selection so that at a time only one of the accordion questions displays their respective answers. The final code for the accordion component made is as follows,
import React, { useState } from "react";
import data from "./data";
function Accordian() {
const [select, setSelect] = useState(null);
const singleSelection = (getCurrentId) => {
setSelect(getCurrentId === select ? null : getCurrentId);
console.log(getCurrentId);
};
return (
<div>
<ul>
<div>
{data.map(({ id, question, answer }) => (
<div>
<button onClick={() => singleSelection(id)}>
{question}
<span>-</span>
</button>
{select === id ? <div>{answer}</div> : null}
</div>
))}
</div>
</ul>
</div>
);
}
export default Accordian;
This was a fairly simple project I built but it really made me happy as I was able to do this all by myself without any help and thus I am sharing this here. Many more projects and articles to come xD
With that note, Thanks for your time, and happy learning!!
Subscribe to my newsletter
Read articles from Shri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
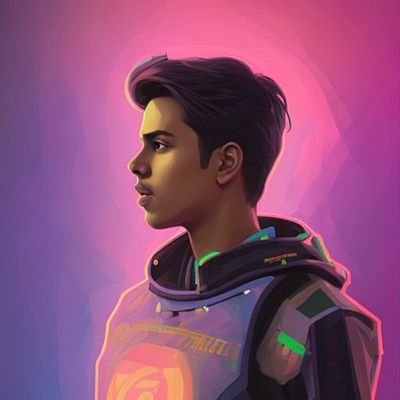
Shri
Shri
I am an aspiring software developer, currently learning Web dev. I write blogs sharing my knowledge as well as my tech journey.