Frontend notes 101

Table of contents
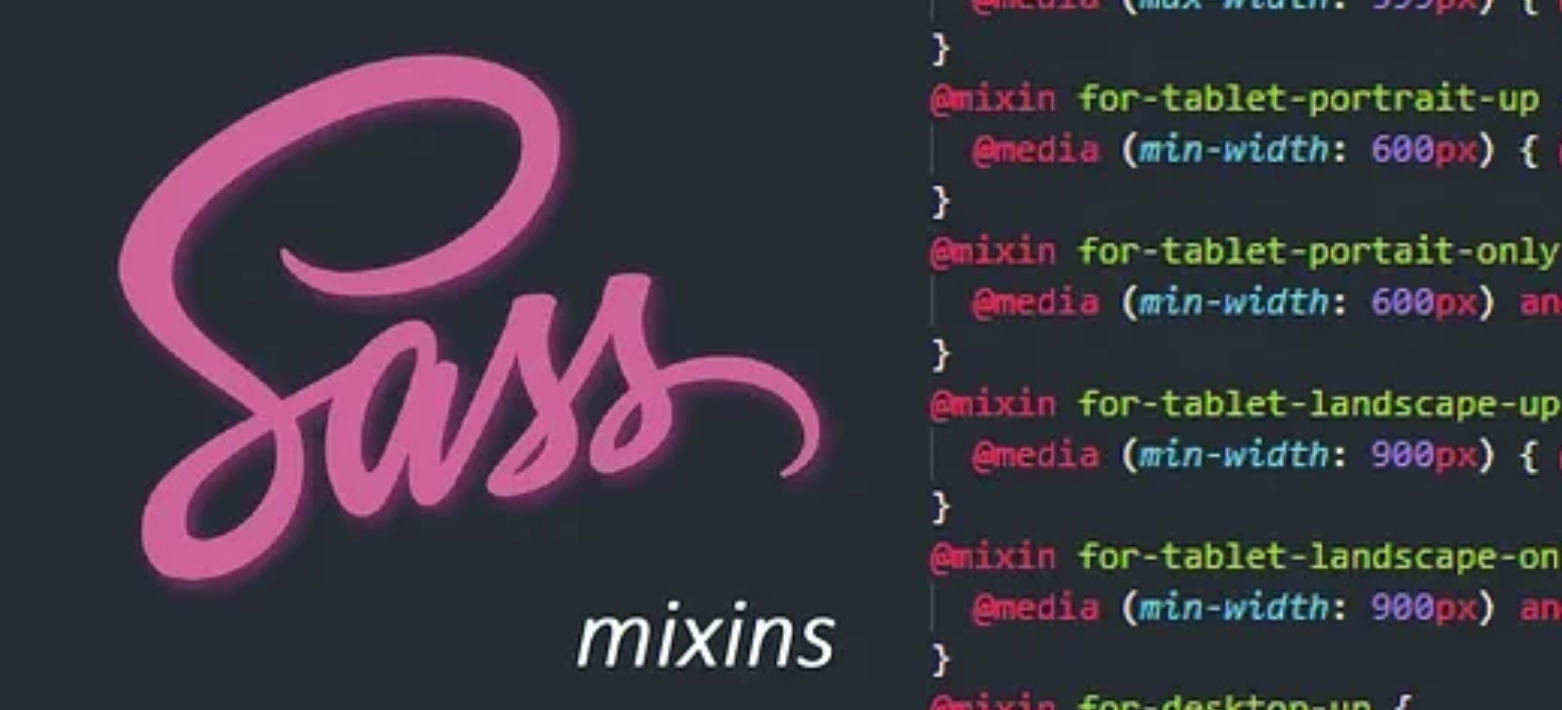
Before I begin, I would like to acknowledge that the ideas I will be sharing in this article, as well as in future ones, were taught to me by a significant person, who entered IT several years ago. He had the goodwill to impart his knowledge to me.
Sass
Something I liked a lot was to have all the styling sheets together in a folder aside from the project. I mean, assuming we're working with SASS it would be:
sass
├── global.scss
├── main.scss
├── mixins.scss
├── componentes
│ ├── footer.scss
│ └── navbar.scss
└── views
├── about.scss
├── contact.scss
└── home.scss
The Magic here for me is on the first three files. Let's take a look:
Global
This file contains styles that apply to the entire document, such as focus-visible styles for interactive elements, and global variables like fonts, colors, and margins. These variables can be declared using both CSS and SASS, but each approach has its differences.
Global variables with CSS (global.css)
// declaration
:root {
--aa-color-primary: #0743a7;
--aa-font-primary: 'Arial', sans-serif;
}
// usage
body {
color: var(--aa-color-primary);
font-family: var(--aa-font-primary);
}
Global variables with SASS (global.scss)
// declaration
$aa-color-primary: #0743a7;
$aa-font-primary: 'Arial', sans-serif;
// usage
body {
color: $aa-color-primary;
font-family: $aa-font-primary;
}
If we have to use these variables in other file, we must include @use:
// Other file.scss
@use 'global';
// global because global.scss is where the variables are declared
.some__class {
background-color: global.$aa-color-primary;
font-family: global.$aa-font-primary;
}
I'd rather use CSS variables, and declare inside :root because by only declaring them there, we'll have them available in the whole document because of :root scope.
Mixins
I will include a link to the documentation at the end of the document. In summary, a mixin is like a preset class used to avoid repetition, and it is also possible to pass values as parameters.
// mixins.scss
@mixin button__something {
color: var(aa-primary-color);
padding: 8px 12px;
}
and for using it, you must inlcude it inside a class or id:
// components/footer.scss
.footer__button--social {
@include button__something;
// other styles...
}
The keywords are @mixin to declare it and @include to use it. The parameterization would be something like:
@mixin button($bg-color, $text-color) {
background-color: $bg-color;
color: $text-color;
padding: 8px 16px;
border: none;
}
.button {
@include button(#007bff, #ffffff);
}
I'd like to point out that because CSS properties are read from top to bottom if you need to override a property of the mixin, you can include the mixin at the top of the class and the property to override below it.
Mobile-First + Mixins
Another cool thing I've learned is that, is possible to make mixins for breakpoints, to avoid using media queries. For those who are new, mobile-first design is the approach that prioritizes user experience and optimization in mobile devices, such as smartphones and tablets before desktop computers.
The main idea would be:
// mixins.scss
@mixin mobile-landscape {
@media screen and (min-width: 480px) {
@content;
}
}
@mixin tablet-portrait {
@media screen and (min-width: 720px) {
@content;
}
}
@mixin tablet-landscape {
@media screen and (min-width: 1024px) {
@content;
}
}
@mixin desktop {
@media screen and (min-width: 1200px) {
@content;
}
}
@mixin large-desktop {
@media screen and (min-width: 1600px) {
@content;
}
}
And The you'd apply it like:
.navbar__list {
display: none;
@include tablet-portrait {
display: flex;
...
}
@include tablet-landscape {
// otros estilos
}
}
As you can appreciate, the styles written first, outside the mixins, are the ones that are applied on mobile view, below 480px.
Main
Finally, main file would be in charge of wrapping up all the imports of the styling sheets (components + global + mixins + views). The idea is to link only one file to the entry point of the project (just include main.scss into index.html or whatever your entry point is) eg:
@import './mixins.scss';
@import './global.scss';
// components
@import './partials/footer.scss';
@import './partials/nav.scss';
// views
@import './page/about.scss';
@import './page/contact.scss';
@import './page/home.scss';
Bibliografia
Sass Basics: Mixins
MDN: Mobile First
Subscribe to my newsletter
Read articles from Francisco directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
