Building Fort Knox for Your Data: Essential API Security Best Practices
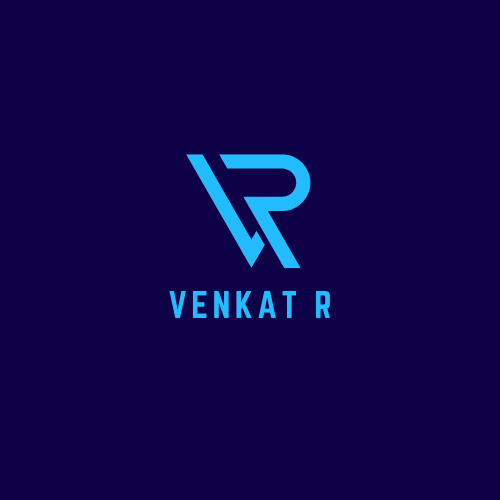
APIs are the backbone of modern applications, enabling seamless communication between systems and unlocking a world of integrations. But with great power comes great responsibility – ensuring your APIs are robust against security threats is paramount. In this post, we'll dive into the key challenges and how to address them, fortifying your APIs like a digital fortress.
Understanding the Threatscape
Let's start by understanding the types of attacks your APIs might face:
Unauthorized Access: Attackers attempting to access resources without proper credentials.
Data Exposure: Sensitive data being leaked due to vulnerabilities.
Injection Attacks: Malicious code injected into API requests.
Denial of Service (DoS): APIs being overwhelmed with requests to disrupt service.
Essential Security Measures
- Authentication: Verifying Who's Knocking
Authentication confirms the identity of the user or system making API requests.
API Keys: Simple but less secure, suitable for low-risk scenarios.
OAuth 2.0: Industry standard for delegated authorization, ideal for third-party applications.
JSON Web Tokens (JWT): Compact, self-contained tokens for stateless authentication.
JavaScript
// Example JWT verification (Node.js)
const jwt = require('jsonwebtoken');
const secretKey = 'your_secret_key';
jwt.verify(token, secretKey, (err, decoded) => {
if (err) {
// Invalid token
} else {
// Valid token, use 'decoded' to access user information
}
});
2. Authorization: What Can They Do?
Authorization determines what actions an authenticated user is permitted to perform.
Role-Based Access Control (RBAC): Assigns permissions based on user roles.
Attribute-Based Access Control (ABAC): Offers finer-grained control based on attributes (e.g., department, project).
JavaScript
// Example RBAC check (pseudocode)
if (user.role === 'admin') {
// Allow administrative actions
} else {
// Restrict to standard user actions
}
- Encryption: Protecting Data in Transit
Encrypting data prevents attackers from snooping on information during transmission.
HTTPS/TLS: Use HTTPS to encrypt all API communication.
JWT (again!): JWTs can be encrypted for additional security.
// Ensure your API endpoints are served over HTTPS (e.g., in your server configuration)
- Data Protection: Handling Sensitive Information
Input Validation: Rigorously validate all user input to prevent injection attacks (SQL injection, XSS).
Hashing: Store passwords and sensitive data using strong hashing algorithms.
Compliance: Understand and adhere to relevant regulations like GDPR and PCI-DSS.
JavaScript
// Example input validation (Node.js)
const validator = require('validator');
if (!validator.isEmail(userInput)) {
// Invalid email, handle error
}
- Preventing Common Attacks
SQL Injection: Use parameterized queries or prepared statements.
XSS Attacks: Sanitize user input before displaying it.
Rate Limiting/Throttling: Prevent abuse by limiting requests per user or IP address.
API Security Best Practices: A Deep Dive
- Security by Design (SbD): The Foundation of Resilience
Threat Modeling: Before writing a single line of code, identify potential threats your API could face. Consider the types of data it handles, who will access it, and how it interacts with other systems.
Secure Coding Standards: Adopt established guidelines (e.g., OWASP Top 10 for APIs) and use secure coding practices throughout development.
Minimum Exposure: Only expose the endpoints and functionality strictly necessary for your API's purpose. Reduce the attack surface by hiding internal implementation details.
- Rigorous Testing: Don't Just Assume It Works
Unit Testing: Test individual components of your API in isolation.
Integration Testing: Verify how different parts of your API interact.
Penetration Testing: Hire ethical hackers to simulate real-world attacks and identify vulnerabilities.
Fuzz Testing: Automatically bombard your API with invalid inputs to uncover unexpected errors.
- Least Privilege Principle (LPP): The Gatekeeper's Mandate
Role-Based Access Control (RBAC): Define roles with specific permissions and assign users to them.
Attribute-Based Access Control (ABAC): Fine-tune access based on user attributes, resource attributes, and environmental conditions.
Time-Based Restrictions: Limit access to certain functions or data based on time of day or other factors.
- Logging and Monitoring: Your API's Security Camera
Centralized Logging: Aggregate logs from all API components into a central repository for analysis.
Real-Time Alerts: Set up alerts to notify you of suspicious activity or potential attacks.
Anomaly Detection: Use machine learning or rule-based systems to identify unusual behavior.
- Patch Management: Keeping Your Defenses Up-to-Date
Automated Updates: Whenever possible, enable automatic updates for your API frameworks, libraries, and dependencies.
Vulnerability Scanning: Regularly scan your API for known vulnerabilities using tools like OWASP ZAP or Burp Suite.
Prompt Patching: Apply security patches as soon as they are released.
Additional Tips and Considerations
API Gateway: Consider using an API gateway to add a layer of security, rate limiting, caching, and other features to your API.
Documentation: Maintain clear, up-to-date documentation about your API's endpoints, authentication mechanisms, and security practices.
Security Training: Educate your development team about API security risks and best practices.
Remember: API security is an ongoing process. Stay informed about the latest threats and continuously adapt your security measures to keep your data safe and your users protected.
- By implementing these comprehensive security measures, you'll create APIs that are not only functional but also resilient against a wide range of threats. Remember, API security is a continuous process that requires vigilance and adaptation to new vulnerabilities.
Subscribe to my newsletter
Read articles from Venkat R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
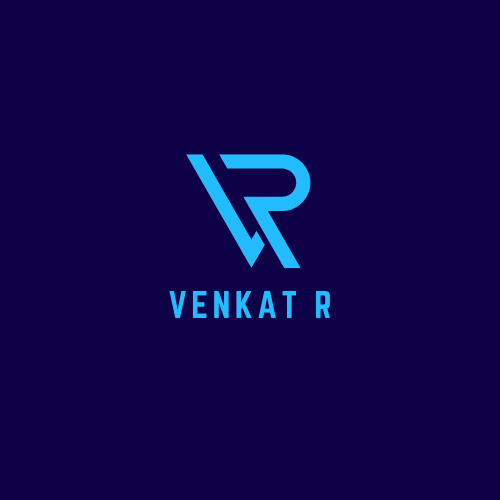
Venkat R
Venkat R
I am a marketer with the capacity to write and market a brand. I am good at LinkedIn. Your brand excellence on LinkedIn is always good with me.