Achieving API Performance: Scaling for Success in High-Demand Environments
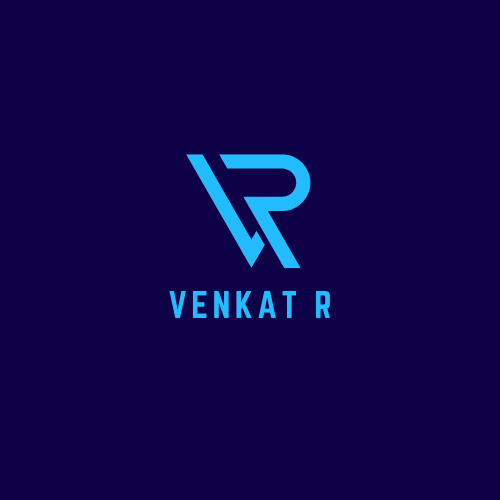
As APIs become the backbone of modern applications, ensuring their scalability and performance under heavy loads is paramount. Let's explore common challenges and solutions, from load balancing and caching to database optimization and monitoring.
1. Load Balancing and Horizontal Scaling
Challenge: A single API server can become a bottleneck as traffic increases, leading to slow response times and even crashes.
Solution:
Load Balancing: Distribute incoming requests across multiple API servers. Common load balancing algorithms include Round Robin, Least Connections, and Weighted Least Connections.
Horizontal Scaling: Add more servers dynamically as demand grows. This ensures that the API can handle increased traffic without sacrificing performance.
Code Example (Nginx Load Balancing):
Nginx
http {
upstream my_api {
server api1.example.com;
server api2.example.com;
# Add more servers as needed
}
server {
listen 80;
location / {
proxy_pass http://my_api;
}
}
}
2. Caching Strategies
Challenge: Repeatedly fetching the same data from the database or other sources can be computationally expensive and slow down the API.
Solution:
Content Delivery Networks (CDNs): Store frequently accessed static content (images, CSS, JS) on servers distributed geographically closer to users, reducing latency.
In-Memory Caching: Keep frequently used data in the application's memory (e.g., Redis, Memcached) for lightning-fast retrieval.
Code Example (Python with Redis Caching):
Python
import redis
cache = redis.Redis(host='localhost', port=6379, db=0)
def get_data_from_api(key):
cached_data = cache.get(key)
if cached_data:
return cached_data
else:
data = fetch_data_from_source() # Get from database or external API
cache.set(key, data)
return data
3. Rate Limiting and Throttling
Challenge: Excessive requests from a single client or unexpected traffic spikes can overwhelm the API and degrade service for all users.
Solution:
Rate Limiting: Set limits on the number of requests allowed within a specific time window (e.g., 100 requests per minute).
Throttling: Gradually reduce the response rate for clients exceeding rate limits to prevent sudden overload.
4. Optimizing Database Queries
Challenge: Inefficient database queries can become major performance bottlenecks, especially with large datasets.
Solution:
Indexing: Create indexes on frequently queried columns to speed up data retrieval.
Query Optimization: Analyze and refactor complex queries, avoid unnecessary joins, and use appropriate data types.
Batching: Fetch data in batches to reduce the number of database round trips.
5. Handling Concurrent Requests
Challenge: Managing multiple requests simultaneously can lead to resource contention and performance degradation.
Solution:
Asynchronous Programming: Use asynchronous frameworks (e.g., Node.js, Python's asyncio) or libraries to handle multiple requests concurrently without blocking the main thread.
Thread Pooling: Limit the number of threads processing requests to prevent excessive resource consumption.
6. Monitoring and Performance Testing Tools
Challenge: Identifying performance bottlenecks and understanding how the API behaves under load requires continuous monitoring and testing.
Solution:
Monitoring: Use tools like Prometheus, Grafana, or New Relic to track key metrics like response time, error rate, and resource usage.
Performance Testing: Simulate realistic traffic patterns with tools like Apache JMeter or Locust to uncover bottlenecks and measure scalability.
Building scalable and performant APIs is a multi-faceted challenge, but by addressing key considerations like load balancing, caching, rate limiting, and database optimization, developers can create robust APIs that thrive under heavy loads.
By employing horizontal scaling and load balancing, APIs can gracefully handle increasing traffic. Caching strategies, both at the edge and in-memory, drastically improve response times for frequently accessed data. Rate limiting and throttling protect APIs from abuse and unexpected spikes. Meanwhile, optimizing database queries and employing asynchronous techniques ensure efficient resource utilization.
Lastly, diligent monitoring and performance testing provide valuable insights into API behavior, allowing for continuous improvement and proactive problem-solving. By mastering these strategies, developers empower their APIs to deliver exceptional performance, reliability, and user satisfaction in today's demanding digital landscape.
Subscribe to my newsletter
Read articles from Venkat R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
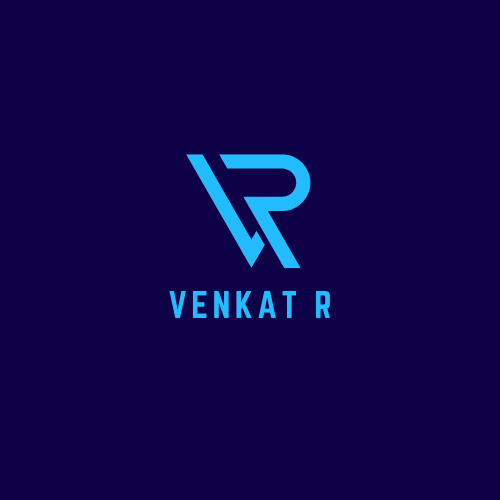
Venkat R
Venkat R
I am a marketer with the capacity to write and market a brand. I am good at LinkedIn. Your brand excellence on LinkedIn is always good with me.