Documentation and Developer Experience Challenges in API Development
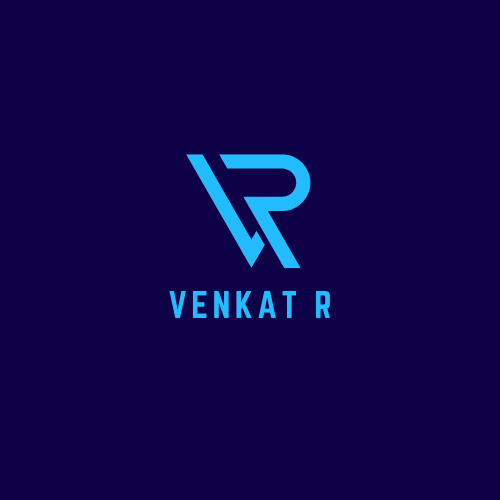
Creating a successful API involves more than just writing functional code. The way developers interact with your API – from initial onboarding to debugging issues – profoundly impacts adoption and satisfaction. This post explores key challenges and best practices in API documentation, design, and developer support.
Writing Clear and Comprehensive API Documentation
Thorough documentation is the cornerstone of a positive developer experience. It acts as a reference guide, a tutorial, and a problem-solving tool.
Essentials of Good API Documentation:
Clear Structure: Organize information logically, grouping endpoints by functionality, data types, or usage patterns. Use a table of contents for easy navigation.
Detailed Endpoint Descriptions: For each endpoint:
HTTP Method (GET, POST, PUT, DELETE, etc.)
Resource Path (URL)
Request Parameters (Including data types, whether required or optional, and examples)
Response Formats (Success and error responses with status codes and sample data)
Authentication and Authorization: Explain how to acquire and use API keys, tokens, or other authentication mechanisms.
Error Handling: Document error codes, their meanings, and recommended actions for developers.
Code Examples: Provide examples in various programming languages to demonstrate request and response handling.
Interactive Features: Consider tools like Swagger UI or Redoc to allow developers to test API calls directly from the documentation.
Versioning: Clearly indicate the API version for each documentation set.
Example Documentation Snippet (Using OpenAPI/Swagger):
YAML
paths:
/items:
get:
summary: Get all items
description: Returns a list of all items.
responses:
'200':
description: Successful operation
content:
application/json:
schema:
type: array
items:
$ref: '#/components/schemas/Item'
Writing Clear and Comprehensive API Documentation
Beyond the Basics: Additional Documentation Elements:
Use Cases: Illustrate how the API can be used to solve real-world problems. Showcasing practical applications helps developers visualize how to integrate the API into their projects.
Rate Limiting and Throttling: If your API has usage limits, clearly document them, including thresholds and how developers can handle rate limiting errors.
Webhooks: If your API supports webhooks for event-driven notifications, document the available events, payloads, and how to set up webhook subscriptions.
Changelog: Maintain a changelog that tracks changes to the API over time, including new features, deprecations, and bug fixes. This helps developers stay informed and adapt their integrations.
Glossary: If your API uses domain-specific terminology, include a glossary to define terms and ensure clear communication.
Tools for Creating and Maintaining Documentation:
OpenAPI/Swagger: A widely adopted standard for describing RESTful APIs. It allows you to create interactive documentation with features like automatic API call testing.
Redoc: A popular tool for generating visually appealing API documentation from OpenAPI specifications.
Stoplight: A comprehensive API design and documentation platform that offers features like visual modeling, mocking, and collaboration.
Read the Docs: A documentation hosting platform well-suited for technical documentation.
Slate: A flexible, open-source documentation framework that allows you to create beautiful, customized docs.
API Design Best Practices
HATEOAS in Practice:
To implement HATEOAS effectively:
Use Link Relation Types (rels): Standard rels like
self
,next
,prev
, andcollection
define the meaning of links in your responses.Include Links in Resource Representations: Embed links within your resource representations (e.g., JSON or XML) to point to related resources or actions.
Make Actions Discoverable: Clients should be able to navigate your API solely by following links in responses, without needing prior knowledge of URLs.
Example HATEOAS Response:
JSON
{ "name": "John Doe", "links": [ { "rel": "self", "href": "/users/123" }, { "rel": "orders", "href": "/users/123/orders" } ] }
Versioning Strategies:
URI Versioning: Include the API version in the URI (e.g.,
/v1/users
).Query Parameter Versioning: Pass the version as a query parameter (e.g.,
/users?version=1
).Custom Header Versioning: Use a custom HTTP header to indicate the version (e.g.,
Api-Version: 1
).
SDK Generation and Client Libraries
Benefits of SDKs and Client Libraries:
Simplified Integration: Developers don't need to write low-level HTTP requests and handle response parsing.
Error Handling: Client libraries often include built-in error handling and retries.
Improved Productivity: Developers can focus on their application logic rather than API details.
Popular SDK Generation Tools:
OpenAPI Generator: A versatile tool that supports generating SDKs and client libraries in a wide range of programming languages from OpenAPI specifications.
Swagger Codegen: Another powerful tool for generating SDKs and server stubs based on OpenAPI/Swagger definitions.
Braze: A commercial platform that offers SDK generation and other API management features.
Sandbox and Testing Environments
Key Features of Sandbox Environments:
Isolation: Sandbox data should be separate from production data to prevent accidental modifications or data leaks.
Realistic Data: Populate the sandbox with representative data to simulate real-world scenarios.
Limited Functionality: You can choose to restrict certain features or actions in the sandbox to maintain control.
Rate Limiting: Consider applying looser rate limits in the sandbox to allow for more extensive testing.
Debugging Tools: Provide tools to help developers monitor API calls, view logs, and debug issues in the sandbox.
Error Handling and Status Codes
Best Practices for Error Handling:
Consistency: Use consistent error formats across your API, including error codes, messages, and optional details.
Informative Error Messages: Provide clear explanations of why an error occurred and suggest possible solutions.
Retry Logic: For transient errors, encourage clients to implement retry logic with exponential backoff.
Developer Onboarding and Support Strategies
Additional Support Channels:
Chat Support: Offer real-time chat support for immediate assistance.
Community Forums: Encourage developers to help each other and share knowledge in forums or discussion groups.
Office Hours: Schedule regular virtual office hours where developers can ask questions and get direct support.
Developer Experience Metrics:
Time to First Hello World (TTFHW): Measure how long it takes a developer to make their first successful API call.
API Usage: Track the number of API calls, successful calls, and errors.
Documentation Usage: Monitor which documentation pages are most viewed and for how long.
Support Ticket Volume and Resolution Time: Track the number of support tickets and how quickly they are resolved.
Subscribe to my newsletter
Read articles from Venkat R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
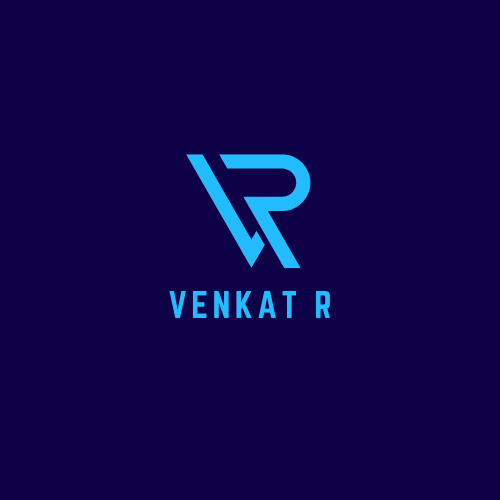
Venkat R
Venkat R
I am a marketer with the capacity to write and market a brand. I am good at LinkedIn. Your brand excellence on LinkedIn is always good with me.