Topic: 12 Understanding Design Patterns in Flutter

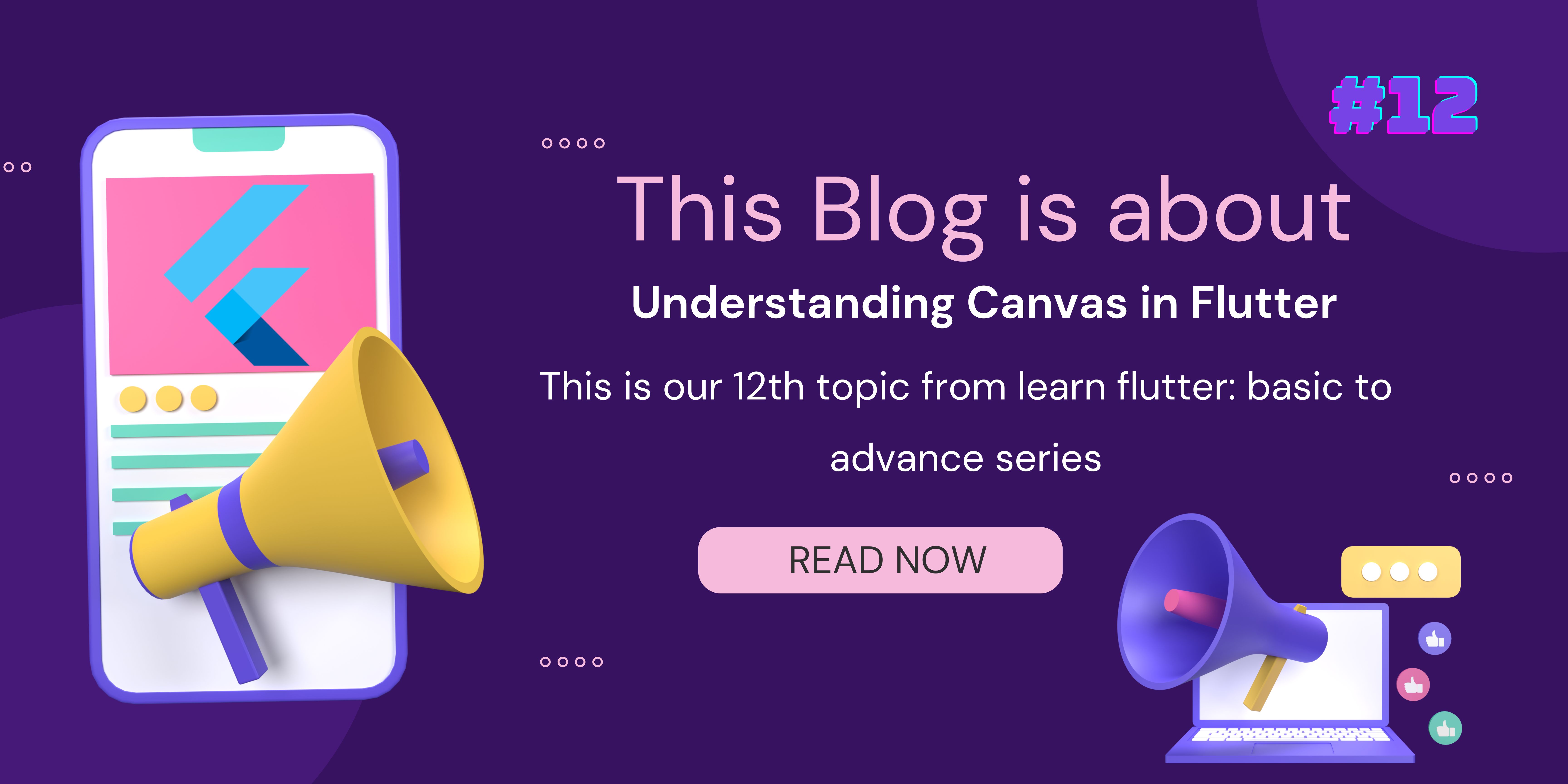
Hello devs, Today we talk about different types of design patterns in Flutter. These design patterns help us to write clean and understandable code for flutter projects. There are so many design patterns available. Use this as per your project requirement.
MVC- Model View Controller
MVC stands for Model-View-Controller. It's an architectural pattern that separates an application into three interconnected components:
Model: The model represents the data and business logic of the application. It's responsible for managing the data and notifying the views of any changes. In Flutter, models are often classes or objects that encapsulate the application's data and provide methods to manipulate that data.
View: The view represents the UI of the application. It's responsible for displaying the data to the user and capturing user input. In Flutter, views are typically represented by widgets, which are the building blocks of the UI.
Controller: The controller acts as an intermediary between the model and the view. It receives user input from the view, interacts with the model to retrieve or update data, and updates the view accordingly. In Flutter, controllers are often implemented using StatefulWidget or StatelessWidget classes.
Applying MVC in Flutter
Now that we understand the basic concepts of MVC, let's see how we can apply it in Flutter development.
Model:
In Flutter, the model is where you define your application's data structures and business logic. This could include classes representing entities such as users, products, or any other data relevant to your application. Here's a simple example of a model class representing a user:
class User {
final String name;
final int age;
User({required this.name, required this.age});
}
View:
The view in Flutter corresponds to the UI components that users interact with. Views are typically built using widgets, which are composable and reusable UI elements. Here's an example of a simple view that displays a user's name and age:
class UserView extends StatelessWidget {
final User user;
UserView({required this.user});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('User Profile')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Name: ${user.name}'),
Text('Age: ${user.age}'),
],
),
),
);
}
}
Controller:
The controller in Flutter acts as a bridge between the model and the view. It listens for user input, interacts with the model to retrieve or update data, and updates the view accordingly. Here's an example of a simple controller that handles user interactions:
class UserController {
final User user;
final BuildContext context;
UserController({required this.user, required this.context});
void updateUserAge(int newAge) {
// Update user's age in the model
user.age = newAge;
// Update the view
Navigator.pop(context);
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => UserView(user: user),
),
);
}
}
Benefits of Using MVC in Flutter
Using the MVC pattern in your Flutter applications offers several benefits:
Separation of Concerns: MVC separates the different aspects of your application, making it easier to manage and maintain.
Reusability: By separating the UI components from the business logic, you can reuse views and models across different parts of your application.
Testability: MVC makes it easier to write unit tests for your application since each component can be tested independently.
Scalability: MVC allows you to scale your application more effectively by keeping the codebase organized and modular.
The MVC pattern is a powerful tool for structuring your Flutter applications in a clear and maintainable way. By separating the concerns of your application into models, views, and controllers, you can build robust and scalable applications that are easier to manage and extend. Whether you're working on a small personal project or a large-scale enterprise application, understanding and applying the MVC pattern will undoubtedly improve the quality and maintainability of your code.
Ok devs, Now we talk about MVVM design pattern.
MVVM- Model View ViewModel
MVVM stands for Model-View-ViewModel. It's an architectural pattern that separates an application into three key components:
Model: The model represents the data and business logic of the application. It's responsible for managing the application's data and business rules. In Flutter, models are typically classes or objects that encapsulate the data and provide methods to manipulate it.
View: The view represents the UI of the application. It's responsible for displaying the data to the user and capturing user input. In Flutter, views are built using widgets, which are the building blocks of the UI.
ViewModel: The ViewModel acts as an intermediary between the model and the view. It exposes the data from the model to the view and contains the presentation logic required to display the data. In Flutter, viewModels are often implemented using classes that extend ChangeNotifier or similar state management solutions.
Applying MVVM in Flutter
Now that we understand the basic concepts of MVVM, let's see how we can apply it in Flutter development.
Model:
In Flutter, the model represents the data and business logic of the application. This could include classes representing entities such as users, products, or any other data relevant to your application. Here's an example of a simple model class representing a user:
class User {
final String name;
final int age;
User({required this.name, required this.age});
}
View:
The view in Flutter corresponds to the UI components that users interact with. Views are typically built using widgets, which are composable and reusable UI elements. Here's an example of a simple view that displays a user's name and age:
class UserView extends StatelessWidget {
final User user;
UserView({required this.user});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('User Profile')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Name: ${user.name}'),
Text('Age: ${user.age}'),
],
),
),
);
}
}
ViewModel:
The ViewModel in Flutter contains the presentation logic required to display the data in the view. It exposes the data from the model to the view and handles any transformations or formatting required. Here's an example of a simple ViewModel class for the user view:
import 'package:flutter/material.dart';
class UserViewModel extends ChangeNotifier {
final User _user;
UserViewModel({required User user}) : _user = user;
String get userName => _user.name;
int get userAge => _user.age;
void updateUserAge(int newAge) {
// Update user's age in the model
_user.age = newAge;
// Notify listeners that the data has changed
notifyListeners();
}
}
Benefits of Using MVVM in Flutter
Using the MVVM pattern in your Flutter applications offers several benefits:
Separation of Concerns: MVVM separates the UI logic from the business logic, making it easier to maintain and test.
Reusability: By separating the UI logic into viewModels, you can reuse the same logic across multiple views.
Testability: MVVM makes it easier to write unit tests for your application since the business logic is decoupled from the UI.
Scalability: MVVM allows you to scale your application more effectively by keeping the codebase organized and modular.
The MVVM pattern is a valuable tool for structuring your Flutter applications in a clear and maintainable way. By separating the concerns of your application into models, views, and viewModels, you can build robust and scalable applications that are easier to manage and extend. Whether you're working on a small personal project or a large-scale enterprise application, understanding and applying the MVVM pattern will undoubtedly improve the quality and maintainability of your code.
These two design patterns we mostly used in every programming language. Now we explore some flutter design patterns.
BloC Pattern- Business Logic Component
BLoC stands for Business Logic Component. It's a design pattern for managing state in Flutter applications. BLoC separates the presentation layer from the business logic and the data layer, making your code more modular and easier to maintain.
In the BLoC pattern, there are three main components:
Business Logic: This is where you encapsulate the logic of your application. It includes data manipulation, validation, and any other business rules.
Events: Events are triggers that represent user actions or system events. They are dispatched to the BLoC to request changes in the application state.
States: States represent the current state of the application. They are emitted by the BLoC in response to events and are used to update the UI.
Applying BLoC in Flutter
Now, let's see how we can implement the BLoC pattern in Flutter.
Business Logic:
The business logic of your application is encapsulated in a BLoC class. This class typically extends Bloc
from the bloc
package. Here's a simple example of a BLoC class that manages the counter state:
import 'package:bloc/bloc.dart';
enum CounterEvent { increment, decrement }
class CounterBloc extends Bloc<CounterEvent, int> {
CounterBloc() : super(0);
@override
Stream<int> mapEventToState(CounterEvent event) async* {
switch (event) {
case CounterEvent.increment:
yield state + 1;
break;
case CounterEvent.decrement:
yield state - 1;
break;
}
}
}
Events:
Events are represented as enums and are dispatched to the BLoC to request changes in the application state. Here's an example of how you can dispatch events to the counter BLoC:
counterBloc.add(CounterEvent.increment);
States:
States represent the current state of the application and are emitted by the BLoC in response to events. You can listen to state changes using a BlocBuilder
widget. Here's an example of how you can use BlocBuilder
to update the UI based on the counter state:
BlocBuilder<CounterBloc, int>(
builder: (context, count) {
return Text('Count: $count');
},
);
Benefits of Using BLoC in Flutter
Using the BLoC pattern in your Flutter applications offers several benefits:
Separation of Concerns: BLoC separates the presentation layer from the business logic, making your code more modular and easier to maintain.
Reactive Programming: BLoC leverages streams to manage state changes, allowing for a reactive programming approach.
Testability: BLoC makes it easier to write unit tests for your application since the business logic is decoupled from the UI.
Scalability: BLoC allows you to scale your application more effectively by keeping the codebase organized and modular.
The BLoC pattern is a powerful tool for managing state in Flutter applications. By separating the presentation layer from the business logic, BLoC makes your code more modular, testable, and scalable. Whether you're working on a small personal project or a large-scale enterprise application, understanding and applying the BLoC pattern will undoubtedly improve the quality and maintainability of your Flutter code.
Okay devs, Let's talk about another design pattern that is available for Flutter Stacked Architecture**.**
Stacked Architecture
Stacked architecture is a design pattern that emphasizes a structured and modular approach to building Flutter applications. At its core, Stacked promotes the separation of concerns, making it easier to manage both the UI and business logic of your app.
In the Stacked architecture, there are typically three main components:
Views: Views represent the UI components of your application. They are responsible for rendering the user interface and capturing user input.
ViewModels: ViewModels act as intermediaries between the views and the data/business logic of your application. They expose the data and functionality required by the view and handle any interactions with the underlying data layer.
Services: Services encapsulate the data and business logic of your application. They are responsible for fetching and manipulating data, performing computations, and executing business logic.
Applying Stacked Architecture in Flutter
Let's take a closer look at how we can implement the Stacked architecture in a Flutter application.
Views:
Views in Flutter represent the UI components of your application. They are typically built using widgets and are responsible for rendering the UI. Here's an example of a simple view that displays a user profile:
class UserProfileView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('User Profile')),
body: Center(
child: Text('Welcome to your profile!'),
),
);
}
}
ViewModels:
ViewModels in Stacked architecture contain the presentation logic required by the view. They expose the data and functionality required by the view and handle any interactions with the underlying data layer. Here's an example of a simple viewModel for the user profile view:
import 'package:stacked/stacked.dart';
class UserProfileViewModel extends BaseViewModel {
String _userName = '';
String get userName => _userName;
Future<void> fetchUserData() async {
// Fetch user data from a service
_userName = await userService.getUserName();
// Notify listeners that the data has changed
notifyListeners();
}
}
Services:
Services in Stacked architecture encapsulate the data and business logic of your application. They are responsible for fetching and manipulating data, performing computations, and executing business logic. Here's an example of a simple service for fetching user data:
class UserService {
Future<String> getUserName() async {
// Simulated API call to fetch user name
await Future.delayed(Duration(seconds: 2));
return 'John Doe';
}
}
Benefits of Using Stacked Architecture in Flutter
Using the Stacked architecture in your Flutter applications offers several benefits:
Separation of Concerns: Stacked promotes the separation of concerns, making it easier to manage both the UI and business logic of your application.
Modularity: Stacked encourages a modular approach to building Flutter applications, allowing you to easily reuse code and components across your app.
Testability: Stacked makes it easier to write unit tests for your application since the business logic is decoupled from the UI.
Scalability: Stacked allows you to scale your application more effectively by keeping the codebase organized and modular.
The Stacked architecture is a powerful tool for building Flutter applications that are both robust and maintainable. By promoting the separation of concerns and modularity, Stacked makes it easier to manage both the UI and business logic of your app. Whether you're working on a small personal project or a large-scale enterprise application, understanding and applying the Stacked architecture will undoubtedly improve the quality and maintainability of your Flutter code.
There are many more design patterns available but I have only explained some of the design patterns that I know and I have used in my project devs.
Alright devs, This is the end of our blog i hope this blog helps you to easily understand design patterns in Flutter. Okay, then We catch up on our next topic data storage in Flutter.
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!