Unraveling JavaScript: A Beginner's Guide to Understanding and Mastering the Web's Versatile Language
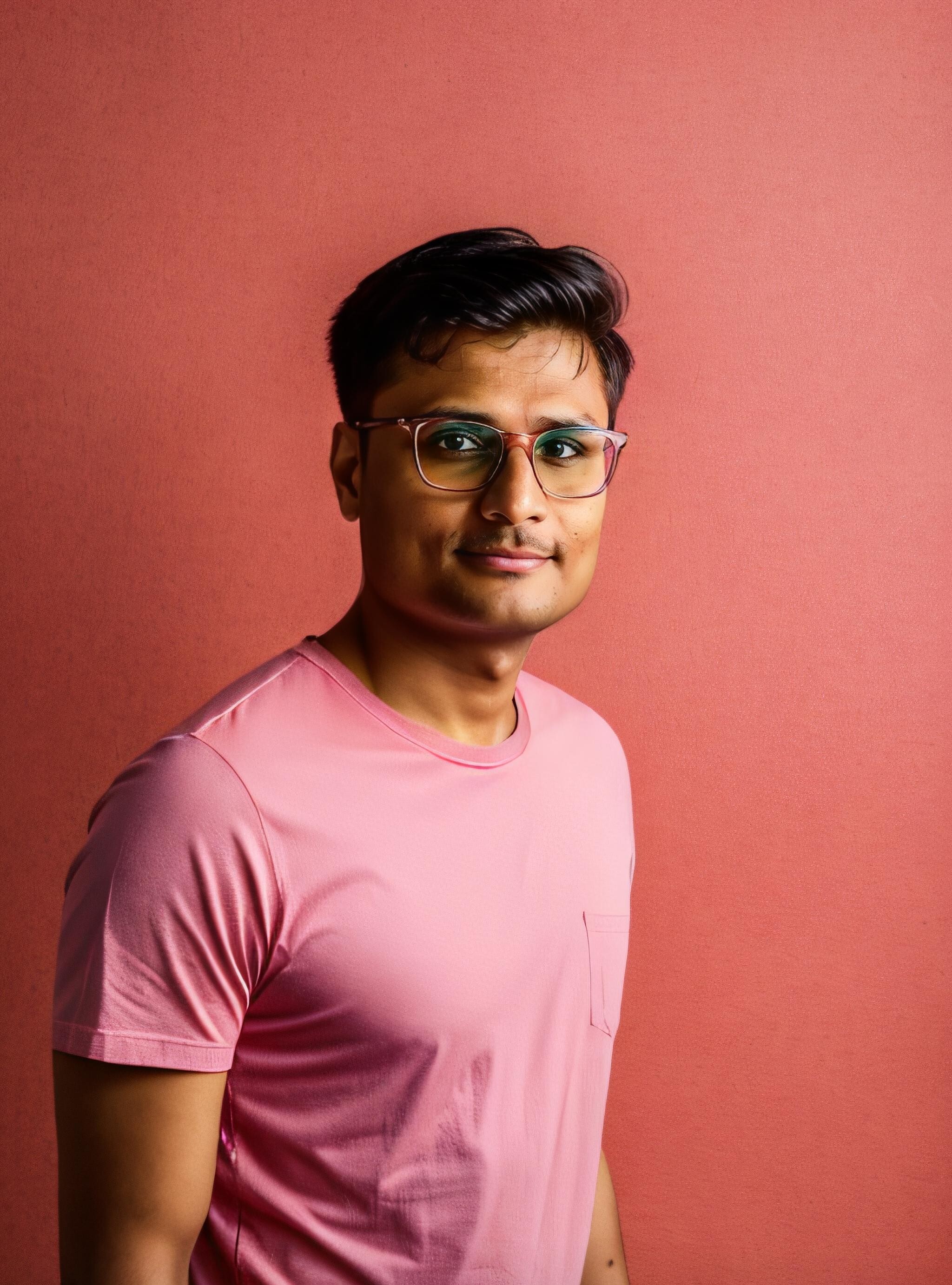
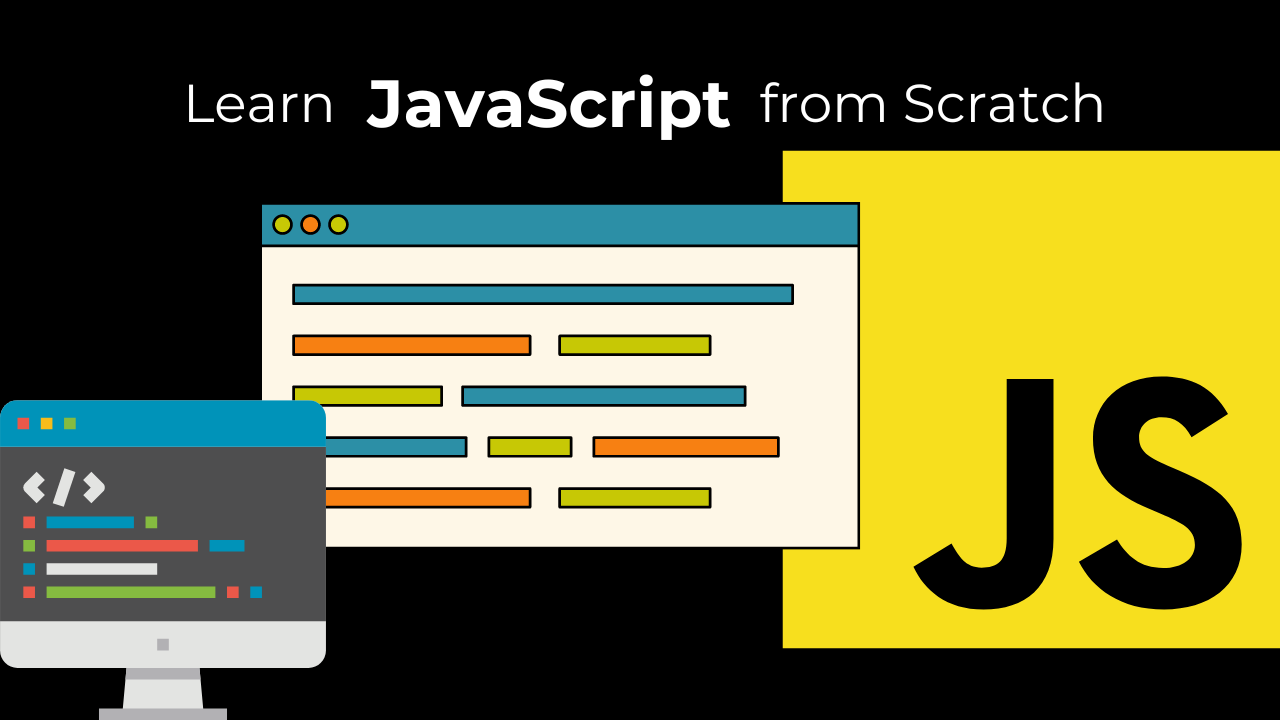
What is JavaScript?
JavaScript is a programming language that was initially created for adding interactivity and dynamic behavior to web pages.
It is a client-side scripting language, which means that the code is executed by the web browser on the user’s computer.
JavaScript is a high-level, interpreted, and dynamically typed language.
It follows the ECMAScript standard, which defines the syntax and behavior of the language.
A brief history of JavaScript:
JavaScript was created in 1995 by Brendan Eich at Netscape Communications Corporation.
Initially, it was called “LiveScript,” but it was later renamed to JavaScript due to a marketing strategy to capitalize on the popularity of Java.
Despite the name, JavaScript is not related to Java and has different syntax and semantics.
Over the years, JavaScript has evolved through various versions and editions, with the latest being ECMAScript 2023.
Use cases of JavaScript:
Web development:
JavaScript is primarily used for building interactive and dynamic websites.
It can manipulate the Document Object Model (DOM), which represents the structure of a web page, allowing developers to modify the content, styles, and behavior dynamically.
Example: Creating dropdown menus, image sliders, form validations, and pop-ups.
// Example: Simple pop-up message
alert("Hello, World!");
Server-side with Node.js:
Node.js allows developers to run JavaScript on the server side, enabling the development of server applications and APIs.
It’s commonly used for building real-time applications, API services, and tooling.
Example: Building a simple HTTP server with Node.js.
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\\n');
});
server.listen(3000, () => {
console.log('Server running at <http://localhost:3000/>');
});
Mobile app development:
With frameworks like React Native and NativeScript, developers can use JavaScript to build mobile apps for both iOS and Android platforms.
These frameworks provide a way to create native UI components using JavaScript and React.
Game development:
JavaScript can be used for building simple browser-based games.
With the introduction of WebGL and HTML5 canvas, JavaScript can also be used for creating more complex and graphically intensive games.
Setting up the development environment:
Code editor:
To write JavaScript code, you’ll need a code editor or an Integrated Development Environment (IDE).
Popular choices include Visual Studio Code, Atom, Sublime Text, and WebStorm.
Web browser:
Since JavaScript is primarily used for web development, you’ll need a modern web browser to run and test your code.
Popular choices include Google Chrome, Mozilla Firefox, Microsoft Edge, and Safari.
Browser developer tools:
Modern web browsers come with built-in developer tools, which provide a JavaScript console, debugger, and other useful utilities for web development.
Example: Opening the developer tools in Google Chrome (F12 or Ctrl+Shift+I on Windows/Linux, Cmd+Option+I on macOS).
// Example: Using the browser console
console.log("Hello, console!");
Now that you’ve gained a solid understanding of what JavaScript is, its history, and its various use cases, it’s time to dive deeper into the language itself.
In the next article, we’ll explore the building blocks of JavaScript: variables and data types, so make sure to follow along and continue your journey toward mastering this versatile language. Stay tuned, and let’s continue unraveling the mysteries of JavaScript together!
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
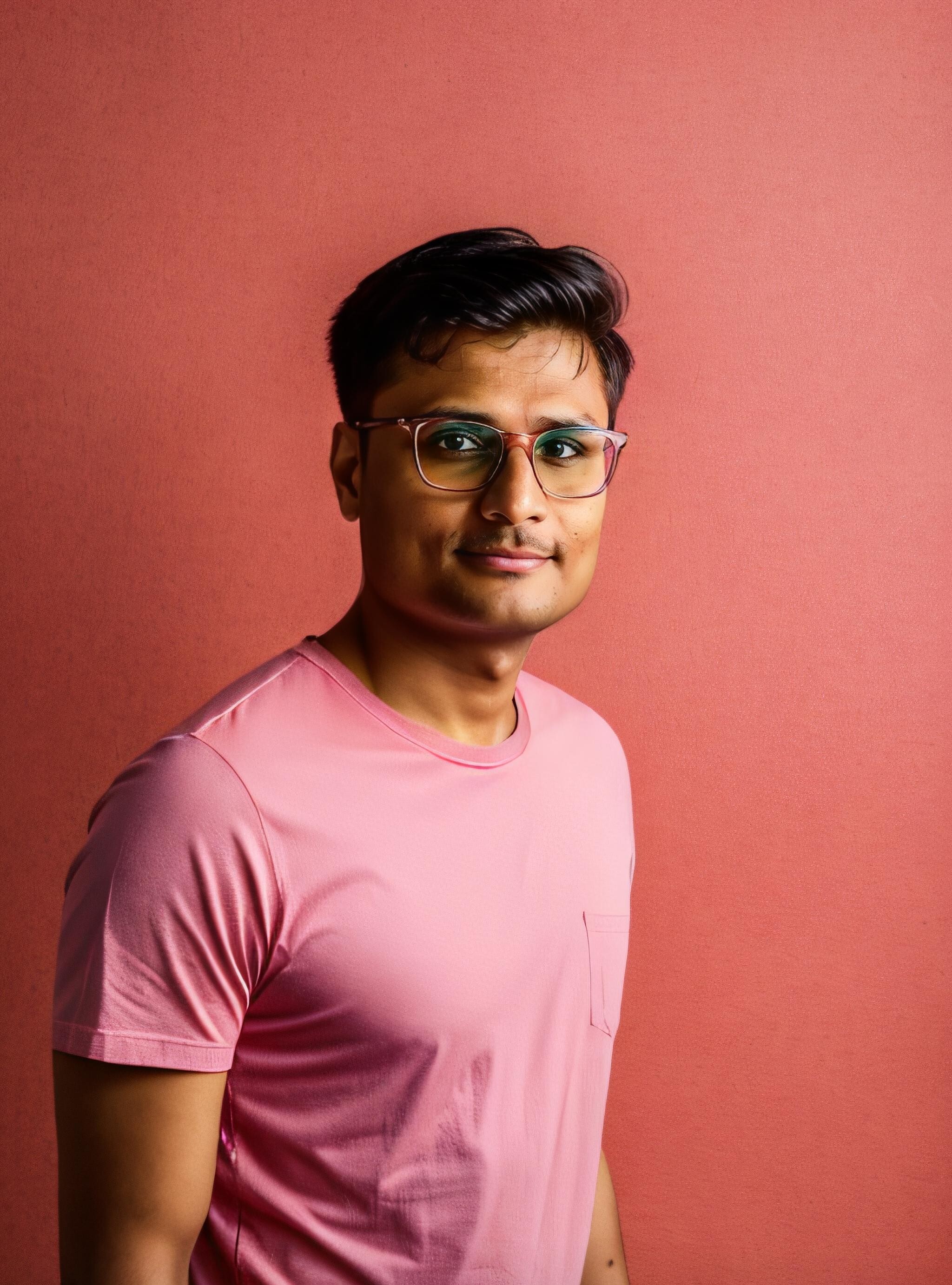