React useContext Hook in Real Life ๐ฏ
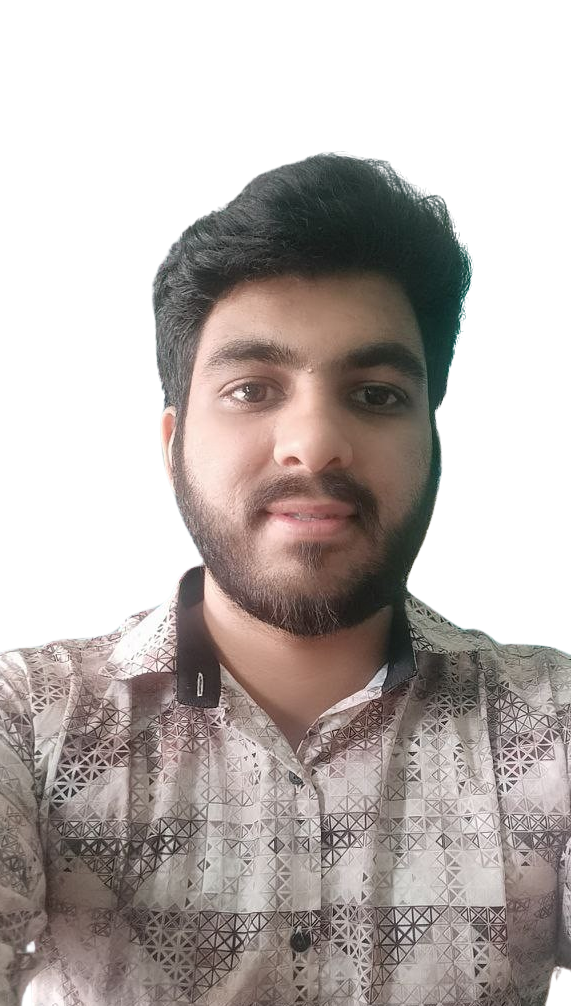
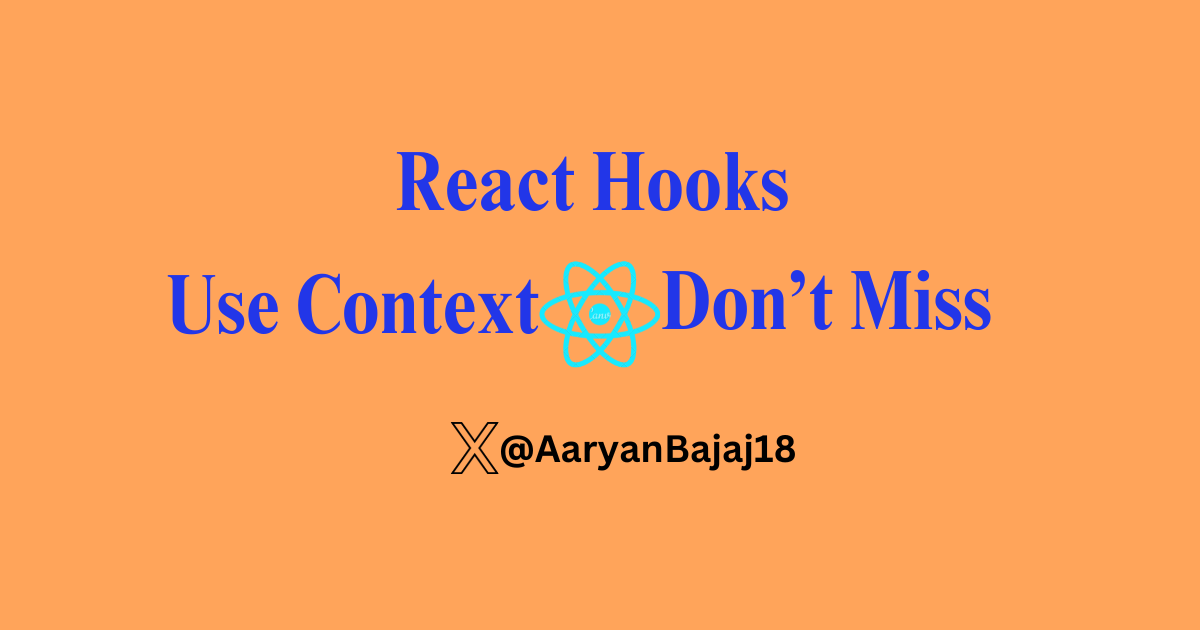
Introduction
In the bustling world of React development, state management is paramount. Ensuring seamless communication across components can be daunting, but with useContext
, it's akin to operating a paperless office: clean, efficient, and highly organized.
Understanding useContext: The Paperless Office of State Management
Imagine a paperless office where information flows smoothly without the clutter of physical documents.
Similarly,
useContext
enables your React components to share state effortlessly.It eliminates the need for prop drilling, making your code cleaner and your development process more efficient.
The Central Theme Switch: Enhancing Your App's Appearance
Consider
useContext
as the central theme switch in your application. With it, you can control the appearance of your components across the entire app.Want to implement dark mode or change the primary color scheme?
useContext
allows you to manage these settings from a single point, ensuring consistency and ease of updates.
Use useContext Wisely: The Central Water Tank Analogy
While
useContext
is powerful, it's essential to use it judiciously. Think of it as a central water tank supplying essential app-wide data, rather than a bucket carrying everything.Overloading
useContext
with too much data can lead to inefficiencies and complexity.
Instead, reserve it for crucial, global state that needs to be accessed by multiple components.
The Symphony of State Management: Combining useContext with Other Hooks
For optimal performance and scalability, combine
useContext
with other hooks likeuseReducer
or custom hooks.This combination creates a symphony of state management, allowing you to handle complex state logic with ease.
Manage themes, user settings, and more in perfect harmony.
Example: Managing Theme with useContext and useReducer
import React, { useReducer, useContext, createContext } from 'react';
const ThemeContext = createContext();
const themeReducer = (state, action) => {
switch (action.type) {
case 'LIGHT_MODE':
return { ...state, theme: 'light' };
case 'DARK_MODE':
return { ...state, theme: 'dark' };
default:
return state;
}
};
const ThemeProvider = ({ children }) => {
const [state, dispatch] = useReducer(themeReducer, { theme: 'light' });
return (
<ThemeContext.Provider value={{ state, dispatch }}>
{children}
</ThemeContext.Provider>
);
};
const ThemeToggle = () => {
const { state, dispatch } = useContext(ThemeContext);
return (
<div>
<p>Current Theme: {state.theme}</p>
<button onClick={() => dispatch({ type: 'LIGHT_MODE' })}>Light Mode</button>
<button onClick={() => dispatch({ type: 'DARK_MODE' })}>Dark Mode</button>
</div>
);
};
const App = () => (
<ThemeProvider>
<ThemeToggle />
</ThemeProvider>
);
export default App;
Conclusion
useContext
is a vital tool in your React toolkit, offering a clean and efficient way to manage global state. By combining it with other hooks, you can create robust and scalable state management solutions. Use it wisely, and your app will run smoothly like a well-oiled machine, or better yet, a paperless office.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
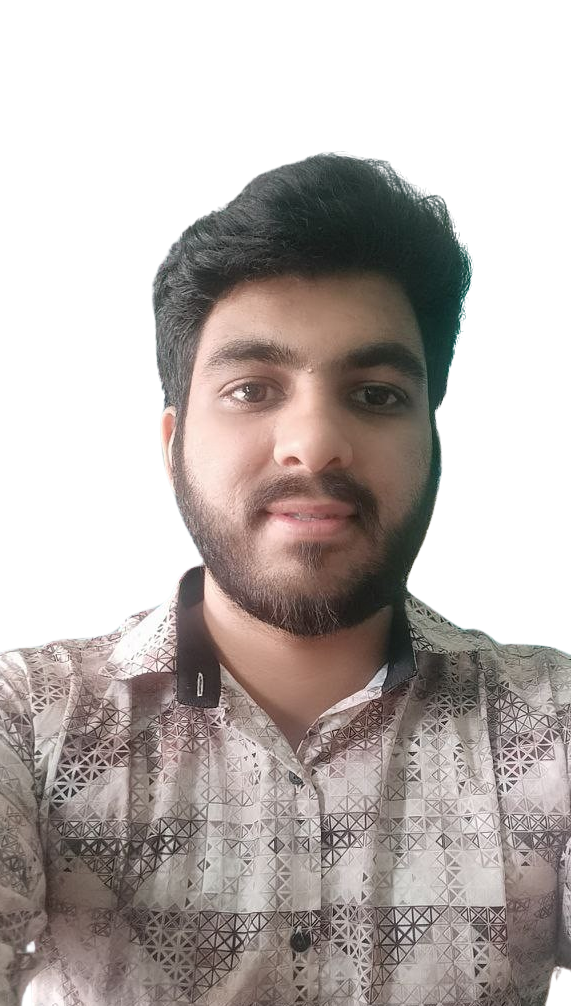
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone ๐๐ป , I'm Aaryan Bajaj , a Full Stack Web Developer from India(๐ฎ๐ณ). I share my knowledge through engaging technical blogs on Hashnode, covering web development.