Streamline User Communication with Laravel Mail and Notifications
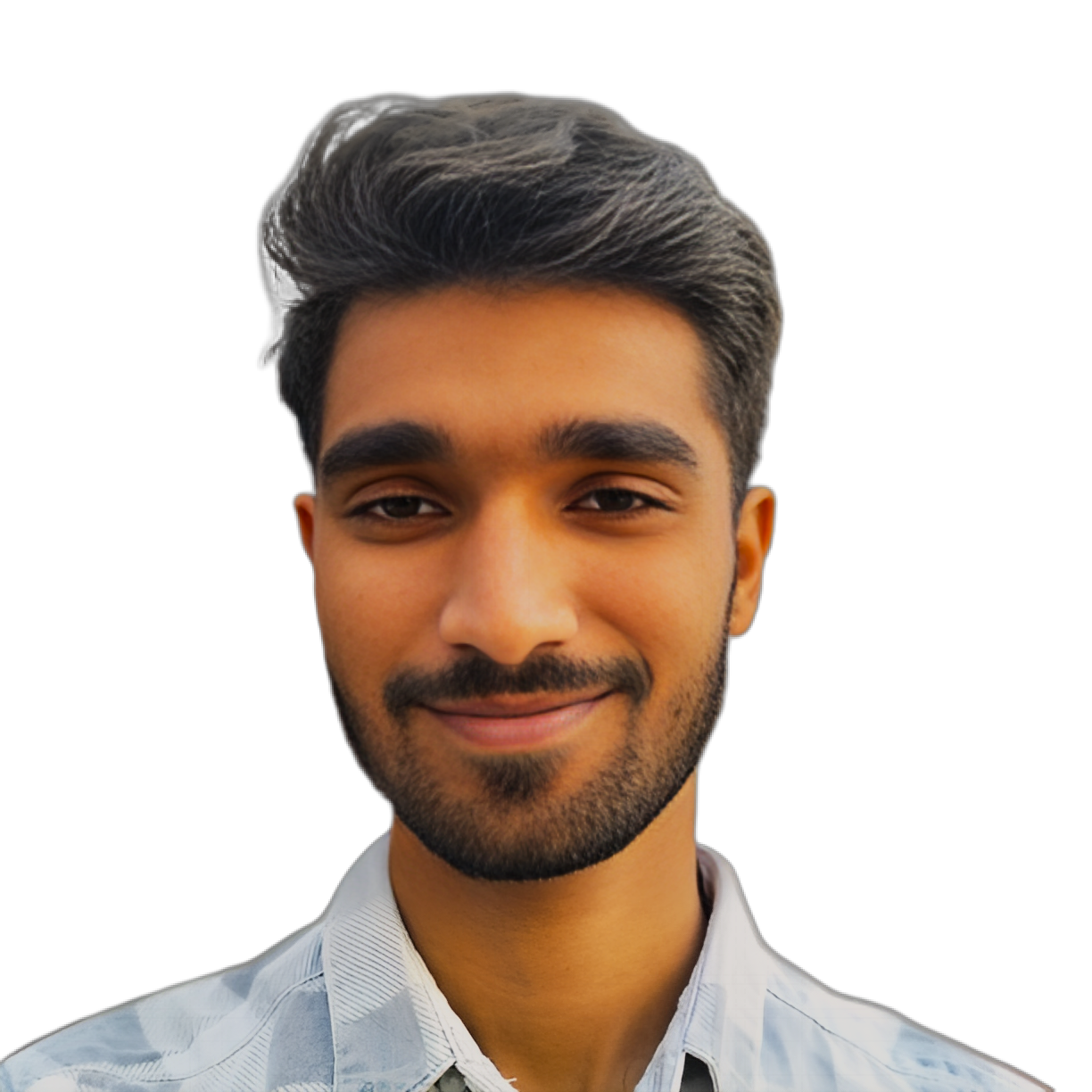
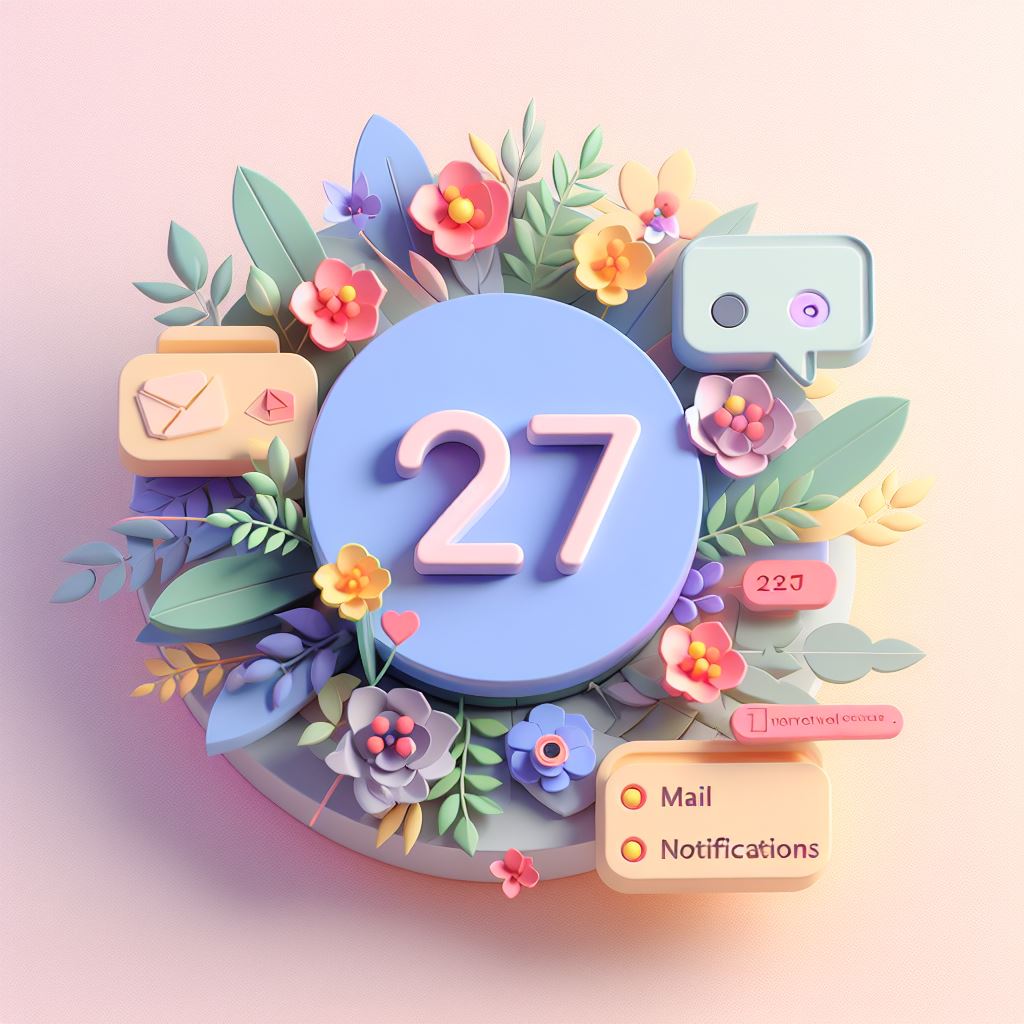
In today's digital landscape, keeping users informed and engaged is paramount. Laravel's built-in Mail and Notification system empowers you to craft a robust communication strategy for your web application. This post dives into the functionalities of Laravel Mail and Notifications, showcasing their benefits and providing a step-by-step tutorial for implementation.
The Power of Laravel Mail and Notifications
Effortless Email Sending: Compose beautiful and informative emails with ease, leveraging pre-built templates and a user-friendly API.
Multi-Channel Communication: Go beyond traditional emails and embrace a variety of notification channels (e.g., SMS, team chat platforms like Slack) to reach users where they are.
Context-Specific Delivery: Tailor notifications to specific user actions or application events, ensuring users receive relevant information at the right time.
Enhanced User Experience: Timely and personalized notifications boost user engagement and satisfaction.
Increased Developer Efficiency: Laravel's intuitive API streamlines notification development and integration within your application.
Vibrant Community Support: Benefit from the extensive Laravel community for assistance and best practices.
Getting Started: A Step-by-Step Tutorial
Project Setup:
Prerequisites: Ensure you have a Laravel project set up. If not, use
composer create-project laravel/laravel your-project-name
.Database Migration: Laravel notifications typically require a database table (
notifications
). You can generate this table usingphp artisan migrate
.
Notification Creation:
Command Line: Use the artisan command to create a new notification class:
php artisan make:notification NewUserNotification
Notification Class: This class resides in the
app/Notifications
directory and serves as the blueprint for your notification.via
Method: Override thevia
method to define the notification channels you want to use (e.g.,['mail']
for email, explore other supported channels in the documentation). You can also return an array of channels for multiple delivery methods.
PHP
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Notifications\Notification;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
class NewUserNotification extends Notification implements ShouldQueue
{
use Queueable;
public function via($notifiable)
{
return ['mail']; // Or an array of channels like ['mail', 'sms']
}
public function toMail($notifiable)
{
return (new MailMessage)
->line('Welcome to our application!')
->action('View Your Account', url('/profile'));
}
// ... other methods for additional channels (e.g., `toSlack` for Slack)
}
Sending Notifications:
notify
Method: Use thenotify
method on your user model (or any model that implements theNotifiable
trait) to send the notification:
PHP
$user = User::find(1);
$user->notify(new NewUserNotification);
Customization and Advanced Features
Email Templates: Laravel provides pre-built email templates (e.g.,
resources/views/vendor/mail/text
) that you can customize to match your application's branding.Queuing Notifications: Utilize Laravel's queue system for asynchronous notification delivery, ensuring smooth performance even with high notification volumes. Consider using the
ShouldQueue
interface on your notification class.Database Notifications: Store notifications in the database for in-app displays within your application. This allows users to revisit notifications later.
Conclusion
Laravel Mail and Notifications offer a powerful and flexible solution for crafting a user-centric communication strategy within your web application. By leveraging these features, you can keep users informed, engaged, and ultimately enhance their overall experience. Remember to explore the Laravel documentation for more in-depth details and examples: https://laravel.com/docs/11.x/notifications.
Additional Tips
Consider A/B testing different notification content and delivery channels to optimize engagement.
Implement clear unsubscribe mechanisms for users who wish to opt out of certain notifications.
Adhere to best practices regarding email deliverability to ensure your notifications reach users' inboxes.
Stay updated with the latest Laravel releases as new notification features and enhancements may be introduced.
I hope this comprehensive guide empowers you to effectively leverage Laravel Mail and Notifications to elevate your application'
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
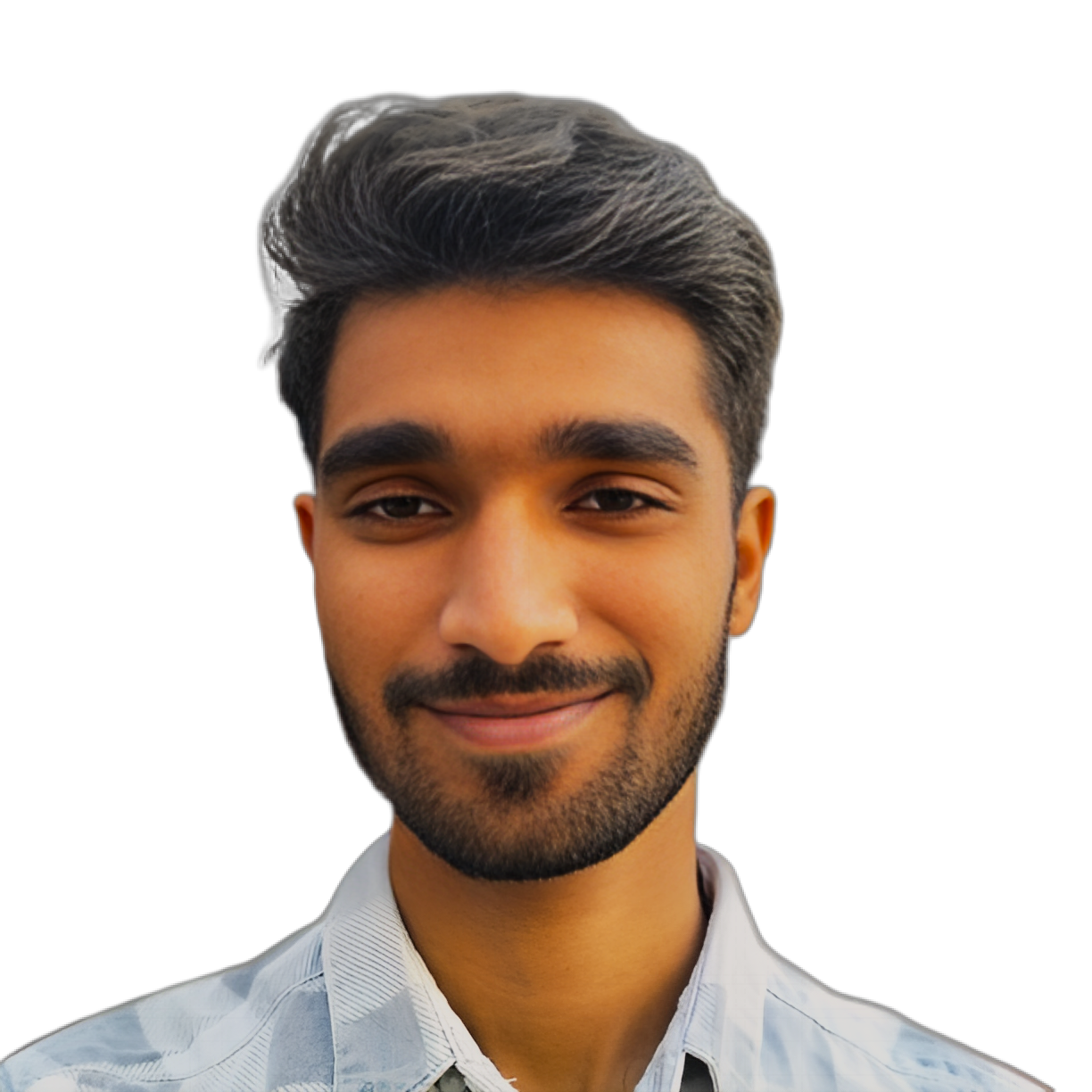
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.