Understanding UDFs in PySpark
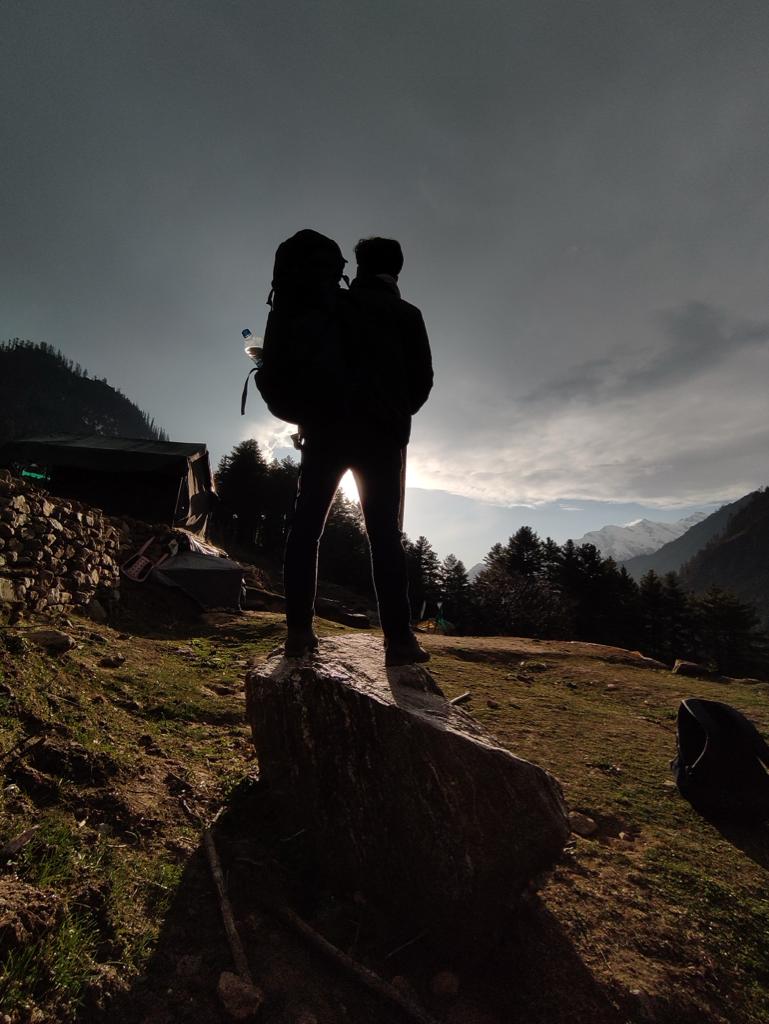
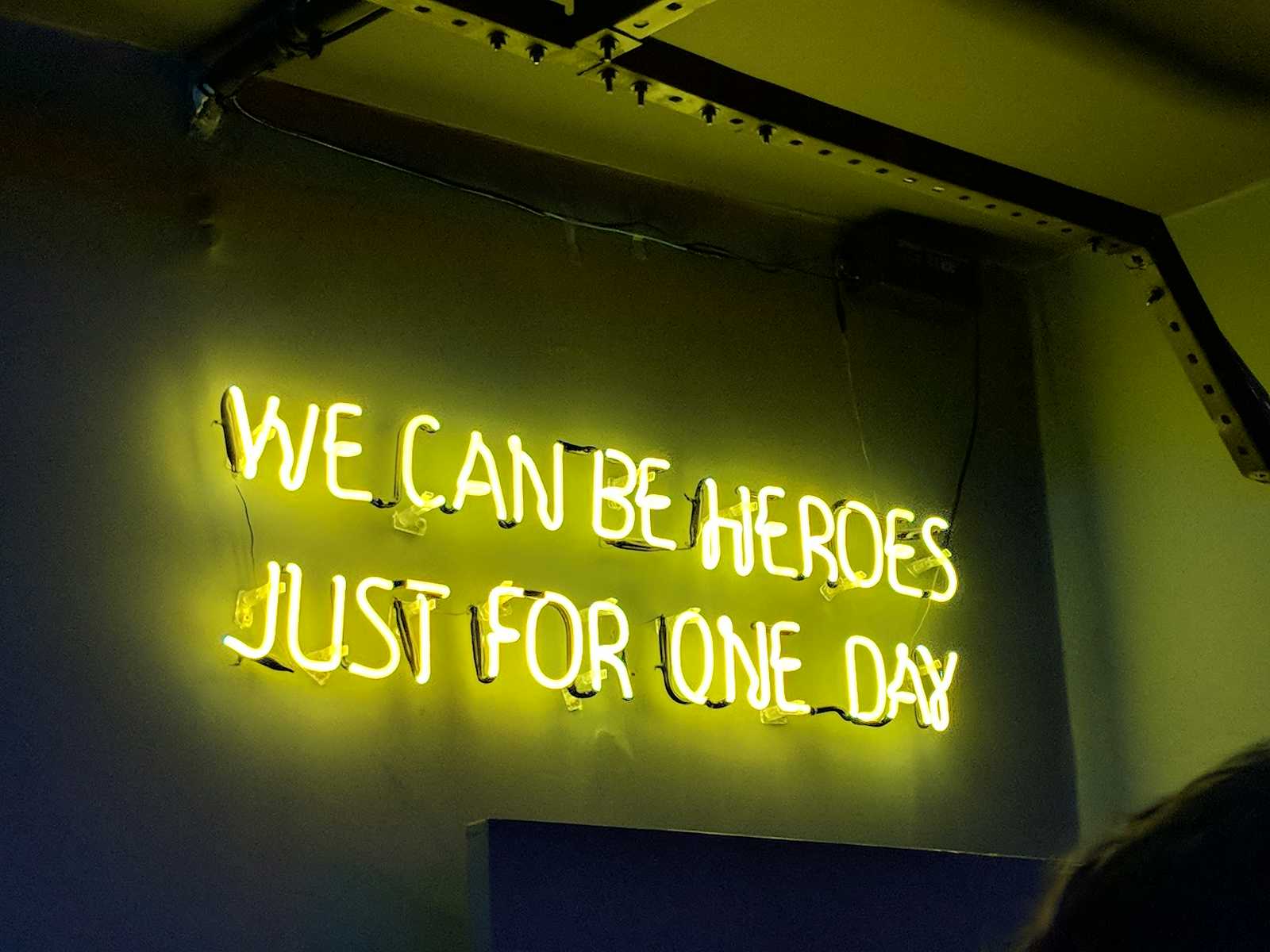
Hey Spark enthusiasts! Today, we're diving into the world of User-Defined Functions (UDFs) in PySpark. UDFs are like custom tools you can build to transform your data exactly how you need it. They're incredibly flexible, but like any powerful tool, they come with trade-offs.
So, should you completely avoid UDFs? Not necessarily! Let's break down the reasons why UDFs can sometimes slow down your Spark programs, and when they might still be your best friend.
UDFs: The Potential Speed Bump
Imagine millions of rows of data racing through your Spark pipeline. Now picture each row needing a detour to Python land for a UDF to work its magic. This data tourism between Python and Java (Spark's home turf) can create a bottleneck, slowing things down. Here's why:
Packing and Unpacking: Every row needs to be carefully packaged (serialized) before its Python trip and unpacked (deserialized) upon return. This process takes time, especially for large datasets. Imagine packing a million suitcases for a vacation โ it takes a while!
Optimizer in the Dark: Spark's built-in optimizer is a peero (pro ๐) at making your code run smoothly. But UDFs are like a black box to it. The optimizer can't see what's happening inside the UDF, so it might not be able to create the most efficient plan for your program. It's like trying to optimize a magic trick โ you can't see how it works, so it's hard to make it faster.
Scaling Struggles: Spark loves to spread tasks across your cluster for maximum speed. But UDFs, being Python-based, don't quite fit into this distributed processing party as well as Spark's native functions. It's like inviting your non-swimmer friend to a pool party โ they can't participate in all the fun.
Code Example: A Simple UDF (But Slow!)
Let's see a basic UDF example to illustrate the data movement:
from pyspark.sql.functions import udf
from pyspark.sql.types import IntegerType
# Define a Python function to square a number
def square_it(x):
return x * x
# Create a UDF specifying the output data type (IntegerType)
squared_udf = udf(square_it, IntegerType())
# Create a sample DataFrame
data = [1, 2, 3, 4]
df = spark.createDataFrame(data, "int")
# Apply the UDF to a new column named "squared"
df_squared = df.withColumn("squared", squared_udf(df.col("value")))
# Display the resulting DataFrame
df_squared.show()
In this example, even though the UDF itself is simple (squaring a number), each value in the "value" column needs to be:
Sent from Spark (Java) to Python.
Processed by the Python function.
Sent back from Python to Spark (Java).
This back-and-forth communication can become a significant bottleneck for large datasets.
UDFs: When Are They Your Hero?
Even with these slowdowns, UDFs can still be heroes in certain situations. Here's when they shine:
Unique Situations: When you need a special kind of data transformation that Spark's built-in functions can't handle, UDFs are your custom code warriors. They allow you to extend Spark's capabilities for specific tasks.
Pandas Power: If you're already using pandas for data manipulation, you can bring those pandas UDFs right into your Spark world for a smooth integration. This can be helpful when you have existing pandas code you want to leverage.
The Takeaway: UDFs Done Right
UDFs are a powerful tool, but use them strategically! Here are some tips for optimal performance and maintainable code:
Explore Alternatives: Can you achieve the same result with Spark's built-in functions or pandas UDFs? These might offer better performance.
Keep it Simple: Complex UDFs with many operations will create a bigger bottleneck than simpler ones. Break down complex logic into smaller, more efficient UDFs.
Arrow Up Your Game: If you must use Python UDFs, consider using Arrow optimization to streamline that data packing and unpacking. Arrow is a data format that can reduce serialization overhead.
Remember, the key is to find the right balance for your project. UDFs can be a valuable tool, but use them strategically for optimal performance and maintainable code. So, keep calm and UDF on, but do it wisely!
Subscribe to my newsletter
Read articles from Deepankar Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
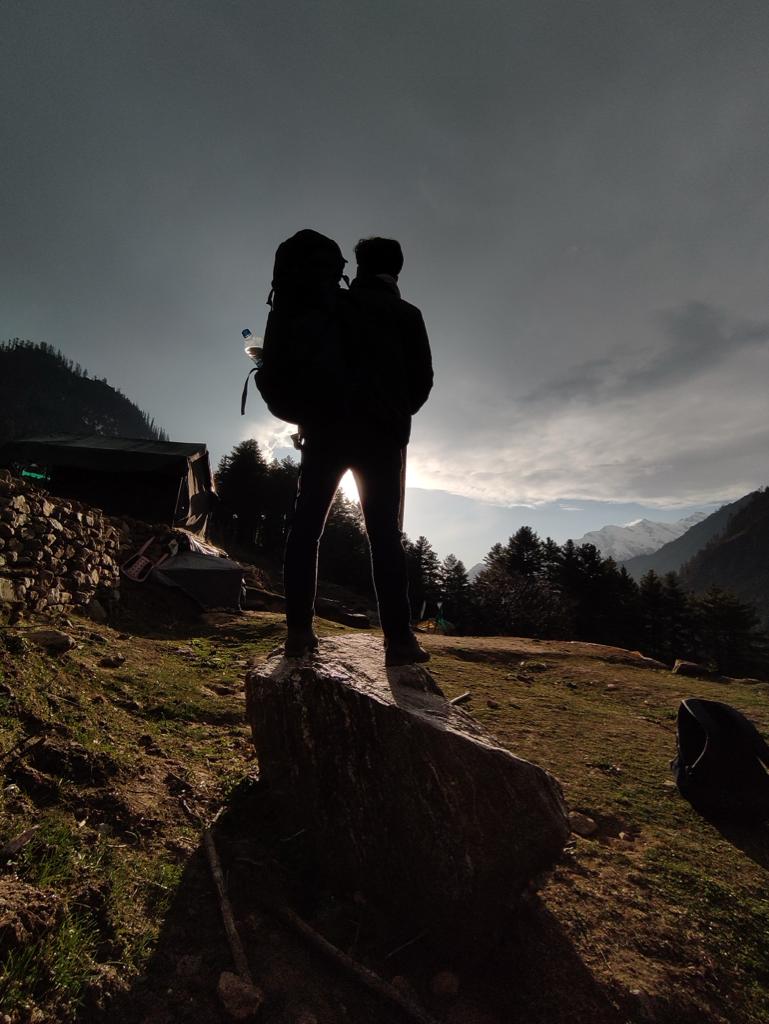
Deepankar Yadav
Deepankar Yadav
I wrangle bytes at Cognizant, making healthcare data sing. By night, I spill the beans (and code) on #dataengineering on Hashnode. Join me to conquer coding & laugh along the way! ๐