Using Python Libraries: Essential Tips
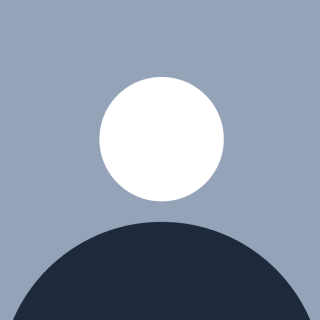

A library in Python is a reusable chunk of code that you may want to include in your programs and projects.
Libraries provide pre-written functionality, a group of modules, which saves you writing code for those functions that are already defined in libraries.
In Python, a module is a single file (or files), kind of like a book ๐, that is imported under one import
and used. It's any file that contains Python code.
Modules can define functions, classes, and variables that you can reference in other Python scripts.
A library, on the other hand, is a collection of modules (or books ๐). It's a way of packaging and distributing multiple modules together, along with other resources. Libraries provide a way of reusing code and can include functions, methods, classes, and more.
For example, the math
library in Python includes a variety of modules such as sqrt
, factorial
, cos
, sin
, etc. When you import the math
library, you have access to all these modules.
Therefore, a module is a single file, while a library is a collection of modules.
Python libraries are a collection of Python packages. A package in Python is simply a way of organizing related code. It's essentially a directory that contains multiple Python scripts. Each script is a module that can define functions, classes, or variables.
For example, the math
library in Python provides mathematical functions. So instead of writing a function to calculate the square root, you can simply import the math
library and call math.sqrt()
.
Here's how you can import and use a function from the math
library:
import math
print(math.sqrt(16)) # Outputs: 4.0
In this code, import math
is importing the math
library, and math.sqrt(16)
is calling the sqrt
function from the math
library to calculate the square root of 16.
Import: is a keyword to import modules into a python script.
The clause contains:
the
import
keyword;the name of the module or library, which is the subject to import.
The instruction may be located anywhere in your code, but it must be placed before the first use of any of the module's entities or at the top of your file. The imported libraries (or modules) can be listed individually or inline.
import math
import sys
# or inline
import math, sys
A namespace is a space (understood in a non-physical context) in which some names exist and the names don't conflict with each other (i.e., there are not two different objects of the same name).
Inside a certain namespace, each name must remain unique.
So this means that you can't create a variable named, pi
, when you are also have brought in the math
library's variable pi
- your version of it will supersede the library's version.
โ ๏ธ So be careful! โ ๏ธ
If the module of a specified name exists and is accessible (a module is in fact a Python source file), Python imports its contents, i.e., all the names defined in the module become known, but they don't enter your code's namespace.
This means that you can have your own entities named
sin
orpi
and they won't be affected by the import in any way.import math print(math.sin(math.pi/2)) # will use pi from module math (qualification) pi = 3.14 # my version of pi that will override math's version of pi print(math.sin(pi/2)) # will use the pi that I created
Best Practices
When you bring in a module or a library, you should only bring in those names that you are going to use in your code.
The nomenclature is from module_or_library import name as alias
, aliasing causes the module to be identified under a different name or shorter name so you don't have to type as much.
import module as alias
# e.g.
import math as m
print(m.sqrt(16))
# aliasing with listing
from module import name as alias
#e.g.
from math import sin as SIN, pi
If you want to see all the available names that exist in a module or a library, you can use the dir()
directory command on it.
import math
for name in dir(math):
print(name, end="\t")
# __doc__ __file__ __loader__ __name__ __package__ __spec__
# acos acosh asin asinh atan atan2 atanh ceil
# comb copysign cos cosh degrees dist e erf erfc
# exp expm1 fabs factorial floor fmod frexp fsum
# gamma gcd hypot inf isclose isfinite isinf isnan
# isqrt lcm ldexp lgamma log log10 log1p log2 modf
# nan nextafter perm pi pow prod radians remainder
# sin sinh sqrt tan tanh tau trunc ulp
For example, if you plan to only use the sin
, pi
, and e
of the math
library, you should ONLY bring in those three and NOT ALL of the names that exist in the math
library.
# listing the entities you using, and not all the things:
from math import sin, pi, e # I'm only using: sine, pi and e
print(sin(pi/2))
print(e)
But if you don't know which names you plan to use or are just want see all the names that the library provides, because you are in the middle of developing a functionality, you can use the import all of the library from module import *
.
This should only be used temporarily, though!
# WARNING: The above should only be used temporarily ,
# unless you know all the names provided by the module,
# this is UNSAFE!
from module import *
# e.g.
from math import *
Be sure to refactor your import statement once you are done! โ
Subscribe to my newsletter
Read articles from Shani Rivers directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shani Rivers
Shani Rivers
I'm a data enthusiast with web development and graphic design experience. I know how to build a website from scratch, but I sometimes work with entrepreneurs and small businesses with their Wix or Squarespace sites, so basically I help them to establish their online presence. When I'm not doing that, I'm studying and blogging about data engineering, data science or web development. Oh, and I'm wife and a mom to a wee tot.