Unveiling the Python Virtual Machine: The Magic Behind Your Code

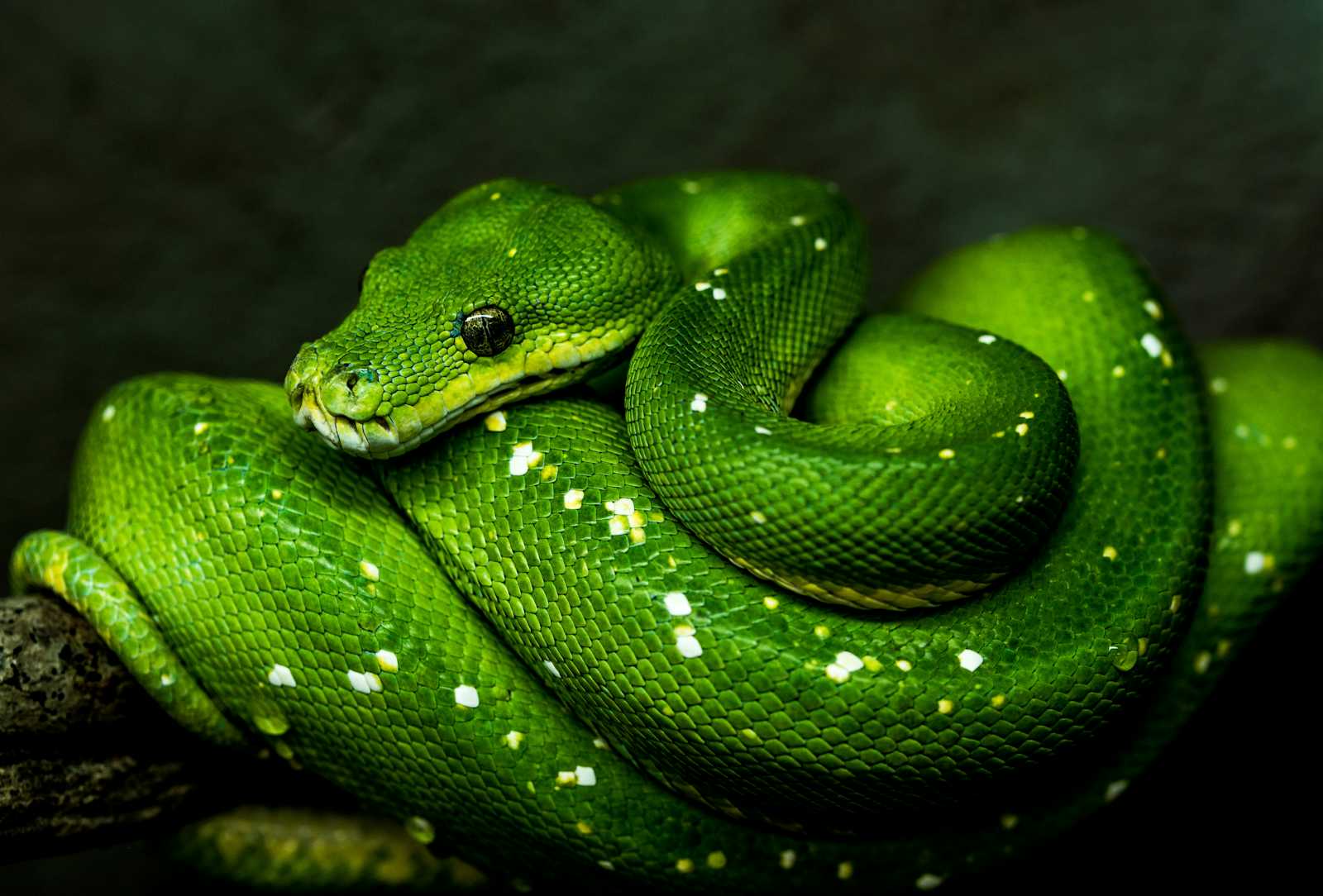
Python is a popular, high-level programming language known for its readability and versatility. Whether you're a seasoned developer or just starting out, Python offers a wide range of applications, from web development and data analysis to artificial intelligence and scientific computing. One of the key components that make Python so powerful is its Virtual Machine (VM), which plays a crucial role in executing your code efficiently. In this article, we'll explore the inner workings of the Python VM, bytecode, and how they contribute to Python's ease of use and portability.
When you install Python on your machine, you're not just getting a programming language; you're also getting a powerful tool known as the Python Virtual Machine (VM). This VM is the engine that drives your Python programs, translating the bytecode generated by the Python interpreter into machine code that your computer's hardware can understand and execute. This isn't just technical jargon—it's the magic that makes Python so versatile and easy to use. But have you ever wondered what really goes on behind the scenes when you run a Python script? Let's dive into the fascinating world of Python bytecode and the Python VM to uncover the secrets that make your code come to life.
Python Bytecode and Portability
If you've ever written or used Python, you're probably familiar with Python source code files ending in .py
. You may have also seen files ending in .pyc
, known as Python "bytecode" files. These files are now stored in a subdirectory called __pycache__
in Python 3. Bytecode files prevent Python from re-parsing your source code every time it runs, saving time.
This process ensures that your Python code can run on any machine with the Python VM installed, making Python highly portable.
The VM handles low-level execution details, such as memory management and garbage collection, allowing you to focus on writing your code.
The Python VM provides a layer of abstraction between your code and the hardware, enhancing security and stability.
It also allows for dynamic typing and automatic memory management, simplifying development.
But beyond "Oh, that's Python bytecode," do you know what's in those files and how Python uses them?
I'm excited to take you through what Python bytecode is, how Python uses it to execute your code, and how knowing about it can help you. Let's dive in!
How Python Works
Python is known as an interpreted language, which means your source code gets translated into native CPU instructions while the program runs. But there's more to it! Like many interpreted languages, Python compiles your source code into instructions for a virtual machine. The Python interpreter runs this virtual machine, and this intermediate format is called "bytecode."
So, those .pyc
files that Python creates aren't just a "faster" or "optimized" version of your source code; they're the bytecode instructions that Python's virtual machine runs when your program executes.
Bytecode optimization affects real-world performance by reducing the time required for code execution.
When Python source code is compiled to bytecode, it eliminates the need for the interpreter to parse and analyze the code every time it runs, thereby speeding up execution.
Optimized bytecode can also reduce memory usage and improve cache efficiency, leading to faster load times and reduced latency in applications.
This is particularly beneficial in large-scale systems and applications with high-performance requirements, where even small improvements can lead to significant gains in overall efficiency.
Additionally, optimized bytecode can help in better resource management, enabling smoother and more responsive application behavior.
Examples of Bytecode
Here's a simple "Hello, World!" program in Python:
print("Hello, World!")
When compiled to bytecode, it looks like this (using Python 3.9 as an example):
1 0 LOAD_NAME 0 (print)
2 LOAD_CONST 0 ('Hello, World!')
4 CALL_FUNCTION 1
6 POP_TOP
8 LOAD_CONST 1 (None)
10 RETURN_VALUE
Another example, let's consider a function that adds two numbers:
def add(a, b):
return a + b
The bytecode for this function might look like:
1 0 LOAD_FAST 0 (a)
2 LOAD_FAST 1 (b)
4 BINARY_ADD
6 RETURN_VALUE
When compiled to bytecode, it looks like this (using Python 3.12 as an example):
If you write the add()
function and use the CPython interpreter to run it, Python will execute the bytecode shown above. It might seem strange at first, so let's break it down and understand what's happening.
Frozen Binaries
Frozen bytecode files are fixed and do not change once generated unless the source code itself is modified. This "freezes" the code into a specific state that remains constant until updated. While not traditional binary executables like those produced by languages such as C or C++, Python bytecode files are still considered binaries because they contain machine-level instructions for the Python Virtual Machine (VM). These instructions, though not human-readable, are essential for execution by the VM.
Bytecode files enhance execution speed, and Python uses a diffing algorithm based on timestamps to determine when to regenerate bytecode.
Bytecode files change when source files are modified, and multiple versions of bytecode files in the program directory ensure compatibility with different Python interpreters.
Different Python interpreters handle bytecode in unique ways.
Different Python Interpreters
CPython, the standard Python interpreter, compiles Python source code into bytecode and executes it on the Python Virtual Machine (PVM).
PyPy, an alternative interpreter, also compiles Python code to bytecode but includes a Just-In-Time (JIT) compiler to optimize execution speed by translating bytecode to machine code at runtime.
Other interpreters like Jython and IronPython compile Python code to bytecode compatible with the Java Virtual Machine (JVM) and the .NET framework, respectively, offering interoperability with those ecosystems.
Python Virtual Machine
PVM stands for Python Virtual Machine. It's the component of the Python runtime environment that executes Python bytecode. When you write Python code, it's first compiled into bytecode (a low-level representation of your code), which is then executed by the Python Virtual Machine.
Loading the Code: When the Python interpreter starts, it loads the Python source code file or bytecode file into memory.
Parsing (for Source Code): If the file is a Python source code file (
.py
), the interpreter first parses the source code to generate the corresponding bytecode.Execution: Once the code is loaded into memory and, if necessary, compiled into bytecode, the PVM starts executing the instructions. It enters a loop where it iterates through the bytecode instructions one by one, executing each instruction.
Continued Execution: The PVM continues this loop until it reaches the end of the bytecode or encounters a termination condition, such as a return statement or an unhandled exception.
Handling External Events: During execution, the PVM may also interact with the external environment, such as reading from files, writing to the console, or handling user input.
Exiting: Once the execution is complete or an error occurs that cannot be handled, the Python interpreter exits.
In summary, understanding Python bytecode and the Python Virtual Machine (PVM) can greatly enhance your efficiency and effectiveness as a developer. Bytecode ensures faster execution and portability across different systems, while the PVM handles the intricate details of execution, memory management, and security. By grasping these concepts, you can write more optimized and robust Python code, making the most of this powerful and versatile language.
References and Further Reading
Subscribe to my newsletter
Read articles from Md. Afzal Hassan Ehsani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Md. Afzal Hassan Ehsani
Md. Afzal Hassan Ehsani
I am a student who loves programming. 🌱 Exploring Devops. 🌱I spent time on learning cool stuffs.