Table Locking Using Spring: A Comprehensive Guide
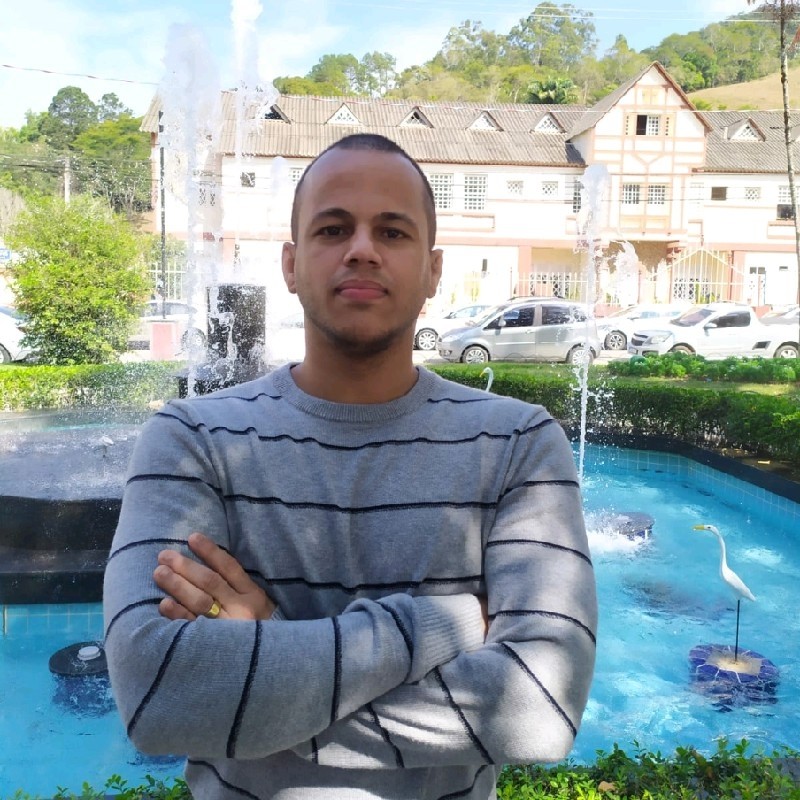
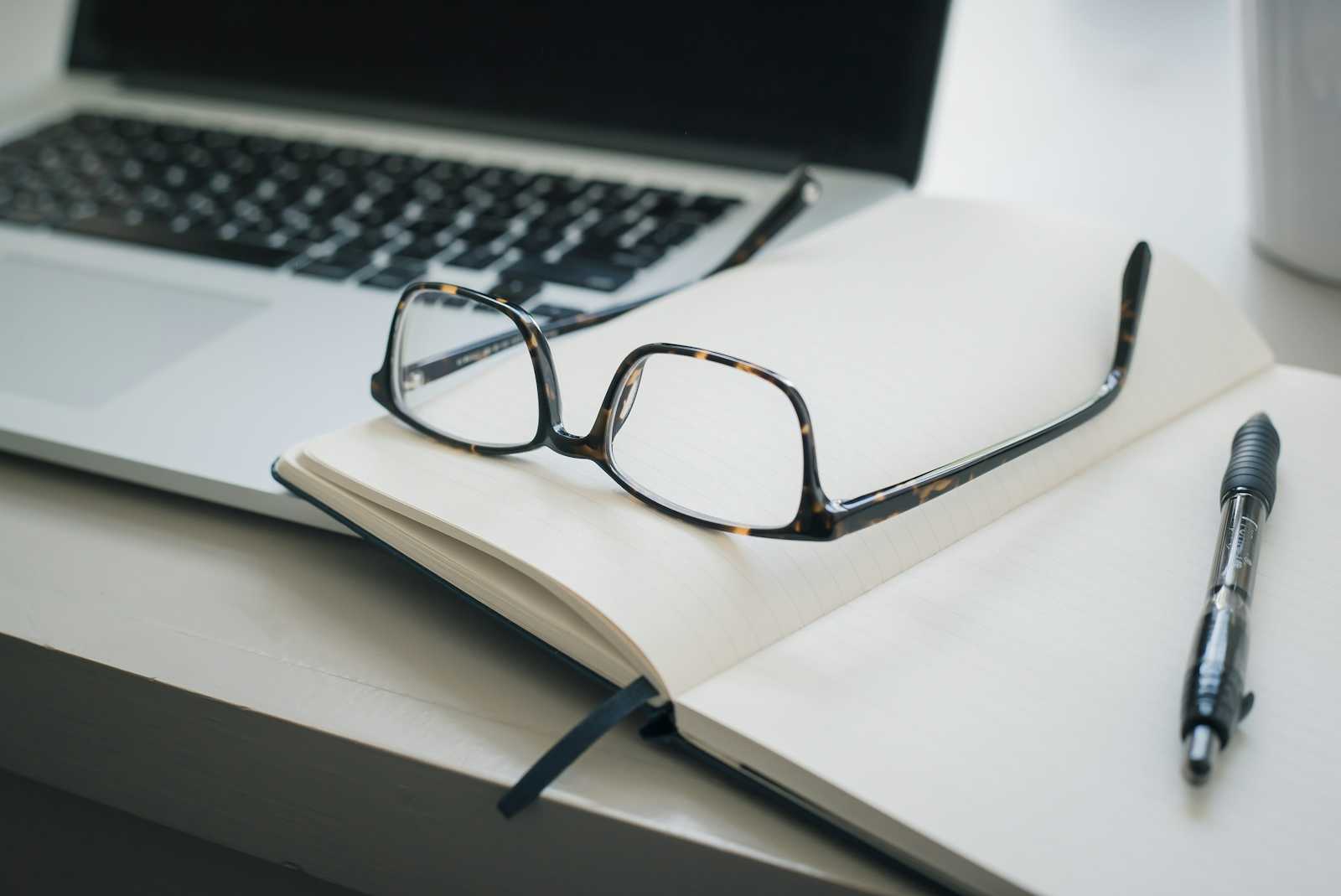
Introduction
In applications that use databases, it is common to need to control concurrent access to data to avoid inconsistencies and ensure integrity. Table locks are an essential technique to achieve this goal. In this article, we will explore what table locks are, why we should use them, their benefits and drawbacks, and how to implement them using the Spring framework, with a special focus on pessimistic and optimistic locks.
What is a Table Lock?
A table lock is a synchronization mechanism used to control simultaneous access to data in a database table. When a table is locked, read and/or write operations are restricted to avoid conflicts and ensure data consistency. There are two main types of locks:
Shared Lock (Read Lock): Allows multiple transactions to read the table simultaneously but blocks write operations.
Exclusive Lock (Write Lock): Allows only one transaction to access the table for reading or writing, blocking all other transactions.
Why Use Table Locks?
Table locks are essential to maintain the integrity and consistency of data in scenarios of concurrent access. Here are some reasons to use locks:
Ensure Data Consistency: Prevent reading partially modified data.
Prevent Race Conditions: Avoid two transactions modifying the same data simultaneously.
Maintain Data Integrity: Ensure write operations are completed without interruptions.
Benefits of Table Locks
Data Consistency:
Ensures that the data read by a transaction is consistent and not in an intermediate state of modification by another transaction.
Example: In an e-commerce application, during stock updates, a lock ensures that the number of available items is not read incorrectly while being updated.
Transactional Integrity:
Ensures that transactions are executed atomically, where all operations are completed successfully or none are applied.
Example: In a banking system, a table lock can ensure that fund transfers between accounts are executed correctly without interference.
Deadlock Prevention:
Using appropriate locks can minimize the occurrence of deadlocks, where two or more transactions are stuck waiting for each other.
Example: In a reservation system, the use of locks can prevent two users from reserving the same seat simultaneously.
Drawbacks of Table Locks
Reduced Performance:
Locks can decrease system performance as they block access to data, causing other transactions to wait until the lock is released.
Example: In a high-concurrency system, excessive use of locks can slow down read and write operations.
Potential for Deadlocks:
If not managed correctly, locks can lead to deadlocks, where two or more transactions are stuck waiting for each other.
Example: Two processes trying to access two resources in reverse order can cause a deadlock if both apply exclusive locks.
Complexity in Implementation:
Implementing and managing locks can add complexity to the code and make maintenance difficult.
Example: Keeping the locking logic correct in a distributed system can be challenging and error-prone.
Types of Locks: Pessimistic vs. Optimistic
There are two main approaches to implementing locks in databases: pessimistic and optimistic. Let's explore each of them in detail.
Pessimistic Locking
Pessimistic locking assumes that conflicts over data access are common and thus locks the data as soon as a transaction starts operating on it. This prevents other transactions from accessing the same data until the lock is released.
When to use:
High-contention scenarios, where multiple transactions frequently try to access the same data.
Critical applications where data integrity is of utmost importance.
Advantages:
Ensures data consistency during the transaction.
Prevents race conditions, ensuring that one transaction finishes before another begins.
Disadvantages:
This can lead to performance issues as other transactions are blocked until the lock is released.
Potential for deadlocks if not managed correctly.
Implementation Example:
import javax.persistence.LockModeType;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class ProductService {
@PersistenceContext
private EntityManager entityManager;
@Transactional
public void updateProductStock(Long productId, int quantity) {
Product product = entityManager.find(Product.class, productId,
LockModeType.PESSIMISTIC_WRITE);
product.setStock(product.getStock() - quantity);
entityManager.persist(product);
}
}
Optimistic Locking
Optimistic locking assumes that conflicts are rare and thus allows multiple transactions to read and modify the data simultaneously. Conflict checking is done only at the update time. If a conflict is detected, the transaction is aborted and can be retried.
When to use:
Low-contention scenarios, where it is rare for multiple transactions to access the same data simultaneously.
Applications where performance is more critical and data can be easily reprocessed in case of conflict.
Advantages:
Better performance in low-contention environments.
Fewer locks, allowing greater concurrency.
Disadvantages:
This can lead to transaction reprocessing in case of conflicts.
Less suitable for high-contention scenarios.
Implementation Example:
import org.springframework.dao.OptimisticLockingFailureException;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class ProductService {
@Transactional
public void updateProductStock(Product product, int quantity) {
try {
product.setStock(product.getStock() - quantity);
entityManager.merge(product);
} catch (OptimisticLockingFailureException e) {
// Handle optimistic locking exception
}
}
}
Conclusion
Table locking is a fundamental technique for ensuring data consistency and integrity in applications that involve concurrent access to database tables. Pessimistic locking is suitable for high-contention scenarios, providing robust data consistency and preventing race conditions, though it may lead to performance bottlenecks and potential deadlocks. Optimistic locking, on the other hand, excels in low-contention environments, offering better performance and greater concurrency, albeit at the risk of transaction reprocessing in case of conflicts.
By leveraging the capabilities of the Spring framework, developers can implement both pessimistic and optimistic locking mechanisms effectively, tailoring their approach to the specific needs of their applications. Whether ensuring secure financial transactions or maintaining data synchronization in distributed systems, understanding and utilizing table locks is crucial for building reliable, high-performance applications that handle concurrent data access with precision and efficiency.
Subscribe to my newsletter
Read articles from André Felipe Costa Bento directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
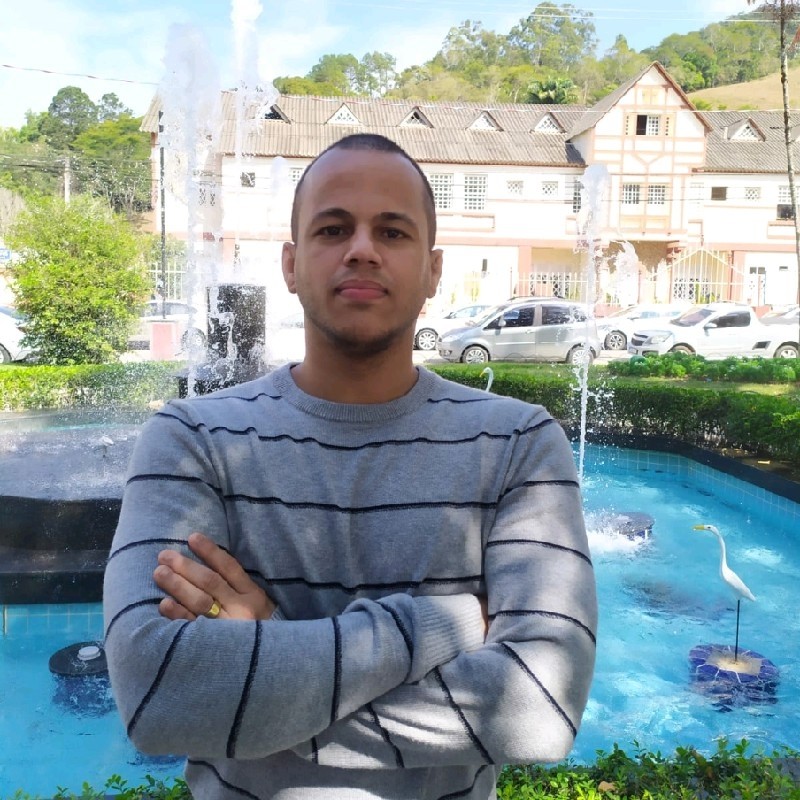
André Felipe Costa Bento
André Felipe Costa Bento
Fullstack Software Engineer.