Unleash the Power of Laravel Database Seeding: Streamline Your Development Workflow
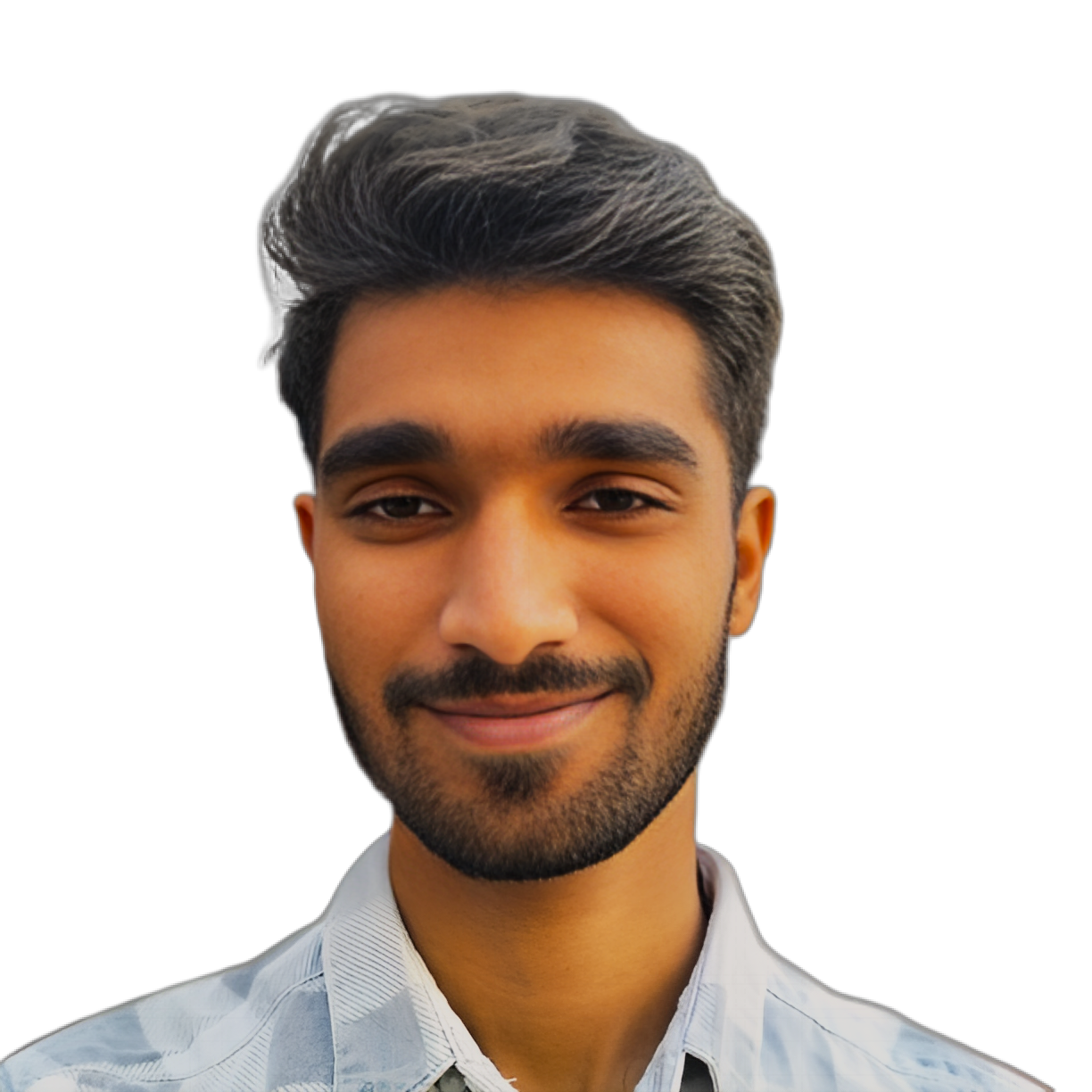
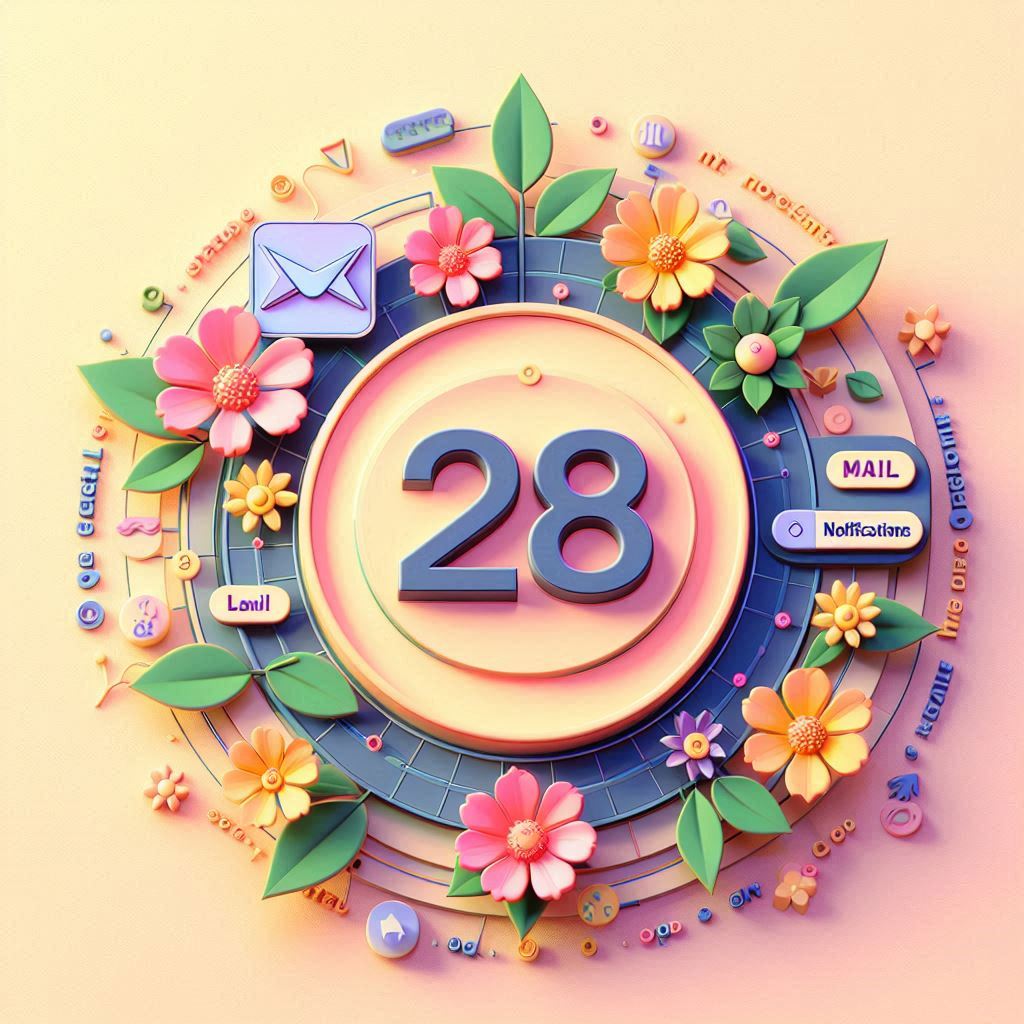
Introduction:
Have you ever found yourself drowning in manual data entry while setting up your Laravel development environment? Do you yearn for a more efficient and streamlined way to populate your database with test data for development and testing purposes? Look no further than Laravel Database Seeding! This powerful feature empowers you to establish a consistent initial state for your database, ensuring your application functions flawlessly with realistic data.
What is Laravel Database Seeding?
Laravel Database Seeding provides a built-in mechanism to effortlessly generate test data within your database using seed classes. These classes define the specific data you want to insert into your tables, offering a robust solution to:
Expedite Development: Ditch the tedious process of manually entering test data, saving you valuable time and effort.
Maintain Consistency: Guarantee your application interacts with the same initial database state throughout development and testing, fostering reliable results.
Boost Code Maintainability: Separate test data generation logic from your application code, promoting clean and organized codebases.
Enhance Collaboration: Facilitate seamless teamwork by sharing a standardized development environment with consistent test data.
How Does Seeding Work in Laravel?
The seeding process in Laravel follows a straightforward approach:
Seed Class Creation: Begin by creating seed classes that extend the
Seeder
base class provided by Laravel.run
Method Definition: Within your seed class, define therun
method. This method houses the logic responsible for inserting data into your database tables.Data Insertion Techniques: Leverage various techniques to insert data:
Laravel Query Builder: While functional, this approach might require more manual coding.
Eloquent Model Factories (Recommended): The recommended approach, model factories offer a more concise and expressive way to generate realistic test data by mimicking your application's models.
Tutorial: Seeding Your Laravel Database
Let's embark on a hands-on tutorial to experience the magic of Laravel Database Seeding in action:
Project Setup:
Ensure you have a Laravel project established. If not, you can create one using Laravel's installer:
laravel new my-laravel-project
Navigate to your project's root directory using your terminal:
cd my-laravel-project
Generate Seed Class:
Employ Laravel's Artisan command to generate a seed class:
php artisan make:seeder UsersTableSeeder
This command creates a
UsersTableSeeder.php
file within yourdatabase/seeds
directory.
Define Seeding Logic:
Open the
UsersTableSeeder.php
file and modify itsrun
method to utilize Eloquent model factories for data generation:<?php namespace Database\Seeders; use Illuminate\Database\Seeder; use App\Models\User; // Import your User model class UsersTableSeeder extends Seeder { public function run() { User::factory()->count(10)->create(); // Create 10 users using the User factory } }
Run Seeding Command:
Execute the following Artisan command to execute the seeding process:
php artisan db:seed
This command instructs Laravel to locate and execute all seed classes within the
database/seeds
directory.
Explanation:
In this example, we've created a UsersTableSeeder
class that leverages the User
model factory (assuming you have one defined for your User
model) to generate 10 user records within your database.
Additional Considerations:
DatabaseSeeder Class: Laravel provides a
DatabaseSeeder
class located atdatabase/seeds/DatabaseSeeder.php
. This class serves as an entry point for seeding your database. You can modify it to control the execution order of your seed classes using thecall
method.Custom Seeding Logic: While model factories provide a convenient approach, you might encounter scenarios where you require custom logic for data generation. Feel free to tailor the
run
method to suit your specific needs.
Conclusion:
By embracing Laravel Database Seeding, you'll significantly enhance your development workflow. You'll save precious time, ensure consistent test data, maintain clean code, and streamline collaboration. So, the next time you encounter the need to populate your Laravel database for development.
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
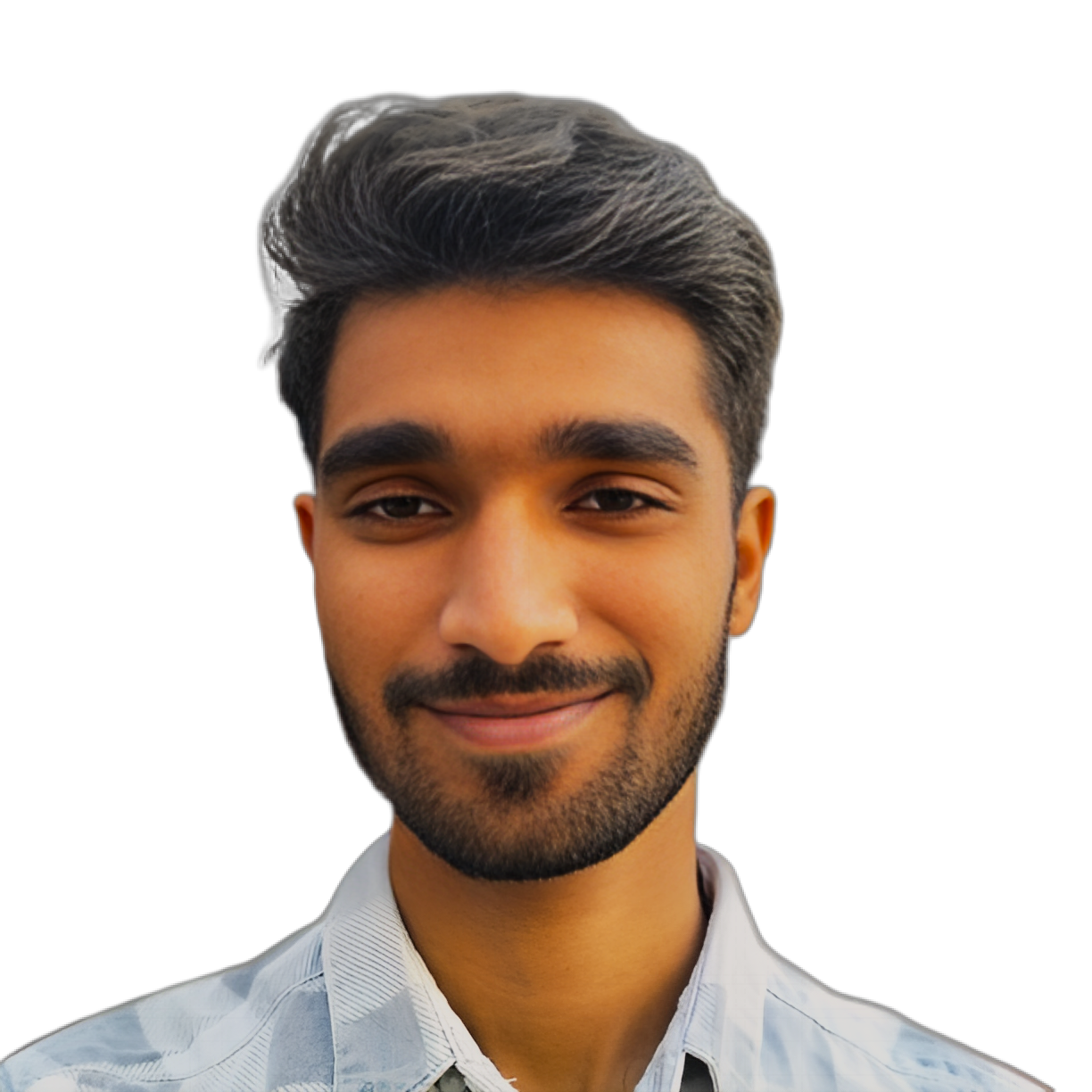
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.