Understanding Database Keys

Table of contents
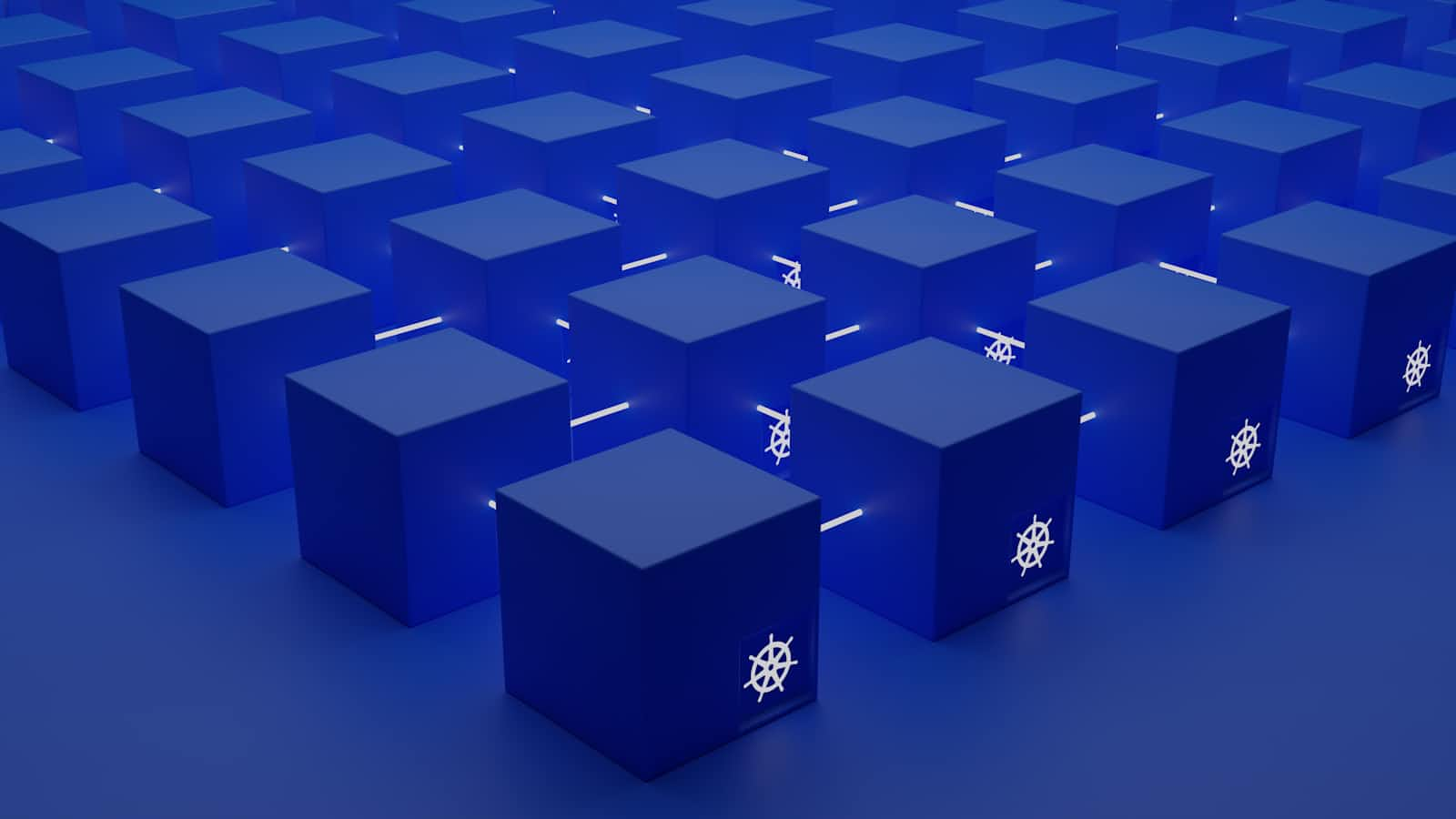
This is a Series of Article to start the series visit here.
In Laravel, database keys play a crucial role in defining relationships between different tables and ensuring data integrity. Keys help maintain the integrity and efficiency of the database by enforcing uniqueness and establishing relationships between tables. In this article, we'll explore the different types of keys used in Laravel migrations and how they are implemented. In this article we will use Laravel 11.
Primary Key
A primary key uniquely identifies each record in a table. In Laravel migrations, you can define a primary key using the increments
or bigIncrements
method. Here's an example:
Schema::create('users', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email')->unique();
$table->timestamps();
});
In this example, the id
column is defined as the primary key using the bigIncrements
method, which generates a big integer auto-incrementing ID.
Foreign Key
Foreign keys establish relationships between tables by referencing the primary key of another table. In Laravel migrations, you can define a foreign key using the foreign
method. Here's an example of creating a foreign key in a migration:
Schema::table('posts', function (Blueprint $table) {
$table->id();
$table->foreignId('user_id')->constrained()->onDelete('cascade');
$table->string('title');
$table->string('slug')->unique();
$table->text('content')->nullable();
$table->timestamps();
});
In this example, we're adding a user_id
column to the posts
table, which references the id
column of the users
table. The foreign
method establishes this relationship.
Unique Key
A unique key ensures that the values in a column are unique across all records in the table. In Laravel migrations, you can define a unique key using the unique
method. Here's an example:
Schema::create('roles', function (Blueprint $table) {
$table->id();
$table->string('name')->unique();
$table->timestamps();
});
In this example, the name
column is defined as unique using the unique
method. This ensures that each role name is unique within the roles
table.
Composite Key
A composite key consists of multiple columns that, together, uniquely identify each record in a table. In Laravel migrations, you can define a composite key using the primary
method with an array of column names. Here's an example:
Schema::create('orders', function (Blueprint $table) {
$table->foreignId('user_id')->constrained()->onDelete('cascade');
$table->foreignId('product_id')->constrained()->onDelete('cascade');
$table->primary(['customer_id', 'product_id']);
});
In this example, the composite key consists of the customer_id
and product_id
columns, which together uniquely identify each order. We use the primary
method to define this composite key.
Conclusion
Understanding and properly utilizing database keys is essential for designing efficient and maintainable database schemas in Laravel applications. Whether it's defining primary keys for uniqueness, foreign keys for relationships, unique keys for data integrity, or composite keys for complex relationships, Laravel provides powerful tools for managing database keys effectively.
By leveraging these features, Laravel developers can ensure the integrity and efficiency of their database structures, leading to robust and scalable applications.
Subscribe to my newsletter
Read articles from Saiful Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saiful Alam
Saiful Alam
An Expert software engineer in Laravel and React. Creates robust backends and seamless user interfaces. Committed to clean code and efficient project delivery, In-demand for delivering excellent user experiences.