React useCallback Hook in Real Lifeđź’Ż
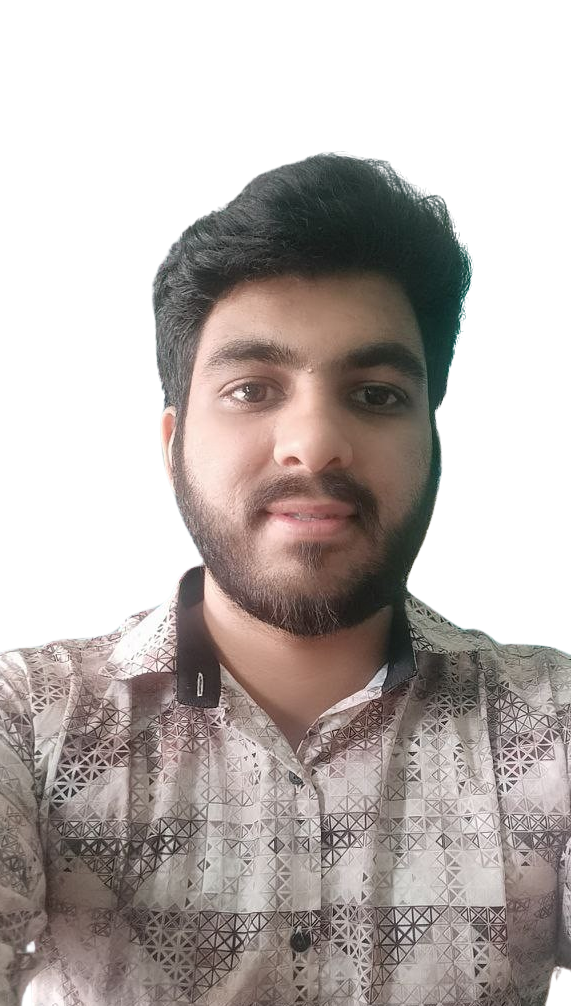
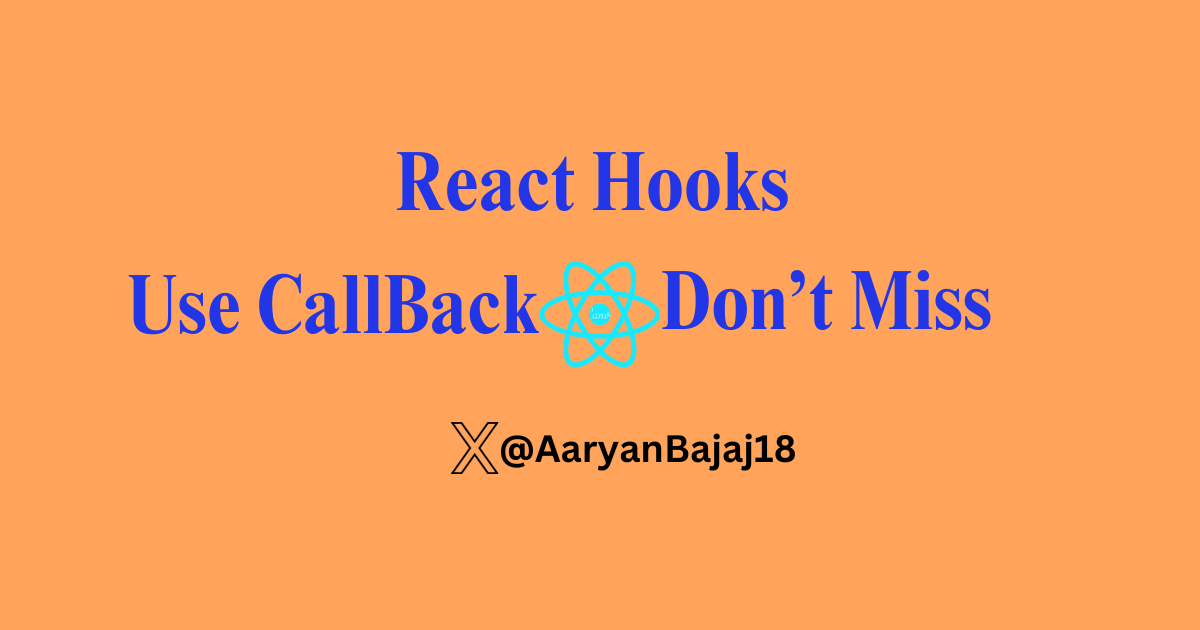
In the dynamic world of React development, optimizing performance is crucial for creating efficient and responsive applications. One powerful tool in the React developer’s toolkit is the useCallback
hook.
Stop Rerunning Functions with useCallback
Imagine a sprinter conserving energy for the final lap instead of wasting it on unnecessary movements.
That's what
useCallback
does for your React functions.It ensures that functions only execute when they actually change, preventing unnecessary reruns and conserving your app’s performance.
Controlling Re-Renders with useCallback
Think of
useCallback
as a control panel button that only lights up when settings change.This hook prevents needless function recreation, which can be a common source of performance bottlenecks in React applications.
By using
useCallback
, you can manage your re-renders more efficiently, ensuring that your app remains responsive and smooth.
Fine-Tuning Components with useCallback
Using
useCallback
for callback reference equality is like fine-tuning a high-performance engine.It ensures that the references to your callback functions remain consistent unless their dependencies change.
When combined with
useMemo
, you achieve peak performance, much like optimizing every part of an engine to run seamlessly.
import React, { useState, useCallback, useMemo } from 'react';
const ExpensiveComponent = React.memo(({ compute, count }) => {
return <div>Computed Value: {compute(count)}</div>;
});
const App = () => {
const [count, setCount] = useState(0);
const [text, setText] = useState('');
const compute = useCallback((num) => {
// Simulate an expensive computation
let result = 0;
for (let i = 0; i < 1000000000; i++) {
result += num;
}
return result;
}, []);
const memoizedValue = useMemo(() => compute(count), [compute, count]);
return (
<div>
<input value={text} onChange={(e) => setText(e.target.value)} />
<button onClick={() => setCount(count + 1)}>Increment</button>
<ExpensiveComponent compute={compute} count={count} />
<div>Memoized Value: {memoizedValue}</div>
</div>
);
};
export default App;
In this example, useCallback
ensures that the compute
function is only recreated when its dependencies change, while useMemo
optimizes the computation result caching.
Balancing Performance with useCallback
While
useCallback
is a powerful tool, it's not a silver bullet. Balance is key. Use it strategically in large applications where expensive re-renders can impact performance.It's like balancing gears and clocks for optimal precision.
Overusing
useCallback
can lead to unnecessary complexity, so apply it where it truly benefits your app’s performance.
Conclusion
Mastering useCallback
is a vital step in optimizing your React applications. By preventing unnecessary function re-creation and managing re-renders efficiently, you can ensure your app performs at its best. Remember, strategic use of useCallback
, combined with other hooks like useMemo
, can transform your app into a high-performance machine.
Thank you for diving into the world of useCallback
with us! We hope this guide has provided you with valuable insights and practical tips to optimize your React applications.
If you found this post helpful, don’t forget to share it with your fellow developers and join the conversation in the comments below.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
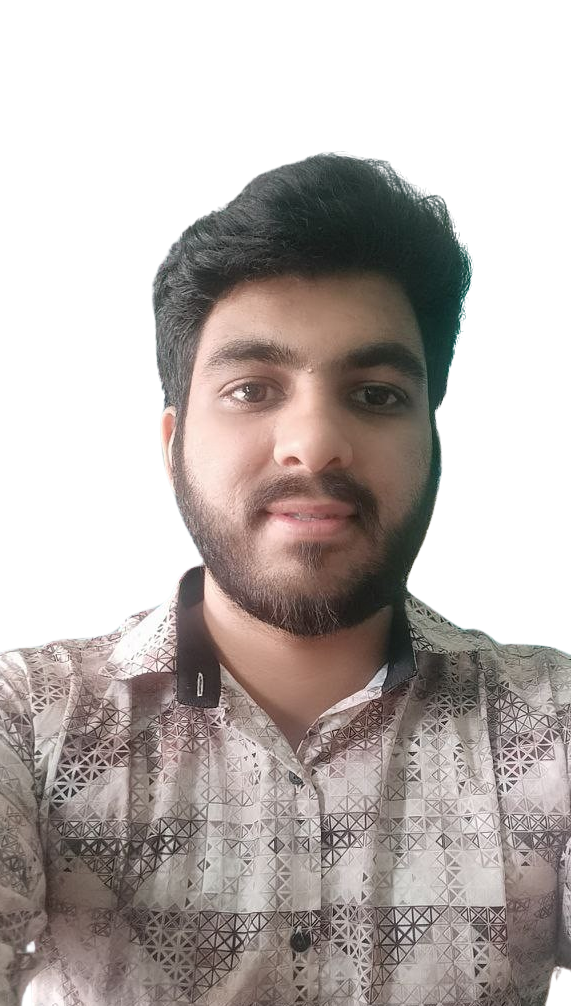
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone 👋🏻 , I'm Aaryan Bajaj , a Full Stack Web Developer from India(🇮🇳). I share my knowledge through engaging technical blogs on Hashnode, covering web development.