Understanding DataFrames in Machine Learning: A Comprehensive Guide
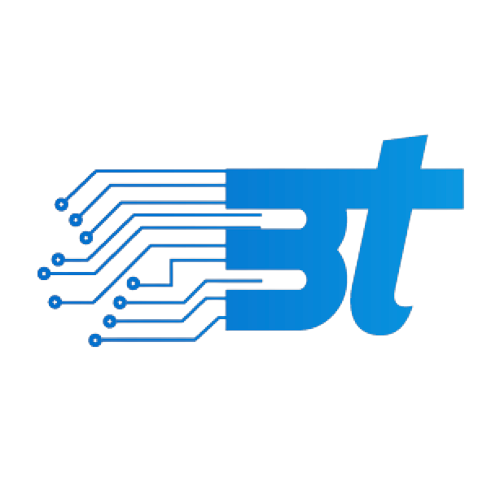
Table of contents
- Introduction
- What is a DataFrame?
- Creating a DataFrame
- Loading a Dataset into Pandas
- Displaying a Few Records
- Finding a Summary of the DataFrame
- Slicing and Indexing
- Value Counts and Cross-Tabulation
- Sorting in Dataframes
- Creating a New Column
- Grouping and Aggregating
- Joining Dataframes
- Re-Naming Columns
- Applying Operations to Multiple Columns
- Filtering Records based on Conditions
- Removing Columns or Rows from a Dataset
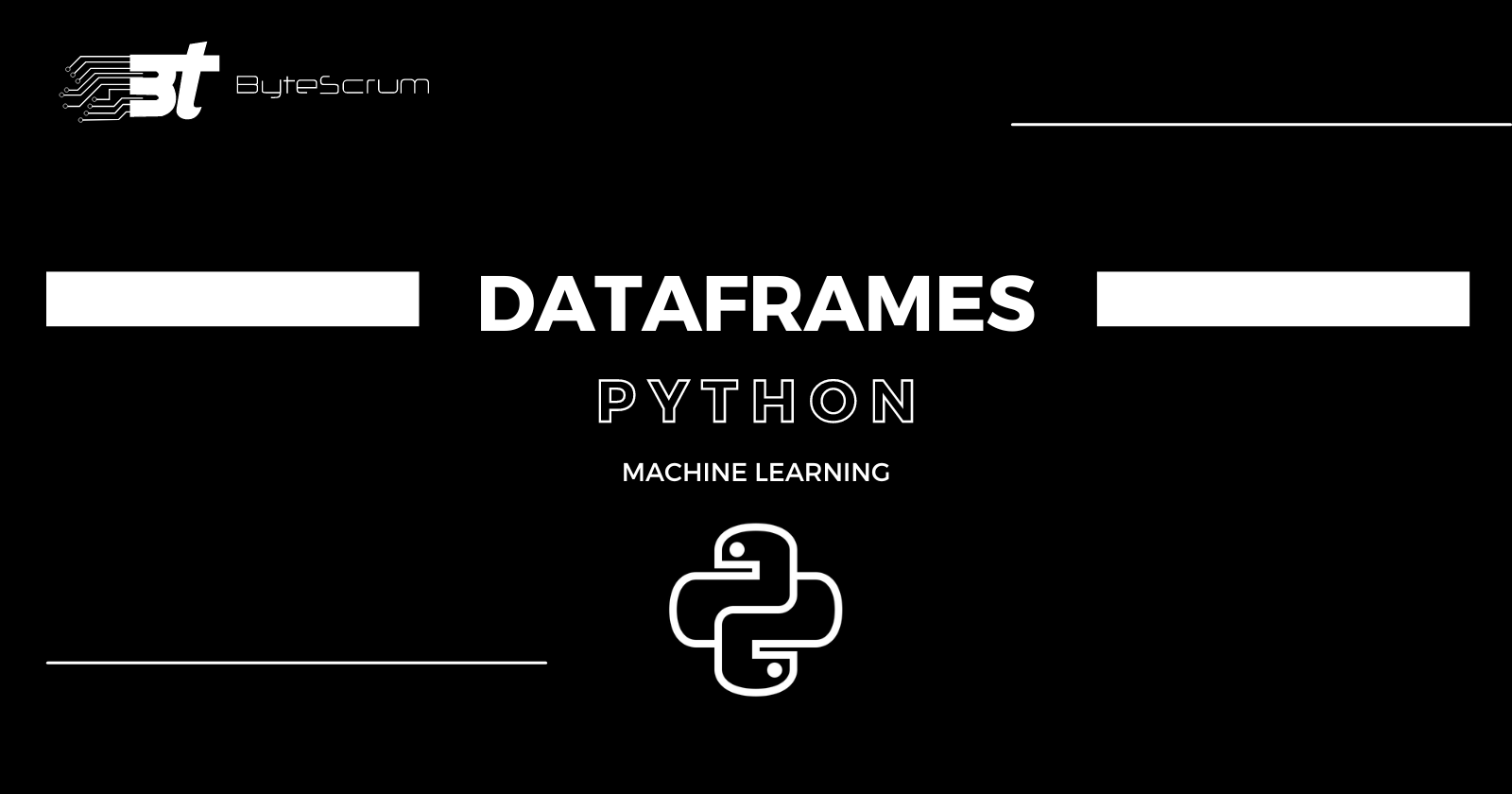
Introduction
DataFrames are a fundamental concept in machine learning and data analysis. They provide a way to organize and manipulate data in a tabular format, similar to a spreadsheet or a database table. In this blog post, we will explore what DataFrames are, how they are used in machine learning, and some common operations performed on them.
What is a DataFrame?
A DataFrame is a two-dimensional, size-mutable, and heterogeneous tabular data structure with labeled axes (rows and columns). It is a key data structure provided by libraries like pandas in Python for data manipulation and analysis.
Creating a DataFrame
You can create a DataFrame from various data sources, such as lists, dictionaries, or external files like CSV or Excel. Here's an example of creating a DataFrame from a dictionary:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'San Francisco', 'Los Angeles']}
df = pd.DataFrame(data)
print(df)
Loading a Dataset into Pandas
You can load a dataset into a DataFrame using pandas' read_csv()
function. This function reads the contents of a CSV file into a DataFrame, allowing you to work with the data in a structured format.
import pandas as pd
df = pd.read_csv('dataset.csv')
Displaying a Few Records
To display the first few records of a DataFrame, you can use the head()
method. This method returns the specified number of rows from the beginning of the DataFrame.
print(df.head(3))
Finding a Summary of the DataFrame
To get a summary of the DataFrame, you can use the info()
and describe()
methods. The info()
method provides information about the DataFrame, including the data types of each column and the number of non-null values. The describe()
method generates descriptive statistics for numerical columns in the DataFrame.
print(df.info())
print(df.describe())
Slicing and Indexing
You can slice and index a DataFrame using column names and row indexes. This allows you to select specific rows and columns from the DataFrame.
# Selecting a single column
print(df['column_name'])
# Slicing rows
print(df[2:5])
Value Counts and Cross-Tabulation
To get the count of unique values in a column, you can use the value_counts()
method. This method returns a Series containing counts of unique values. You can also create a cross-tabulation of two columns using the crosstab()
method.
print(df['column_name'].value_counts())
# Cross-tabulation
print(pd.crosstab(df['column1'], df['column2']))
Sorting in Dataframes
You can sort a DataFrame by one or more columns using the sort_values()
method. This method allows you to sort the DataFrame based on the values in one or more columns, in ascending or descending order.
# Sort by a single column
print(df.sort_values('column_name'))
# Sort by multiple columns
print(df.sort_values(['column1', 'column2']))
Creating a New Column
You can create a new column in a DataFrame by assigning values to it. This allows you to add calculated or derived values to the DataFrame based on existing columns.
df['new_column'] = df['column1'] + df['column2']
Grouping and Aggregating
You can group data in a DataFrame based on one or more columns and then perform aggregate functions on the grouped data. This allows you to calculate summary statistics for different groups in the data.
# Group by a single column
print(df.groupby('column_name').mean())
# Group by multiple columns
print(df.groupby(['column1', 'column2']).sum())
Joining Dataframes
You can join two DataFrames based on a key column using the merge()
method. This allows you to combine data from two different DataFrames into a single DataFrame.
df1 = pd.DataFrame({'key': ['A', 'B', 'C'], 'value': [1, 2, 3]})
df2 = pd.DataFrame({'key': ['A', 'B', 'D'], 'value': [4, 5, 6]})
merged_df = pd.merge(df1, df2, on='key', how='inner')
Re-Naming Columns
You can rename columns in a DataFrame using the rename()
method. This allows you to change the names of one or more columns in the DataFrame.
df.rename(columns={'old_name': 'new_name'}, inplace=True)
Applying Operations to Multiple Columns
You can apply a function to multiple columns in a DataFrame using the apply()
method. This allows you to perform the same operation on multiple columns simultaneously.
df[['column1', 'column2']] = df[['column1', 'column2']].apply(lambda x: x * 2)
Filtering Records based on Conditions
You can filter records in a DataFrame based on conditions using boolean indexing. This allows you to select rows that meet specific criteria.
filtered_df = df[df['column_name'] > 10]
Removing Columns or Rows from a Dataset
You can remove columns or rows from a DataFrame using the drop()
method. This allows you to remove unwanted columns or rows from the DataFrame.
# Remove columns
df.drop(['column1', 'column2'], axis=1, inplace=True)
# Remove rows
df.drop([0, 1, 2], axis=0, inplace=True)
Conclusion
Share your thoughts
What are your favorite DataFrame operations, and how have they helped you in your data analysis projects? Share your thoughts and experiences below!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
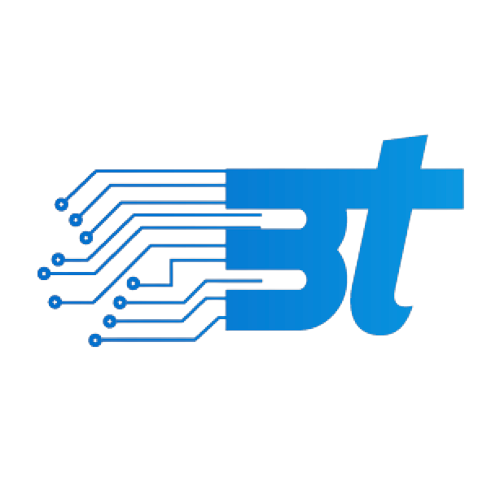
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.