List Comprehensions: Your Pythonic One-Liner for Lists

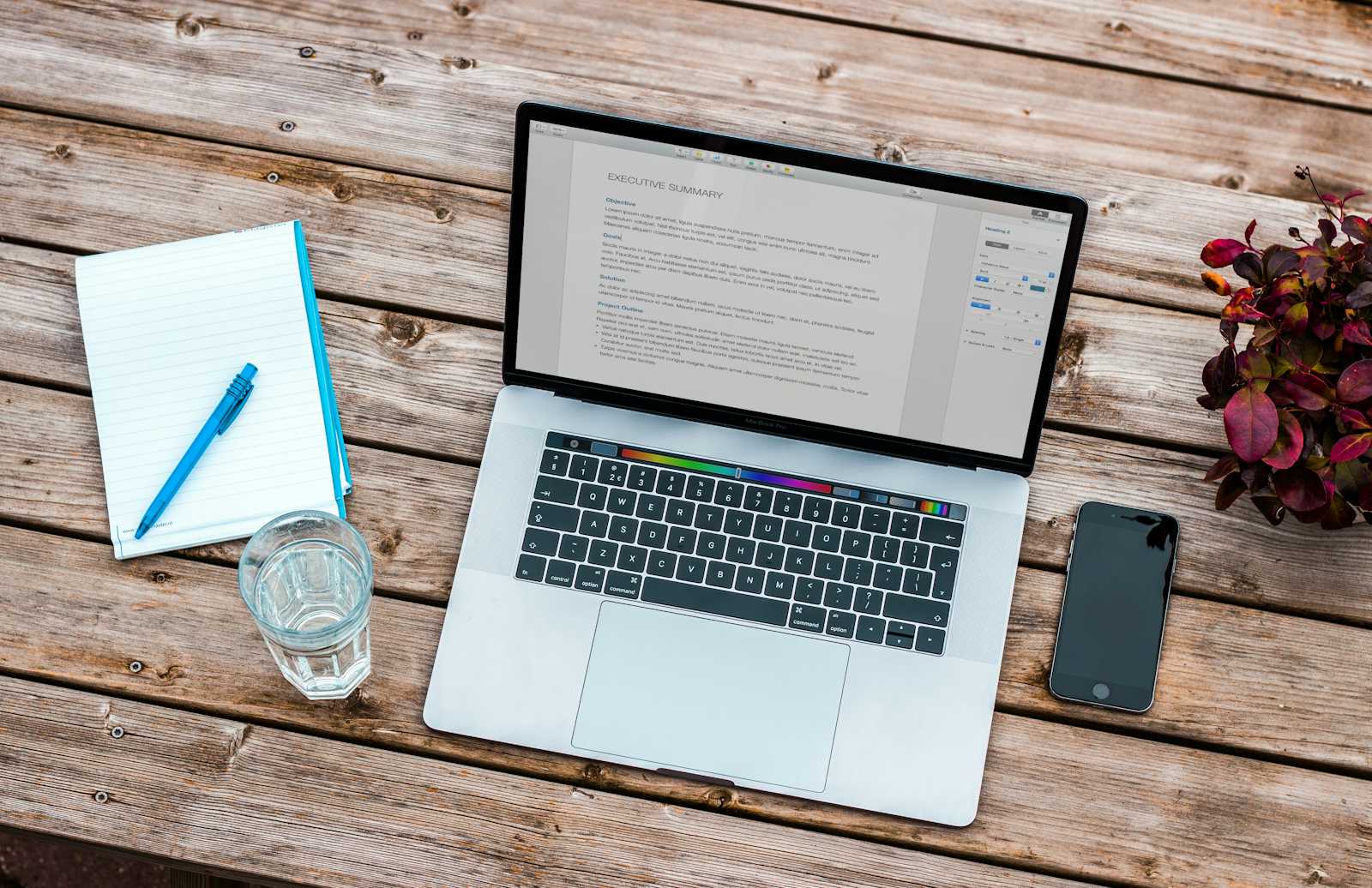
Today's topic is a game-changer for Python programmers: list comprehensions. They offer a concise and elegant way to create new lists based on existing data. Unlike other languages, Python provides this powerful tool to reduce code and improve readability.
What are List Comprehensions?
List comprehensions are a way to generate a new list by iterating over an existing iterable object (like a list, string, or range) and applying an expression to each element. They are like tiny for loops in square brackets, making your code more compact and readable.
Traditionally vs. List Comprehensions
Imagine you have a list of numbers [1, 2, 3]
and want a new list where each number is incremented by one. Traditionally, you'd use a for loop:
numbers = [1, 2, 3]
incremented_numbers = []
for number in numbers:
incremented_numbers.append(number + 1)
With list comprehensions, you can achieve the same in one line:
numbers = [1, 2, 3]
incremented_numbers = [num + 1 for num in numbers]
List Comprehension Breakdown:
[]
: Square brackets define the new list being created.expression
: This is what you want to do to each item in the iterable. In our example,num + 1
add 1 to each number.for item in iterable
: This iterates over each item in the original list (numbers
in our case), assigning it to the temporary variablenum
.
Let's Get Coding!
Challenge 1: Doubling Numbers
Try this yourself! Create a list doubled_numbers
that contains each number from numbers
doubled. Use the provided template and hit "Run" to see if your code works:
numbers = [1, 2, 3]
doubled_numbers = [ for num in numbers]
Challenge 2: Splitting Strings
List comprehensions aren't just for numbers! Take the string "Jack". Can you create a list named letters_list
containing each letter using a list comprehension?
Python
name = "Jack"
letters_list = [ for letter in name]
Bonus Challenge: Conditional Filtering
List comprehensions can be even more powerful with conditional statements. Suppose we have a list of names and want to extract only short names (four letters or less). Here's how:
names = ["Alex", "Beth", "Caroline", "Dave", "Eleanor", "Freddy"]
short_names = [name for name in names if len(name) <= 4]
Going Beyond the Basics
List comprehensions can be used for more complex tasks as well:
Nested List Comprehensions: Create lists within lists. For example, generating a multiplication table.
List Comprehensions with Lambda Functions: Combine lambda functions with list comprehensions for concise anonymous functions within the expression.
List Comprehensions vs. for Loops: When to Use Each
While list comprehensions are generally preferred for their readability and compactness, there are situations where for loops might be more suitable:
Modifying the original list: If you need to modify the original list while iterating, for loops offer more control.
Complex logic within the loop: For complex logic within the loop body, for loops might be easier to understand.
List Comprehensions: Your Pythonic Ally
List comprehensions are a valuable tool for Python programmers. They offer a concise, elegant, and Pythonic way to manipulate lists and other iterable objects. So next time you're coding, remember the power of list comprehensions and write cleaner, more efficient code!
Stay tuned for more Pythonic adventures, and until next time, happy coding!
Subscribe to my newsletter
Read articles from Rahul Bansod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rahul Bansod
Rahul Bansod
Software Engineer with a robust foundation in Computer Science complemented by practical experience in system administration, containerization technologies, and scripting languages. Skilled in Python, C++, JavaScript, Django, Node.js, and Flask, with expertise in Kubernetes, Docker, and Openshift. Possessing a keen aptitude for quickly mastering new technologies and leveraging them to tackle intricate challenges. Eager to contribute to the innovation and growth of a dynamic development environment