Database Join Queries

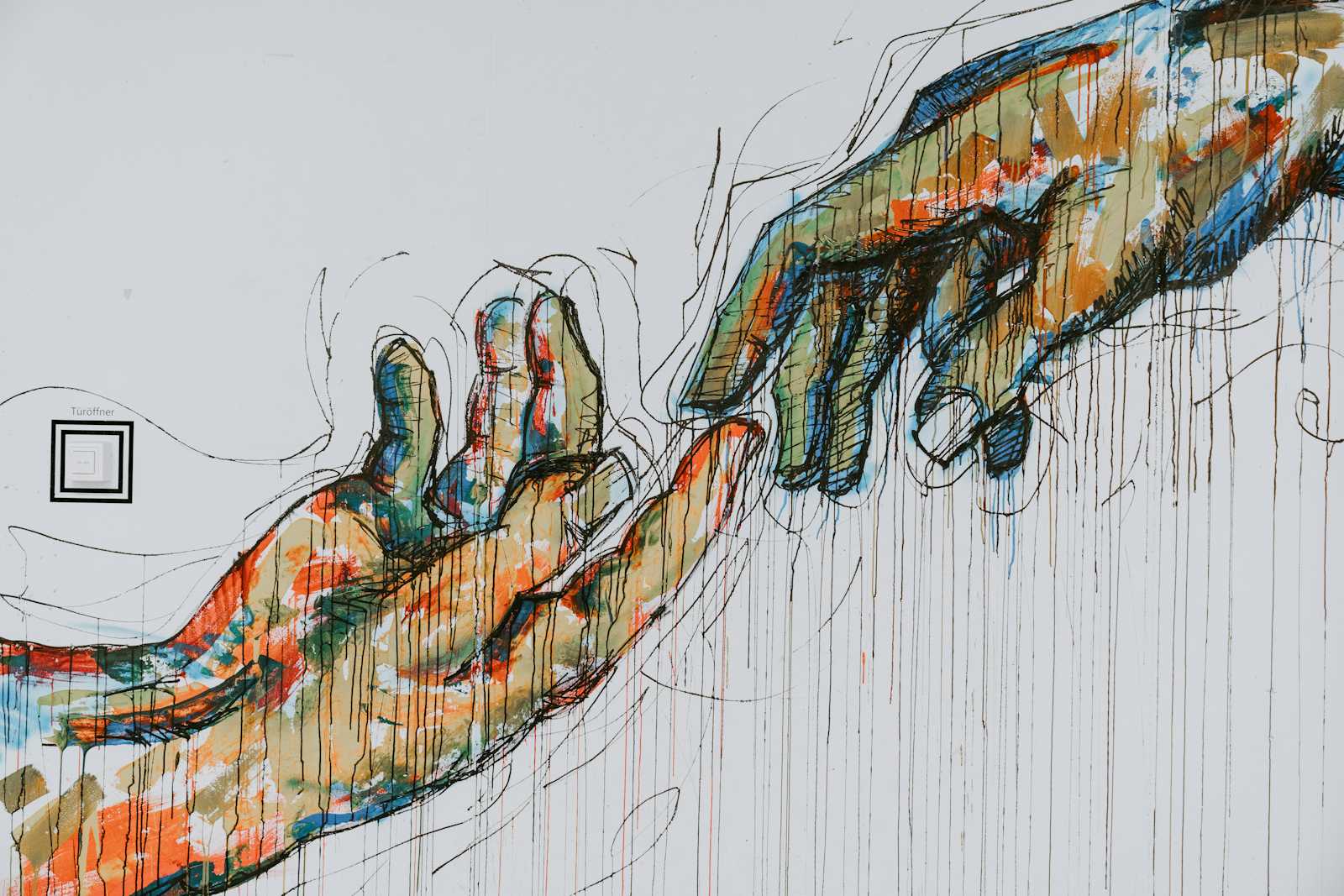
This is a Series of Article to start the series visit here.
In the realm of web development, database interactions are crucial for storing, retrieving, and manipulating data efficiently. Laravel, a popular PHP framework, offers robust support for database operations, making it a favorite among developers. One fundamental aspect of working with databases is mastering the art of join queries. In this article, we'll delve into various aspects of database join queries using MySQL, illustrating each concept with practical examples implemented in Laravel.
Understanding MySQL Join Types
MySQL joins are a fundamental concept for retrieving data from multiple tables in your database. Here's a breakdown of the different join types you'll encounter:
1. INNER JOIN (or Simple JOIN):
This is the most common type of join.
It returns only records that have matching values in both tables based on the join condition.
Imagine it like finding the intersection between two sets of data.
2. OUTER JOINs:
Outer joins return records from one table (the outer table) along with matching records from the other table (the inner table).
There are two main types of outer joins:
LEFT JOIN (or LEFT OUTER JOIN):
This returns all records from the left table, and matching records from the right table.
For rows in the left table without a match in the right table, null values are filled in the right table's columns.
RIGHT JOIN (or RIGHT OUTER JOIN):
This is the opposite of LEFT JOIN.
It returns all records from the right table, and matching records from the left table.
Null values are filled in the left table's columns for unmatched rows in the right table.
3. FULL JOIN:
A FULL JOIN returns all records from both tables, regardless of whether there's a match in the other table.
It combines the results of a LEFT JOIN and a RIGHT JOIN.
Rows without matches in either table will have null values in the corresponding columns.
4. CROSS JOIN (Cartesian Product):
This is the least commonly used join type.
It creates a new result set by pairing every row from one table with every row from the other table.
This can result in a very large dataset, so use it cautiously.pen_spark
Let's illustrate these concepts with a scenario. Consider two tables: users
and orders
. We want to retrieve a list of users along with their corresponding orders, if any.
SELECT *
FROM users
INNER JOIN orders ON users.id = orders.user_id;
To implement this sql query in Laravel we can use like this below.
$users = User::join('orders', 'users.id', '=', 'orders.user_id')
->select('*')
->get();
Grouping Results With Aggregate Functions
Aggregate functions process multiple rows of data and return a single summarized value. Common examples include:
COUNT(*)
: Counts the total number of rows in a group (often used with*
to indicate all rows).SUM(column_name)
: Calculates the total sum of a specific column's values within a group.AVG(column_name)
: Computes the average value of a specific column for each group.MIN(column_name)
: Returns the minimum value of a specific column within a group.MAX(column_name)
: Returns the maximum value of a specific column within a group.
Grouping Data:
The GROUP BY
clause specifies the column(s) to use for grouping the results. Rows with the same value(s) in the specified column(s) are grouped together, and aggregate functions are then applied to each group.
Example: Counting Orders per User
SELECT users.name, COUNT(orders.id) AS order_count
FROM users
LEFT JOIN orders ON users.id = orders.user_id
GROUP BY users.id;
Explanation:
SELECT: We select two columns:
users.name
and the result ofCOUNT(orders.id)
aliased asorder_count
.FROM: We specify the
users
table as the source.LEFT JOIN: We perform a LEFT JOIN with the
orders
table on the conditionusers.id = orders.user_id
.GROUP BY: We group the results by the
users.id
column.
This query retrieves the name of each user and the total number of orders they have placed. Users without any orders will still be included with a NULL
value for order_count
.
Laravel Example:
$results = User::select('users.name', DB::raw('COUNT(orders.id) AS order_count'))
->leftJoin('orders', 'users.id', '=', 'orders.user_id')
->groupBy('users.id')
->get();
Explanation:
User::: We start with the
User
model.select: We define the columns to be retrieved:
users.name
and the result ofDB::raw('COUNT(orders.id) AS order_count')
.leftJoin: Similar to the SQL query, we perform a LEFT JOIN.
groupBy: We group the results by
users.id
.get: We execute the query and retrieve the results as a collection of
User
models.
This code achieves the same functionality as the SQL query, but with a more Laravel-specific syntax using Eloquent.
Performing Multiple Joins in One Query
In real-world scenarios, it's common to join more than two tables in a single query to retrieve comprehensive data. Laravel provides an elegant syntax for achieving this with its ORM (Object-Relational Mapping) capabilities.
Suppose we have three tables: users
, orders
, and products
. We want to retrieve user details along with the products they have ordered.
SELECT users.name, orders.id AS order_id, products.name AS product_name
FROM users
INNER JOIN orders ON users.id = orders.user_id
INNER JOIN products ON orders.product_id = products.id;
$results = User::select('users.name', 'orders.id AS order_id', 'products.name AS product_name')
->join('orders', 'users.id', '=', 'orders.user_id')
->join('products', 'orders.product_id', '=', 'products.id')
->get();
Filtering Aggregated Data
Filtering aggregated data involves applying conditions to aggregated results. This is commonly done using the HAVING
clause in SQL queries.
For instance, let's filter users based on the total number of orders they've placed:
SELECT users.name, COUNT(orders.id) AS order_count
FROM users
LEFT JOIN orders ON users.id = orders.user_id
GROUP BY users.id
HAVING order_count > 5;
In Laravel, achieving the same result is straightforward:
$users = User::select('users.name', DB::raw('COUNT(orders.id) AS order_count'))
->leftJoin('orders', 'users.id', '=', 'orders.user_id')
->groupBy('users.id')
->having('order_count', '>', 5)
->get();
Conclusion
By understanding the various join types, leveraging aggregate functions, performing multiple joins, and filtering aggregated data, developers can efficiently retrieve and manipulate data from multiple tables. With MySQL and Laravel's powerful tools and syntax, developers have everything they need to handle complex data operations seamlessly, ensuring the smooth functioning of their applications.
Subscribe to my newsletter
Read articles from Saiful Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saiful Alam
Saiful Alam
An Expert software engineer in Laravel and React. Creates robust backends and seamless user interfaces. Committed to clean code and efficient project delivery, In-demand for delivering excellent user experiences.