Level Up Your PHP with Laravel Collections
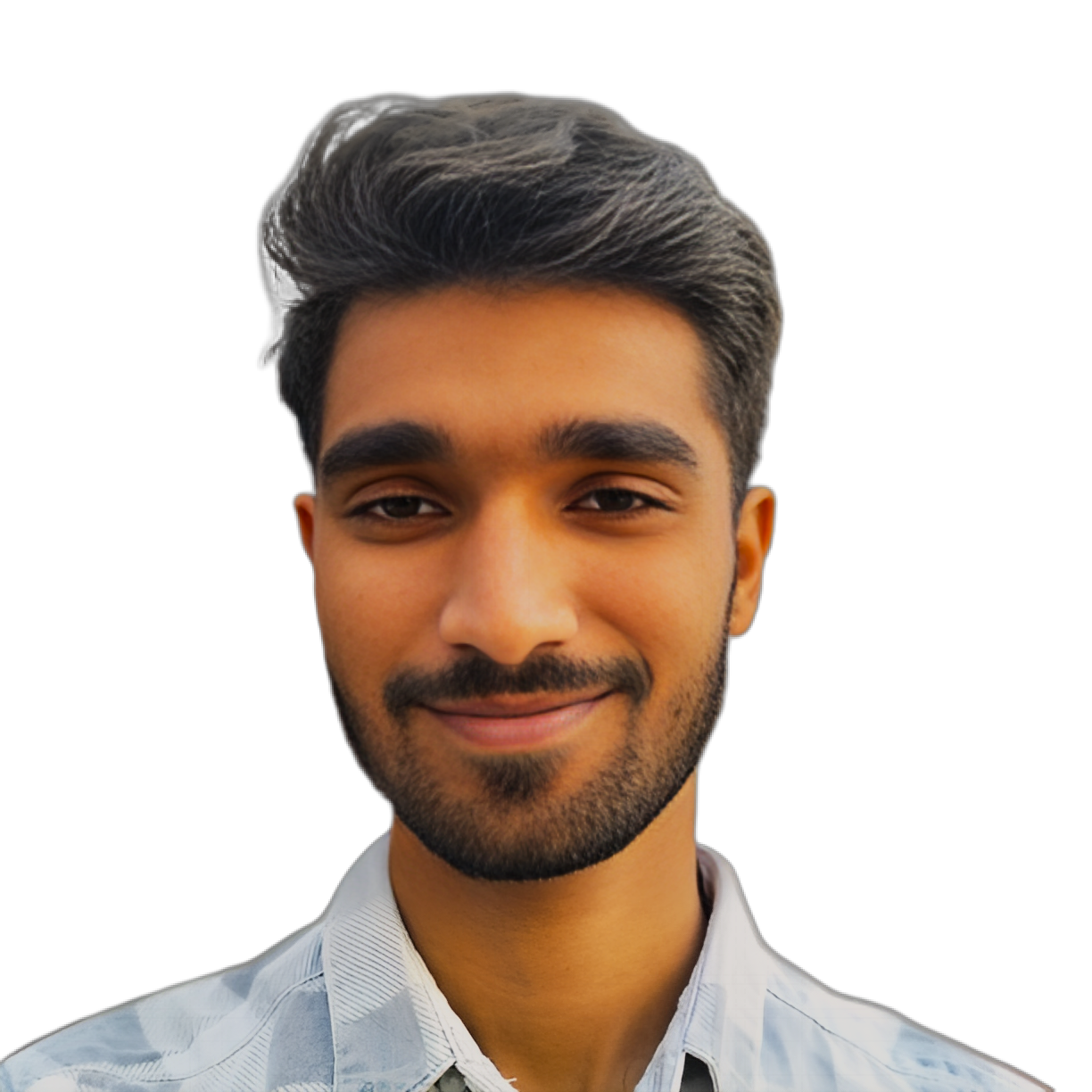
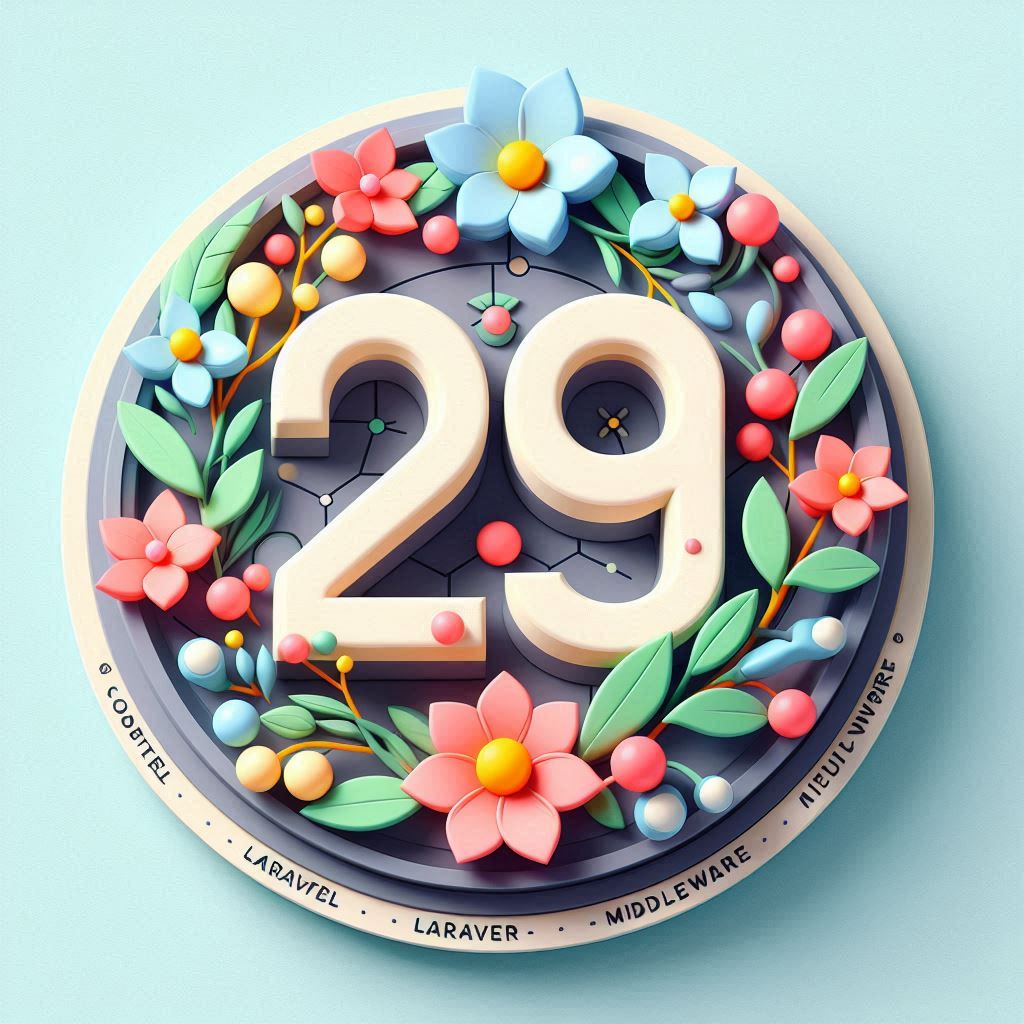
Laravel collections are a game-changer for managing data in your Laravel applications. They offer a powerful and intuitive way to manipulate, organize, and transform your data, leading to cleaner, more efficient code. In this blog post, we'll dive deep into Laravel collections, exploring their functionalities and how to implement them in your projects.
What are Laravel Collections?
Imagine a toolbox overflowing with various tools. Working with raw PHP arrays can feel like juggling all those tools at once. Laravel collections, on the other hand, are like a sleek, organized set specifically designed for the task at hand. They provide a wrapper around native PHP arrays, offering a collection of methods tailored for working with data in a more functional way.
The Power of Collections
Here's what makes Laravel collections so powerful:
- Fluent Methods: Collections allow you to chain multiple methods together for complex data transformations. This "fluent interface" makes your code incredibly expressive and readable. Think of it like writing a sentence where each method is a word that builds on the previous one. For example, you can filter a collection of users, map them to their usernames, and finally pluck only the usernames:
PHP
$usernames = User::all()->filter(function ($user) {
return $user->isActive();
})->map(function ($user) {
return $user->username;
})->pluck('username');
Immutability: Unlike native PHP arrays, collections are immutable. This means that when you apply a method like
filter
ormap
, it doesn't modify the original data source. Instead, it creates a new collection with the desired changes. This prevents unintended side effects and makes debugging easier.Higher-Order Messages: Collections come pre-equipped with powerful methods like
map
,filter
, andreduce
that handle common data operations in a concise and functional way. These methods eliminate the need for verbose loops and conditional statements, keeping your code clean and focused.
Implementing Collections in Your Laravel Application
Let's get our hands dirty and see how to implement collections in your Laravel project.
- Creating a Collection:
There are two main ways to create a collection:
- From an Array:
PHP
$numbers = collect([1, 2, 3, 4, 5]);
- From a Database Query:
PHP
$users = User::all()->toCollection();
- Filtering Data:
Use the filter
method to extract a subset of data based on a specific condition:
PHP
$activeUsers = User::all()->filter(function ($user) {
return $user->isActive();
});
- Transforming Data:
The map
method allows you to apply a closure (anonymous function) to each item in a collection:
PHP
$usernames = User::all()->map(function ($user) {
return $user->username;
});
- Extracting Specific Data:
The pluck
method is handy for extracting a single property from each item in a collection:
PHP
$userEmails = User::all()->pluck('email');
- Aggregating Data:
Collections offer methods like sum
, average
, min
, and max
for performing calculations on numerical data:
PHP
$totalPosts = Post::all()->sum('count');
These are just a few examples of the many ways you can leverage Laravel collections. The documentation provides a comprehensive list of available methods and their functionalities https://laravel.com/docs/11.x/collections.
The Laravel Collections Advantage
By incorporating Laravel collections into your development workflow, you'll experience several benefits:
Cleaner Code: Fluent methods and higher-order messages lead to more concise and readable code.
Improved Maintainability: Immutability ensures your code is less prone to bugs and easier to maintain.
Functional Approach: Collections encourage a functional programming style, promoting cleaner and more expressive code.
Conclusion
Laravel collections are a powerful tool that can significantly enhance your development experience. They offer an intuitive and efficient way to manage data in your Laravel applications. So, the next time you're working with data, consider using collections to streamline your workflow and write cleaner, more maintainable code.
We encourage you to explore the Laravel documentation and experiment with collections in your projects. You'll be surprised by how much they can improve your development process!
Bonus Tip: There are many online tutorials and resources available that delve deeper into specific collection methods and applications. Don't hesitate to explore them to further expand your knowledge.
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
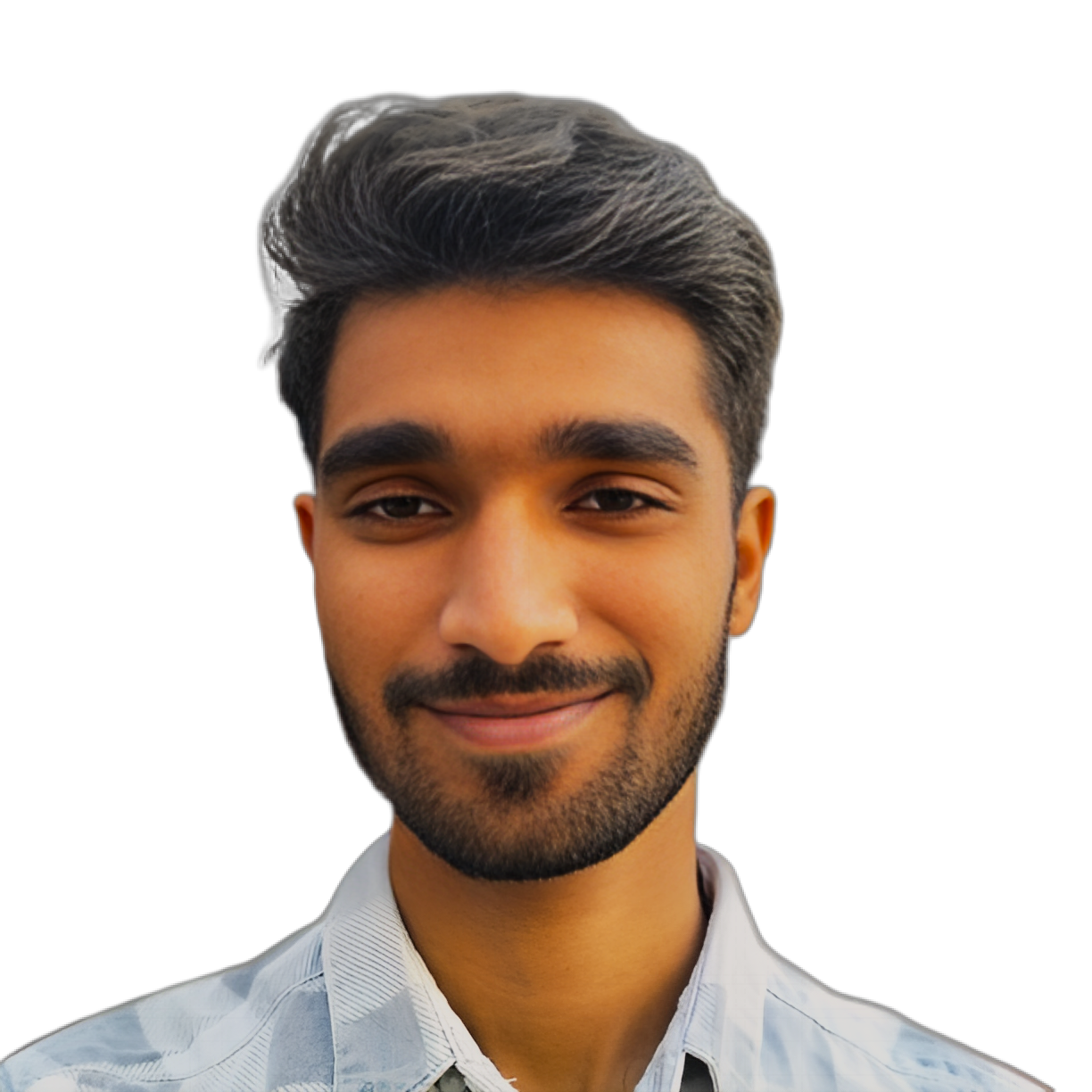
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.