Session 1 - Python Fundamentals
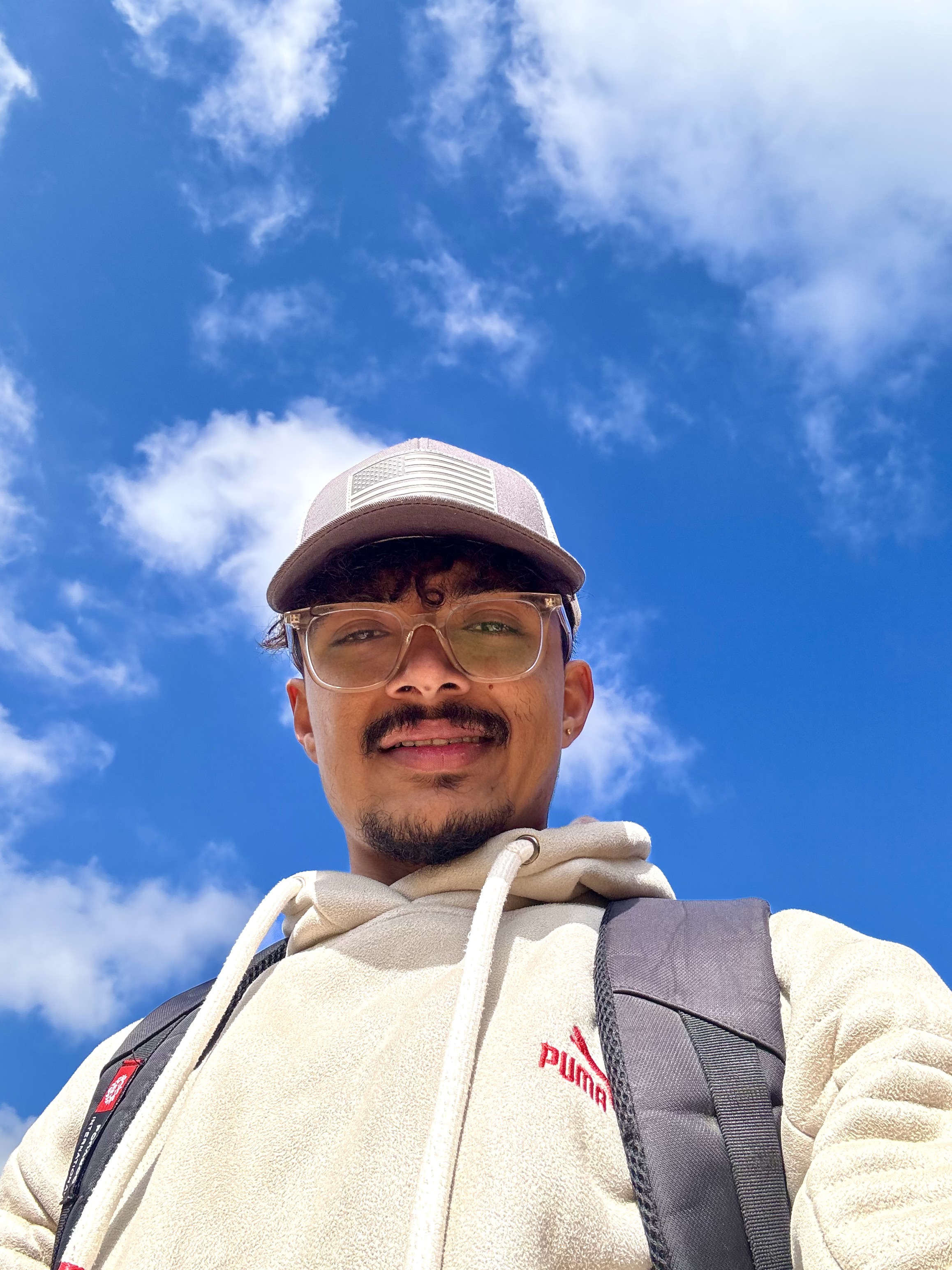
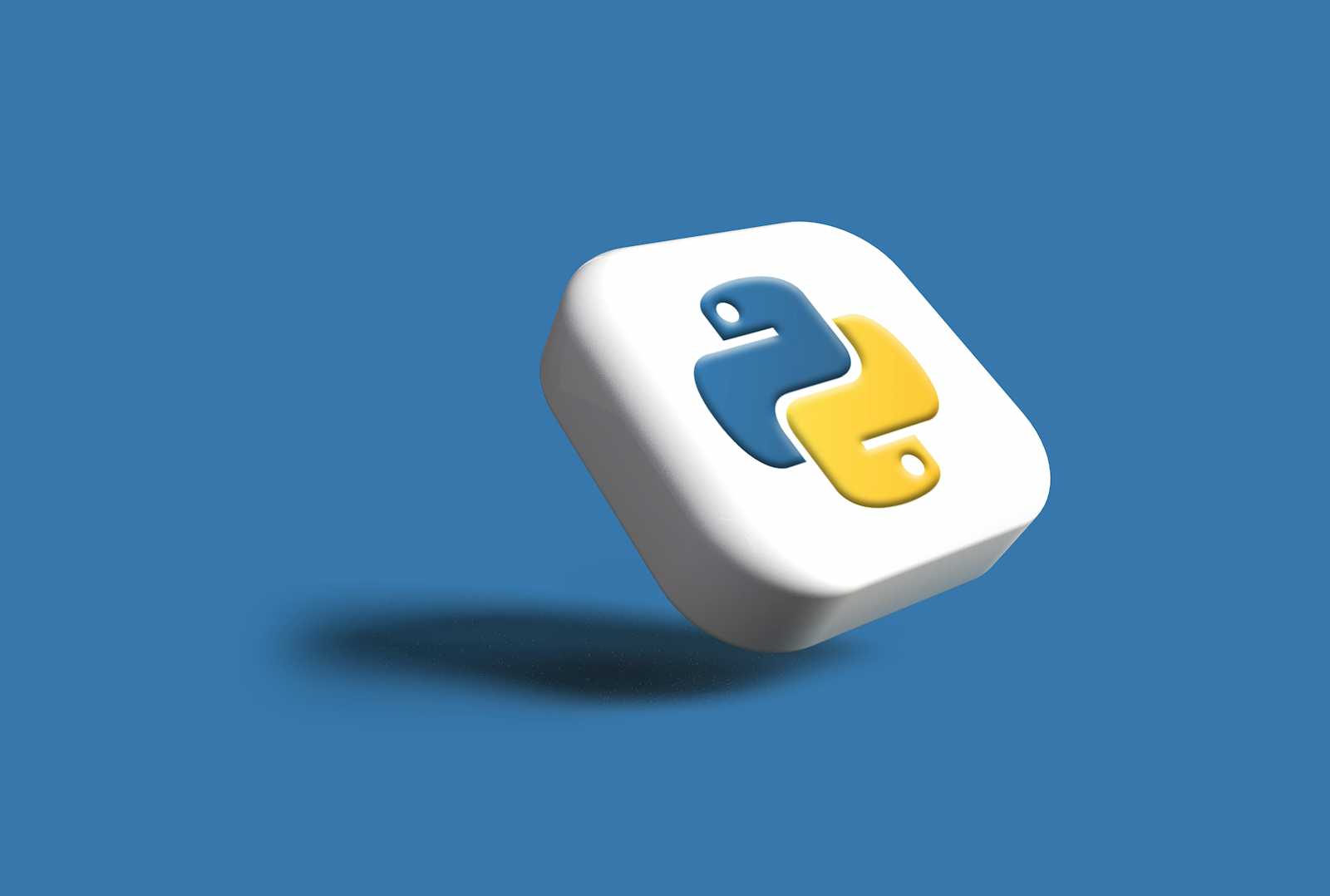
Python is a programming language that has become very famous in recent years. It is powerful, flexible, and easy to learn. Since it was first released in 1991 by Guido van Rossum, Python has been a great choice for both new and experienced coders because it focuses on making code easy to read and simple.
Powerful Libraries: Tools like NumPy, Pandas, Matplotlib, and Scikit-Learn simplify data analysis, manipulation, visualization, and machine learning.
Community and Resources: A large, active community and abundant resources (tutorials, courses, documentation) provide ample learning support.
Versatility: Python integrates well with other technologies and is used across various fields, making it a flexible tool.
Industry Demand: Many top companies use Python for data science, enhancing job prospects and career opportunities.
Python Output
print('Hello World')
#Output: Hello World
print('Hello', 1, 4.5, True) # multiple item print
#Output: Hello 1 4.5 True
print('Hello', 1, 4.5, True, sep = '/') # each item seperated by /
#Output: Hello/1/4.5/True
print('Hello', end = '-') # Default newline
print('World')
#Output: Hello-World
Remember, python is case-sensitive language means is Print() and print() are not same.
Data Type
In every programming language data type are important, python has integer, float, boolean, string, complex, list, tuple, sets, dictionary.
# Integer
print(8)
print(1e308) # handel till 1* 10 ^ 308 integers
#Float
print(23.3)
print(1.7e308) # handel till 1 * 10 ^ 308 floats
# Boolean
# True | False
print(True)
# String
print('Hey I am a string')
# NOTE: Python doesn't have char dtype only string
# Complex
print(5+6j)
#Output:
# 8
# inf
# 23.3
# inf
# True
# Hey I am a string
# (5+6j)
# List
print([1,2,3,4,5])
# Tuple
print(12,13,14,15)
# Dictionary
print({Fruit: 'Apple', Name: 'Beta'}
# Set
print({1,2,3,4,5})
#Output:
# [1, 2, 3, 4, 5]
# 12 13 14 15
# {'name': 'Ninja', 'gender': 'Male', 'weight': 60}
# {1, 2, 3, 4, 5}
Variables
Variables in python on the other hand, are like labeled containers that store any kind of information. For eg: You are a web developer and want to show login user name after s/he login-in successfully [ Hello, [var_name] ] for this, you just define a variable name and as user login in you pass that name to var_name . So, in simple term variables are container for future use.
name = 'myName' print(name) # No need to explicitly declear dtype it automatically know a = 5 b = 6 print(a + b) # Output: 11 # In python, variable don't need to declear at the top like C/ C++, # as you need you declear it.
โInterview Question:1. Difference between Dynamic vs Static Typing?
- In dynamic, you explicitly tell data-type while declaring variable.
Eg. int a = 5; [ C/ C++/ Java]
- In static, you don't need to declare data-type, interpreter automatically know. Eg. a = 5
2. Difference between Dynamic vs Static Binding?
- In dynamic binding, variable you created doesn't have fixed data-type meaning we can change it's data-type as need.
Eg. a = 5 | a = 'apple' โ
- In static binding, once you define a variable data-type then it's fixed you can't change its data-type.
Eg. int num = 50 | string num = 'hello' โ
Keywords and Identifiers
Simply, keyword are the reserved word which are predefined in python and have special meanings to the compiler. We cannot use this 36 keywords as variable name, function name in python.
Identifiers are the names given by programmer to variables, functions, classes. There are some rules we need to follow to create a valid identifiers.
You can't start name with a digit.
1name = 'myName' โ
You cannot use any special character except underscore
_
first_name = 'John'
โAny other than underscore
_
are not valid to create an identifiers.Identifiers cannot be keywords
User Input
Taking input from user is important as a programmer. There are two type of software: static and dynamic software. Static software are those software which do not interact with user (only provide information). For eg: clock, calendar, a blog, college website (mostly static). Dynamic software is where user interact with software (input). For eg: Youtube, booking ride, placing food order and so on. In today's world, almost 90 % of software are dynamic software.
To make, such dynamic software, we need to take input from the user first.
# Taking input from user and store in user_name variable
user_name = input("Enter your name:")
print("Hello, {0}! How are you?".format(user_name))
# Output:
# Enter your name: rajesh
# Hello, rajesh! How are you?
Task :Write a program to take 2 numbers from user and print sum of two numbers.
Type Conversion
In python, we have two type conversion, implicit and explicit. In implicit type conversion, compiler internally convert the type with programmer interaction. But, implicit doesn't work all the time. Whereas, explicit type conversion, programmer change the type of data manually.
# Implicit
print(5+6.6) # 11.6
print(type(5),type(5.6)) # <class 'int'> <class 'float'>
## Python automatically convert the type but may not work always.
print(4 + '4') # Error
# Explicit
# string to integer
type(int('4')) # int
int(4.5) # 4
int(4+5j) # Error [can't convert complex to integer]
#### So, type conversion only work when possible.
str(5) # '5'
float(6) # 6.0
Literals
Literals are the raw values that you store in variable. There are many way to set literals in python we will look on them one by one.
a = 0b1010 # Binary Literals b = 100 # Decimal Literal c = 0o310 # Octal Literal d = 0x12c # Hexadecimal Literal # Float Literal float_1 = 10.5 float_2 = 1.5e2 # 1.5 * 10^2 float_3 = 1.5e-3 # 1.5 * 10^-3 # Complex Literal x = 3.14j print(a, b, c, d) # 10 100 200 300 print(float_1, float_2,float_3) # 10.5 150.0 0.0015 print(x, x.imag, x.real) # 3.14j 3.14 0.0
Moreover, in python we can store unicode (emojis) and raw strings.
unicode = u"\U0001f600\U0001F606\U0001F923"
raw_str = r"raw \n string"
print(unicode) # ๐๐๐คฃ
print(raw_str) # raw \n string
# In python, boolean True (1) and False (0) treated as a number
# So, we can do as follows,
a = True + 4
b = False + 10
print(a) # 5
print(b) # 10
None Literals
In python like other programming we cannot declare variable beforehand. To do so, we can used None (a variable with no value).
k # error can't do
a = None # use None to clear no value variable
print(a) # None
Practice Question
1. WAP that will convert celsius value to Fahrenheit.
2. WAP to take 2 numbers from user and swap them without using any special python syntax.
3. WAP to find the sum of squares of first n natural numbers where n will be provided by the user.
4. Given the first 2 terms of an Arithmetic Series. Find the Nth term of the series. Just assume all inputs are provided by user.
Happy Coding! ๐ง๐ปโ๐ป
Subscribe to my newsletter
Read articles from Manoj Paudel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
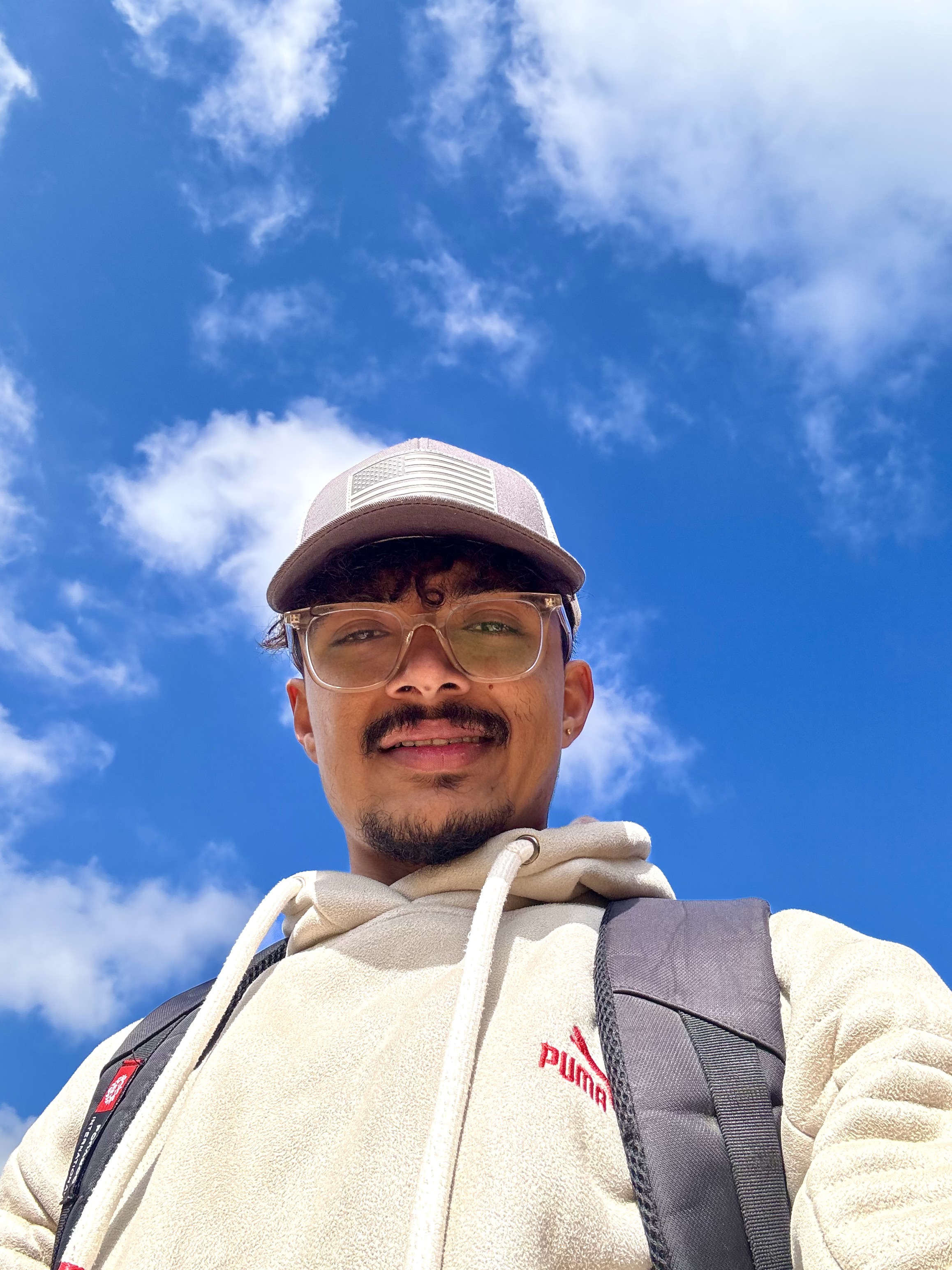
Manoj Paudel
Manoj Paudel
Hey there! I'm Manoj Paudel, a third-year Computer Science student diving into the world of data science. I'm excited to share my learning journey through my blogs.