Unlocking the Power of Node.js: Understanding Node-Modules
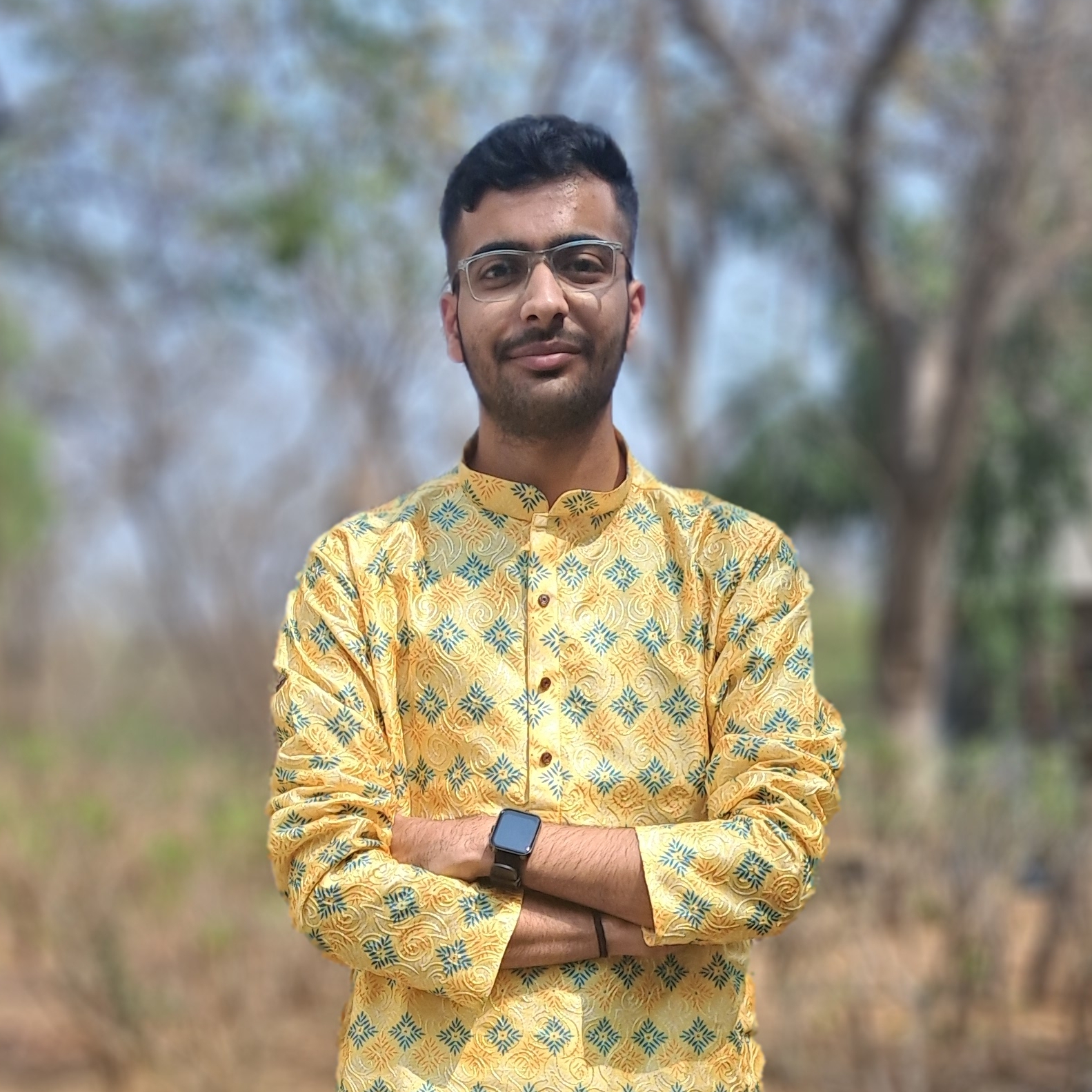
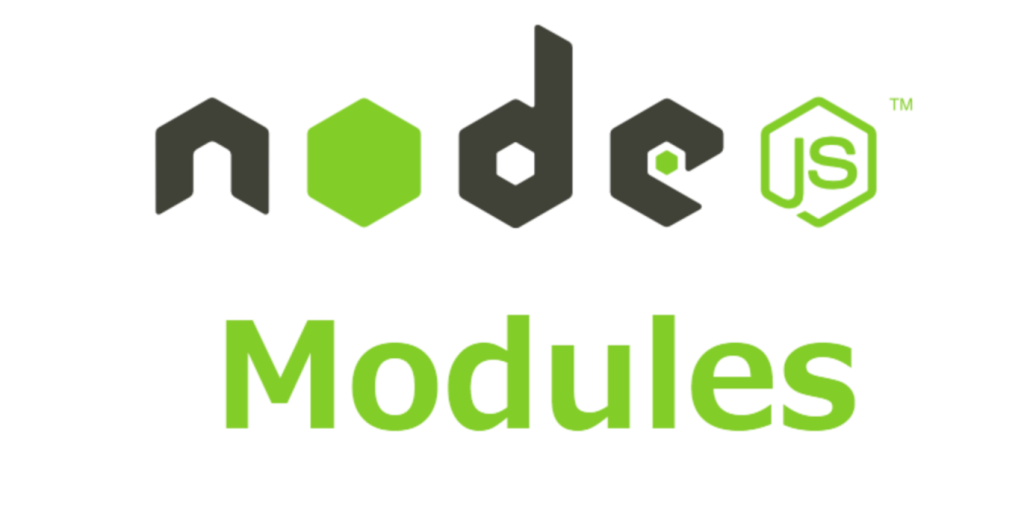
Node.js modules are reusable pieces of code that can be included in a Node.js application. They allow for better code organization and reuse. Node.js comes with a set of built-in modules (e.g., fs
for file system operations, http
for creating web servers), and you can also create your own custom modules.
Types of Modules in Node.js
There are three types of Node.js modules :
Core Modules : These are inbuilt in Node.js, examples of such modules are 'fs', 'http', 'path', etc.
Third-party Modules : These modules are developed by other fellow developers and are available to use on NPM library. For example, 'express' and 'lodash'.
Custom Modules : User created modules which are available for usage locally on a system for a specific functionality.
Exporting Modules :
Let's take an example of JavaScript functions which we need to export as modules :
const add = (a,b) => a + b;
const sub = (a,b) => a - b;
const mul = (a,b) => a * b;
const div = (a,b) => a / b;
Now, there are two methods to export modules :
First method, should be used if the module exports single function or object
const add = (a,b) => a + b;
const sub = (a,b) => a - b;
const mul = (a,b) => a * b;
const div = (a,b) => a / b;
module.exports = { add, sub, mul, div }; // exports the module
Second method, should be used if the module exports multiple functions or objects
exports.add = (a,b) => a + b;
exports.sub = (a,b) => a - b;
exports.mul = (a,b) => a * b;
exports.div = (a,b) => a / b;
Importing and using node modules
const math = require('./math'); // importing a custom module
console.log(math.add(1,2));
console.log(math.sub(1,2));
console.log(math.mul(1,2));
console.log(math.div(1,2));
const path = require('path'); // importing an inbuilt module
By organizing your code into modules, you can create more maintainable and reusable code. This modular approach is essential for building scalable and manageable Node.js applications.
In conclusion, understanding Node.js modules is crucial for any developer looking to build efficient and scalable applications. By leveraging core, third-party, and custom modules, you can enhance code organization, promote reuse, and streamline development processes. Embracing this modular approach will not only improve the maintainability of your code but also enable you to tackle complex projects with greater ease and efficiency. Happy coding!
Peace! โ๐ป
Subscribe to my newsletter
Read articles from Sanchit Pahurkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
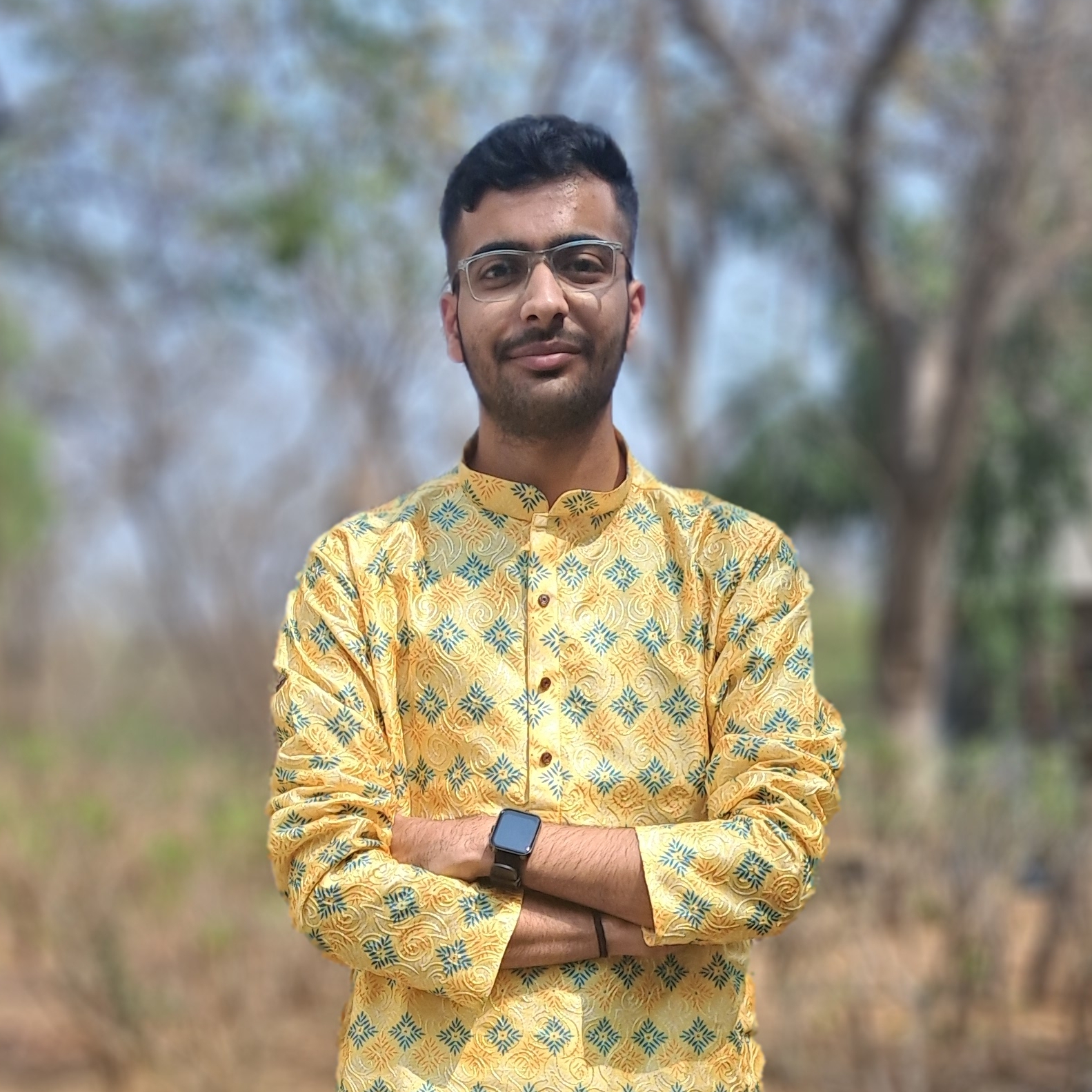