Flutter Networking: A Comprehensive Guide to HTTP Requests, JSON Parsing, and File Transfers
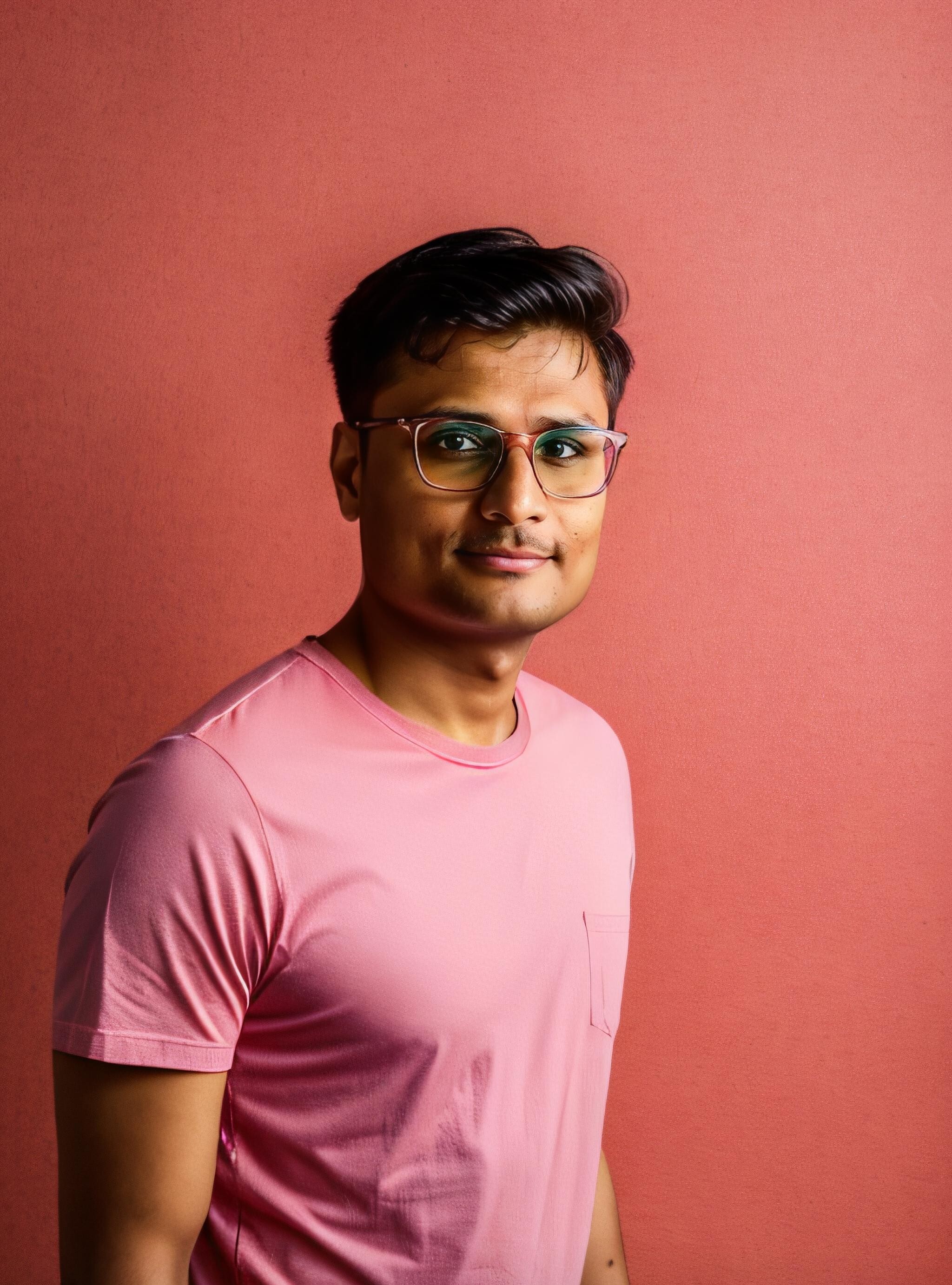
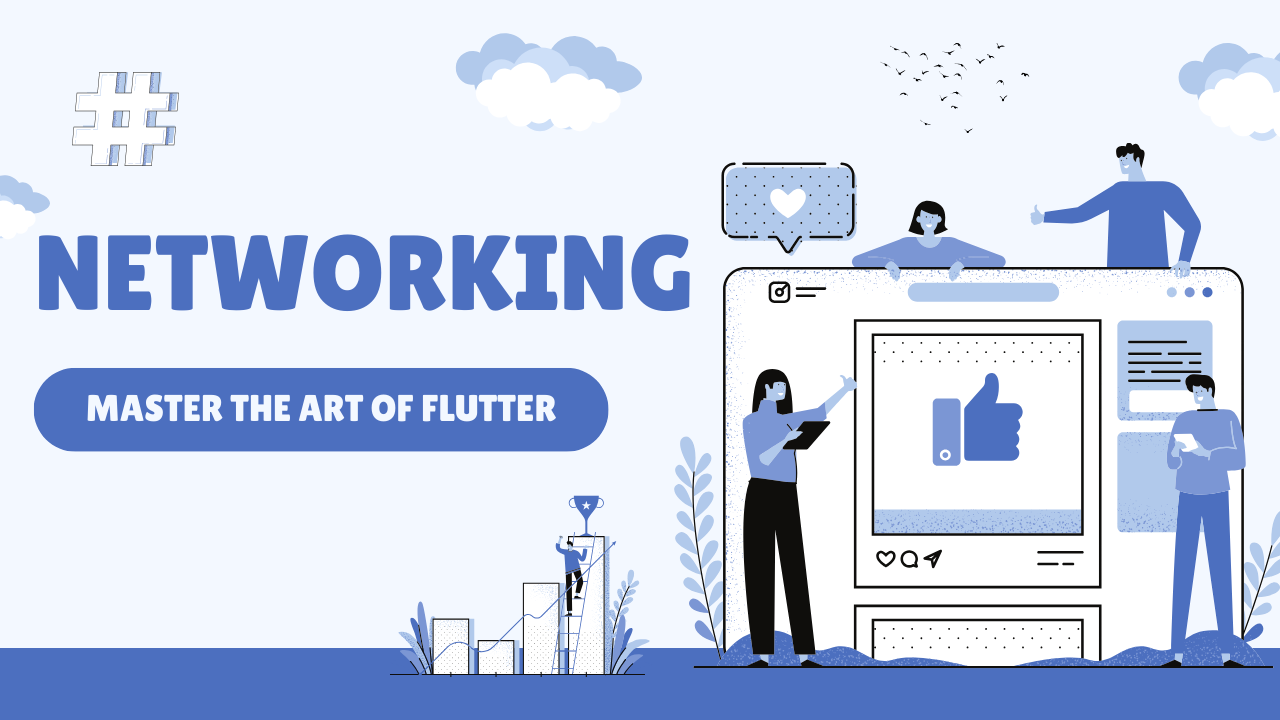
Making HTTP Requests
One of the fundamental tasks in networking is making HTTP requests to retrieve data from a server. Flutter provides the http
package, which makes it easy to send HTTP requests. With the http
package, you can make GET, POST, PUT, DELETE, and other types of requests.
Here’s an example of making a GET request using the http
package:
import 'package:http/http.dart' as http;
void fetchData() async {
var url = '<https://api.example.com/data>';
var response = await http.get(url);
if (response.statusCode == 200) {
// Process the response data
var data = response.body;
// ...
} else {
// Handle error
print('Request failed with status: ${response.statusCode}');
}
}
In the above code, we import the http
package and use the get
method to make a GET request to the specified URL. We then check the response status code to determine if the request was successful or not.
HTTP requests can also include headers, query parameters, and request bodies. The http
package provides methods and classes to handle these aspects of HTTP requests. For example, you can set headers using the headers
property of the request object, and you can send JSON data in the request body using the post
method and the body
parameter.
Parsing JSON Responses
When working with APIs, it’s common to receive data in JSON format. Flutter provides built-in support for parsing JSON responses. The dart:convert
library provides the json.decode
method to parse JSON strings into Dart objects.
Here’s an example of parsing a JSON response:
import 'dart:convert';
void parseJson() {
var jsonString = '{"name": "John", "age": 30}';
var jsonData = json.decode(jsonString);
var name = jsonData['name'];
var age = jsonData['age'];
print('Name: $name, Age: $age');
}
In the above code, we import the dart:convert
library and use the json.decode
method to parse the JSON string into a Map
object. We can then access the values in the Map
using their keys.
Sometimes, JSON responses can be more complex, with nested objects and arrays. In such cases, you can use the json.decode
method to parse the JSON string into a hierarchy of Dart objects. You can then access the nested objects and arrays using dot notation or index notation.
Displaying Data from a Server
Once you have retrieved data from a server, you may want to display it in your application. Flutter provides various widgets to display data, such as Text
, ListView
, and GridView
.
Here’s an example of displaying data in a ListView
:
import 'package:flutter/material.dart';
class DataList extends StatelessWidget {
final List<String> items;
DataList(this.items);
@override
Widget build(BuildContext context) {
return ListView.builder(
itemCount: items.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(items[index]),
);
},
);
}
}
In the above code, we define a DataList
widget that takes a list of strings as a parameter. We use the ListView.builder
widget to dynamically create list items based on the length of the items
list. Each list item is represented by a ListTile
widget, which displays the text from the items
list.
You can customize the appearance of the displayed data by using different Flutter widgets and applying styles and layouts. For example, you can use the Text
widget to display text, the Image
widget to display images, and the Card
widget to create a card-like layout for each item in the list.
Uploading and Downloading Files
In some cases, you may need to upload or download files from a server. Flutter provides the http
package to handle file upload and download operations. With the http
package, you can create MultipartRequest
objects to upload files and send them to the server.
Here’s an example of uploading a file:
import 'package:http/http.dart' as http;
void uploadFile() async {
var url = '<https://api.example.com/upload>';
var file = // File object to upload
var request = http.MultipartRequest('POST', Uri.parse(url));
request.files.add(await http.MultipartFile.fromPath('file', file.path));
var response = await request.send();
if (response.statusCode == 200) {
// Handle success
print('File uploaded successfully');
} else {
// Handle error
print('File upload failed with status: ${response.statusCode}');
}
}
In the above code, we create a MultipartRequest
object and add a MultipartFile
to it. We then send the request using the send
method and check the response status code to determine if the file upload was successful.
You can also download files from a server using the http
package. The http
package provides methods to make GET requests and download files directly to the device's storage. You can then use the downloaded files in your Flutter application.
Conclusion
By understanding and implementing these concepts, you’ll be able to build powerful networking functionality in your Flutter applications.
In the next article, we will explore various advanced tools and platforms that can be integrated with Flutter to enhance the functionality of your applications.
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
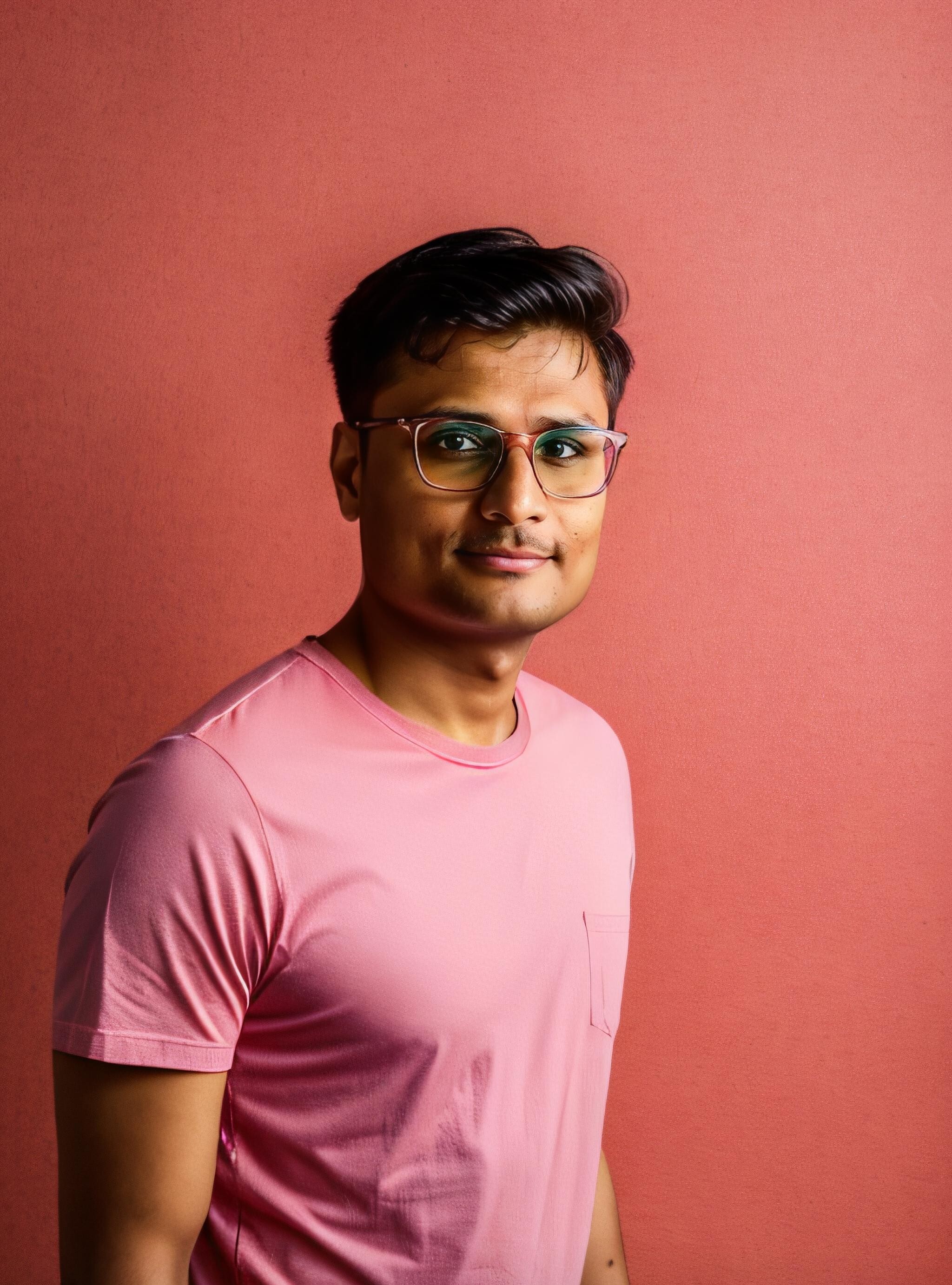