Mastering Control Flow in JavaScript: A Comprehensive Guide
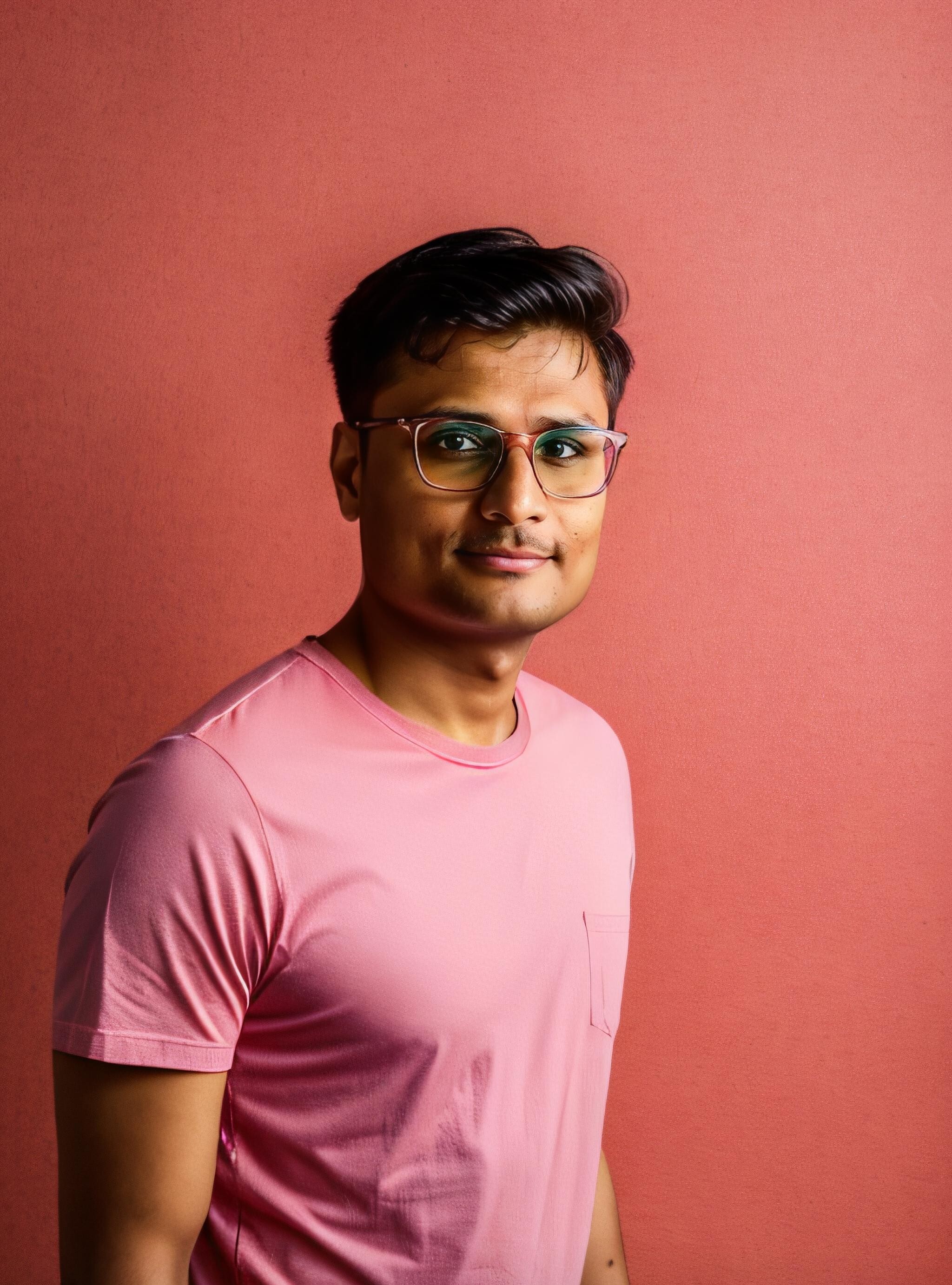
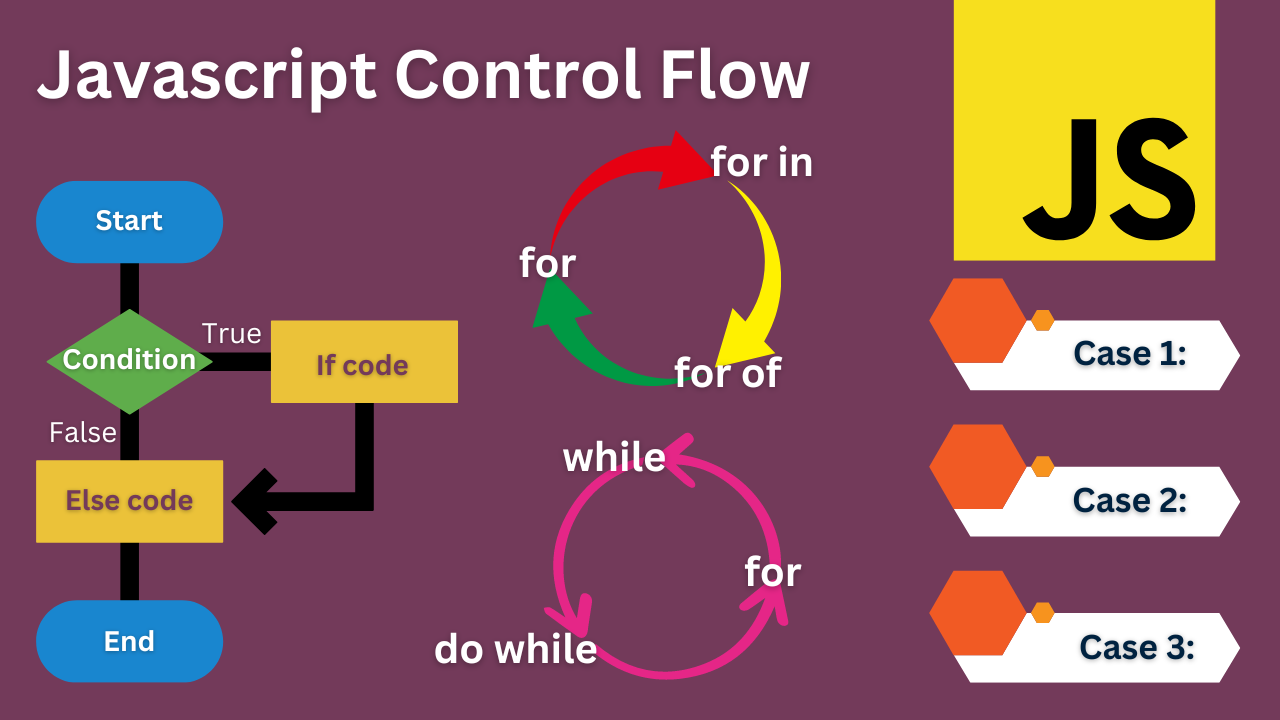
Conditional statements
Conditional statements are used to execute different blocks of code based on certain conditions.
The
if
statement is the most common conditional statement in JavaScript.
let age = 18;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
- You can add additional conditions using
else if
.
let grade = 85;
if (grade >= 90) {
console.log("A");
} else if (grade >= 80) {
console.log("B");
} else if (grade >= 70) {
console.log("C");
} else {
console.log("Failed");
}
- The
switch
statement is another way to handle multiple conditions.
let day = 3;
switch (day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
default:
console.log("Invalid day");
}
Truthy and falsy values:
In JavaScript, values are implicitly coerced to booleans when used in conditional statements.
Falsy values:
false
,0
,0
,0n
,null
,undefined
,NaN
,''
(empty string)All other values are considered truthy.
let x = 0;
if (x) {
console.log("Truthy"); // This block will not execute
} else {
console.log("Falsy"); // This block will execute
}
Ternary operator:
The ternary operator is a shorthand way to write simple
if...else
statements.Syntax:
condition ? valueIfTrue : valueIfFalse
let age = 20;
let canVote = age >= 18 ? "Yes" : "No";
console.log(canVote); // Output: "Yes"
Loops:
Loops are used to execute a block of code repeatedly.
The
for
loop is used when you know the number of iterations.
for (let i = 0; i < 5; i++) {
console.log(i); // Output: 0 1 2 3 4
}
- The
while
loop is used when the number of iterations is unknown and depends on a condition.
let count = 0;
while (count < 5) {
console.log(count); // Output: 0 1 2 3 4
count++;
}
- The
do...while
loop is similar to thewhile
loop but executes the block at least once.
let count = 0;
do {
console.log(count); // Output: 0 1 2 3 4
count++;
} while (count < 5);
- The
for...in
loop is used to iterate over the properties of an object.
let person = { name: "Alice", age: 30 };
for (let prop in person) {
console.log(prop + ": " + person[prop]); // Output: name: Alice, age: 30
}
- The
for...of
loop is used to iterate over the values of an iterable object, such as an array.
let fruits = ["apple", "banana", "orange"];
for (let fruit of fruits) {
console.log(fruit); // Output: apple banana orange
}
Break and continue statements:
The
break
statement is used to exit a loop prematurely.The
continue
statement is used to skip the current iteration of a loop.
for (let i = 0; i < 10; i++) {
if (i === 5) {
break; // Exit the loop when i is 5
}
console.log(i); // Output: 0 1 2 3 4
}
for (let i = 0; i < 10; i++) {
if (i === 5) {
continue; // Skip the iteration when i is 5
}
console.log(i); // Output: 0 1 2 3 4 6 7 8 9
}
Now that you’ve grasped the fundamentals of control flow in JavaScript, including conditional statements, truthy and falsy values, loops, and control statements like break and continue, you’re ready to take your skills to the next level.
In the next article, we’ll dive deeper into the world of functions, exploring various types of functions, closures, recursion, and more. Stay tuned, and get ready to unlock the true potential of JavaScript!
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
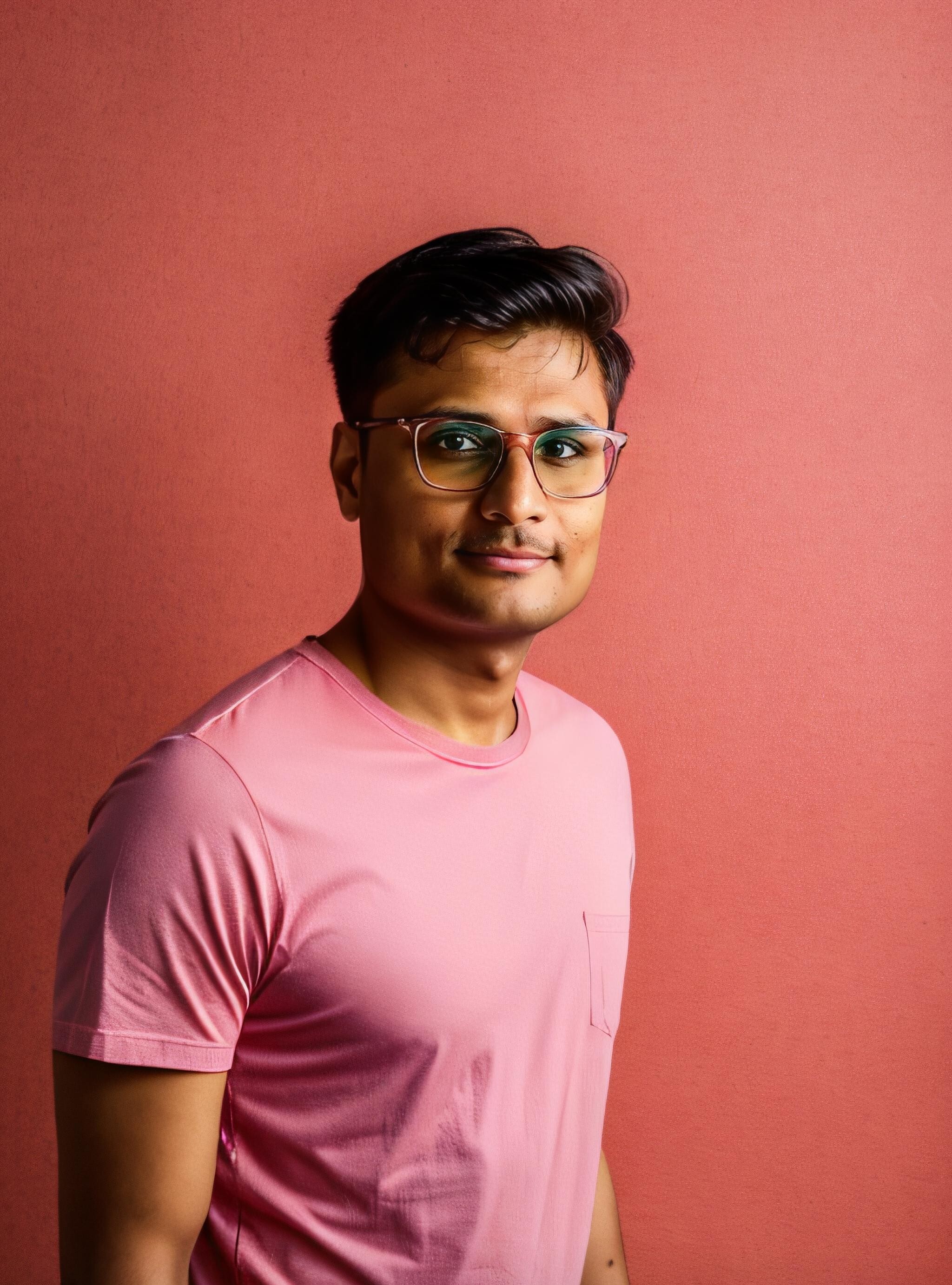