React useMemo Hook in Real Life๐ฏ
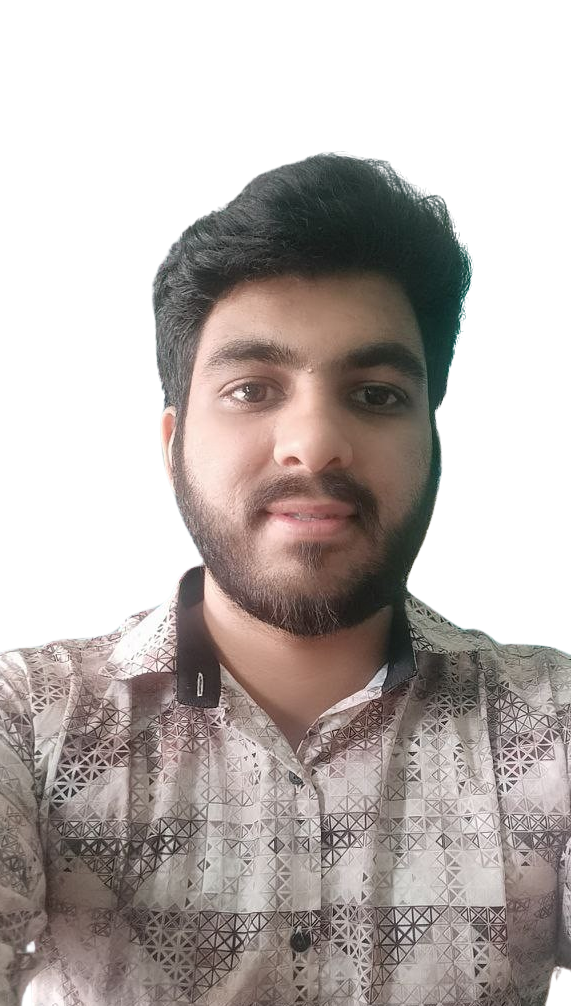
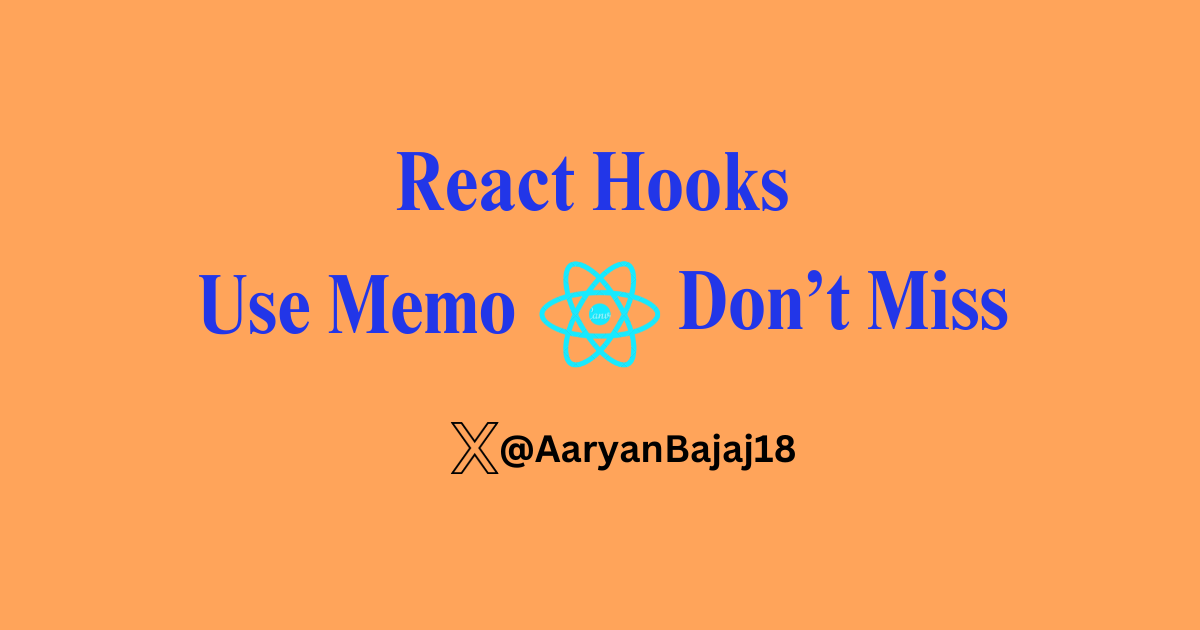
In the realm of React development, performance is paramount. Ensuring that your application runs efficiently and swiftly can be a challenge, especially when dealing with expensive calculations and complex UIs. Enter useMemo
, a powerful hook designed to optimize performance by memoizing expensive calculations.
Speed Up Your React App with useMemo
Imagine your app as a well-organized library. Every time you need a specific book, it's right where it should be, saving you the hassle of searching through stacks of unrelated volumes.
That's the essence of
useMemo
.By memoizing computed data,
useMemo
prevents unnecessary recalculations, keeping your app snappy and efficient.
Optimize Expensive Calculations with useMemo
Think of
useMemo
as a chef using a cookbook. The chef doesn't rewrite the recipe every time they cook; they reference it only when adding new ingredients.Similarly,
useMemo
recalculates values only when their dependencies change.This selective recalculation ensures that your app performs expensive operations only when necessary, optimizing its overall performance.
Mastering useMemo for React Optimization
Using
useMemo
effectively is akin to an architect meticulously planning a building.The architect uses tools only when necessary to ensure precision and efficiency.
Similarly, you should use
useMemo
only for expensive computations.Correctly managing dependencies is crucial to avoid wasted calculations and ensure your app runs smoothly.
Optimize Complex UIs with useMemo
If your React app feels slow, especially with large lists and datasets,
useMemo
is your ally.It's like an artist with a palette of paints, remembering the exact shades mixed previously so they don't have to recreate them each time.
This memory capability is invaluable for optimizing complex UIs, ensuring your app doesn't redo unnecessary work.
import React, { useState, useMemo } from 'react';
const ExpensiveCalculationComponent = ({ data }) => {
const computeExpensiveValue = (data) => {
// Simulate an expensive calculation
let result = 0;
for (let i = 0; i < data.length; i++) {
result += data[i];
}
return result;
};
const expensiveValue = useMemo(() => computeExpensiveValue(data), [data]);
return <div>Expensive Value: {expensiveValue}</div>;
};
const App = () => {
const [data, setData] = useState([1, 2, 3, 4, 5]);
return (
<div>
<ExpensiveCalculationComponent data={data} />
<button onClick={() => setData([...data, data.length + 1])}>Add Data</button>
</div>
);
};
export default App;
In this example, useMemo
ensures that the expensive calculation is only performed when the data array changes, optimizing the component's performance.
Conclusion
Mastering useMemo
is a game-changer for optimizing React applications. By preventing unnecessary recalculations and efficiently managing dependencies, you can keep your app fast and responsive. Remember, like a well-organized library or a meticulous architect, strategic use of useMemo
can transform your app's performance.
Thank you for exploring the power of useMemo
! I hope this guide has provided valuable insights and practical tips to optimize your React applications. If you found this blog helpful, please share it with your fellow developers and join the conversation in the comments below.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
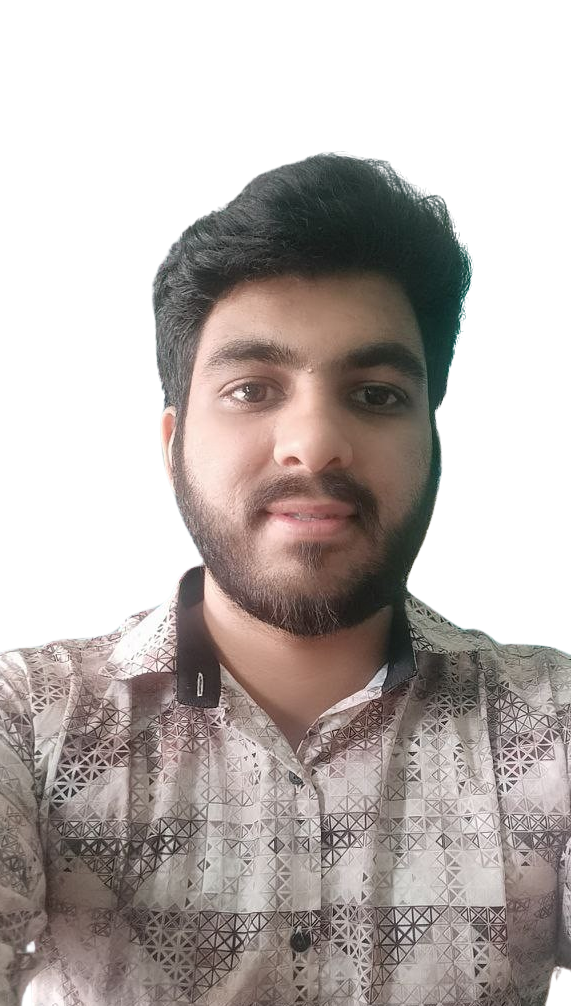
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone ๐๐ป , I'm Aaryan Bajaj , a Full Stack Web Developer from India(๐ฎ๐ณ). I share my knowledge through engaging technical blogs on Hashnode, covering web development.