Enhancing Security in Your E-Commerce Platform: Integrating AWS Cognito with Your React Application [Part - 1]
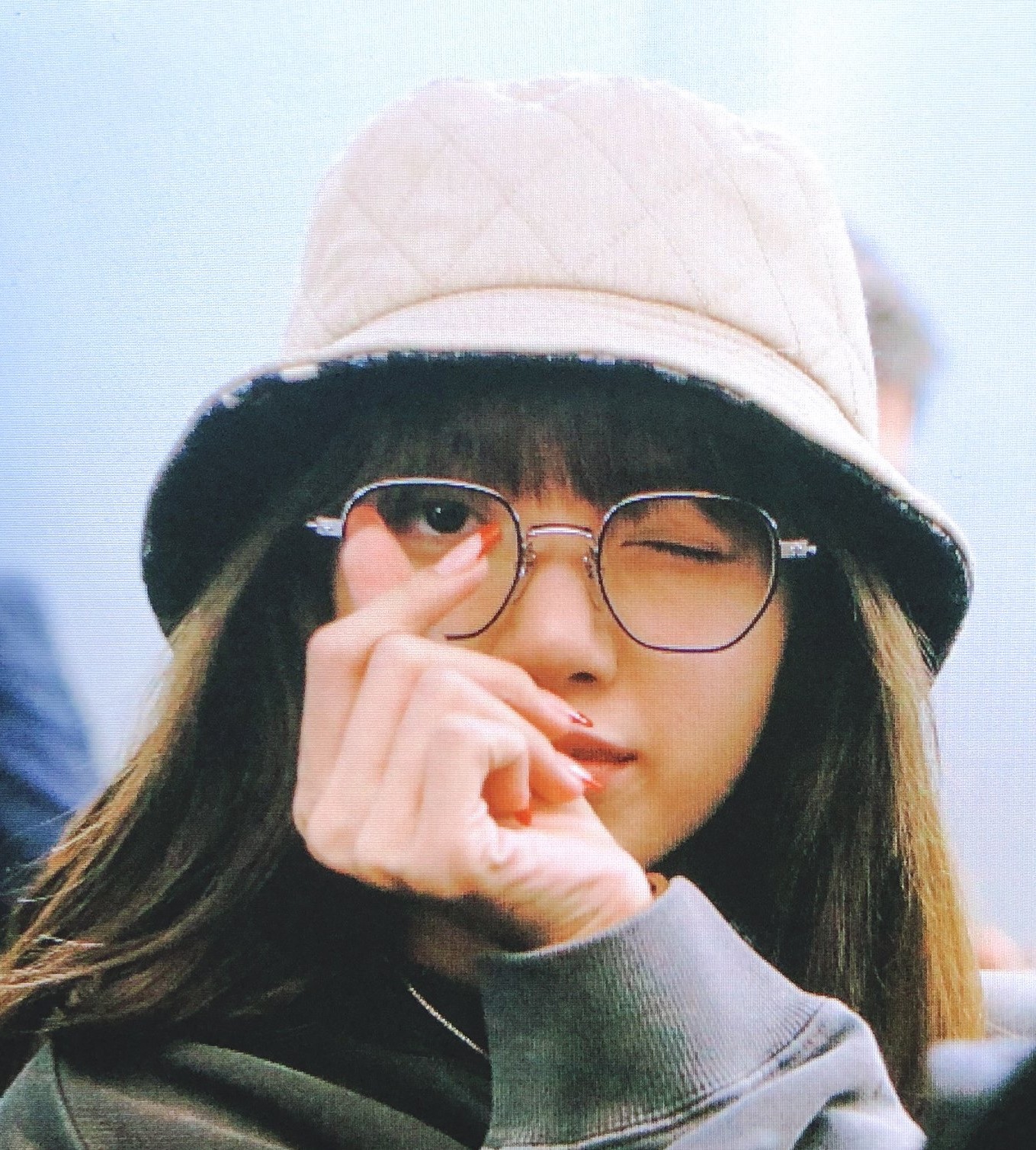
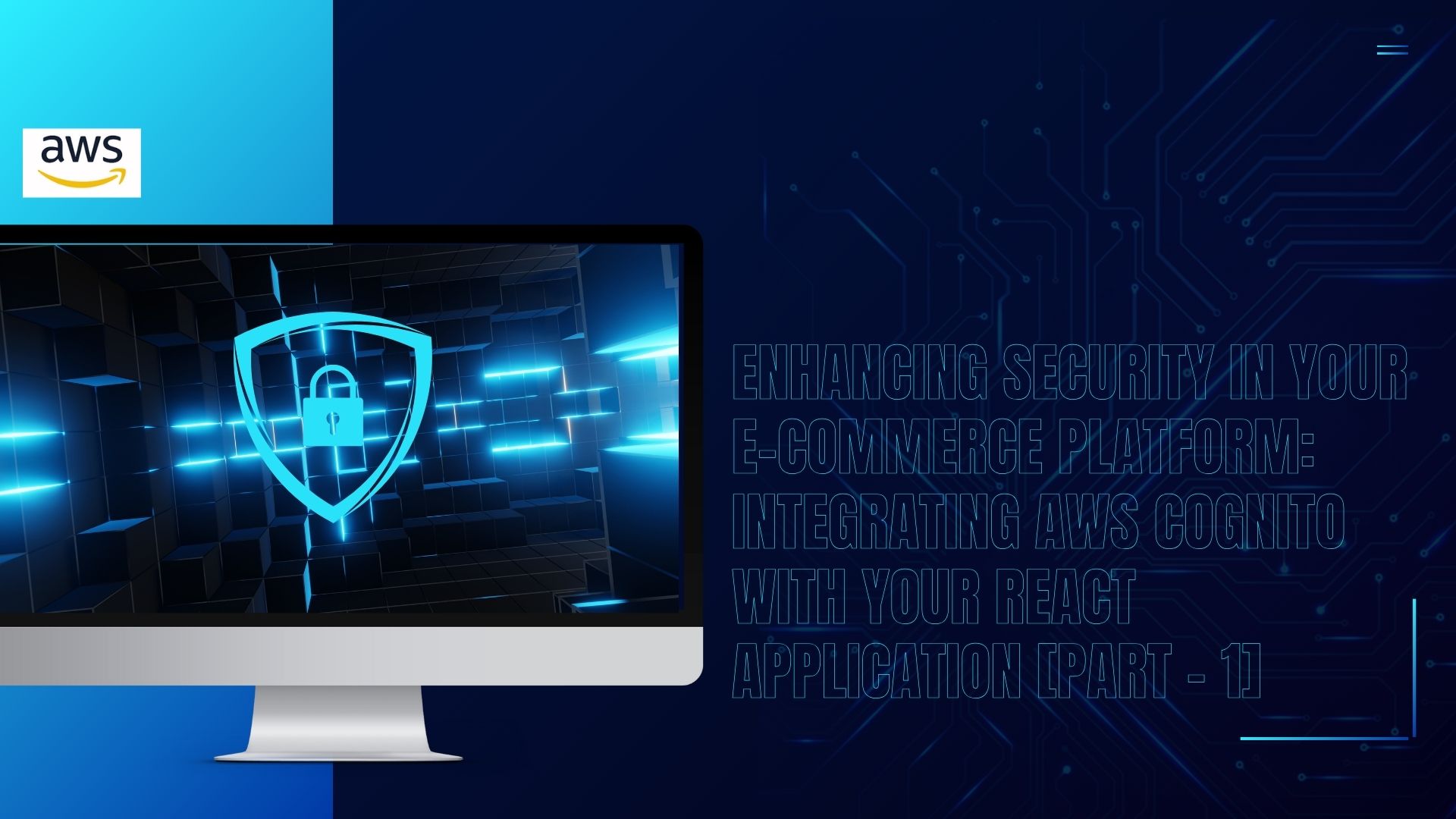
Introduction:
Welcome back to the journey of architecting the future of e-commerce. In this installment, we delve into the cornerstone of user trust and security: authentication. Join me as I explore the intricacies of implementing user authentication using AWS Cognito, the foundation of my e-commerce platform's security infrastructure.
Why AWS Cognito?
Why did I choose AWS Cognito for user authentication? Because it’s the Swiss Army knife of authentication solutions. It covers everything from user registration to multifactor authentication, ensuring comprehensive security for digital identities. It's like having a security team that never sleeps.
Amazon Cognito provides a simple yet effective way to manage user identities and sync data across devices. It keeps app data secure and available, both online and offline, offering a smooth user experience. Integration with major login providers like Amazon, Facebook, and Google makes identity creation easy and enhances security.
React JS
ReactJS, a Facebook creation, is a JavaScript library for creating dynamic web interfaces using a component-based system. Components, self-contained units that render HTML, can be reused and combined to build complex UIs. React also allows components to manage their own data ("state"), enhancing single-page applications' responsiveness to user actions or data changes.
When you dive into advanced React, you deal with state management using Redux or Context API, use React Hooks for functional components, optimize performance, and integrate with SSR/SSG technologies for high-performance apps. It's like upgrading from a regular car to a high-speed race car.
Key Insights
Integrating AWS Cognito into a React application offers significant benefits for developers working on e-commerce platforms. It simplifies user authentication, providing a comprehensive suite of features including user registration, multifactor authentication, and seamless integration with major login providers. This not only enhances the security of user identities but also streamlines the user registration process, making it easier for customers to engage with the platform. Additionally, AWS Cognito's ability to store app-specific data in the AWS Cloud eliminates the need for backend coding or infrastructure management, allowing developers to focus on enhancing app experiences.
Prerequisites
Working React App [Getting Started]
Active AWS account with Administrative Privileges [Sign up now]
JavaScript Fundamentals [Check Here]
Setting up AWS Cognito
Here is a step-by-step guide to help you set up Amazon Cognito.
step 1: Open Amazon Cognito Console (Start Here)
For Further Steps, you can visit the link [Here]
Setup React JS App
Step 1: Install required dependencies
- amazon-cognito-identity-js is an SDK that enables JavaScript applications to sign up, authenticate users, view, delete, and update user attributes within the Amazon Cognito Identity service.
npm install amazon-cognito-identity-js
- react-router-dom is a lightweight, fully-featured client-side and server-side routing library for React to build user interfaces. It operates wherever React does, including on the web, on servers with Node.js, and in React Native environments.
npm install react–router–dom
Step 2: Configure AWS Cognito in Your Application
In the /src
directory, create a UserPool.js
file and insert the provided code. Remember to substitute your User Pool ID and Client ID, which can be obtained from AWS Cognito.
import { CognitoUserPool } from "amazon-cognito-identity-js";
const poolData = {
UserPoolId: "Enter your Userpool ID",
ClientId: "Enter your Client ID",
};
const userPool = new CognitoUserPool(poolData);
export default userPool;
Implement User Registration
Step 3: Implement User Registration
Having AWS Cognito set up, you're ready to add user registration functionality. Go ahead and create a Components
folder inside your src
directory. Then, within this folder, add a new file named Register.js
. This file will contain the code for your registration component.
Here's code snippet that handles user registration:
- Imports and State Initialization
import { CognitoUser, CognitoUserAttribute } from 'amazon-cognito-identity-js';
import { useState } from 'react';
import UserPool from '../../UserPool';
function Register() {
const [username, setUsername] = useState('');
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [verifyProcess, setVerifyProcess] = useState(false);
const [OTP, setOTP] = useState('');
// ...
}
- User Registration:
const onSubmit = (e) => {
e.preventDefault();
const attributeList = [];
attributeList.push(
new CognitoUserAttribute({ Name: 'email', Value: email })
);
UserPool.signUp(username, password, attributeList, null, (err, data) => {
if (err) {
console.log(err);
alert("Couldn't sign up");
} else {
console.log(data);
setVerifyProcess(true);
alert('User Added Successfully');
}
});
};
- Account Verification:
const verifyAccount = (e) => {
e.preventDefault();
const user = new CognitoUser({ Username: username, Pool: UserPool });
user.confirmRegistration(OTP, true, (err, data) => {
if (err) {
console.log(err);
alert("Couldn't verify account");
} else {
console.log(data);
alert('Account verified successfully');
window.location.href = '/login';
}
});
};
- Render Method:
return (
<div className="register">
<h2>Create your account</h2>
<h3>Begin shopping, join us today!</h3>
{verifyProcess === false ? (
<form onSubmit={onSubmit}>
UserName
<input
type="text"
value={username.toLowerCase().trim()}
onChange={(e) => setUsername(e.target.value)}
/>
<br />
Email
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<br />
Password
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<br />
<button className="reg" type="submit">Register</button>
</form>
) : (
<form onSubmit={verifyAccount}>
Enter the OTP:
<input
type="text"
value={OTP}
onChange={(e) => setOTP(e.target.value)}
/>
<br />
<button type="submit">Verify</button>
</form>
)}
</div>
);
}
export default Register;
The code imports necessary components and initializes state variables. The onSubmit
function registers a user with Cognito User Pools by creating a CognitoUserAttribute
for the user's email and calling the signUp
method.
The verifyAccount
function verifies the user's account by creating a CognitoUser
instance and calling the confirmRegistration
method with the provided OTP. If successful, the user is redirected to the login page.
Step 4: Configuring Route for Registration Page
In App.js, firstly, import the Register
component from its respective module located at ./Components/Register
.
Next, set up a route for the registration page by assigning /register
as the path and <Register />
as the component to render when this path is accessed.
import { BrowserRouter, Route, Routes } from 'react-router-dom';
import './App.css';
import Register from './Components/Register';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="/register" element={<Register />} />
</Routes>
</BrowserRouter>
);
}
export default App;
Upon visiting http://localhost:3000/register
, the application will automatically display the Register
component, allowing users to initiate the registration process.
Step 5: Run and Access the Registration Page
You can now start running the application with the following command.
npm start
Navigate to the local development site at http://localhost:3000/register
to see the details displayed.
After filling in the registration details and clicking the Register button, proceed to enter the OTP received via email and click on the Verify button to confirm your registration with AWS Cognito.
Step 6: Verify with AWS Cognito
Now check the users tab in Cognito Console to check the registered user list.
Conclusion
In this segment, I highlighted the essential role of user authentication in bolstering the security and reliability of my e-commerce platform, achieving seamless user registration through the development of a registration component within my serverless React.js application, powered by AWS Cognito. Looking forward, my future endeavors will delve into the intricacies of user login processes, leveraging AWS Cognito alongside React.js and the 'amazon-cognito-identity-js' library, showcasing AWS Cognito's adaptability in various operational aspects such as integration with AWS AppSync, authentication with external entities, and safeguarding AWS services via identity pools.
Subscribe to my newsletter
Read articles from Sri Durgesh V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
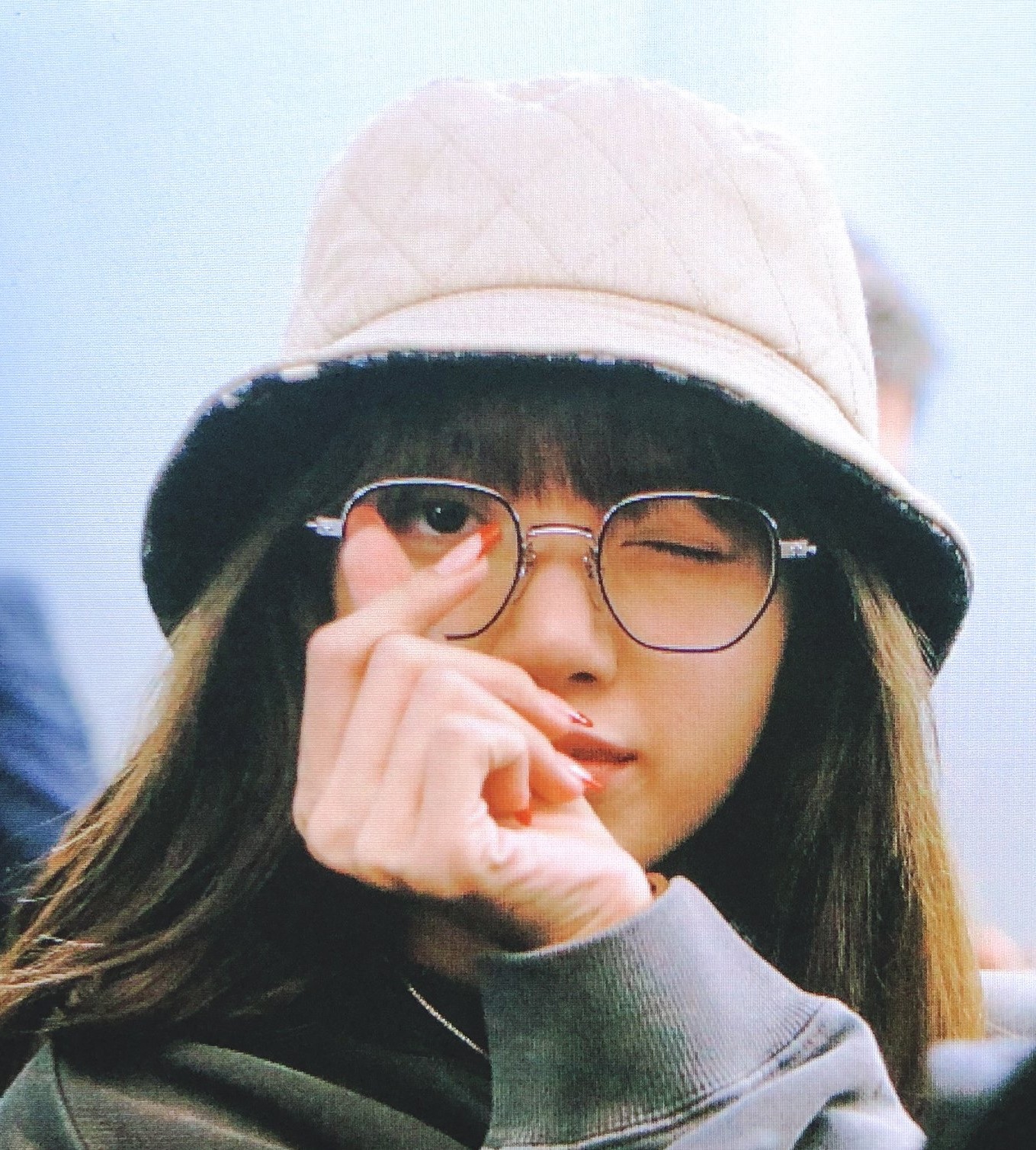
Sri Durgesh V
Sri Durgesh V
As a Cloud Enthusiast, this blog documents my journey with Amazon Web Services (AWS), sharing insights and project developments. Discover my experiences and learn useful information about different AWS cloud services.