Practical JavaScript Date and Time Handling for Web Developers

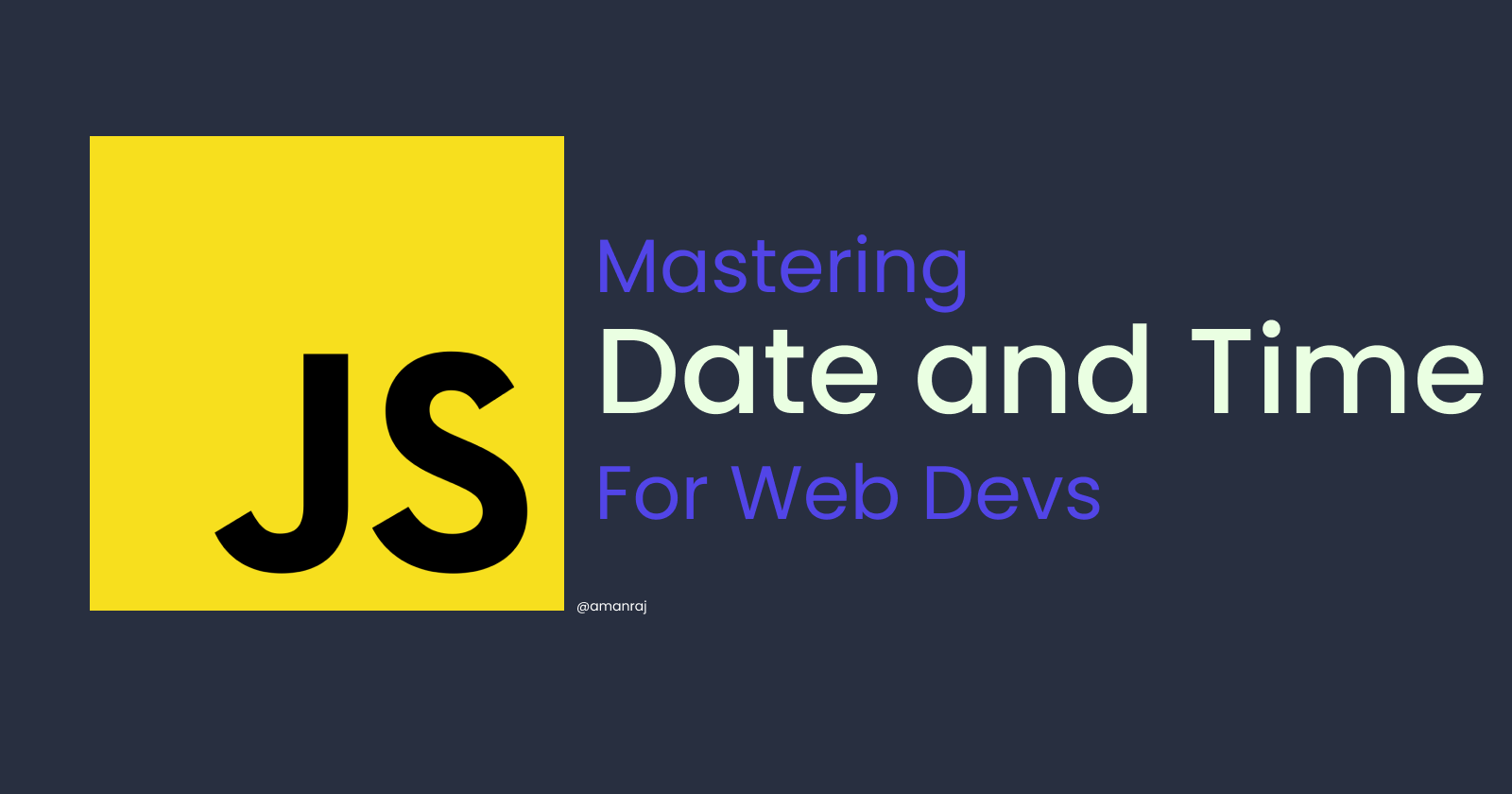
As a web developer many time we need to access the current time and date in out code to show in the interface or to save some activity timestamps data.
In this article we are going to understand dates and times in JavaScript and master it so lets get started.
Accessing the current date and time.
to access dates and time there is a Date()
Object in JavaScript
let now = new Date();
console.log(now);
Output: 2024-05-17T12:21:40.198Z
in this JavaScript code snippet we are initiating the Date()
object using new
keyword this creates a instance🤔 for the object and this has access of all properties and methods defined by the date prototype.
console.log(typeof myDate);
// Output: object
🤔Instance in JavaScript is a specific object created from a class, containing its own unique data while sharing the structure and behavior defined by the class.
can i pass arguments to Date() ??🤔
yes you can You can also create a Date
object for a specific date and time by passing arguments to the Date
constructor.
let specificDate = new Date('2024-05-17T10:30:00');
console.log(specificDate);
Output: 2024-05-17T05:00:00.000Z
how can i access only year? or month? or day?
Date
object comes with various methods to get and set different parts of the date and time, such as getFullYear()
, getMonth()
, setDate()
, etc.
let date = new Date();
console.log(date.getFullYear());
Output: 2024
console.log(date.getMonth() + 1);
Output: 5 (current month)
// Add 1 to get the correct month value because JS counts from 0 to 11 month
let date = new Date('2024-05-15');
Output: May 15, 2024
date.setDate(20);
// Sets the day to 20
console.log(date);
Outputs: Mon May 20 2024 00:00:00 GMT
note: here you can give any number in date month is max of 31 days so it will start from next month you can also give -ve number as argument it will count back!!
simply the argument is just count of day till end of month then counts day of next or prev month!!
eg.
let date = new Date('2024-05-15');
// May 15, 2024
date.setDate(-1);
// Sets the date to April 29, 2024
console.log(date);
// Outputs: Tue Apr 29 2024 00:00:00 GMT
Now how can i access only current time? 🤔
to extracting time from Date()
object we can use some methods getHours()
, getMinutes()
, and getSeconds()
to get the current hours, minutes, and seconds.
let now = new Date();
let hours = now.getHours();
let minutes = now.getMinutes();
let seconds = now.getSeconds();
To display the time in a readable format, you might want to format these components into a string. Here’s a simple way to format it as HH:MM:SS
:
let formattedTime = now.toLocaleTimeString();
console.log(formattedTime);
//Outputs the current time, e.g., "14:05:09 pm
"
Some more ways to accessing time in different format:
let myDate = new Date()
console.log(myDate.toString()); // Fri May 17 2024 19:15:06 GMT+0530 (India Standard Time)
console.log(myDate.toDateString()); //Fri May 17 2024
console.log(myDate.toLocaleString()); //17/5/2024, 7:15:06 pm
More simiar Date() methods:
getDate(), getDay(), getFullYear(), getHours(), getMilliseconds(), getMinutes(), getMonth(), getSeconds(), getTime(), getUTCDate(), getUTCDay(), getUTCFullYear(), getUTCHours(), getUTCMilliseconds(), getUTCMinutes(), getUTCMonth(), getUTCSeconds(), setDate(), setFullYear(), setHours(), setMilliseconds(), setMinutes(), setMonth(), setSeconds(), setTime(), setUTCDate(), setUTCFullYear(), setUTCHours(), setUTCMilliseconds(), setUTCMinutes(), setUTCMonth(), setUTCSeconds(), toDateString(), toISOString(), toJSON(), toLocaleDateString(), toLocaleTimeString(), toLocaleString(), toString(), toTimeString(), toUTCString()
Bonus: we can also pass parameters in the toLocaleString()
method to format the date string according to specific preferences.
eg.
const date = new Date();
const options = {
weekday: "long",
year: "numeric",
month: "long",
day: "numeric", };
console.log(date.toLocaleString("en-US", options));
This will output the current date in the long format, like "Monday, May 17, 2024
", using English language and United States conventions.
We have understood Date()
object here and we can now perform any task related to date and time in JavaScript. 🧑🏿💻
Thanks for reading! If you enjoyed this article, consider following to my Hashnode blog account for more updates and insightful content. Feel free to leave a comment below sharing your thoughts, questions, or feedback. Let's stay connected!
Follow me on X | Connect on LinkedIn | Visit my GitHub
Happy Coding🎉
Copyright ©2024 Aman Raj. All rights reserved.
Subscribe to my newsletter
Read articles from Aman Raj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aman Raj
Aman Raj
I’m a full-stack developer and open-source contributor pursuing B.Tech in CSE at SKIT, Jaipur. I specialize in building scalable web apps and AI-driven tools. With internship experience and a strong portfolio, I’m actively open to freelance projects and remote job opportunities. Let’s build something impactful!