Unleash the Power of Search in Your Laravel App with Scout
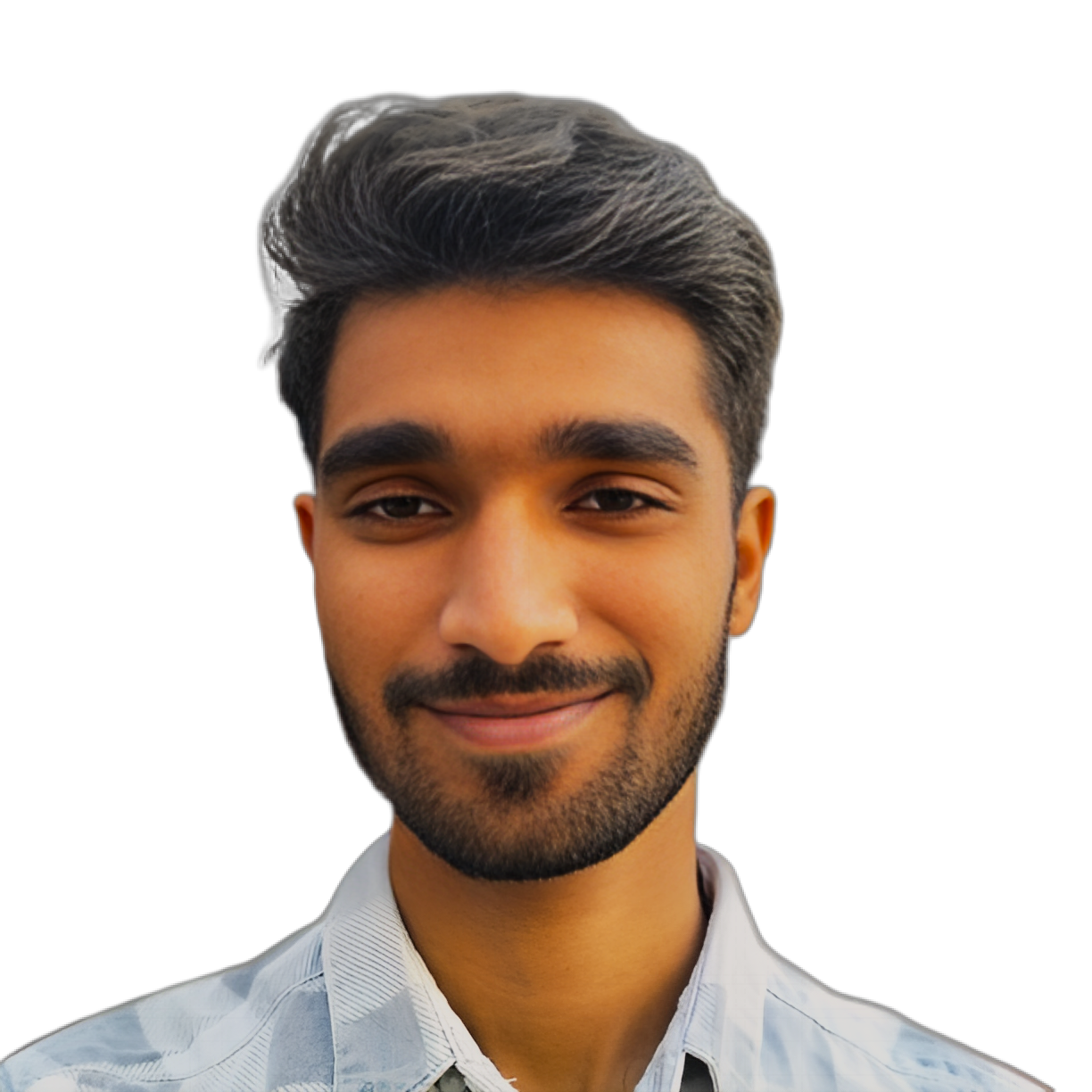

In today's digital landscape, a seamless search experience is no longer a luxury; it's an expectation. Users want to find what they need quickly and effortlessly, and for Laravel developers, this often translates to wrestling with complex search logic. But fear not, fellow coders, for there's a hero in our midst: Laravel Scout!
Scout is a fantastic tool that simplifies the process of adding full-text search functionality to your Laravel applications. With Scout, you can ditch the headaches of managing intricate search algorithms and focus on building features that truly matter to your users.
How Does Scout Work its Magic?
Scout's magic lies in its ability to connect your Laravel application to various search backends through drivers. Think of drivers as adapters that allow Scout to communicate with different search engines. Popular options include cloud-based solutions like Algolia and Meilisearch, or you can even leverage the full-text search capabilities of your existing database (MySQL or PostgreSQL).
But that's not all! Scout also employs the power of Model Observers. These observers act as silent guardians, automatically keeping your search indexes synchronized with your application data. Whenever you create, update, or delete a model instance, Scout's observers ensure the corresponding search indexes are updated in real-time, guaranteeing users always have access to the most relevant results.
Why Choose Laravel Scout?
The benefits of using Laravel Scout are numerous, making it a valuable asset for any Laravel developer:
Effortless Search Integration: Scout streamlines the process of adding search functionality, saving you valuable development time. No more building complex search logic from scratch!
Enhanced User Experience: Your users will be delighted with the lightning-fast and accurate search results powered by Scout.
Unmatched Scalability: Scout scales effortlessly as your application grows and your data volume increases.
Developer Productivity Boost: Scout empowers developers by freeing them from the burden of building and maintaining custom search solutions, allowing them to focus on core application features.
Getting Started with Laravel Scout: A Step-by-Step Tutorial
Now that you're eager to unleash the power of Scout in your Laravel application, let's dive into a step-by-step tutorial to get you started:
1. Installation:
The first step is to add Scout to your Laravel project using Composer:
Bash
composer require laravel/scout
2. Driver Configuration:
Scout utilizes drivers to connect to various search backends. Here's an example of configuring the Algolia driver within your config/scout.php
file:
PHP
'default' => [
'driver' => 'algolia',
'options' => [
'id' => env('ALGOLIA_APP_ID'),
'secret' => env('ALGOLIA_SECRET'),
],
],
Remember to replace ALGOLIA_APP_ID
and ALGOLIA_SECRET
with your actual Algolia credentials.
3. Making Your Models Searchable:
To enable search functionality for a model, add the Searchable
trait to your model class:
PHP
<?php
namespace App\Models;
use Laravel\Scout\Searchable;
class Post extends Model
{
use Searchable;
// ... other model properties and methods
}
4. Defining Searchable Attributes:
By default, Scout will search all text columns in your model. However, you can specify which attributes to include in the search using the toSearchableUsing
method:
PHP
public function toSearchableUsing()
{
return [
'title',
'content',
];
}
5. Performing Searches:
Scout provides a convenient interface for performing searches on your models. Here's an example of searching for posts containing the term "Laravel":
PHP
$results = Post::search('Laravel')->get();
This code snippet will search the title
and content
attributes of your Post
model for the term "Laravel" and return a collection of matching results.
6. Customization and Advanced Features:
Scout offers a wide range of customization options and advanced features beyond the scope of this basic tutorial. We highly recommend exploring the comprehensive Laravel Scout documentation for a deeper dive: https://laravel.com/docs/11.x/scout
Conclusion
Laravel Scout is a powerful tool that simplifies adding robust search functionality to your Laravel applications. With its user-friendly approach and extensive capabilities, Scout empowers you to create a seamless search experience that keeps your users happy and engaged. So, what are you waiting for? Start integrating Scout into your Laravel projects today and unleash the power of search!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
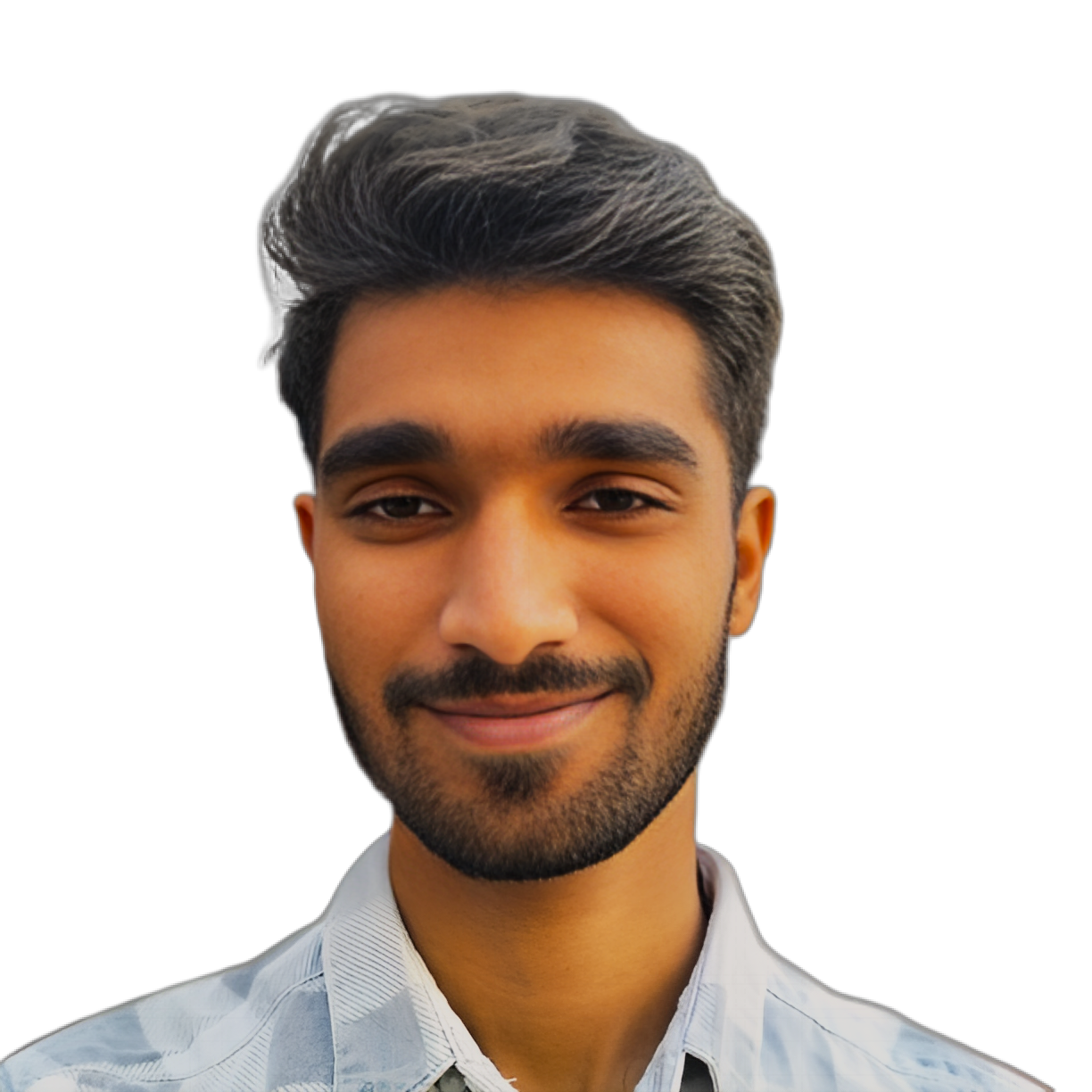
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.