Building a Scalable Component Library in React: Best Practices and Pitfalls

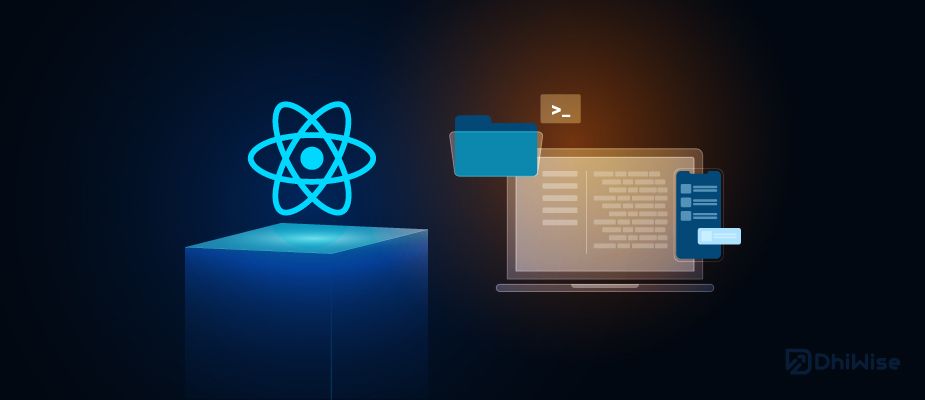
Introduction:
Creating a scalable component library in React is a game-changer for modern web development. It empowers teams to build consistent, reusable, and maintainable UI components that can be leveraged across multiple projects. However, crafting such a library requires a strategic approach to ensure scalability and efficiency. In this blog post, we will explore the best practices and common pitfalls to watch out for when building a scalable component library in React.
Best Practices for Building a Scalable Component Library
Component Modularity:
Principle: Design components to be highly modular, focusing on single responsibilities. Each component should do one thing and do it well.
Implementation: Break down large components into smaller, reusable subcomponents. For example, a complex form component can be divided into smaller input, button, and validation components.
Consistent Styling:
Principle: Maintain a consistent look and feel across your component library by using a unified styling approach.
Implementation: Use CSS-in-JS solutions like styled-components or Emotion, or opt for a CSS preprocessor like SASS. Ensure you have a centralized theme or style guide that all components adhere to.
Comprehensive Documentation:
Principle: Well-documented components make it easier for developers to understand and utilize them effectively.
Implementation: Use tools like Storybook to create an interactive component explorer and documentation site. Provide clear examples, prop definitions, and usage guidelines.
Prop Management:
Principle: Carefully manage and validate component props to ensure flexibility and robustness.
Implementation: Utilize TypeScript or PropTypes to enforce prop types and default values. Design components to accept a wide range of props while maintaining clarity and simplicity.
Accessibility:
Principle: Ensure that your components are accessible to all users, including those with disabilities.
Implementation: Follow WAI-ARIA guidelines and use accessibility testing tools like Axe. Implement keyboard navigation, screen reader support, and other accessibility best practices.
Testing and Quality Assurance:
Principle: Rigorous testing ensures that your components are reliable and perform well across different environments.
Implementation: Write unit tests using frameworks like Jest and React Testing Library. Implement visual regression testing with tools like Chromatic or Percy to catch unexpected changes.
Version Control and Semantic Versioning:
Principle: Manage component library versions systematically to handle updates and changes gracefully.
Implementation: Follow semantic versioning (semver) to communicate changes. Use tools like Lerna for managing mono repo and maintaining multiple packages.
Common Pitfalls to Avoid
Over-Engineering:
Issue: Trying to anticipate every possible use case can lead to overly complex and bloated components.
Solution: Start simple and iterate based on actual needs and feedback. Focus on creating flexible, composable components rather than one-size-fits-all solutions.
Neglecting Performance:
Issue: Poorly optimized components can degrade application performance, leading to slow load times and a sluggish user experience.
Solution: Optimize component rendering using techniques like memoization and lazy loading. Regularly profile and test performance, especially for critical and complex components.
Lack of Standardization:
Issue: Inconsistent naming conventions, styling methods, and coding practices can lead to a fragmented and difficult-to-maintain codebase.
Solution: Establish and enforce coding standards and guidelines. Conduct regular code reviews and ensure all contributors are aligned with the best practices.
Ignoring Scalability Concerns:
Issue: Failing to consider how the component library will scale can lead to challenges as the codebase grows.
Solution: Design components with reusability and extensibility in mind. Organize your library in a way that makes it easy to add new components without cluttering the existing structure.
Inadequate Documentation:
Issue: Poor or missing documentation can make it difficult for other developers to use and contribute to the component library.
Solution: Invest time in creating thorough and clear documentation. Update the documentation regularly as the library evolves.
Conclusion
Building a scalable component library in React requires careful planning, adherence to best practices, and an awareness of common pitfalls. By focusing on modularity, consistency, documentation, accessibility, and robust testing, you can create a powerful tool that enhances development efficiency and application quality. Avoiding common mistakes like over-engineering and neglecting performance will ensure that your component library remains a valuable asset as your projects grow and evolve. With the right approach, your component library will not only streamline your current development process but also pave the way for future innovation and scalability.
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.