To NOT or NOT to NOT NOT?: Why We Use !! In JavaScript
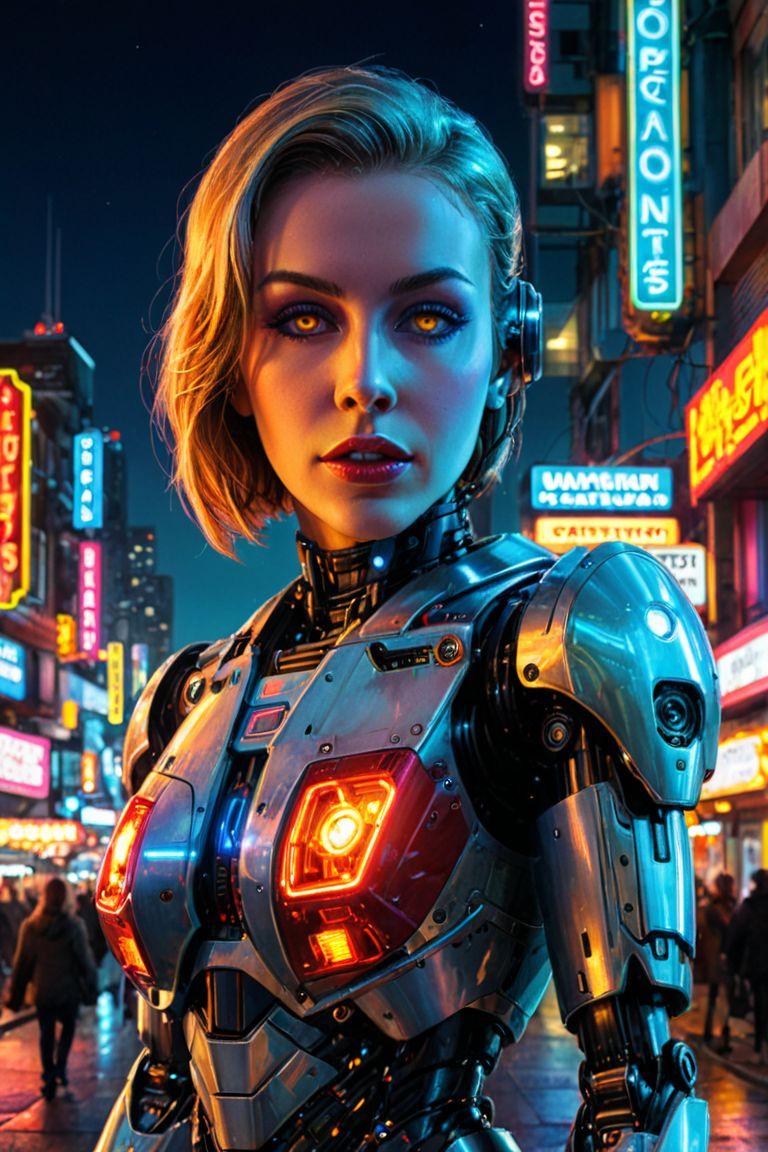
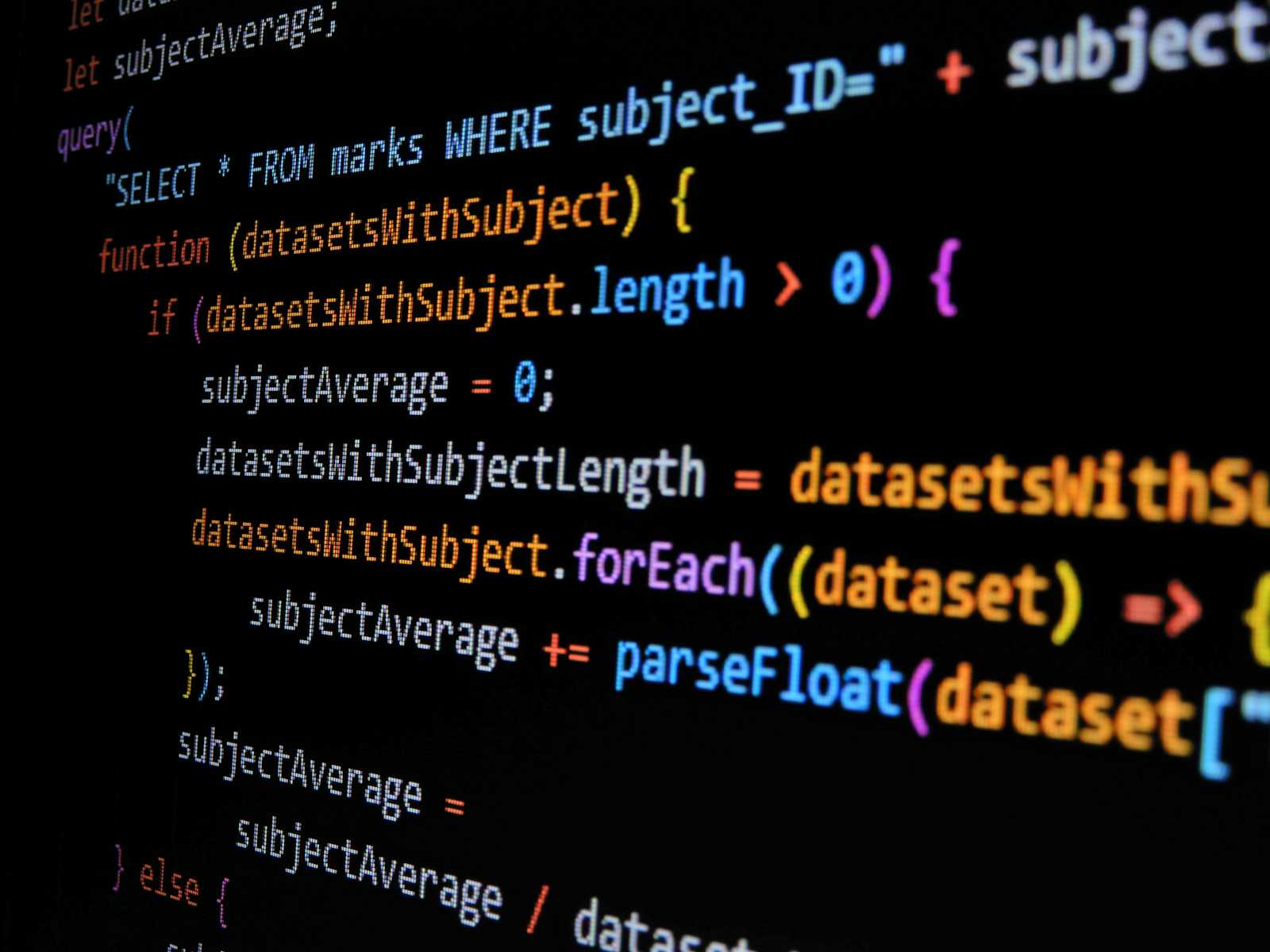
When I was working through my basic JavaScript fundamentals materials for my bootcamp with Flatiron School, I came upon the concept of logical operators for the first time.
Logical operators are fairly simple to understand. ! will operate on an expression and simply return the opposite of that expression's truthiness in a Boolean. If something is true, it will return as false. If something is false, it will return as true.
In short, ! is an operator that simply switches the truthiness/falsiness of something.
The idea of using !! (NOT NOT) is therefore even simpler to understand. If ! makes a truthy value falsey, using it twice will:
Make the truthy expression falsey, and...
Return the expression's Boolean form, which as a truthy expression, will simply return as "true."
It makes a lot of sense and is so simple in fact that the whole idea of !! had me completely puzzled. Have you ever encountered something so simplified that you figure the concept absolutely must be more complicated and you absolutely must be missing the point?
!! was that "something" for me.
So I got to thinking...
While this concept is extremely simple, I couldn't quite grasp why the heck we would ever need to use this. This likely won't be the last concept I encounter while learning JavaScript that seems at first to be entirely redundant.
So I did a little bit of digging about why we use !!, and the following is what I found.
Why Use !! in JavaScript?
JavaScript is full of neat tricks and shortcuts that can make coding easier and more efficient. One such trick is using the double exclamation mark (!!) to convert any value to its Boolean equivalent. In this post, we'll explore why and how you might use !! in your code, complete with examples to illustrate its usefulness.
Simplifying Code
Using !! is a quick and concise way to convert any value to a Boolean. This can make your code easier to read and write. Instead of writing Boolean(value), you can just write !!value.
Checking Truthiness in Conditions
When you need to ensure a value is explicitly converted to true or false, using !! can be very useful. For example, when checking the existence or validity of a variable before proceeding with some logic, !! ensures that the condition evaluates to a Boolean.
Ensuring Consistent Boolean Values
Sometimes, you might want to store a Boolean value rather than a truthy or falsy value. Using !! helps convert any variable to a strict true or false value.
Examples
To get a better understanding of this, here are a couple of examples...
Example 1: Validating User Input
What you want to validate if the user's input is not empty before processing it? Here's how that might look...
let userInput = prompt("Enter your name:");
let isValidInput = !!userInput;
if (isValidInput) {
console.log("Thank you for your input!");
} else {
console.log("Input cannot be empty.");
}
Here, we can use !!userInput to make sure that isValidInput is true if the user entered something, and false if they did not.
Example 2: Checking API Response
What if you wanted to check to see if an API response contained an error? Here's how you might use !! for that purpose...
let response = {
data: "Some data",
error: null
};
let hasError = !!response.error;
if (hasError) {
console.log("There was an error in the response.");
} else {
console.log("Response is successful.");
}
In this example, !!response.error converts null to false, and ensures that hasError is a Boolean. This makes the condition clear and straightforward.
It's pretty obvious after some further research and due diligence that !! really is as simple as it first seemed. What wasn't so obvious at first was the reasoning behind why we might need to use it.
And now we know!
Some source links...
Subscribe to my newsletter
Read articles from Makala O’Siggins directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
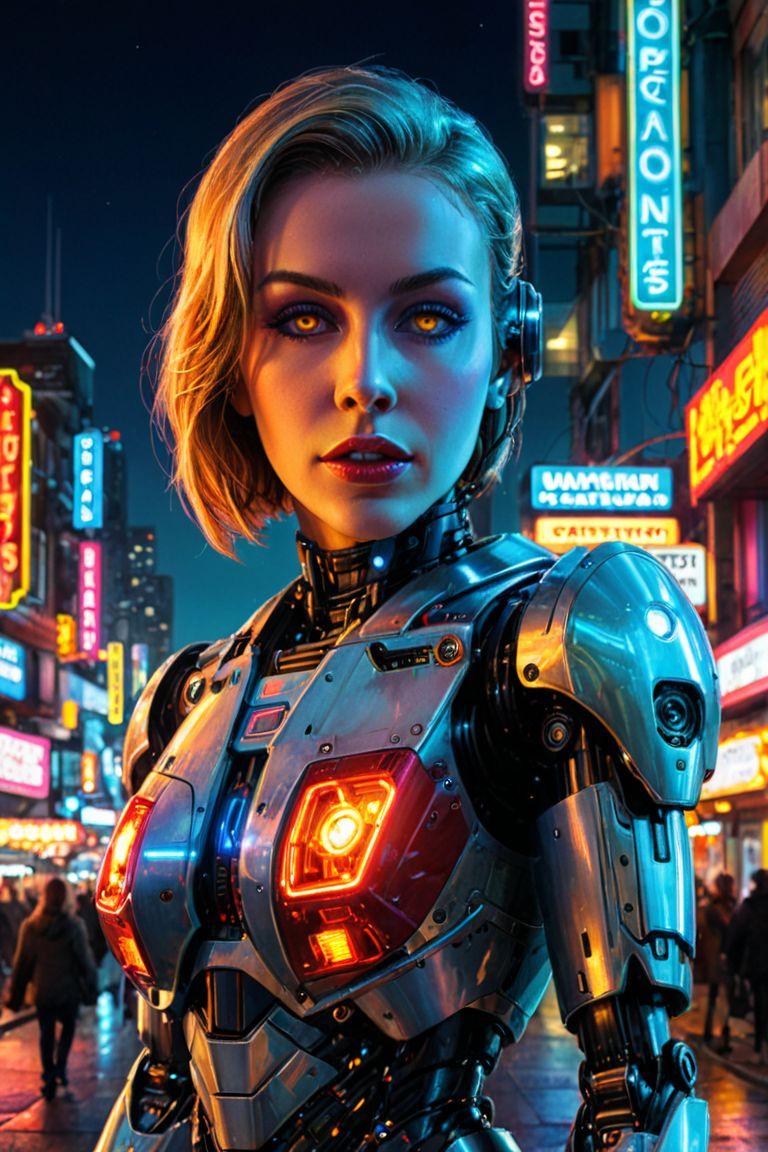