The Art of JavaScript Functions: A Comprehensive Exploration
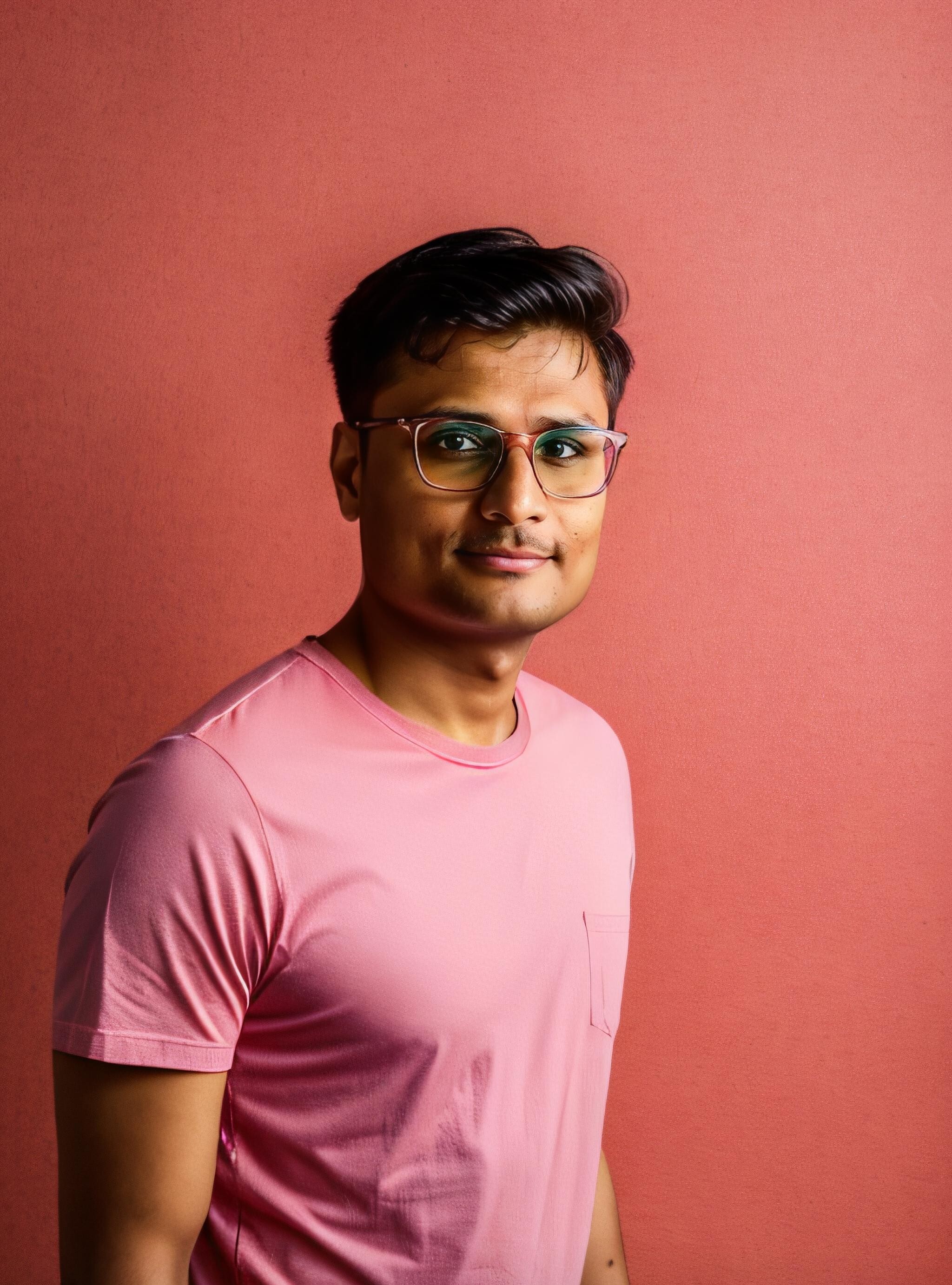

Functions are reusable blocks of code that encapsulate logic and perform specific tasks. We will understand functions with real-world web application examples that demonstrate the practical use of functions in JavaScript.
Function declaration and function expression:
- In JavaScript, you can define functions using two different syntaxes: function declarations and function expressions.
Function declaration:
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet("Alice"); // Output: Hello, Alice!
Function expression:
const greet = function(name) {
console.log(`Hello, ${name}!`);
};
greet("Bob"); // Output: Hello, Bob!
- The main difference is that function declarations are hoisted meaning they can be called before they are defined, while function expressions are not hoisted.
Let’s start by creating a simple web page with a button and a heading.
We’ll define a function to update the heading’s text when the button is clicked.
<!DOCTYPE html>
<html>
<head>
<title>Functions Example</title>
</head>
<body>
<h1 id="heading">Initial Title</h1>
<button id="updateBtn">Update Heading</button>
<script>
// Function declaration
function updateHeading() {
const heading = document.getElementById('heading');
heading.textContent = 'New Title';
}
// Function expression
const updateBtn = document.getElementById('updateBtn');
updateBtn.addEventListener('click', function() {
updateHeading();
});
</script>
</body>
</html>
Parameters and arguments:
Functions can accept input values called parameters.
When you call a function, you pass in values called arguments.
function multiply(a, b) { // a and b are parameters
return a * b;
}
console.log(multiply(3, 4)); // Output: 12 (3 and 4 are arguments)
Let’s create a simple calculator web application.
We’ll define a function that takes two numbers as parameters and performs an operation based on a third argument.
<!DOCTYPE html>
<html>
<head>
<title>Calculator Example</title>
</head>
<body>
<input type="number" id="num1" placeholder="Enter first number">
<input type="number" id="num2" placeholder="Enter second number">
<select id="operation">
<option value="add">Addition</option>
<option value="subtract">Subtraction</option>
<option value="multiply">Multiplication</option>
<option value="divide">Division</option>
</select>
<button id="calculateBtn">Calculate</button>
<h2 id="result"></h2>
<script>
function calculate(num1, num2, operation) {
let result;
switch (operation) {
case 'add':
result = num1 + num2;
break;
case 'subtract':
result = num1 - num2;
break;
case 'multiply':
result = num1 * num2;
break;
case 'divide':
result = num1 / num2;
break;
}
return result;
}
const calculateBtn = document.getElementById('calculateBtn');
calculateBtn.addEventListener('click', function() {
const num1 = parseFloat(document.getElementById('num1').value);
const num2 = parseFloat(document.getElementById('num2').value);
const operation = document.getElementById('operation').value;
const result = calculate(num1, num2, operation);
document.getElementById('result').textContent = `Result: ${result}`;
});
</script>
</body>
</html>
Return statement:
The
return
statement is used to return a value from a function.If no
return
statement is used, the function returnsundefined
.
function square(num) {
return num * num;
}
console.log(square(5)); // Output: 25
Let’s create a simple form validation example.
We’ll define a function that checks if a password meets certain requirements and returns a boolean value.
<!DOCTYPE html>
<html>
<head>
<title>Password Validation Example</title>
</head>
<body>
<input type="password" id="password" placeholder="Enter password">
<button id="validateBtn">Validate Password</button>
<p id="message"></p>
<script>
function validatePassword(password) {
const minLength = 8;
const hasUppercase = /[A-Z]/.test(password);
const hasLowercase = /[a-z]/.test(password);
const hasNumber = /\\d/.test(password);
return (
password.length >= minLength &&
hasUppercase &&
hasLowercase &&
hasNumber
);
}
const validateBtn = document.getElementById('validateBtn');
const messageElement = document.getElementById('message');
validateBtn.addEventListener('click', function() {
const password = document.getElementById('password').value;
const isValid = validatePassword(password);
if (isValid) {
messageElement.textContent = 'Password is valid!';
messageElement.style.color = 'green';
} else {
messageElement.textContent = 'Password is not valid. It must contain at least 8 characters, including uppercase, lowercase, and numbers.';
messageElement.style.color = 'red';
}
});
</script>
</body>
</html>
Arrow functions:
Arrow functions (or fat arrow functions) are a more concise syntax for writing function expressions.
They use the
=>
syntax and have an implicitreturn
for single-line expressions.
const greet = (name) => `Hello, ${name}!`;
console.log(greet("Charlie")); // Output: Hello, Charlie!
const multiply = (a, b) => a * b;
console.log(multiply(3, 4)); // Output: 12
Let’s create a simple to-do list application.
We’ll use arrow functions to define event handlers and array methods.
<!DOCTYPE html>
<html>
<head>
<title>To-Do List Example</title>
</head>
<body>
<input type="text" id="todoInput" placeholder="Enter a task">
<button id="addBtn">Add Task</button>
<ul id="todoList"></ul>
<script>
const todoInput = document.getElementById('todoInput');
const addBtn = document.getElementById('addBtn');
const todoList = document.getElementById('todoList');
const tasks = [];
addBtn.addEventListener('click', () => {
const task = todoInput.value.trim();
if (task) {
tasks.push(task);
todoInput.value = '';
renderTasks();
}
});
const renderTasks = () => {
todoList.innerHTML = '';
tasks.forEach((task, index) => {
const li = document.createElement('li');
li.textContent = task;
const deleteBtn = document.createElement('button');
deleteBtn.textContent = 'Delete';
deleteBtn.addEventListener('click', () => {
tasks.splice(index, 1);
renderTasks();
});
li.appendChild(deleteBtn);
todoList.appendChild(li);
});
};
</script>
</body>
</html>
Default parameters:
- You can set default values for parameters in case no arguments are provided.
function greet(name = "Anonymous") {
console.log(`Hello, ${name}!`);
}
greet(); // Output: Hello, Anonymous!
greet("David"); // Output: Hello, David!
Let’s create a simple greeting form.
We’ll define a function that takes a name as a parameter and uses a default value if no name is provided.
<!DOCTYPE html>
<html>
<head>
<title>Greeting Example</title>
</head>
<body>
<input type="text" id="nameInput" placeholder="Enter your name">
<button id="greetBtn">Greet</button>
<p id="greeting"></p>
<script>
function greet(name = 'Anonymous') {
return `Hello, ${name}!`;
}
const nameInput = document.getElementById('nameInput');
const greetBtn = document.getElementById('greetBtn');
const greetingElement = document.getElementById('greeting');
greetBtn.addEventListener('click', () => {
const name = nameInput.value.trim();
const greeting = greet(name);
greetingElement.textContent = greeting;
});
</script>
</body>
</html>
Rest and spread operators:
The rest operator (
...
) is used to collect multiple arguments into an array.The spread operator (
...
) is used to spread the elements of an array (or any iterable) into individual arguments.
function sum(...numbers) {
return numbers.reduce((total, num) => total + num, 0);
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5]; // [1, 2, 3, 4, 5]
Let’s create a simple shopping cart application.
We’ll use the rest operator to collect product prices as arguments and the spread operator to combine arrays.
<!DOCTYPE html>
<html>
<head>
<title>Shopping Cart Example</title>
</head>
<body>
<button id="addToCartBtn">Add to Cart</button>
<ul id="cartList"></ul>
<p id="total"></p>
<script>
const cartList = document.getElementById('cartList');
const totalElement = document.getElementById('total');
const cart = [];
function addToCart(...prices) {
cart.push(...prices);
renderCart();
}
function renderCart() {
cartList.innerHTML = '';
cart.forEach(price => {
const li = document.createElement('li');
li.textContent = `$${price.toFixed(2)}`;
cartList.appendChild(li);
});
const total = cart.reduce((sum, price) => sum + price, 0);
totalElement.textContent = `Total: $${total.toFixed(2)}`;
}
const addToCartBtn = document.getElementById('addToCartBtn');
addToCartBtn.addEventListener('click', () => {
addToCart(9.99, 14.99, 7.99);
});
</script>
</body>
</html>
Call, apply, and bind methods:
These methods are used to control the
this
value and pass arguments to functions.call
andapply
invoke the function immediately, whilebind
returns a new function with thethis
value bound.
const person = {
name: "Alice",
greet: function(message, name) {
console.log(`${message}, ${name}!`);
}
};
const greetAlice = person.greet.bind(person, "Hello"); // Bind `this` to `person` object
greetAlice("Bob"); // Output: Hello, Bob!
const greetEveryone = person.greet.bind(person, "Hi, everyone!");
greetEveryone(); // Output: Hi, everyone!
Let’s create a simple example that demonstrates the use of call
, apply
, and bind
methods.
We’ll define an object with a method and use these methods to control the this
value and pass arguments.
<!DOCTYPE html>
<html>
<head>
<title>Call, Apply, and Bind Example</title>
</head>
<body>
<button id="callBtn">Call Greet</button>
<button id="applyBtn">Apply Greet</button>
<button id="bindBtn">Bind Greet</button>
<p id="output"></p>
<script>
const person = {
name: 'Alice',
greet: function(greeting, punctuation) {
const message = `${greeting}, ${this.name}${punctuation}`;
document.getElementById('output').textContent = message;
}
};
const callBtn = document.getElementById('callBtn');
const applyBtn = document.getElementById('applyBtn');
const bindBtn = document.getElementById('bindBtn');
callBtn.addEventListener('click', function() {
person.greet.call({ name: 'Bob' }, 'Hello', '!');
});
applyBtn.addEventListener('click', function() {
person.greet.apply({ name: 'Charlie' }, ['Hi', '?']);
});
const boundGreet = person.greet.bind({ name: 'David' }, 'Welcome');
bindBtn.addEventListener('click', function() {
boundGreet('.');
});
</script>
</body>
</html>
Callback functions:
Callback functions are functions that are passed as arguments to other functions.
They are commonly used in asynchronous programming and event handling.
function greeting(name, callback) {
const message = `Hello, ${name}!`;
callback(message); // Invoke the callback function
}
function displayMessage(message) {
console.log(message);
}
greeting("Alice", displayMessage); // Output: Hello, Alice!
Let’s create a simple example that demonstrates the use of callback functions.
We’ll define a function that performs an asynchronous operation (simulated with setTimeout
) and invokes a callback function when it's completed.
<!DOCTYPE html>
<html>
<head>
<title>Callback Example</title>
</head>
<body>
<button id="loadDataBtn">Load Data</button>
<p id="data"></p>
<script>
function loadData(callback) {
setTimeout(function() {
const data = 'This is the loaded data.';
callback(data);
}, 2000);// Simulating an asynchronous operation
}
const loadDataBtn = document.getElementById('loadDataBtn');
const dataElement = document.getElementById('data');
loadDataBtn.addEventListener('click', function() {
loadData(function(data) {
dataElement.textContent = data;
});
});
</script>
</body>
</html>
Closures:
A closure is a function that has access to variables in its outer (enclosing) function’s scope, even after the outer function has finished executing.
Closures are used for data privacy, event handling, and creating private variables and methods.
function outerFunction(outerVar) {
const outerValue = `Outer value: ${outerVar}`;
function innerFunction(innerVar) {
const innerValue = `Inner value: ${innerVar}`;
console.log(`${outerValue} ${innerValue}`);
}
return innerFunction;
}
const myInnerFunction = outerFunction("Hello");
myInnerFunction("World"); // Output: Outer value: Hello Inner value: World
Let’s create a simple example that demonstrates the use of closures.
We’ll define an outer function that returns an inner function, which has access to the outer function’s variables, even after the outer function has finished executing.
<!DOCTYPE html>
<html>
<head>
<title>Closure Example</title>
</head>
<body>
<button id="incrementBtn">Increment</button>
<p id="count"></p>
<script>
function createCounter() {
let count = 0;
return function() {
count++;
document.getElementById('count').textContent = `Count: ${count}`;
}
}
const incrementCounter = createCounter();
const incrementBtn = document.getElementById('incrementBtn');
incrementBtn.addEventListener('click', incrementCounter);
</script>
</body>
</html>
Recursion:
Recursion is a programming technique where a function calls itself until a certain condition is met.
It’s commonly used to solve problems that can be broken down into smaller instances of the same problem.
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
console.log(factorial(5)); // Output: 120 (5 * 4 * 3 * 2 * 1)
Let’s create a simple example that demonstrates the use of recursion.
We’ll define a function that calculates the factorial of a number using recursion.
<!DOCTYPE html>
<html>
<head>
<title>Recursion Example</title>
</head>
<body>
<input type="number" id="numInput" placeholder="Enter a number">
<button id="calculateBtn">Calculate Factorial</button>
<p id="result"></p>
<script>
function factorial(n) {
if (n === 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
const numInput = document.getElementById('numInput');
const calculateBtn = document.getElementById('calculateBtn');
const resultElement = document.getElementById('result');
calculateBtn.addEventListener('click', function() {
const num = parseInt(numInput.value);
if (!isNaN(num) && num >= 0) {
const result = factorial(num);
resultElement.textContent = `The factorial of ${num} is ${result}.`;
} else {
resultElement.textContent = 'Please enter a valid non-negative number.';
}
});
</script>
</body>
</html>
Functions are a fundamental aspect of programming, and mastering them will allow you to write more modular, reusable, and maintainable code in JavaScript. By encapsulating logic into functions, you can create more organized and structured applications.
Now that you’ve mastered the intricacies of JavaScript functions, including declarations, expressions, parameters, arrow functions, closures, and recursion, you’re ready to dive into the next level of your JavaScript journey. In the upcoming article, we’ll explore the world of arrays, covering various array methods, destructuring, and advanced techniques for efficient data manipulation. Stay tuned, and get ready to supercharge your JavaScript skills!
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
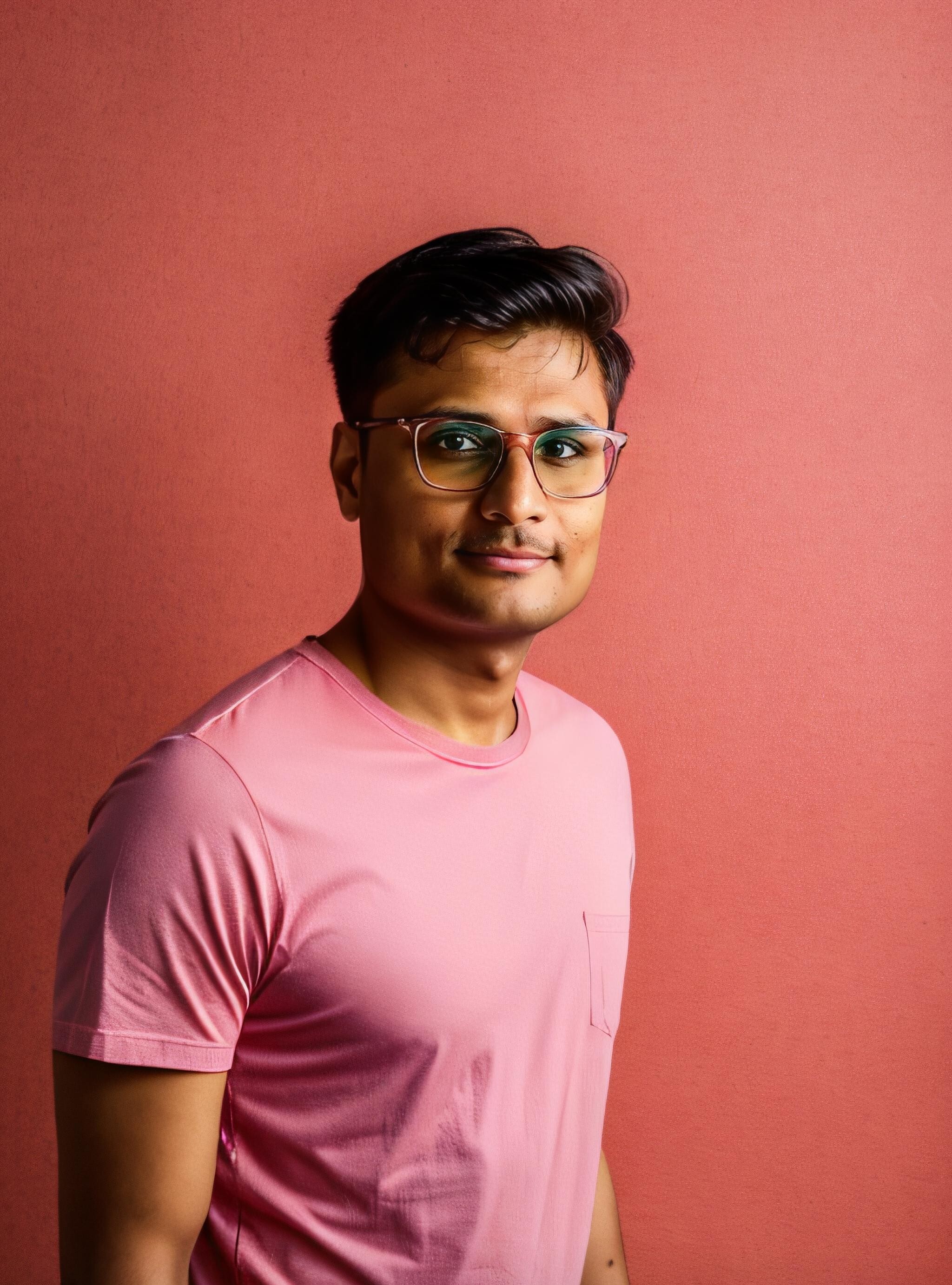