What is Drizzle ORM?
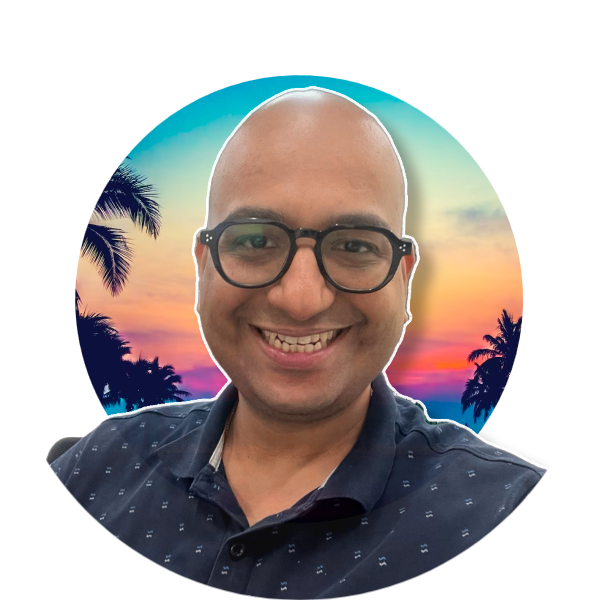
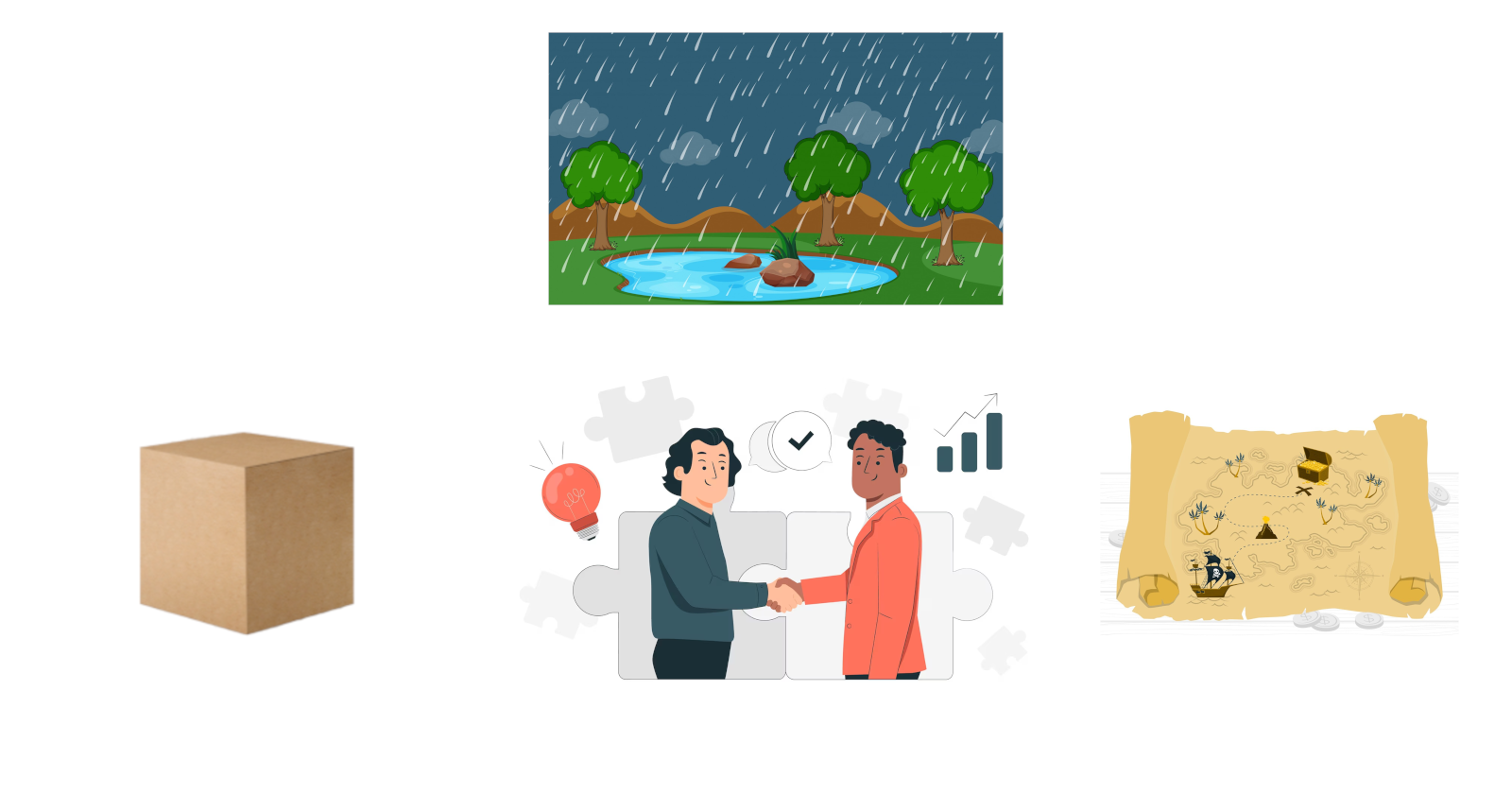
Intro
In the ever-evolving tech landscape, where data management and application development intersect, emerges Drizzle ORM—a modern, lightweight, serverless-ready Object-Relational Mapping (ORM) tool designed for TypeScript applications. At its core, Drizzle simplifies the process of relational data management while maintaining strong adherence to SQL, giving developers the flexibility and power of traditional SQL with the added benefits of modern ORM features.
Drizzle stands out by combining ease of use with high performance, ensuring minimal overhead in both learning and execution. This makes it an attractive option for developers looking to streamline database operations without sacrificing the control and robustness provided by SQL. Whether you're building small applications or scaling up to enterprise-level systems, Drizzle ORM equips you with the tools necessary for efficient data handling, migrations, and schema management.
Simple explanation
Imagine you have a giant toy box (database) where your toys (data) are kept. Drizzle ORM is like your smart helper who knows exactly where each toy should go and how to find them quickly. It makes sure you can play (access and manage your data) very easily without having to remember where everything is placed.
Examples
1. Defining a Table
export const users = pgTable('users', {
id: serial('id').primaryKey(),
name: varchar('name', { length: 256 }),
});
- Create a user table: This example shows how to define a table for users with a unique ID and a name.
2. Fetching Data
await db.select().from(users).where(eq(users.id, 10));
- Retrieve user information: This code fetches details for the user with an ID of 10.
3. Joining Tables
await db.select()
.from(users)
.leftJoin(profiles, eq(profiles.userId, users.id))
.where(eq(users.id, 10));
- Fetch user with profile: Here, data from both the users and profiles tables are combined to provide comprehensive user information.
4. Updating Data
await db.update(users).set({ name: 'New Name' }).where(eq(users.id, 10));
- Update user's name: This modifies the name of the user with the ID of 10 to 'New Name'.
5. Inserting Data
await db.insert(users).values({ name: 'Alice' });
- Add a new user: This code snippet adds a new user named Alice to the users table.
Use Cases
Rapid Development
Quick setup: Get your database and backend running in minutes.
Less boilerplate code: Drizzle reduces the amount of repetitive code you need to write.
Application Scaling
Efficient queries: Drizzle ensures optimal query performance, crucial for high-load applications.
Serverless compatibility: Perfect for applications with variable usage patterns.
Data Integrity
Type safety: Drizzle's TypeScript integration helps prevent common data typing errors.
Automatic migrations: Safeguard and streamline schema evolution.
Microservices Architecture
Decoupled components: Each service can interact with the database independently through Drizzle.
Consistent API: Drizzle provides a consistent querying interface across various microservices.
Educational Projects
Easy learning curve: Straightforward for beginners to pick up and use effectively.
Comprehensive documentation: Well-documented features make learning and implementation smooth.
Conclusion
Drizzle ORM represents a significant leap forward in ORM technology, particularly for TypeScript developers. By reducing complexity and enhancing performance, Drizzle enables developers to focus more on crafting business logic rather than getting bogged down by database schema management. Its simplicity, coupled with powerful features, makes Drizzle a compelling choice for projects of all sizes. As the tech community continues to embrace Drizzle, it is set to redefine norms around data handling in modern web development.
References -
Images Attribution
Image by storyset on Freepik
Image by brgfx on Freepik
Image The box on Freepik
Image by storyset on Freepik
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
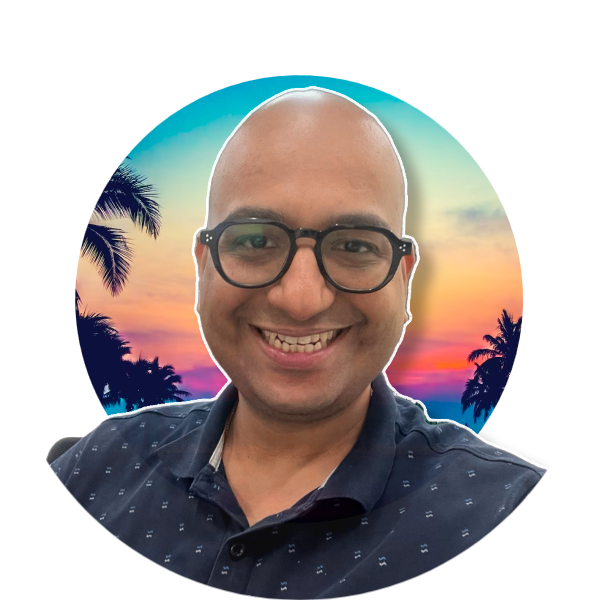
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.