Rotate Image
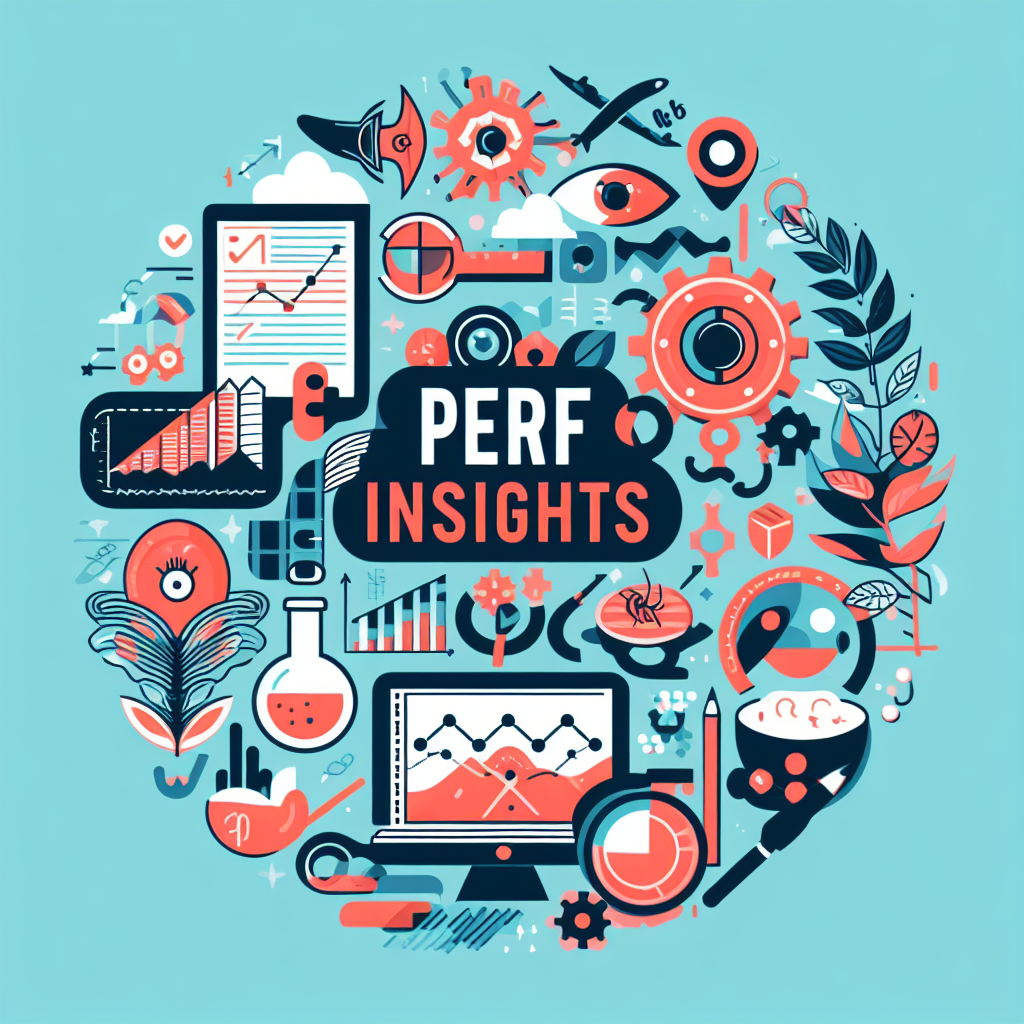
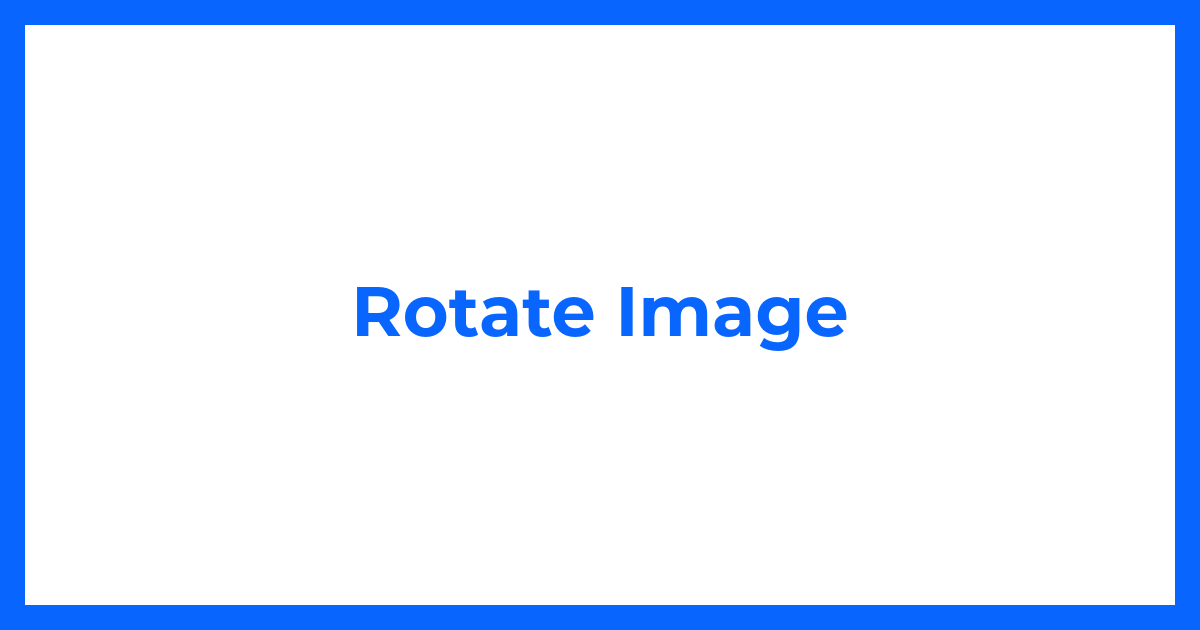
You are given an n x n
2D matrix
representing an image, rotate the image by 90 degrees (clockwise).
You have to rotate the image in-place, which means you have to modify the input 2D matrix directly. DO NOT allocate another 2D matrix and do the rotation.
LeetCode Problem - 48
class Solution {
// Method to rotate the given n x n 2D matrix by 90 degrees clockwise
public void rotate(int[][] matrix) {
int n = matrix.length; // Length of the matrix
// Step 1: Transpose the matrix
// Swap elements at positions (i, j) with (j, i)
for(int i = 0; i < n; i++){
for(int j = i + 1; j < n; j++){
int temp = matrix[i][j]; // Temporarily store the value at matrix[i][j]
matrix[i][j] = matrix[j][i]; // Assign the value at matrix[j][i] to matrix[i][j]
matrix[j][i] = temp; // Assign the temporarily stored value to matrix[j][i]
}
}
// Step 2: Reverse each row
// Swap elements in each row to reverse the row
for(int i = 0; i < n; i++){
for(int j = 0; j < n / 2; j++){
int temp = matrix[i][j]; // Temporarily store the value at matrix[i][j]
matrix[i][j] = matrix[i][n - j - 1]; // Assign the value at matrix[i][n - j - 1] to matrix[i][j]
matrix[i][n - j - 1] = temp; // Assign the temporarily stored value to matrix[i][n - j - 1]
}
}
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
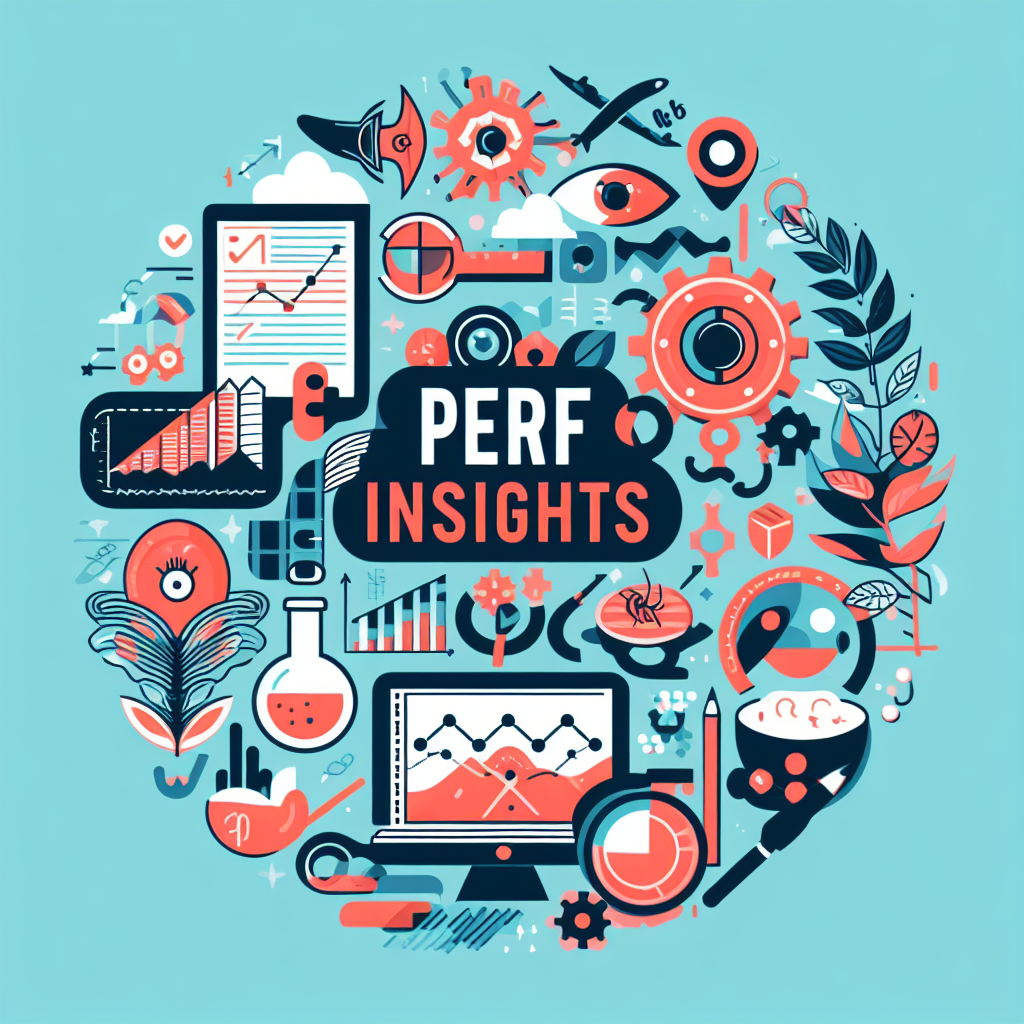
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.