JavaScript Functions Explained: The Essential Guide for Beginners
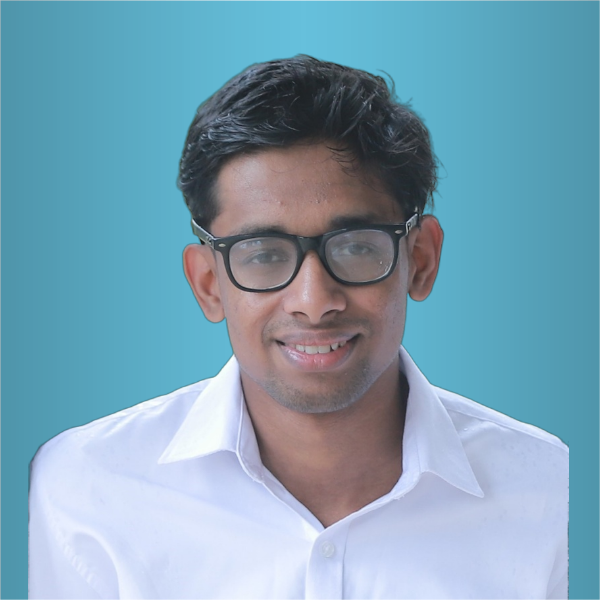
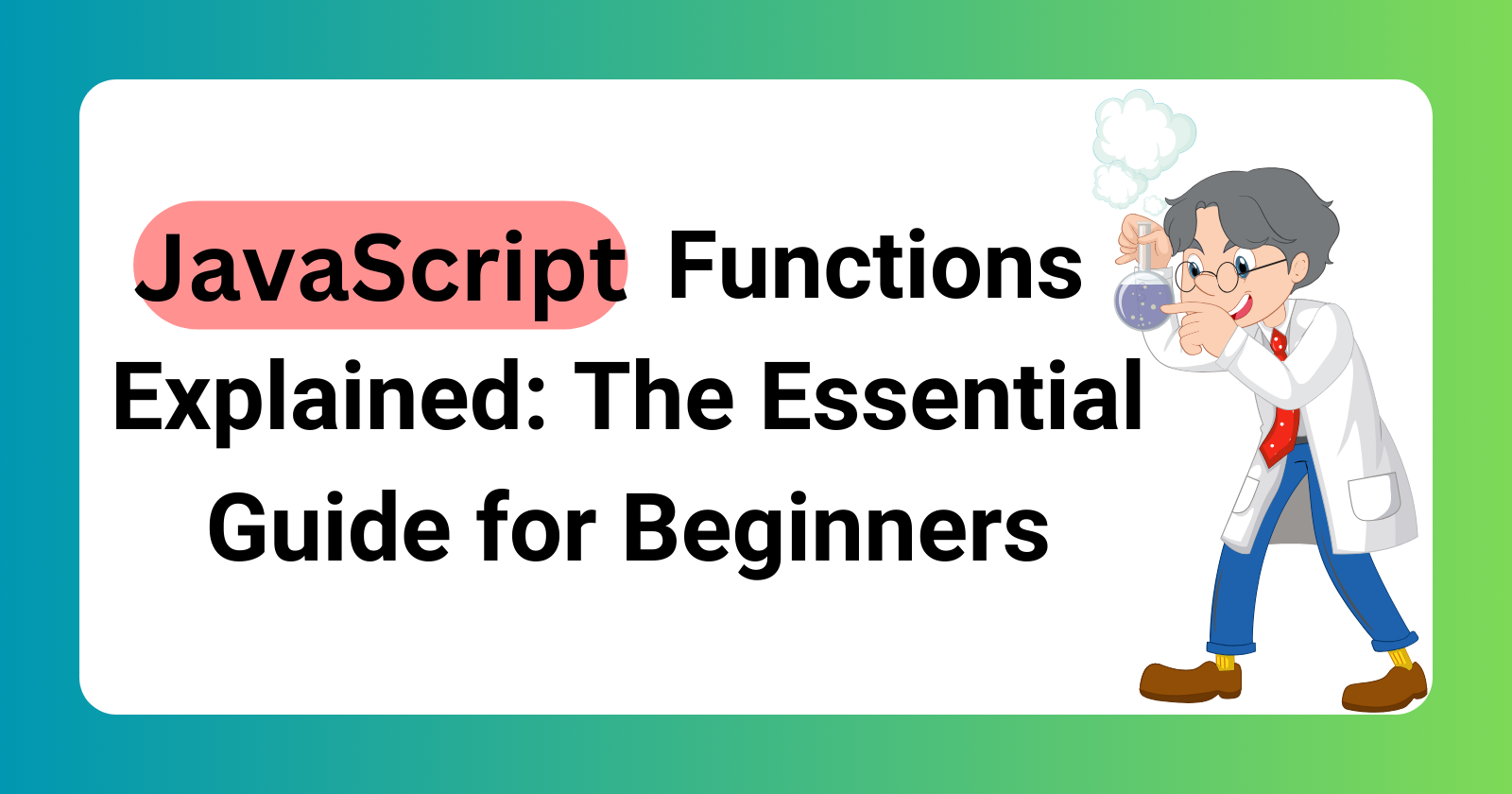
Once upon a time, in a magical land called Codeville, there were these little helpers called Functions. Now, imagine Functions as little wizards who can do special tasks for us.
Let's meet our first Function, named MagicMaker. MagicMaker's job is to make things disappear. So, whenever you say, "Hey MagicMaker, make this vanish !" MagicMaker waves its wand, and poof! It's gone!
Then there's another Function named SpellCaster. SpellCaster is really good at making things change colors. When you say, "Hey SpellCaster, turn this red! " it works its magic and, ta-da! It's red !
Now, here's the coolest part. You can tell these little wizards to remember certain spells. For example, MagicMaker can remember how to make an apple vanish, and SpellCaster can remember how to turn a flower blue.
So, when you ask MagicMaker to make the apple vanish, it already knows the spell and makes it disappear without you having to tell it how. And SpellCaster, when asked to turn a flower blue, knows just what to do because it remembered the spell.
That's just like Functions in JavaScript ! You can create them to do special tasks, and you can tell them to remember how to do those tasks. So, whenever you need that task done again, you can just call the Function, and it knows exactly what to do!
And just like our little wizards, Functions in JavaScript can make your code more magical and fun to work with !
Creating a Function โ๏ธ
To create a function, you use the function
keyword followed by a name, parentheses, and curly braces to define the code block.
Curly braces {}
are used to define the beginning and end of the function's code block. Variables declared inside a block (enclosed by curly braces) are scoped to that block. This means that variables declared within a function's curly braces are local to that function and cannot be accessed from outside the function. This helps prevent unintended side effects and variable name conflicts.
When defining a function, parentheses are used to enclose the parameters that the function accepts. Parameters are placeholders for values that will be passed to the function when it is called. Don't be afraid. We will discuss this in the blog post in the future. ๐คซ
function greet() {
console.log("Hello there !");
}
In this example "greet" is name of the function.
Calling a Function
Creating a function alone isn't enough; you must call or invoke the function to execute its code. This is done by writing the function's name followed by parentheses.
greet();
This will log Hello there ! to the console.
Parameters & Arguments
Functions can accept inputs called parameters, which act as variables within the function. You define parameters within the parentheses when declaring the function.
function milkPrice(bottles) {
var cost = bottles*100;
console.log("Milk Price is " + cost);
}
An argument is the actual value that is passed to the function when it is called. It's the data that gets assigned to the corresponding parameter inside the function. Arguments are passed inside the parentheses when you call the function.
When calling the milkPrice
function, you pass a value for bottles
, like so:
milkPrice(4);
This will log Milk price is 400 to the console.
Return Statement
Functions can produce outputs using the return
statement, allowing them to return a value to the code that called them.
function add(a, b) {
return a + b;
}
We can store the return
value value of the function in a variable. For example, when calling the above function, consider the passing of two values 3 and 5 as arguments. Then 3+5=8 is return from the function. The return value is stored in a variable called result
.
var result = add(3, 5);
Function Expressions
You can also define functions using function expressions, where you assign a function to a variable.
var multiply = function(a, b) {
return a * b;
};
var product = multiply(4, 6); // product will be 24
Here you will see that the function has no name. Therefore, functions expressions are sometimes referred to as anonymous functions. [We will discuss anonymous functions later] But function expression can be created even with a name.
var multiply = function procuct(a, b) {
return a * b;
};
var product = multiply(4, 6); // product will be 24
Even though the function expression has a name (product
), it is assigned to a variable (multiply
) and can be called using that variable. The name product
is only accessible inside the function itself and is useful for recursion or debugging purposes.
Arrow Functions ๐น
Arrow functions, introduced in ECMAScript 6 (ES6), provide a more concise syntax for writing functions in JavaScript compared to traditional function expressions. They offer a shorthand notation for writing function expressions, making code more readable and often more elegant.
Traditional method
function add(a, b) { return a + b; } console.log("Result is " + add(3, 5));//This will log "Result is 8"
Function Expression
const add = function(a, b){ return a + b; } console.log("Result is " + add(2, 4));//This will log "Result is 6"
Arrow Functions
const add = (a, b) => { return a + b; } console.log("Result is " + add(1, 3));//This will log "Result is 4"
If the function body is a single statement, you can omit the curly braces
{}
and thereturn
keyword, and the expression will be implicitly returned.const add = (a, b) => a + b; console.log("Result is " + add(0, 2));//This will log "Result is 2"
If the function has only one parameter, you can further simplify the function declaration as follows.
const power = x => x*x; console.log(x + " to the power " + x " is " + power(2));//This will log "2 to the power 2 is 4"
Anonymous Functions ๐ค
Anonymous functions, also known as function expressions, are functions that are defined without a name. Instead of being declared with the function
keyword followed by an identifier (the function name), anonymous functions are created inline, typically as part of a larger expression or assignment.
var add = function(x, y) {
return x + y;
};
In this example, the add
variable is assigned the value of an anonymous function that takes two parameters x
and y
and returns their sum.
To define an anonymous function as an arrow function, you simply omit the function name and use the arrow function syntax (parameters) => { /* code */ }
.
// Arrow function as an anonymous function
var add = (x, y) => {
return x + y;
};
// Example usage
var result = add(5, 3);
console.log(result); // Output: 8
Before we learn about purposes of anonymous functions, let's learn about Callback functions and setTimeout function.
Callback Functions ๐
Function Sequence
JavaScript functions are executed in the sequence they are called. Not in the sequence they are defined.
function function1() {
console.log("Hello");
}
function function2() {
console.log("Goodbye");
}
function function3() {
console.log("Aayubowan");
}
function1();
function2();
Here first logs "Hello" and then logs "Goodbye" .
function function1() {
console.log("Hello");
}
function function2() {
console.log("Goodbye");
}
function function3() {
console.log("Aayubowan");
}
function2();
function1();
But here first logs "Goodbye" and then logs "Hello" .
We can also call for another function to work in a particular function.
function function1() {
console.log("India");
}
function function2() {
console.log("Sri Lanka");
function1();
}
function function3() {
console.log("USA");
}
function2();
The code logs "Sri Lanka" first, then "India". This is because function2
calls function1
after logging "Sri Lanka". So, when function1
is called, it logs "India" to the console.
Callback Functions
Callback functions are functions that are passed as arguments to other functions and are invoked (called or executed) by those functions at a later time, typically in response to some event or condition.
Suppose we want to call function3
after executing function1
. You can do this as follows:
function function1(callback) {
console.log("India");
callback();
}
function function2() {
console.log("Sri Lanka");
}
function function3() {
console.log("USA");
}
function1(function3);
The code logs "India" first, then "USA". This is because function1
calls the callback function (function3
) after logging "India", and when function3
is called, it logs "USA". (callback
is the parameter name of function1
. We can use any name for this)
Callback functions can even pass arguments.
function function1(callback) {
console.log("India");
const randNum = Math.random();
callback(randNum);
}
function function2() {
console.log("Sri Lanka");
}
function function3(ran) {
console.log("USA");
console.log(ran);
}
function1(function3);
The code logs "India" first, then "USA" followed by the value of the randomly generated number. This is because function1
calls the callback function (function3
) after logging "India", and when function3
is called, it logs "USA" and the value of the random number passed as an argument.
setTimeout Function โฐ
setTimeout()
is a function used to execute a specified function or piece of code after a specified delay in milliseconds.
setTimeout(function, milliseconds);
The function
parameter specifies the function to be executed. The milliseconds
parameter specifies the number of milliseconds after which the function should be executed.
For example, if you want to display a message after 2 seconds (=2000 mili seconds), you would use setTimeout()
like this:
setTimeout(function() {
console.log("Hello, world!");
}, 2000);
Purposes of Anonymous Functions
Callback Functions
They are often used as callback functions, which are functions passed as arguments to other functions to be executed later, typically in response to an event or asynchronous operation.
// Example of using an anonymous function as a callback setTimeout(function() { console.log("This message will be logged after 1 second"); }, 1000);
Immediately Invoked Function Expressions (IIFE)
Anonymous functions can be invoked immediately after they are defined, creating a private scope for encapsulating variables and avoiding global namespace pollution.
// IIFE using an anonymous function (function() { var x = 10; console.log("Inside IIFE: " + x); // Outputs: "Inside IIFE: 10" })();
Higher-Order Functions
They can be passed as arguments to higher-order functions, which are functions that accept other functions as parameters or return functions as results.
// Higher-order function that takes an anonymous function as a parameter function processNumber(num, callback) { return callback(num); } // Using processNumber with an anonymous function var result = processNumber(5, function(num) { return num * 2; }); console.log(result); // Outputs: 10
The provided code defines a higher-order function,
processNumber
, which takes a number and a callback function as arguments. InsideprocessNumber
, the callback function is called with the given number. An anonymous function that doubles its input is then passed toprocessNumber
along with the number 5. This anonymous function receives 5, multiplies it by 2, and returns 10, which is then returned byprocessNumber
. Finally, the result (10) is printed to the console. This demonstrates how a higher-order function can use a callback to process data flexibly.
This beginner friendly article introduces JavaScript functions through a amazing story about magical wizards named MagicMaker and SpellCaster. It covers the basics of creating, calling, and using functions, including parameters, arguments, and return statements. The article also delves into function expressions, arrow functions, anonymous functions, callback functions, and the setTimeout function, providing practical examples step by step and explanations to make your code more efficient and fun.
Attribution of Images: Designed by Freepik
Subscribe to my newsletter
Read articles from Pasindu Basnayake directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
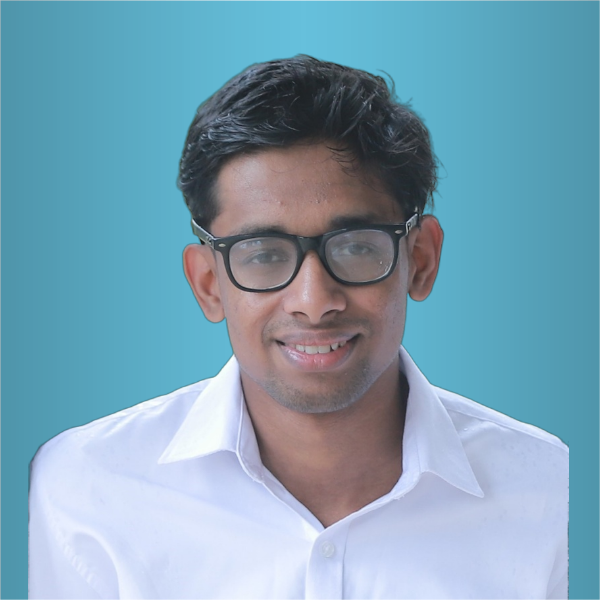
Pasindu Basnayake
Pasindu Basnayake
Hi, I am Pasindu Basnayake from Sri Lanka, a very small beautiful island. Through my articles, I share the experiences of my coding journey and explanations of various concepts with you. I am learning coding by myself. I know this is a difficult but beautiful journey. ๐ข My aim is to help someone like me who is learning coding by himself. If you can learn even a small thing through my articles, that is my happiness. ๐๐ฑ