Passing variables from one Batch to another
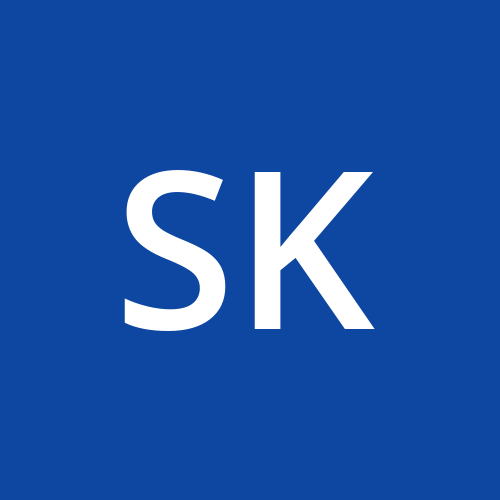
Problem Statement - Let's discuss a scenario where we want to query all the Account records from one Batch and then for further processing or comparisions we wanted to have a copy of the same in another Batch.
Scenario - We have created two Batch classes namely
AccountBatch - Queries all Account records and passes the list onto the Contact Batch(Batch 2).
ContactBatch - Queries all Contact and display's the Account's queries in Account Batch (Batch 1).
Code Solution - In order to pass the List from one Batch Job to another we will use Database.Stateful and it will accumulate all the accounts queried in the scope in a public List variable.
Then we will iterate over the scope in execute method and add each account to the public list varible.
Now comes the most important point which is to pass this variable to another batch and we will take the help of a parameterized constructor.
See the below Classes.
Class Name - AccountBatch
public class AccountBatch implements Database.Batchable<sObject>,Database.Stateful{
public List<Account> accList = new List<Account>();
Integer accSize = 0;
public Database.QueryLocator start(Database.BatchableContext bc)
{
String query = 'Select Id,Name,Active__c from Account';
return Database.getQueryLocator(query);
}
public void execute(Database.BatchableContext bc , List<Account> scope)
{
for(Account acc : scope)
{
accList.add(acc);
accSize++;
}
}
public void finish(Database.BatchableContext bc)
{
System.debug('Acc List in Account Batch '+accList);
System.debug('Acc List size in Account Batch'+accSize);
//now let's execute the second batch class and pass the above public accList as a parameterized constructor
Database.executeBatch(new ContactBatch(accList),200);
}
}
Class Name - Contact Batch
public class ContactBatch implements Database.Batchable<sObject>{
public List<Account> accListNew = new List<Account>();
//declaring a constructor of the Batch class which will initialize the above accListNew variable with the contents of accList from AccountBatch
public ContactBatch(List<Account> accList)
{
accListNew = accList;
}
public Database.QueryLocator start(Database.BatchableContext bc)
{
String query = 'Select id,Name from Contact';
return Database.getQueryLocator(query);
}
public void execute(Database.BatchableContext bc , List<Contact> scope)
{
//Your Logic
}
public void finish(Database.BatchableContext bc)
{
System.debug('Debug from finish of ContactBatch');
System.debug('Account List coming from Account Batch to Contact Batch'+accListNew);
}
}
Debug logs screenshots :
Subscribe to my newsletter
Read articles from SHARIQUE KALAM directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
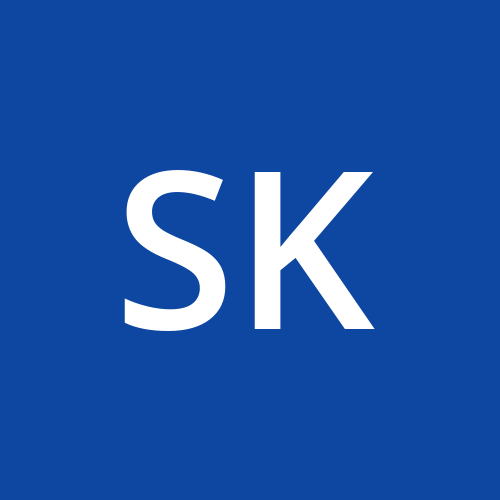
SHARIQUE KALAM
SHARIQUE KALAM
I am a Salesforce developer with strong intention to learn full stack Salesforce Development and I am here to share my learnings and also to learn.