STACKS in C++
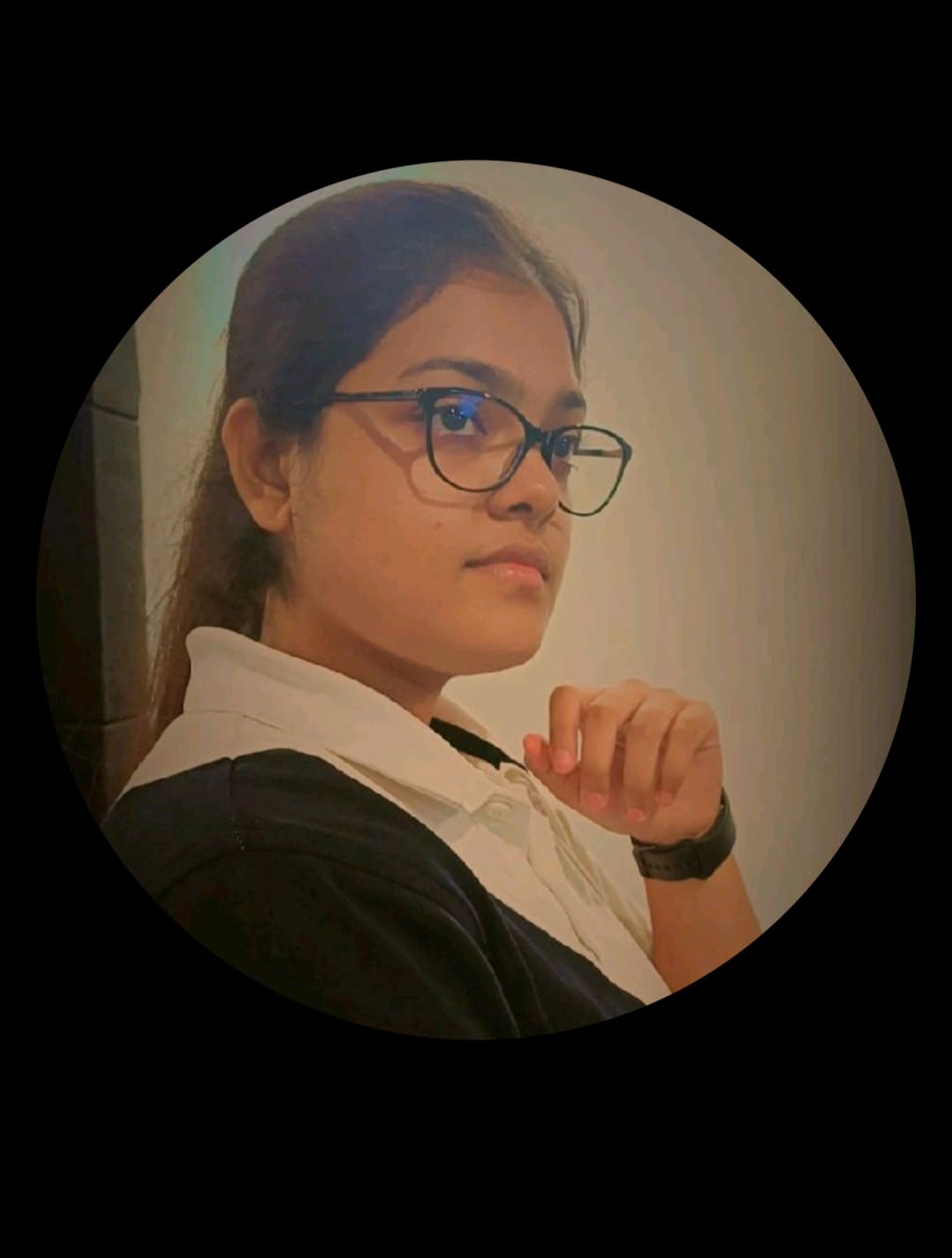
Table of contents
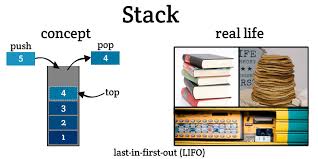
WHAT IS STACK?
It is a linear data structure and also an abstract data type ,where we can do only operation in a specific manner. It works on the LIFO (last in first out) or FILO (first in last out) system.
LIFO means the elements which is inserted last, comes out first.
FILO means the elements which is inserted first, comes out last.
STACK REPRESENTATION THROUGH DIAGRAM
SOME BASIC OPERATIONS IN STACK
Push : Adding elements
Pop : Removing elements
Peek : Returning of topmost element
IsFull : If stack is full
IsEmpty : Check if stack is empty
SOME TERMS NEED TO BE REMEMBER
If stack is full then (top==capacity-1) means stack overflow means no element is inserted. Otherwise we increment the value of top by 1 (top=top+1).
If stack is empty then (top==-1) means stack underflow means we cannot the remove the element from stack because there is no element. Otherwise we decrement the value of top by 1(top=top-1).
STACK IMPLEMENTATION USING ARRAY
#include<iostream>
#include<climits>
using namespace std;
class stackusingarray{
int *data;
int nextIndex;
int capacity;
public:
stackusingarray(int totalSize){
data=new int(totalSize);
nextIndex=0;
capacity=totalSize;
}
int size(){
return nextIndex;
}
bool isEmpty(){
return nextIndex==0;
}
void push(int element){
if(nextIndex==capacity){
cout<<"stack if full."<<endl;
return;
}
data[nextIndex]=element;
nextIndex++;
}
int pop(){
if(isEmpty()){
cout<<"stack is empty"<<endl;
return INT_MIN;
}
nextIndex--;
return data[nextIndex];
}
int top(){
return data[nextIndex-1];
}
};
int main(){
stackusingarray s(4);
s.push(10);
s.push(20);
s.push(30);
s.push(40);
s.push(50);
cout<<s.top()<<endl;
cout<<s.pop()<<endl;
cout<<s.pop()<<endl;
cout<<s.size()<<endl;
cout<<s.isEmpty()<<endl;
return 0;
}
STACK IMPLEMENTATION USING LINKED LIST
#include<iostream>
using namespace std;
class node {
public:
int data;
node* next;
node(int data) {
this->data = data;
next = nullptr;
}
};
class stackusinglinkedlist {
node* head;
int size;
public:
stackusinglinkedlist() {
head = nullptr;
size = 0;
}
int getsize() {
return size;
}
bool isEmpty() {
return size == 0;
}
void push(int element) {
node* newnode = new node(element);
newnode->next = head;
head = newnode;
size++;
}
int pop() {
if (isEmpty()) {
return 0;
}
int ans = head->data;
node* temp = head;
head = head->next;
delete temp;
size--;
return ans;
}
int top() {
if (isEmpty()) {
return 0;
}
return head->data;
}
};
int main() {
stackusinglinkedlist s;
s.push(10);
s.push(20);
s.push(30);
s.push(40);
s.push(50);
cout << s.top() << endl;
cout << s.pop() << endl;
cout << s.pop() << endl;
cout << s.pop() << endl;
s.push(60);
cout << s.getsize() << endl;
cout << s.isEmpty() << endl;
return 0;
}
Subscribe to my newsletter
Read articles from Kali Baranwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
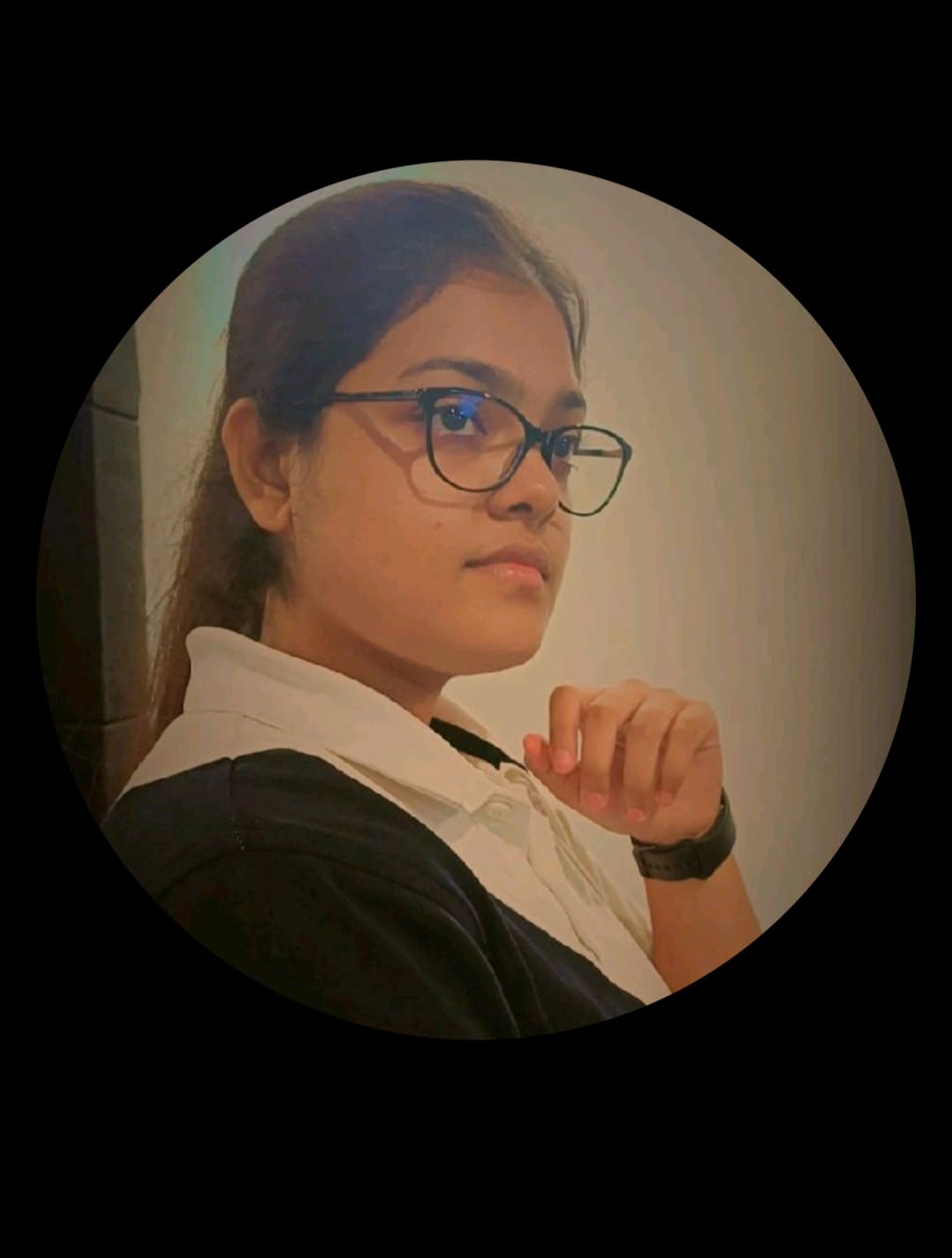
Kali Baranwal
Kali Baranwal
"๐ Hello, fellow developers! I'm Kali Baranwal , a 2nd year student . I've , exploring in another blog post also. Skilled in frontend developer, UI/UX design , C++ Join me as I share insights, of notes here on Hashnode .๐ Beyond technical skills, I'm also passionate about continuous learning and personal development. Let's connect, learn, and grow together!"