How To Use MySQL With MERN?

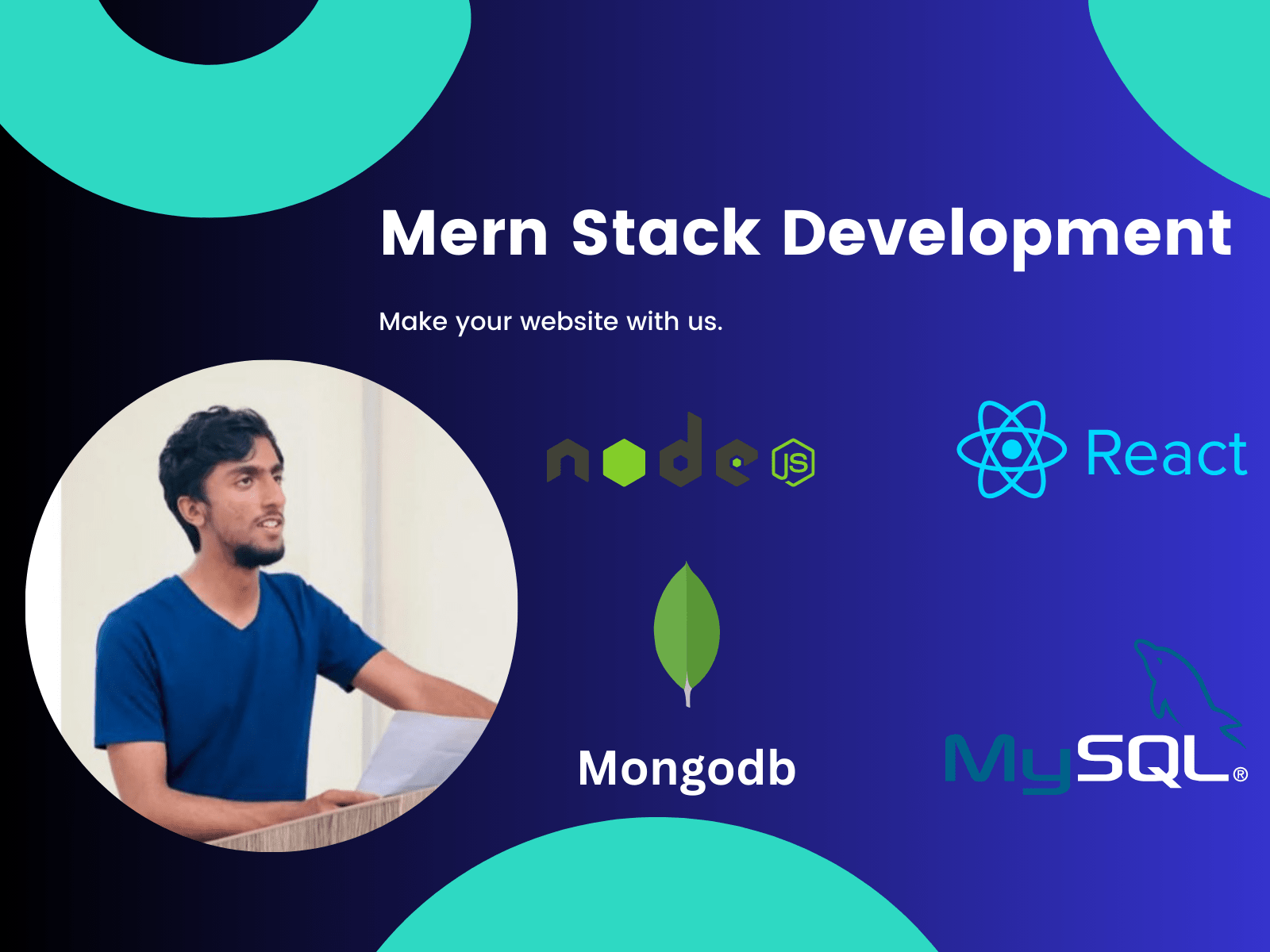
Introduction
The MERN stack, comprising MongoDB, Express.js, React, and Node.js, is a popular choice for building dynamic web applications. However, you may prefer using a relational database like MySQL instead of MongoDB for its robust querying capabilities and structured data management. Integrating MySQL with the MERN stack involves configuring MySQL, connecting it to the Node.js server via Express, and ensuring seamless data communication with the React frontend. This approach retains the flexibility and power of the MERN stack while leveraging MySQL's advantages, providing a versatile solution for developers seeking efficient and scalable application development.
Easy Steps To Use MySQL With MERN
Using MySQL with the MERN stack (MongoDB, Express.js, React, Node.js) involves replacing MongoDB with MySQL as the database layer. One can join the MERN Stack Online Course to learn how to integrate MERN with MySQL.
Here is a comprehensive guide on how to integrate MySQL into a MERN application:
1. Setting Up MySQL
Install MySQL:
· Download and install MySQL from the official website.
· Set up your MySQL server and create a database for your application.
Create a Database and User:
· Open the MySQL shell and log in as root:
“mysql -u root –p”
· Create a new database:
“CREATE DATABASE myapp;”
· Create a new user and grant all privileges on the new database:
“CREATE USER 'myappuser'@'localhost' IDENTIFIED BY 'password';
GRANT ALL PRIVILEGES ON myapp.\ TO 'myappuser'@'localhost';*
FLUSH PRIVILEGES;”
2. Setting Up Node.js and Express
Initialize a Node.js Project:
· Create a new directory for your project and initialize it:
“mkdir myapp
cd myapp
npm init –y”
Install Required Packages:
· Install Express and MySQL packages:
“npm install express mysql”
3. Connecting to MySQL in Node.js
Create a Database Connection:
· Create a file db.js to manage the MySQL connection:
“const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'myappuser',
password: 'password',
database: 'myapp'
});
connection.connect((err) => {
if (err) throw err;
console.log('Connected to MySQL!');
});
module.exports = connection;”
4. Creating Express Routes
Set Up Express Server:
· Create server.js to configure your Express server:
“const express = require('express');
const app = express();
const db = require('./db');
app.use(express.json());
app.get('/', (req, res) => {
res.send('Hello World!');
});
// Example route to get data from MySQL
app.get('/users', (req, res) => {
db.query('SELECT \ FROM users', (err, results) => {*
if (err) throw err;
res.json(results);
});
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});”
5. Integrating React
Create React App:
· Set up a React application using Create React App in a separate directory:
“npx create-react-app client
cd client”
Proxy Requests to the Node.js Server:
· Add a proxy to the package.json of your React app:
“"proxy": "http://localhost:3000""
Fetch Data from Express API:
· In your React component (e.g., App.js), fetch data from the Express server:
“import React, { useState, useEffect } from 'react';
function App() {
const [users, setUsers] = useState([]);
useEffect(() => {
fetch('/users')
.then(response => response.json())
.then(data => setUsers(data))
.catch(error => console.error('Error fetching data:', error));
}, []);
return (
<div>
<h1>Users</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
export default App;”
6. Running the Application
· Start the Node.js server:
“node server.js”
· Start the React development server:
“cd client
npm start”
As a Mern Stack Developer, you can follow these steps to replace MongoDB with MySQL in a typical MERN stack setup. The key changes include setting up MySQL, connecting it to your Express server, and ensuring your React frontend communicates correctly with the backend. This setup allows you to leverage the strengths of relational databases while maintaining the flexibility and structure of the MERN stack.
Conclusion
By integrating MySQL into the MERN stack, you leverage the relational database's strengths while retaining the stack's flexibility. This involves setting up MySQL, configuring the Node.js backend to connect to MySQL, and ensuring smooth data flow between the backend and React frontend. This setup enhances your application's data management capabilities.
Subscribe to my newsletter
Read articles from Pankaj Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pankaj Sharma
Pankaj Sharma
Hi, I’m Pankaj Sharma from Noida and working as an educational blogger.