Understanding Git and Version Control Systems (VCS)
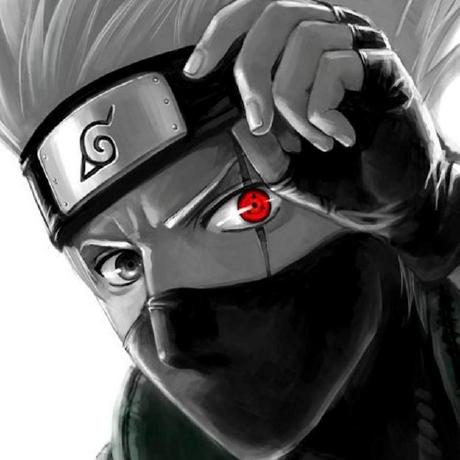
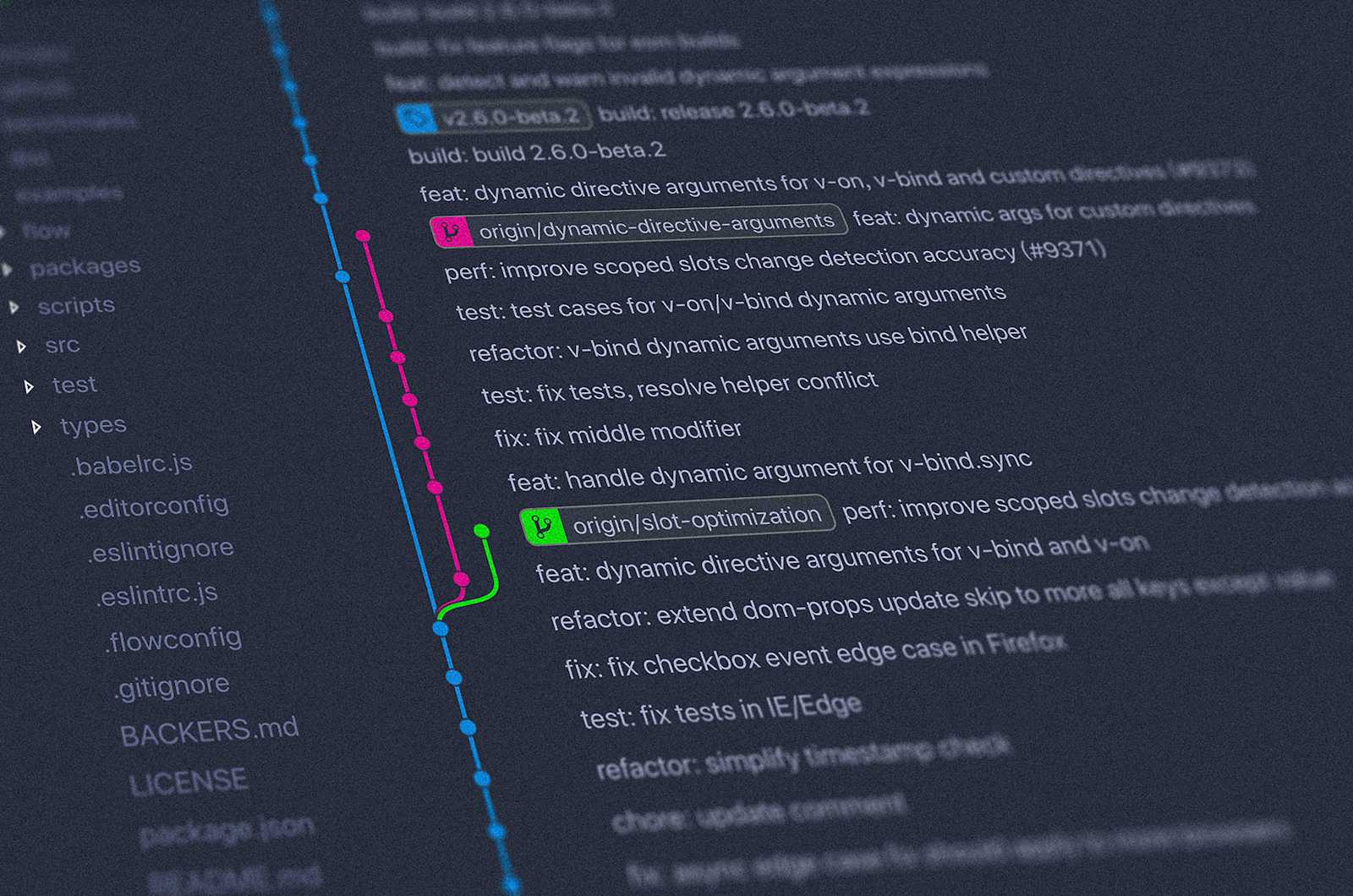
In the world of software development, managing changes efficiently is crucial. This is where Version Control Systems (VCS) come into play. They help track changes, coordinate work among multiple developers, and maintain project history. Git, a distributed version control system, has become the most widely used tool for these purposes. Let's explore what VCS and Git are, their importance, practical Git commands, and effective Git branching strategies.
What is a Version Control System (VCS)?
A Version Control System (VCS) is a tool that helps track changes to files and coordinates work among multiple people. It allows you to:
Revert files back to a previous state.
Revert the entire project back to a previous state.
Compare changes over time.
See who last modified something that might be causing a problem.
Identify who introduced an issue and when.
Popular VCS tools include Git, Mercurial, and Subversion.
Types of VCS
Centralized Version Control Systems (CVCS):
Central Repository: Stores all versioned files on a single server.
Examples: CVS, Subversion (SVN).
Pros: Simple to understand, centralized repository, file locking.
Cons: Single point of failure, network dependency, slower operations, limited offline access.
Distributed Version Control Systems (DVCS):
Distributed Repository: Each user has a complete copy of the repository.
Examples: Git, Mercurial, Bazaar.
Pros: Offline access, decentralized workflow, redundancy, better branching and merging, speed, flexibility.
Cons: Complexity, disk space usage, steeper learning curve, merge conflicts, security concerns.
Why Do We Need VCS?
Version Control Systems are essential for collaborative software development. They provide:
Collaboration: Multiple developers can work on the same project simultaneously and merge their changes seamlessly.
History Tracking: Keeps a complete history of changes, invaluable for debugging and auditing.
Reverting Changes: Allows reverting files or the entire project to a previous state if something goes wrong.
Branching and Merging: Enables developers to work on new features or bug fixes in isolation and merge changes into the main codebase when ready.
Conflict Resolution: Helps identify and resolve conflicts when multiple developers make changes to the same file.
Backup: Acts as a backup mechanism, ensuring the project's entire history is stored safely.
What is Git?
Git is a Distributed Version Control System (DVCS) created by Linus Torvalds in 2005 for the development of the Linux kernel. It has since become one of the most widely used version control systems due to its flexibility, speed, and robustness.
Key Features of Git:
Distributed Development: Each developer has a copy of the entire repository, including its full history. This allows for offline work and local operations.
Branching and Merging: Powerful branching and merging capabilities make it easy to work on multiple features or bug fixes simultaneously.
Speed and Performance: Designed to be fast and efficient, even with large projects and repositories.
Data Integrity: Uses a cryptographic hash function (SHA-1) to ensure the integrity of the repository and detect data corruption.
Staging Area: A staging area allows you to review and prepare your changes before committing them.
Open Source: Git is open source and supported by a large community of developers and contributors.
Non-linear Development: Allows for flexible branching and merging, enabling non-linear development workflows.
Git is used by millions of developers and organizations worldwide for version control and collaboration on software projects.
Practical Git Commands
Here's a collection of practical Git commands to help you get started and manage your repositories effectively. These commands are presented as Bash scripts for convenience.
Configuration Commands
#!/bin/bash
# Configure Git username
git config --global user.name "Your Name"
# Configure Git email
git config --global user.email your.email@example.com
# Set default editor for Git
git config --global core.editor "code --wait"
# Edit the global configuration file
git config --global -e
Repository Initialization
#!/bin/bash
# Initialize a new Git repository
git init
Staging and Committing Changes
#!/bin/bash
# List files in the repository
git ls-files
# Add a single file to the staging area
git add file_name
# Add multiple files to the staging area
git add file1 file2
# Commit staged changes with a message
git commit -m "Commit Message"
Removing and Renaming Files
#!/bin/bash
# Remove a file from the repository
git rm file_name
# Rename a file in the repository
git mv original_file_name new_file_name
Viewing Changes and History
#!/bin/bash
# Check the status of the repository
git status
# Show differences between the working directory and the staging area
git diff --staged
# View the commit history
git log
Branching and Merging
#!/bin/bash
# Create a new branch
git branch branch_name
# Switch to the new branch
git checkout branch_name
# Merge another branch into the current branch
git merge branch_name
Working with Remote Repositories
#!/bin/bash
# Add a new remote repository
git remote add origin https://github.com/user/repo.git
# Push changes to the remote repository
git push origin branch_name
# Pull changes from the remote repository
git pull origin branch_name
Full Script Example
Here's a complete script that incorporates several of the above commands for initializing a repository, configuring user details, creating a branch, making changes, and pushing to a remote repository:
#!/bin/bash
# Configure Git user details
git config --global user.name "Your Name"
git config --global user.email your.email@example.com
# Initialize a new Git repository
git init
# Create a README file
echo "# My Project" > README.md
# Add README file to the staging area
git add README.md
# Commit the README file
git commit -m "Initial commit with README"
# Create a new branch
git branch develop
# Switch to the new branch
git checkout develop
# Create a sample file
echo "print('Hello, World!')" > hello.py
# Add the sample file to the staging area
git add hello.py
# Commit the sample file
git commit -m "Add hello.py script"
# Add a remote repository
git remote add origin https://github.com/user/repo.git
# Push changes to the remote repository
git push -u origin develop
echo "Repository setup and initial push completed."
To execute the script, save it as setup_
repo.sh
, give it execute permissions, and run it:
chmod +x setup_repo.sh
./setup_repo.sh
This script will guide you through setting up a repository, configuring user details, creating a branch, and pushing to a remote repository, all in one go.
Git Branching Strategies
Effective branching strategies are essential for managing a clean and efficient Git workflow. Here are some popular Git branching strategies:
1. Git Flow
Git Flow is a robust branching model introduced by Vincent Driessen. It uses two main branches:
master
: The production-ready state.develop
: The main branch for ongoing development.
Other supporting branches include:
Feature Branches: For developing new features. Created from
develop
and merged back intodevelop
.Release Branches: For preparing releases. Created from
develop
and merged into bothdevelop
andmaster
.Hotfix Branches: For critical bug fixes in production. Created from
master
and merged back into bothmaster
anddevelop
.
Example Git Flow Commands
# Create and switch to a new feature branch
git checkout -b feature/awesome-feature
# Merge the feature branch back into develop
git checkout develop
git merge feature/awesome-feature
git branch -d feature/awesome-feature
# Create and switch to a new release branch
git checkout -b release/1.0.0
# Merge the release branch into both develop and master
git checkout master
git merge release/1.0.0
git checkout develop
git merge release/1.0.0
git branch -d release/1.0.0
# Create and switch to a hotfix branch
git checkout -b hotfix/critical-bug
# Merge the hotfix branch into both master and develop
git checkout master
git merge hotfix/critical-bug
git checkout develop
git merge hotfix/critical-bug
git branch -d hotfix/critical-bug
2. GitHub Flow
GitHub Flow is a simpler branching strategy, ideal for continuous delivery. It involves:
main
: The production-ready state.Feature Branches: For developing new features. Created from
main
and merged back intomain
via pull requests.
Example GitHub Flow Commands
# Create and switch to a new feature branch
git checkout -b feature/awesome-feature
# Push the feature branch to the remote repository
git push -u origin feature/awesome-feature
# Create a pull request on GitHub and merge it into main
# After merging, delete the feature branch locally and remotely
git checkout main
git pull origin main
git branch -d feature/awesome-feature
git push origin
Conclusion: Embracing Git for Efficient Version Control
In the realm of software development, mastering version control is akin to wielding a powerful tool that ensures collaboration, traceability, and reliability throughout the development lifecycle. Through this exploration of Version Control Systems (VCS) and Git, we've journeyed from understanding the fundamentals to practical commands and effective branching strategies.
Version Control Systems, whether centralized or distributed, serve as the backbone of collaborative coding efforts. They offer a plethora of benefits, including the ability to revert changes, track project history, resolve conflicts, and facilitate seamless collaboration among developers.
At the heart of modern version control lies Git, a distributed system renowned for its speed, flexibility, and robustness. With features like distributed development, branching, and merging, Git empowers developers to work efficiently, whether individually or as part of a team.
Our journey delved into practical Git commands, from initialization to configuration, committing changes, and interacting with remote repositories. We've also explored two prominent branching strategies: Git Flow and GitHub Flow, each offering a distinct approach to managing development workflows.
As we conclude, it's evident that embracing Git and VCS is not merely a choice but a necessity for modern software development teams. By adopting best practices, leveraging powerful tools, and embracing collaboration, teams can navigate the complexities of version control with confidence, ensuring the delivery of high-quality software products, one commit at a time.
Subscribe to my newsletter
Read articles from Prajwal Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
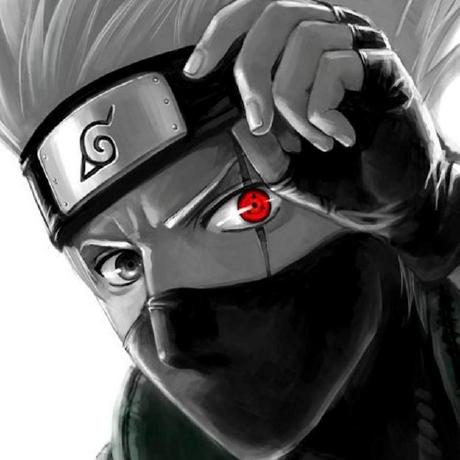