How to Send Email Notifications and OTPs Using Spring Boot
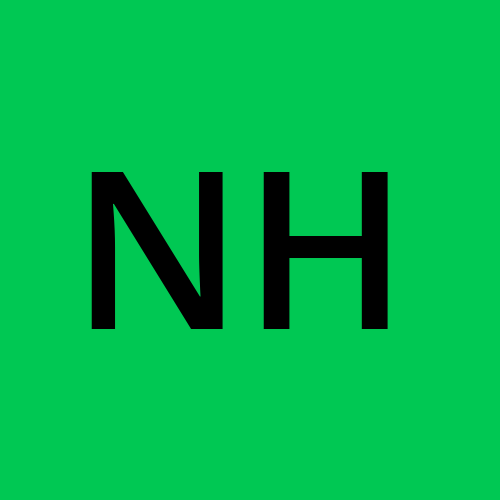
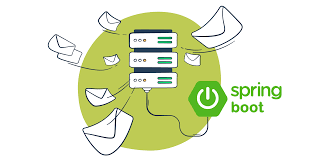
In today's digital age, email notifications and One-Time Passwords (OTPs) have become essential for enhancing user engagement and security. Spring Boot, with its robust and easy-to-use framework, makes implementing these features straightforward. In this blog, I'll guide you through the process of setting up email notifications and sending OTPs using Spring Boot.
Table of Contents
Introduction
Setting Up the Spring Boot Project
Configuring Email Properties
Creating the Email Service
Sending Email Notifications
Generating and Sending OTPs
Conclusion
1. Introduction
Email notifications keep users informed about important updates, while OTPs add an extra layer of security to your application. In this tutorial, we will use Spring Boot to send both email notifications and OTPs. Let's get started!
2. Setting Up the Spring Boot Project
First, create a new Spring Boot project using Spring Initializr or your preferred method. Include the following dependencies:
Spring Web
Spring Boot Starter Mail
Spring Security (optional, for generating secure OTPs)
Your pom.xml
should look something like this:
xmlCopy code<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-core</artifactId>
</dependency>
</dependencies>
3. Configuring Email Properties
Next, configure your email properties in application.properties
or application.yml
. You will need to provide your email service credentials:
propertiesCopy codespring.mail.host=smtp.example.com
spring.mail.port=587
spring.mail.username=your_email@example.com
spring.mail.password=your_password
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
4. Creating the Email Service
Create a service to handle email sending. This service will use Spring Boot's JavaMailSender
to send emails.
javaCopy codeimport org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.stereotype.Service;
@Service
public class EmailService {
@Autowired
private JavaMailSender mailSender;
public void sendEmail(String to, String subject, String text) {
SimpleMailMessage message = new SimpleMailMessage();
message.setTo(to);
message.setSubject(subject);
message.setText(text);
mailSender.send(message);
}
}
5. Sending Email Notifications
Now, let's create a REST controller to handle email notifications.
javaCopy codeimport org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class NotificationController {
@Autowired
private EmailService emailService;
@GetMapping("/sendNotification")
public String sendNotification(@RequestParam String to, @RequestParam String subject, @RequestParam String text) {
emailService.sendEmail(to, subject, text);
return "Email sent successfully!";
}
}
6. Generating and Sending OTPs
To generate OTPs, we can use Spring Security's SecureRandomNumberGenerator
. Let's enhance our EmailService
to include OTP generation.
javaCopy codeimport org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.stereotype.Service;
import java.security.SecureRandom;
@Service
public class OtpService {
private final SecureRandom secureRandom = new SecureRandom();
public String generateOtp() {
int otp = 100000 + secureRandom.nextInt(900000);
return String.valueOf(otp);
}
public String encodeOtp(String otp) {
BCryptPasswordEncoder encoder = new BCryptPasswordEncoder();
return encoder.encode(otp);
}
}
Update the controller to send OTPs:
javaCopy codeimport org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class OtpController {
@Autowired
private EmailService emailService;
@Autowired
private OtpService otpService;
@GetMapping("/sendOtp")
public String sendOtp(@RequestParam String to) {
String otp = otpService.generateOtp();
emailService.sendEmail(to, "Your OTP Code", "Your OTP is: " + otp);
return "OTP sent successfully!";
}
}
7. Conclusion
In this blog, we have covered how to set up a Spring Boot project to send email notifications and OTPs. We configured email properties, created an email service, and set up endpoints to send notifications and OTPs. By following these steps, you can easily integrate email functionality into your Spring Boot application.
Feel free to extend this example by adding more features like email templates, OTP expiration, and verification logic. Happy coding!
Subscribe to my newsletter
Read articles from Nipun Hegde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
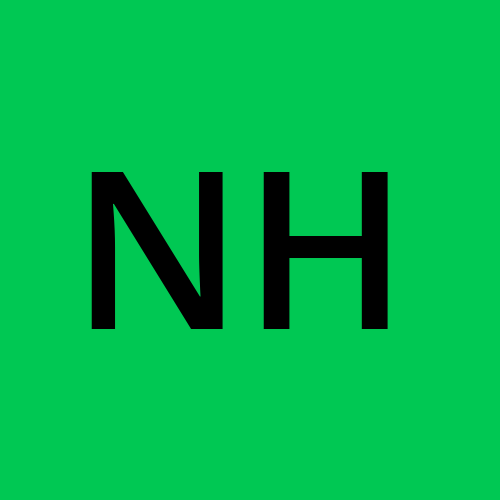
Nipun Hegde
Nipun Hegde
I'm a passionate software engineer always eager to learn and explore new technologies. Beyond coding, I'm deeply interested in DevOps and finance, constantly keeping up with the latest trends and innovations.I also enjoy sharing my knowledge through technical blogs, writing about the exciting things I learn.