Simple Java Interface Code Examples
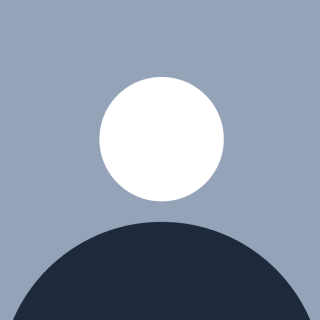
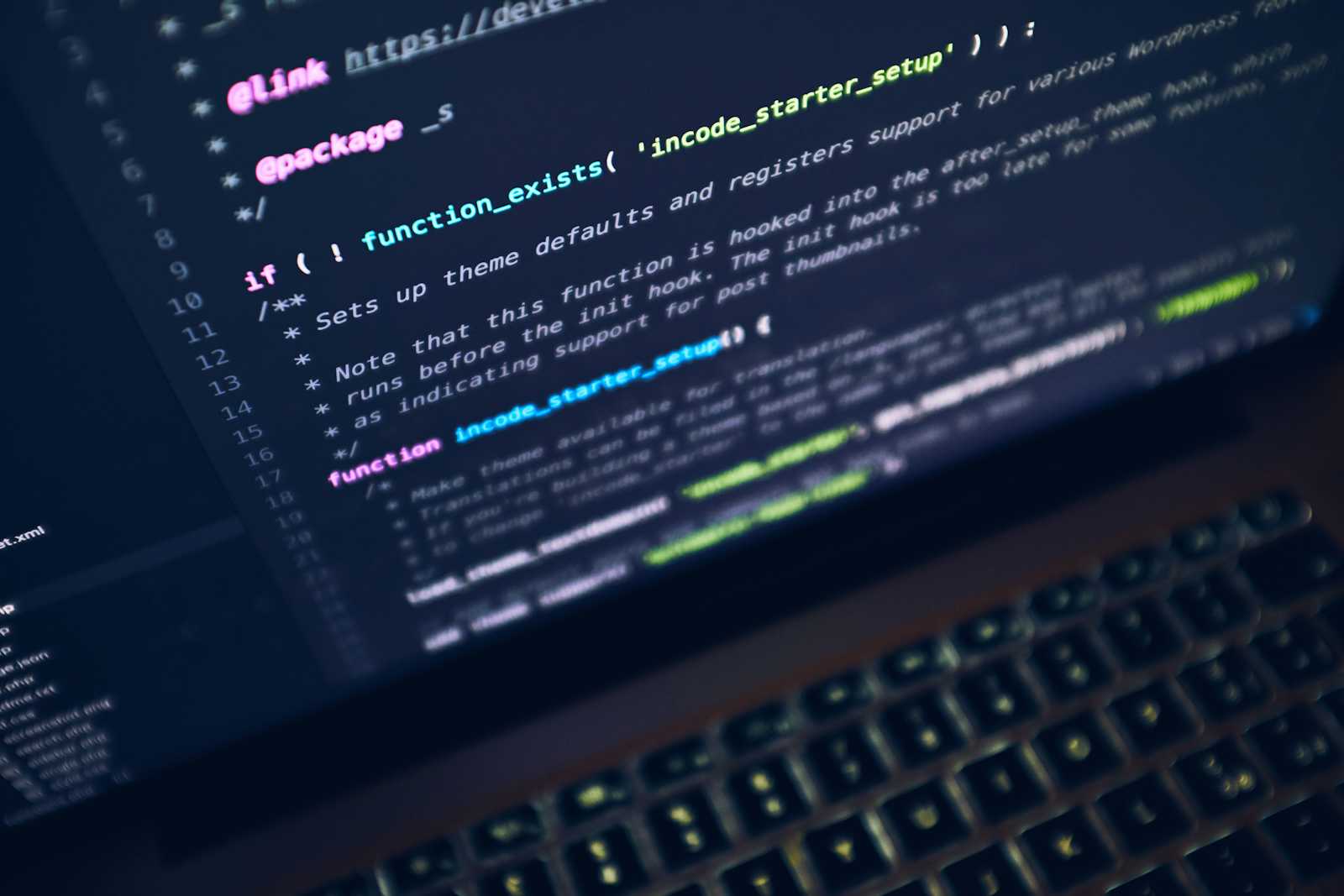
//HERE IN THIS CODE WE IMPLEMENT AN INTERFACE JAVA CODE FOR FACTORIAL AND EVENODD NUMBER CHECK ...
\===============SO THE CODE IS GIVEN BELOW======================
//The first interface in myfirstpr "package"---
package myfirstpr;
public interface I1 {
void fact(int num);
}
//The second interface in myfirstpr "package"---
package myfirstpr;
public interface I2 {
void evenodd(int num);
}
//making new class of "imp" name to implement the interface,you can choose any name of the class ,here you can see the the over-riding concept of the interface--
package myfirstpr;
public class imp implements I1, I2 {
@Override
public void evenodd(int num) {
if(num%2==0) {
System.out.println("The number is even "+num);
}
else {
System.out.println("The number is odd "+num);
}
}
@override
public void fact(int num) {
int fact1=1;
for(int i=1;i<=num;i++) {
fact1=fact1*i;
}
System.out.println("factorial is "+fact1);
}
public static void main(String args[]) {
imp ob = new imp();
ob.fact(10);
ob.evenodd(232);
}
}
//OUTPUT :
factorial is 3628800
The number is even 232
//here the methods like void fact and even odd are given by overriding and the body part means the logic part is written by us.
package myfirstpr;
public interface I1 {
void fact(int num);
}
//next interface I2
package myfirstpr;
public interface I2 {
void evenodd(int num);
}
//implementation in new class
package myfirstpr;
public class imp implements I1, I2 {
@Override
public void evenodd(int num) {
if(num%2==0) {
System.out.println("The number is even "+num);
}
else {
System.out.println("The number is odd "+num);
}
}
@Override
public void fact(int num) {
// TODO Auto-generated method stub
int fact1=1;
for(int i=1;i<=num;i++) {
fact1=fact1*i;
}
System.out.println("factorial is "+fact1);
}
public static void main(String args[]) {
imp ob = new imp();
ob.fact(10);
ob.evenodd(232);
}
}
Subscribe to my newsletter
Read articles from SUDHIR PATEL directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by