Streamline Your Laravel Workflow with Laravel Mix
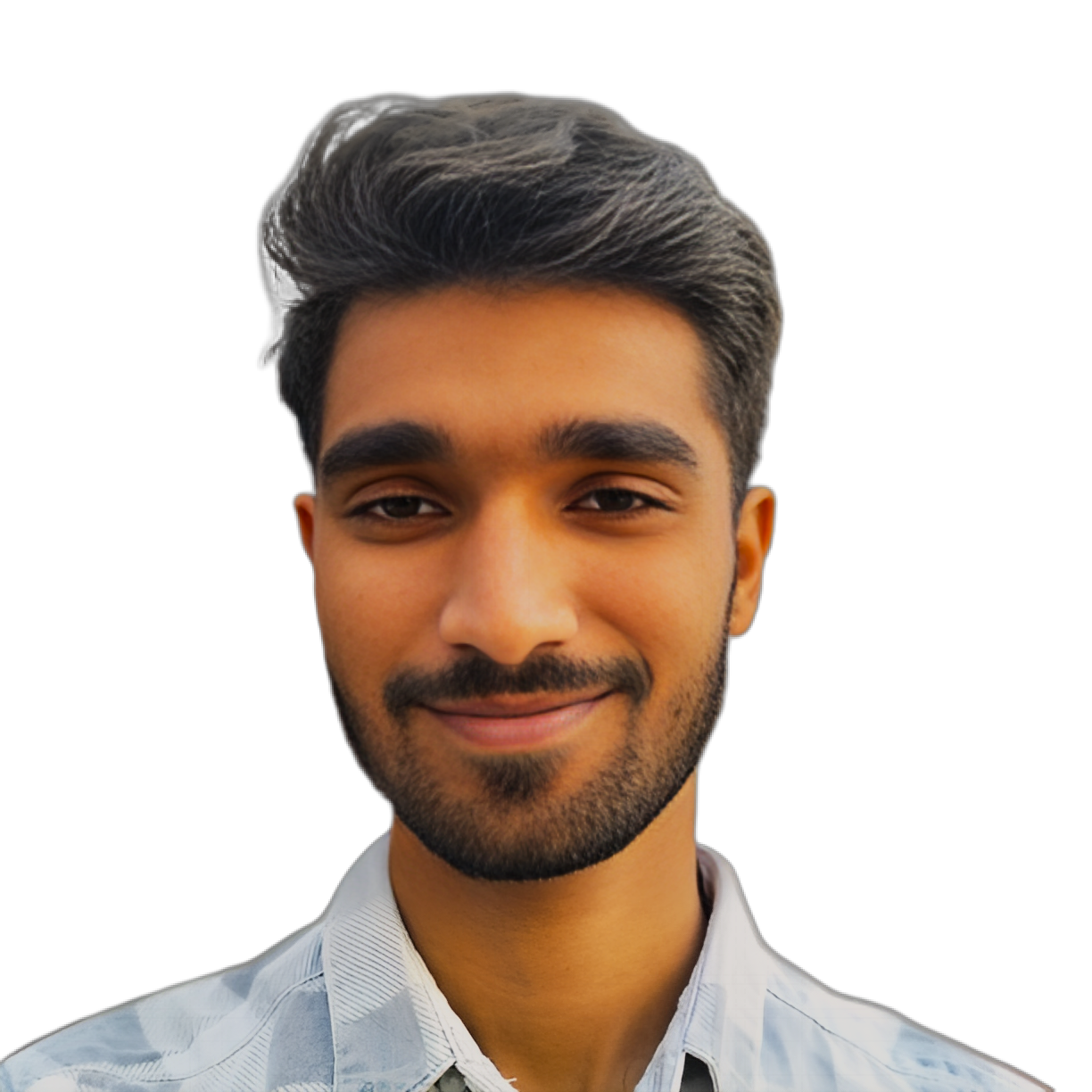
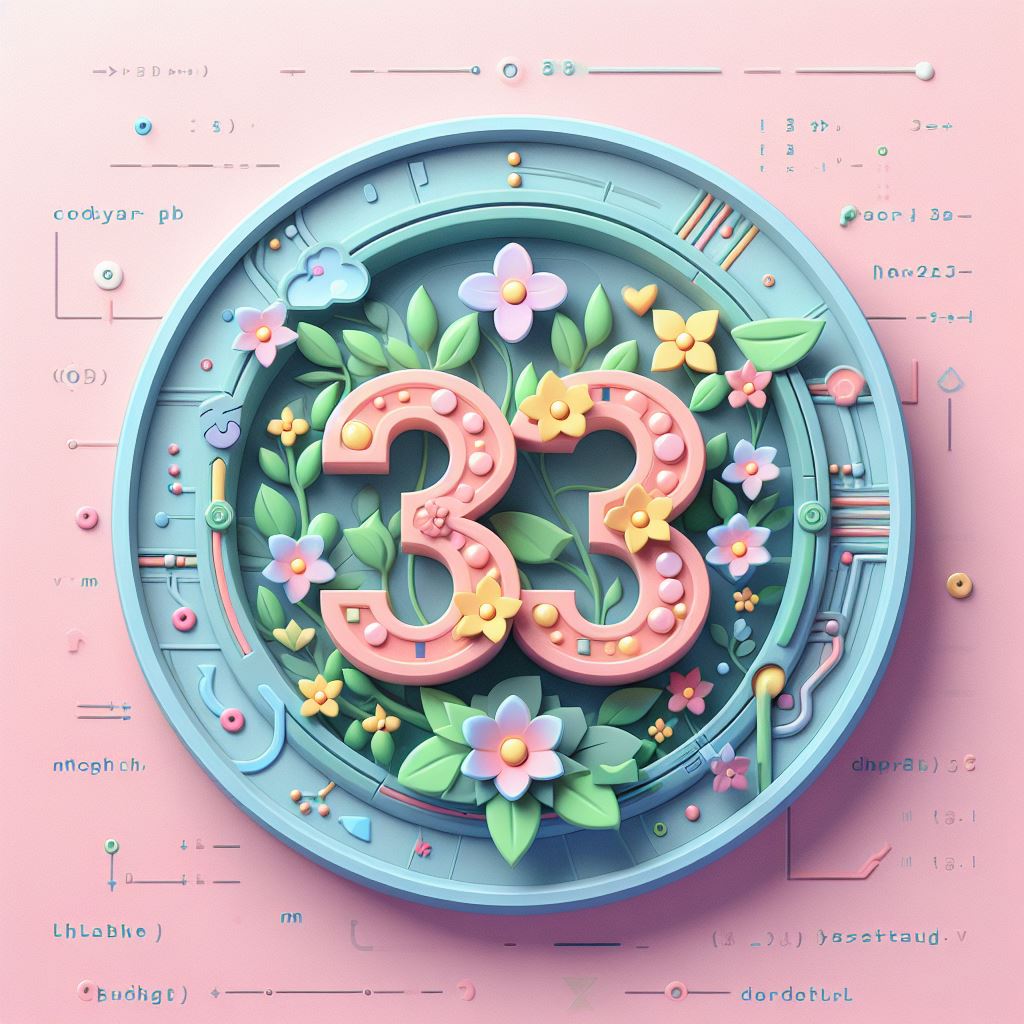
Laravel is a fantastic framework for building modern web applications. But managing assets like CSS, JavaScript, and fonts can become cumbersome as your project grows. That's where Laravel Mix comes in! This powerful tool simplifies asset compilation and management, allowing you to focus on what matters most - building amazing features.
What is Laravel Mix?
Laravel Mix acts as a wrapper around Webpack, a popular module bundler. It provides a clean, developer-friendly API that simplifies complex Webpack configurations. With Laravel Mix, you can:
Effortlessly compile CSS, JavaScript, and fonts
Leverage popular preprocessors like Sass, Less, and CoffeeScript
Automatically add vendor prefixes for cross-browser compatibility
Minify code and generate source maps for optimized production builds
Benefits of Using Laravel Mix
Increased Productivity: Spend less time configuring Webpack and more time building features.
Simplified Workflow: A clean API makes asset management a breeze.
Improved Code Quality: Leverage preprocessors for cleaner and more maintainable code.
Cross-Browser Compatibility: Ensure your styles work seamlessly across different browsers.
Production-Ready Builds: Minified code for faster loading times and source maps for easier debugging.
Getting Started with Laravel Mix
Prerequisites:
A Laravel project
Node.js and npm (or yarn) installed on your system
Installation:
Open your terminal and navigate to your Laravel project's root directory.
Run the following command to install Laravel Mix:
Bash
npm install laravel-mix --save-dev
This command installs Laravel Mix and adds it to your project's development dependencies.
Creating a Mix Configuration File:
Create a new file named
webpack.mix.js
in the root of your Laravel project.This file will house your asset compilation settings and tasks.
Basic Example:
Let's create a basic example to compile a Sass file and a JavaScript file:
JavaScript
const mix = require('laravel-mix');
mix.sass('resources/assets/sass/app.scss', 'public/css')
.js('resources/assets/js/app.js', 'public/js');
Explanation:
We require the
laravel-mix
package.We use the
mix
object to define our compilation tasks.The
mix.sass
method compiles theapp.scss
file located in theresources/assets/sass
directory and outputs the compiled CSS topublic/css/app.css
.The
mix.js
method compiles theapp.js
file located in theresources/assets/js
directory and outputs the compiled JavaScript topublic/js/app.js
.
Compiling Assets:
To compile your assets, run the following command in your terminal:
Bash
npm run dev
This command will trigger the compilation process defined in your webpack.mix.js
file. By default, Laravel Mix watches for changes in your asset files and automatically recompiles them whenever you make modifications.
Additional Features:
Laravel Mix offers a wide range of features beyond basic compilation. Here are some additional functionalities you can explore:
Preprocessor Support: As mentioned earlier, Laravel Mix seamlessly integrates with popular preprocessors like Sass, Less, and CoffeeScript.
Vendor Prefixing: Automatically add vendor prefixes to your CSS styles for cross-browser compatibility.
Code Splitting: Break down your JavaScript code into smaller chunks for faster initial page load times.
Versioning: Generate unique filenames for your compiled assets to prevent caching issues during deployments.
Conclusion
Laravel Mix is a valuable tool for any Laravel developer. It simplifies asset management, improves code quality, and streamlines your development workflow. By leveraging its features, you can focus on building amazing Laravel applications without getting bogged down in complex build configurations.
Ready to take your Laravel development to the next level? Give Laravel Mix a try and experience the benefits for yourself!
Additional Resources:
Laravel Mix Documentation: https://laravel.com/docs/8.x/mix
Laravel Asset Compilation: https://stackoverflow.com/questions/50941780/how-to-use-scss-files-in-laravel
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
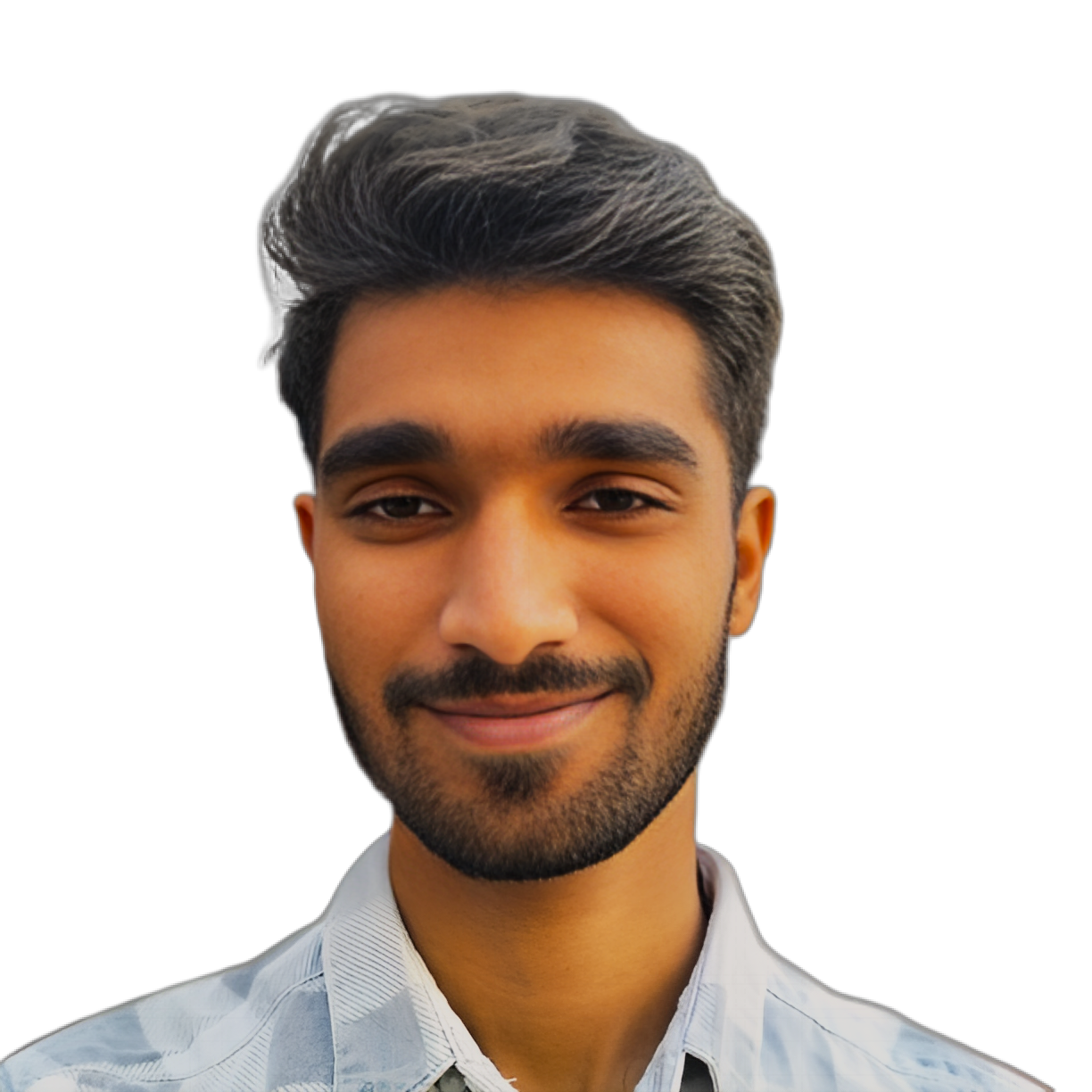
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.