Mastering Flutter BLoC Pattern: CRUD for Seamless State Management
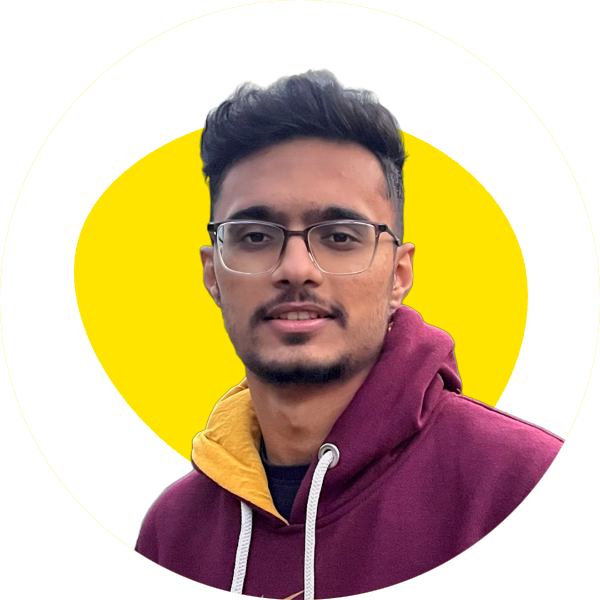
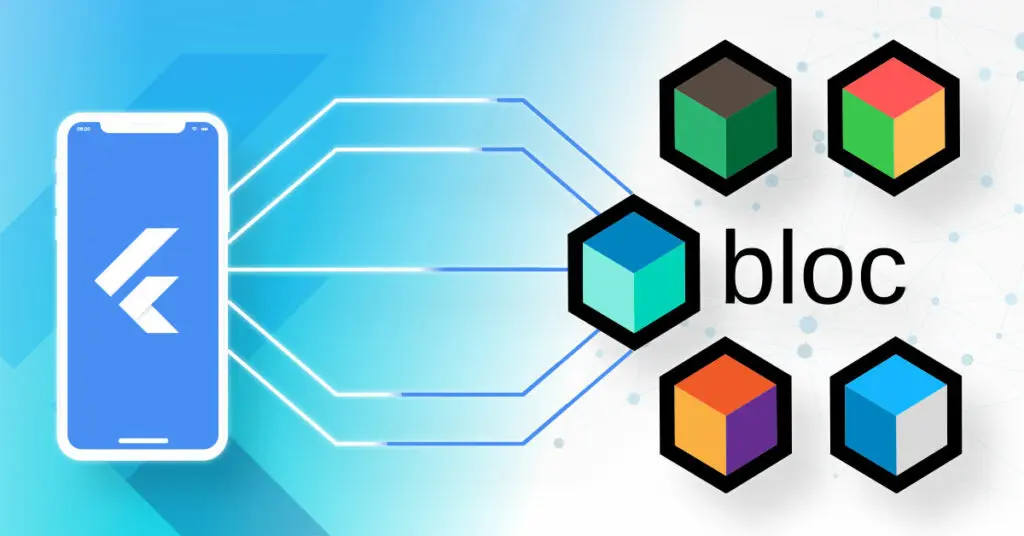
๐ Unleash the Power of BLoC in Flutter
Maintaining and updating the state of your Flutter application can be a daunting task, but with the BLoC (Business Logic Component) pattern, you can take your development skills to the next level. This comprehensive tutorial will guide you through the fundamental concepts and practical implementation of the BLoC pattern using the flutter_bloc
package, empowering you to create robust and scalable Flutter applications.
๐ Understanding the BLoC Pattern
The BLoC pattern is a state management solution that helps you decompose your application's state into smaller, well-defined state machines. These state machines, known as BLoCs, transform events into one or more states, providing a clear and organized way to manage the state of your Flutter app.
The Key Components of BLoC
Events: These are the user actions or external triggers that initiate changes in your application's state.
States: These represent the different conditions or configurations of your application's state.
BLoCs: These are the state machines that handle the transformation of events into states, encapsulating the business logic of your application.
๐ ๏ธ Implementing BLoC in a Flutter App
In this tutorial, we'll dive into a practical example of using the BLoC pattern to create, read, update, and delete (CRUD) users in a Flutter application. Let's get started!
Setting up the BLoC Dependencies
To use the BLoC pattern in your Flutter app, you'll need to add the flutter_bloc
package to your pubspec.yaml
file:
dependencies:
flutter_bloc: ^8.1.1
Defining the User Model
We'll start by creating a User
model that represents the data we'll be managing in our application. This model will have properties like name
, email
, and id
.
Implementing the User List BLoC
Now, let's create the UserListBloc
that will handle the CRUD operations for our users:
UserListEvent
We'll define the events that can be triggered on the UserListBloc
:
AddUserEvent: Triggered when a new user is added.
DeleteUserEvent: Triggered when a user is deleted.
UpdateUserEvent: Triggered when a user is updated.
UserListState
The UserListState
will represent the different states of the user list:
UserListInitial: The initial state with an empty list of users.
UserListUpdated: The state when the list of users has been updated.
UserListBloc
The UserListBloc
will implement the logic for handling the user list events and updating the state accordingly:
addUser: Adds a new user to the list and emits the
UserListUpdated
state.deleteUser: Removes a user from the list and emits the
UserListUpdated
state.updateUser: Updates an existing user in the list and emits the
UserListUpdated
state.
Using the UserListBloc in the UI
Now, let's integrate the UserListBloc
into our Flutter app's UI. We'll use the BlocBuilder
widget to rebuild the UI whenever the state of the UserListBloc
changes.
Displaying the User List
We'll create a UserListView
widget that displays the list of users using a ListView.builder
. Each user will be represented by a UserTile
widget.
Adding a New User
We'll add a floating action button to the app that, when pressed, will display a bottom sheet for creating a new user. The bottom sheet will have text fields for the user's name and email, and an "Add" button that will trigger the AddUserEvent
.
Updating an Existing User
We'll also add an edit button to each UserTile
that, when pressed, will display a bottom sheet for updating the user's information. The bottom sheet will have pre-filled text fields for the user's name and email, and an "Update" button that will trigger the UpdateUserEvent
.
Deleting a User
Finally, we'll add a delete button to each UserTile
that, when pressed, will trigger the DeleteUserEvent
to remove the user from the list.
๐ Elevate Your Flutter Development with BLoC
By mastering the BLoC pattern, you'll be able to create highly maintainable and scalable Flutter applications with a clear separation of concerns between the presentation and business logic. This approach will make your code more organized, testable, and easier to reason about, ultimately improving the overall quality and robustness of your Flutter projects.
Subscribe to my newsletter
Read articles from Nitin Chandra Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
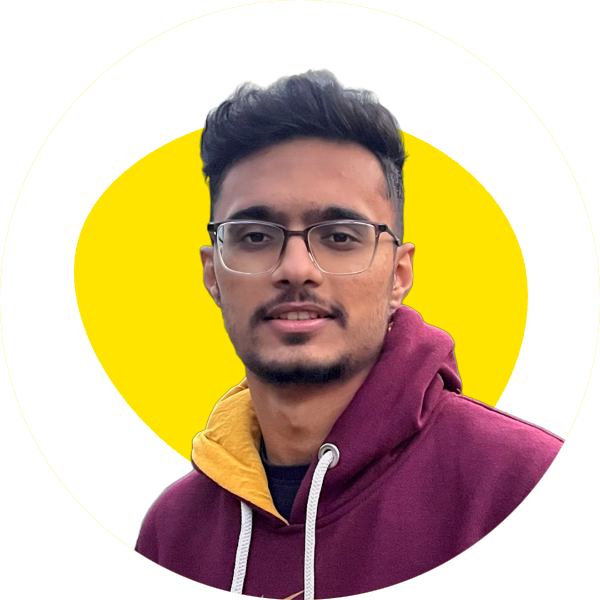