All About the Other Python Iterables: Dictionaries

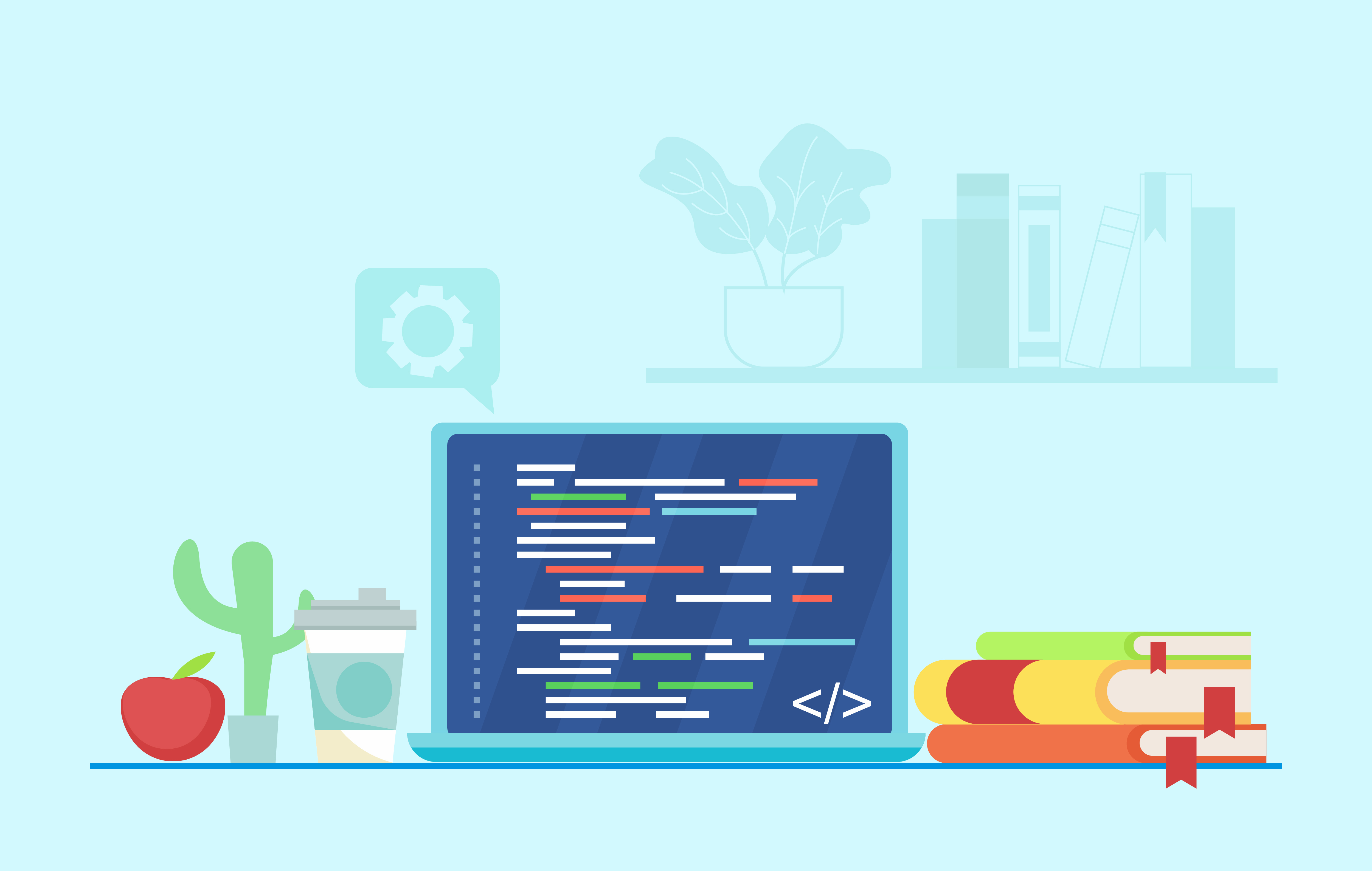
As far as “collectible” data types go, we’ve looked at lists, tuples and sets. In this episode of data types that house other data types, we’d be looking at dictionaries. This type stands out from the others because it has key-value pairs which is a programming-fancy way of saying each key has its value, similar to how you would find keys and values in a dictionary. For instance if I was to find the meaning of the word: “Physics” I may find the definition “Field of Science that causes much migraine to learner” or more accurately “The Scientific study of matter and energy and the relationship between them including the study of forces, heat…” Here we may consider the word Physics to be the key and its definition the value. In another example, if I called out “John!” in a crowded market and there was only one John there (for simplicity’s sake), John would answer to his name. John could be the key to identifying him and the features that make him well…him (his value). If Oche who isn't named John answered, that would be really confusing. Dictionaries can be identified because they look like below;
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#A typical Python dictionary
Dictionaries generally do not allow duplicates, they are mutable and they are ordered (This feature being relatively new from Python 3.7 as previously, they were unordered).
Accessing Dictionary Items
How do we go about gaining access to items in dictionaries and doing stuff with them? We can get single items easily using the get() method and using square brackets. With square brackets, we place the dictionary name and then key of the item in a square bracket;
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
print(Scientists["Charles Darwin"])
#This would print "Biology"
For the get() method:
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
print(Scientists.get("Einstein"))
#This would print "Physics"
If you’d rather print out a list of all the keys in the dictionary, you can do so easily as seen below. Likewise printing all values follows the same pattern;
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#This would print all the keys in the dictionary in list form
print(Scientists.keys())
#This would print all the values in the dictionary in list form
print(Scientists.values())
What if you would want to print out all items in the dictionary? You could use the items() method. Here, tuples of key/value pairs are contained in lists and printed, just like below:
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#This would print all items in the dictionary
print(Scientists.items())
Altering Dictionary Items
If you would like to make changes to dictionary keys by changing their values, you can do so as shown by the snippet below;
Scientists = {
"Einstein": "discovered Relativity",
"Charles Darwin": "known for the theory of Evolution",
"Robert Hooke": "Did a lot, but known for strings",
"Amanda Adoyi": "Not even sure what to eat yet"
}
print(Scientists)
#I can modify the dictionary, using square brackets.
Scientists["Amanda Adoyi"] = "discovered Relativity"
Scientists["Einstein"] = "did Sciency stuff"
print(Scientists)
As you can see from the snippet above, I apparently beat Einstein to discovering relativity, all thanks to my ability to modify dictionaries.
You can also make changes to dictionaries by using the update() method.
Scientists = {
"Einstein": "discovered Relativity",
"Charles Darwin": "known for the theory of Evolution",
"Robert Hooke": "Did a lot, but known for strings",
"Amanda Adoyi": "Not even sure what to eat yet"
}
print(Scientists)
#I can modify the dictionary, using the update method.
Scientists.update({"Amanda Adoyi": "discovered Relativity"})
Scientists.update({"Einstein": "did Sciency stuff"})
print(Scientists)
Adding and Removing Dictionary Items
When adding items to dictionaries we simply include the new key and assign it to a value like below:
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#This would add Nikola Tesla to the dictionary
Scientists["Nikola Tesla"] = "Engineering"
print(Scientists)
Just like with modifying items, a similar thing can be achieved using the update() method.
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#This would add Nikola Tesla to the dictionary
Scientists.update({"Nikola Tesla" : "Engineering"})
print(Scientists)
Removing Dictionary Items
Using pop(), clear(), popitem() and del we can delete whole dictionaries or remove items from them.
Scientists = {
"Einstein": "discovered the Relativity theory",
"Charles Darwin": "known for the theory of Evolution",
"Robert Hooke": "Did a lot, but known for strings",
"Amanda Adoyi": "Not even sure what to eat yet"
}
#pop method removes the item with the specified key name
#here, "Amanda Adoyi" is removed as specified
Scientists.pop("Amanda Adoyi")
#clear removes all the items in the specified dictionary
Scientists.clear()
#popitem removes the last added dictionary item. This only occurs
#in python versions 3.7 and up however; prior to that, random items
#were removed.
Scientists.popitem()
#del removes specified items or the whole dictionary
del Scientists["Amanda Adoyi"] #removes "Amanda Adoyi"
del Scientists #deletes the whole dictionary
Nesting and Accessing Items in Nested Dictionaries
Just like with the other iterables, we can nest dictionaries which essentially means we place dictionaries in dictionaries in dictionaries…you get the point.
Scientists = {
"Polymaths":
{
"Robert Hooke": "Worked in several fields",
"Leonardo da Vinci": "Still doubt he was human",
},
"Champions of Quantum Mechanics":
{
"Erwin Schrodinger": "Something about his cat",
"Karl Heisenberg" : "Was uncertain about a lot of things",
"Max Planck" : "Got really lucky it seems"
},
"probablyVillains":
{
"Thomas Edison": "Genius but seemed selfish",
"Isaac Newton" : "Genius but Kind of a sadist"
}
}
print(Scientists["probablyVillains"]["Thomas Edison"])
#This prints "Genius but seemed selfish"
As you can see from above, we can easily access singular items in these dictionaries in a way similar to how they are handled with lists.
So we see that in order to access value “Genius but seemed selfish” that’s linked to the key “Thomas Edison” we must access the dictionary “Scientists” and then “probablyVillains” before arriving at “Thomas Edison” which would print the value.
Looping Through Dictionaries
This is fairly easy to understand if you’ve read my article on loops and control statements. We can use the for loops to loop through dictionaries in the following ways:
Scientists = {
"Einstein": "Physics",
"Charles Darwin": "Biology",
"Robert Hooke": "Biology, Chemistry and Enginee...I'm confused"
}
#print keys
for i in Scientists:
print(i)
for i in Scientists.keys():
print(i)
#print values
for i in Scientists:
print(Scientists[i])
for i in Scientists.values():
print(i)
#print keys and values
for i,j in Scientists.items():
print(i,j)
If you’d like to see a list of dictionary methods and definitions not mentioned here, check out this article.
Till next time!
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.