An Improved Method for Building APIs using Fast Endpoints
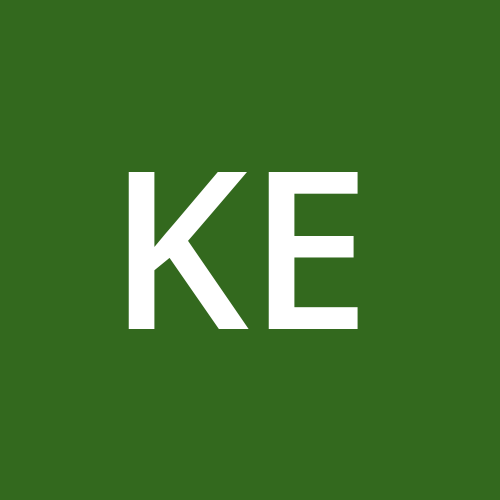
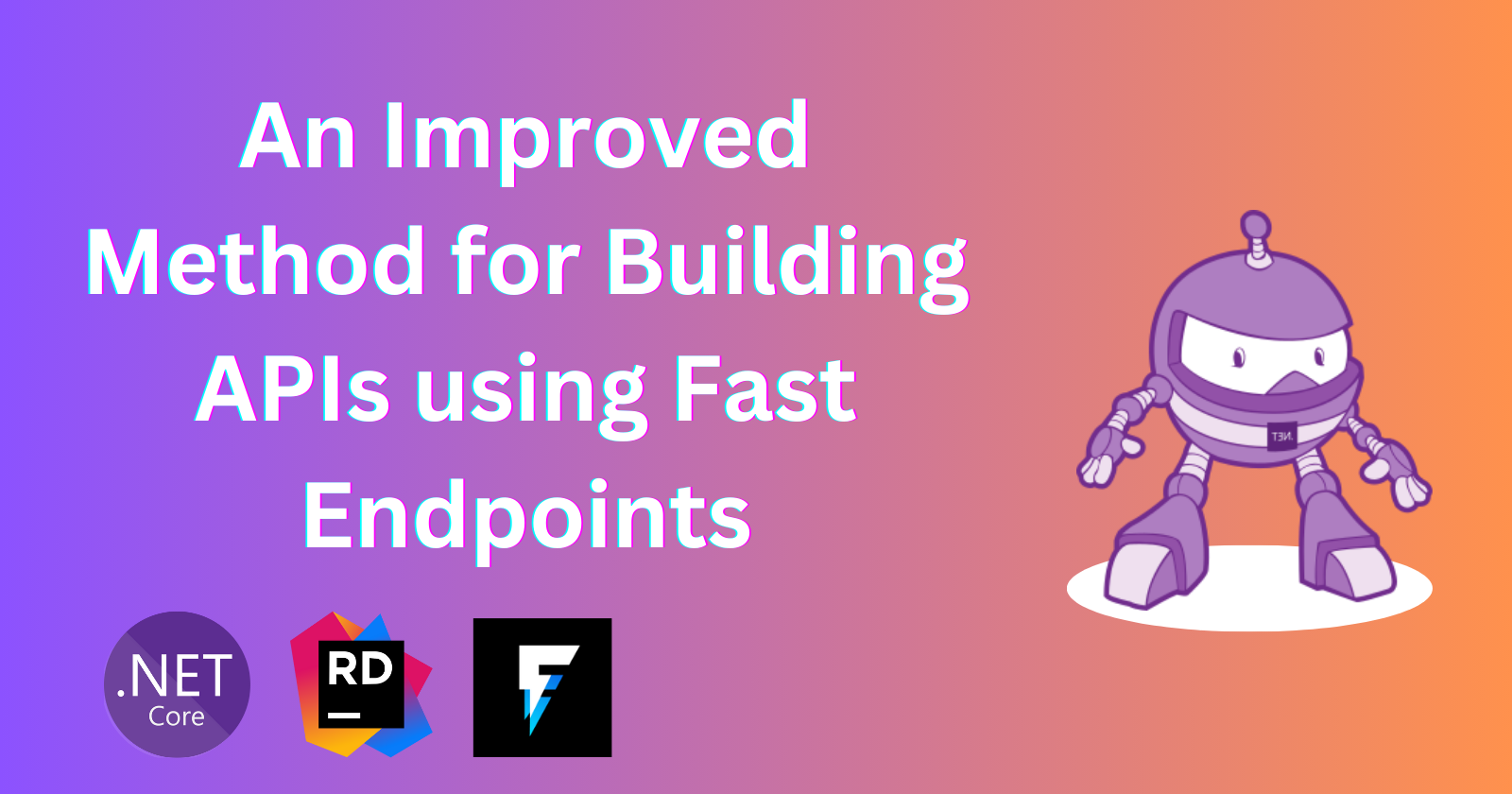
While researching, I discovered a library called Fast Endpoints, which immediately intrigued me. At the time, I had been experimenting with Minimal APIs, appreciating their simplicity and performance compared to MVC Controllers. However, I found that anything beyond basic implementations quickly cluttered the Program.cs
file, necessitating a better approach to code organization.
Having been a strong advocate for the Mediatr library due to its ability to create clean, vertically sliced, and SOLID code that supports versioning, I was disappointed by its reliance on MVC Controllers, which created less than ideal horizontal layers.
Fast Endpoints provides a robust solution, addressing the organisational challenges of Minimal APIs while leveraging the performance benefits. Follow this step-by-step guide to build APIs using Fast Endpoints.
Step 1: Set up your project
Instead of setting up a project from scratch, I want to refer to Building a Web API in .NET article where I have used a minimal and a quick alternative which might work or not depending on the size of your project.
You can get started here: https://fast-endpoints.com/docs/get-started#create-project-install-package
For now, let's install Fast Endpoint and its dependencies. In your project directory, run the following command to install Fast Endpoints:
dotnet add package FastEndpoints
Since I'm using Rider, you can search and install it using Nuget Package Manager:
Let's first update of program.cs file:
using FastEndpoints;
using FastEndpoints.Swagger;
var builder = WebApplication.CreateBuilder(args);
//Add this
builder.Services
.AddFastEndpoints()
.SwaggerDocument();
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
//Add this
app.UseFastEndpoints(c =>
{
c.Endpoints.RoutePrefix = "api";
}).UseSwaggerGen();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseHttpsRedirection();
app.Run();
Now, let's update our endpoints to use Fast Endpoint. Your endpoint should inherit from Endpoint<YourRequestDto, YourResponseDto>. See the examples below
using BookStore.Contracts.Books;
using FastEndpoints;
namespace BookStore.HttpApi.Endpoints.Books;
public class CreateBook: Endpoint<CreateBookInputDto, BookDto>
{
public override void Configure()
{
Post("books/create");
AllowAnonymous();
}
public override async Task HandleAsync(CreateBookInputDto req, CancellationToken ct)
{
//A placeholder, replace this with a call to your db
var res = new BookDto()
{
Title = req.Title,
Isbn = req.Isbn,
PublishedDate = req.PublishedDate
};
await SendAsync(res);
}
}
using FastEndpoints;
using BookStore.Contracts.Books;
namespace BookStore.HttpApi.Endpoints.Books;
public class GetAll : EndpointWithoutRequest<List<BookDto>>
{
public override void Configure()
{
Get("books");
AllowAnonymous();
}
public override async Task HandleAsync(CancellationToken ct)
{
//A placeholder, replace this with a call to your db
await SendAsync(new());
}
}
using FastEndpoints;
using BookStore.Contracts.Books;
using BookStore.Contracts.Requests.Books;
namespace BookStore.HttpApi.Endpoints.Books;
public class GetBookById : Endpoint<GetByIdRequest, BookDto>
{
public override void Configure()
{
Get("books/{bookId}");
AllowAnonymous();
}
public override async Task HandleAsync(GetByIdRequest req, CancellationToken ct)
{
//A placeholder, replace this with a call to your db
var res = new BookDto()
{
Title = "Random",
Isbn = "Random",
PublishedDate = new DateTime()
};
await SendAsync(res);
}
}
Conclusion
By following this guide, you have created a basic API using Fast Endpoints. This approach provides a clean and organized way to define and manage your endpoints, leveraging the simplicity and performance of Minimal APIs while avoiding clutter in your Program.cs
file. As you become more familiar with Fast Endpoints, you can extend your API with additional features and complexities as needed. Happy coding!
Subscribe to my newsletter
Read articles from Khalil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
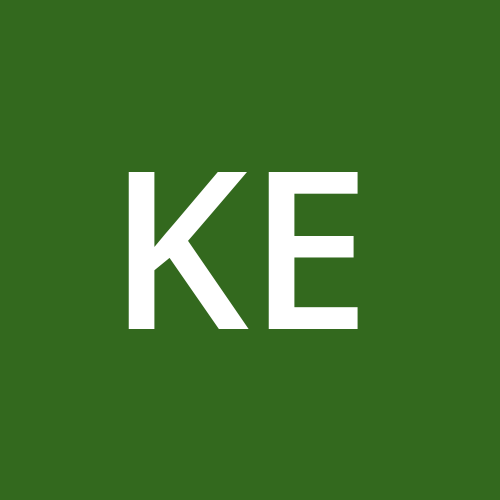