Day 93/100 100 Days of Code
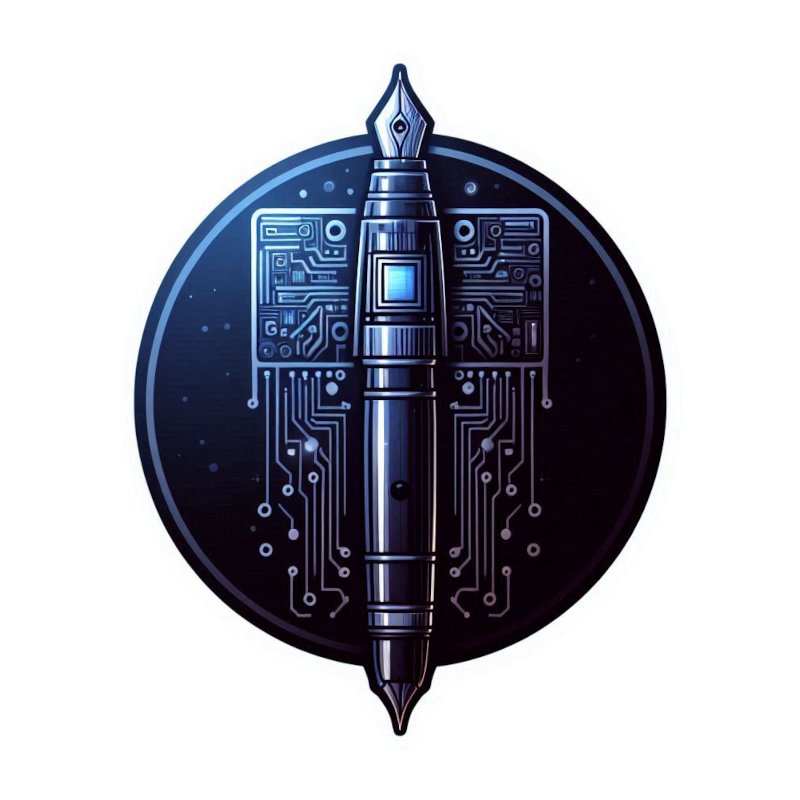
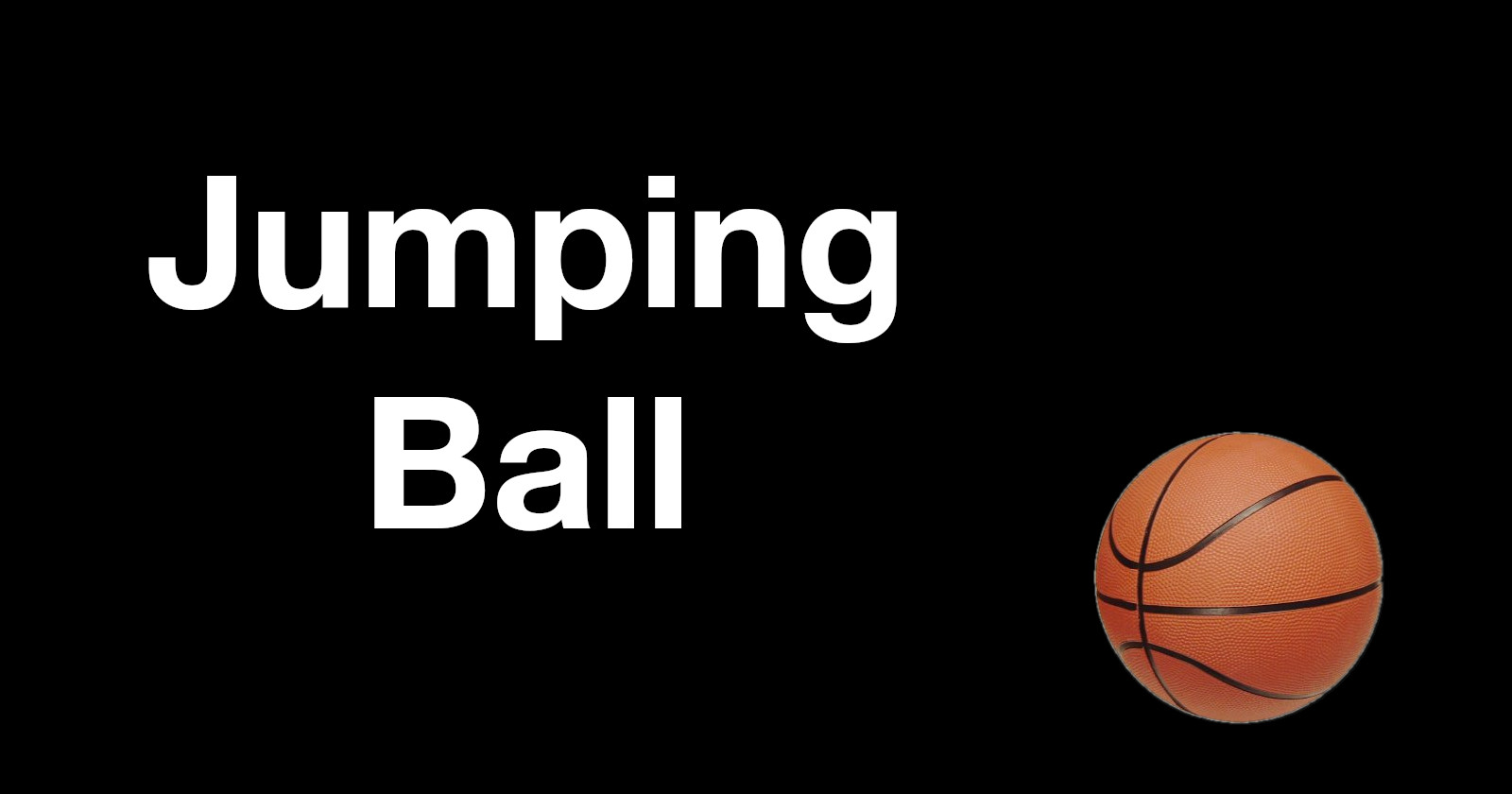
I worked on fixing some very strange issues with strings. Similar to the TTF_OpenFont
command, using miniApplication.location
changed it, which resulted in using the wrong directory path.
This is very strange behaviour. To fix it, I created a new const char*
variable and assigned the location of the miniApplication
to it. This solved the problem, but I am not sure why the string gets changed. The solution is not very pretty and I need to do some adjustments. the location
of the Resource
structure should be const.
const char *miniAppModuleLocation = miniApplication.location;
printf("%s\n", miniAppModuleLocation);
char *testCommand = "test -f ";
char *fileLocation = "app/output/calculations.csv";
char *createCommand = calloc(strlen(testCommand) + strlen(miniAppModuleLocation) + strlen(fileLocation),
sizeof(char));
strcpy(createCommand, testCommand);
strcat(createCommand, miniAppModuleLocation);
strcat(createCommand, fileLocation);
printf("%s\n", createCommand);
int getCalcFile = system(createCommand);
printf("%s\n", createCommand);
free(createCommand);
if (getCalcFile == 0)
{
char *cdCommand = "cd ";
char *removeCommand = " && rm calculations.csv";
createCommand = calloc( strlen(miniApplication.location) + strlen(cdCommand) + strlen(removeCommand),
sizeof(char));
getCalcFile = system(createCommand);
free(createCommand);
if (getCalcFile != 0)
{
printf("Calculations CSV removal failed\n, %d", errno);
exit(EXIT_FAILURE);
}
}
Also, I updated the GetResults function to read the output CSV file. It is still very buggy but it's getting there.
struct Results GetResults(char *location, char *value)
{
struct Results results;
char *commandPartFirst = "cd ";
char *commandPartSecond = " && fpm run -- ";
char *command = calloc( strlen(location) + strlen(value) + strlen(commandPartFirst) + strlen(commandPartSecond),
sizeof(char));
char *contentsCSV = "/app/output/calculations.csv";
char *csvLocation = calloc(strlen(location) + strlen(contentsCSV), sizeof(char));
strcpy(csvLocation, location);
strcat(csvLocation, contentsCSV);
printf("%s\n", csvLocation);
strcpy(command, commandPartFirst);
strcat(command, location);
strcat(command, commandPartSecond);
strcat(command, value);
int getSystemInfo = system(command);
if (getSystemInfo != 0)
{
printf("Something went wrong!\n");
results.maxHeight = 0;
results.timeToMaximumHeight = 0;
results.timeToLand = 0;
return results;
}
FILE *file = fopen(csvLocation, "r");
char line[1024];
int counter = 0;
while(fgets(line, 1024, file))
{
if (counter == 0)
{
counter++;
continue;
}
char *temp = strdup(line);
char *stopFirstString;
char *stopSecondString;
char *stopThirdString;
printf("Get Result 1: %s\n", GetField(temp, 0));
printf("Get Result 2: %s\n", GetField(temp, 1));
printf("Get Result 3: %s\n", GetField(temp, 2));
results.maxHeight = strtod(GetField(temp, 0), &stopFirstString);
results.timeToMaximumHeight = strtod(GetField(temp, 1), &stopSecondString);
results.timeToLand = strtod(GetField(temp, 2), &stopThirdString);
free(temp);
}
printf("%s\n", command);
free(command);
free(csvLocation);
fclose(file);
return results;
}
Subscribe to my newsletter
Read articles from Chris Douris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
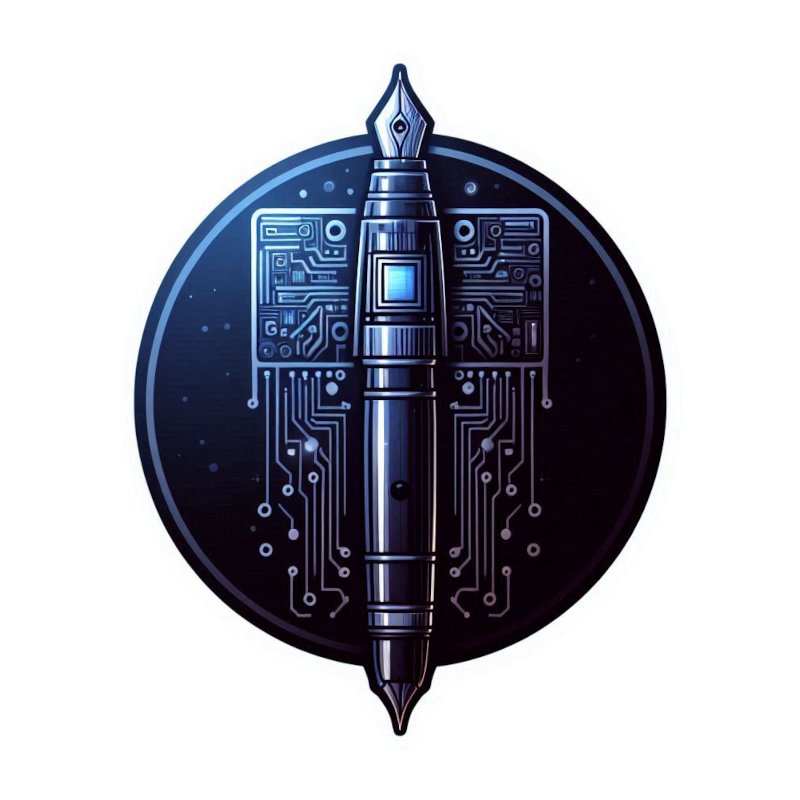
Chris Douris
Chris Douris
AKA Chris, is a software developer from Athens, Greece. He started programming with basic when he was very young. He lost interest in programming during school years but after an unsuccessful career in audio, he decided focus on what he really loves which is technology. He loves working with older languages like C and wants to start programming electronics and microcontrollers because he wants to get into embedded systems programming.