Understanding the Single Responsibility Principle (SRP) of SOLID Principles
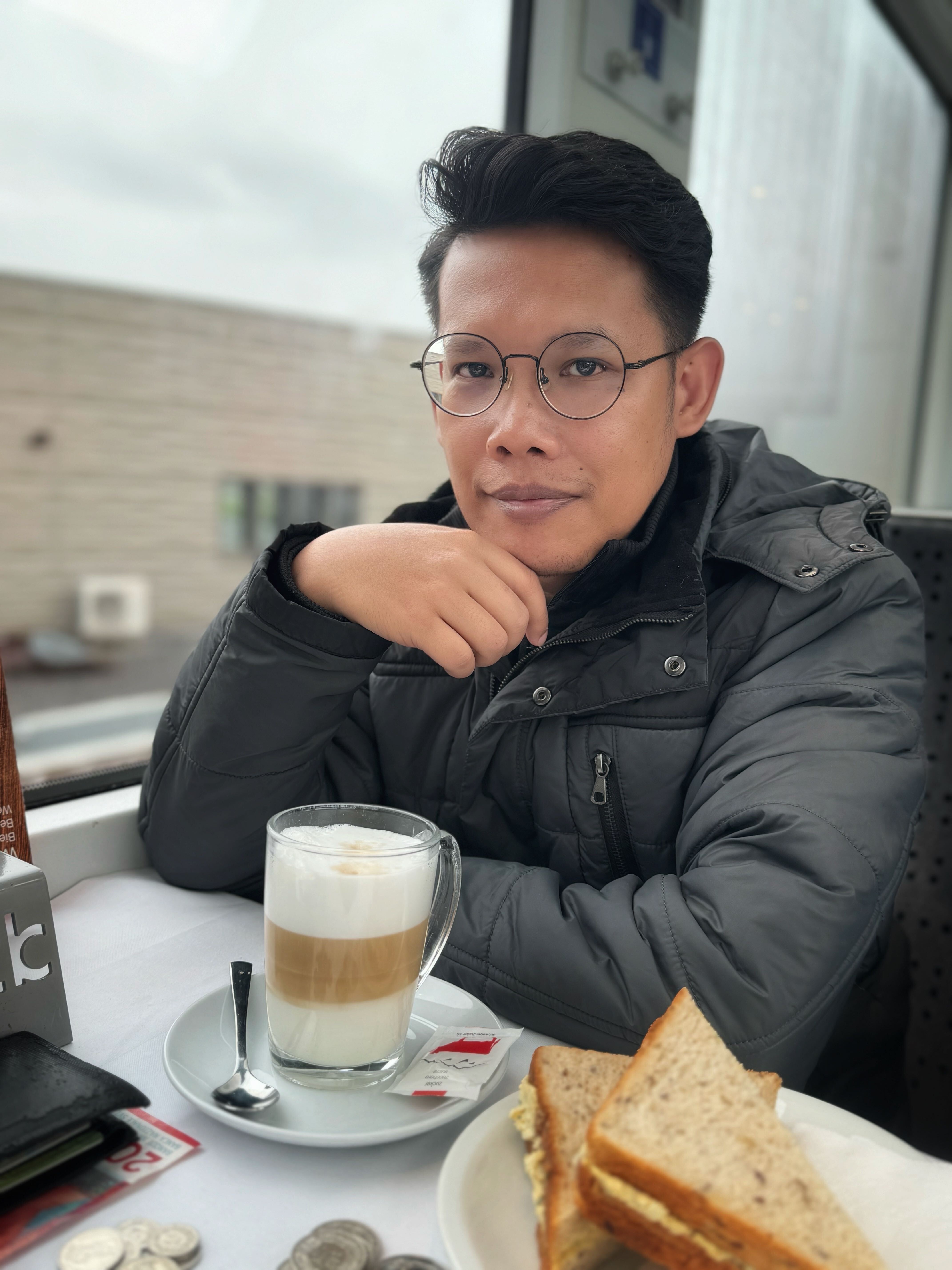
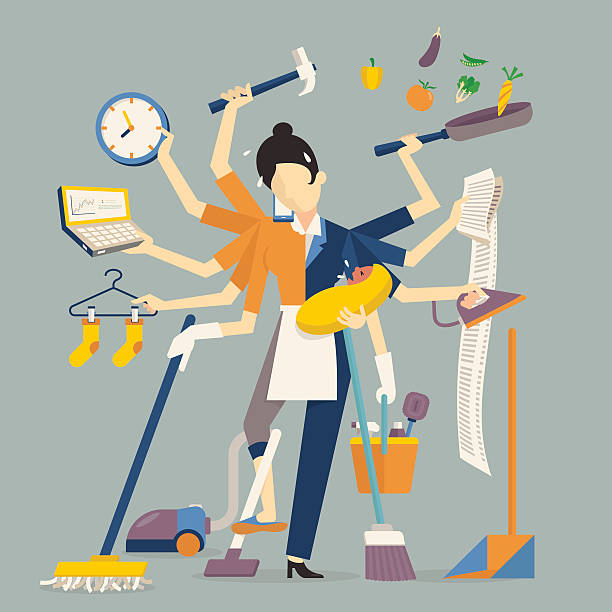
When it comes to writing good code, there's a set of principles known as SOLID that can help make your software more understandable, flexible, and easier to maintain. Let’s dive into what these principles are and focus specifically on the Single Responsibility Principle (SRP).
A Refresher: What are SOLID Principles?
As a quick refresher, especially if you've read my previous article about SOLID, these principles stand for:
Single Responsibility Principle (SRP): A class should have only one reason to change, meaning it should only have one job or responsibility.
Open-Closed Principle (OCP): Software entities should be open for extension but closed for modification. This allows you to add new functionality without altering existing code.
Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types. Any subclass should be usable in place of its parent class without the user noticing.
Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use. A class should only implement methods that are of interest to it.
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
The Single Responsibility Principle (SRP)
Today's article will focus specifically on the Single Responsibility Principle (SRP). The rest of the principles will be covered in future articles.
The Single Responsibility Principle is all about making sure that a class only does one thing. There should be only one reason for the change. This can significantly simplify your code and make it more maintainable.
Benefits of SRP:
Easier to Understand and Maintain: When a class has a single responsibility, you can easily understand its purpose and functionality without being distracted by unrelated tasks.
Reduced Risk of Bugs: Modifying a class with one responsibility lowers the risk of introducing bugs in unrelated parts of the system.
Improved Testability: Testing is simpler and more straightforward because each class has a clear focus.
Increased Reusability: Classes with a single responsibility are more likely to be reusable in different contexts or applications.
Easier to Scale and Refactor: It’s simpler to extend or refactor parts of your application when each class has a single responsibility.
Example of SRP Violation
Let’s illustrate how the Book
class might violate SRP. Here's an example where the Book
class does too much:
public class Book {
private String title;
private String author;
private String text;
// Constructor
public Book(String title, String author, String text) {
this.title = title;
this.author = author;
this.text = text;
}
// Getters
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getText() {
return text;
}
// Method to print the book's text to the console
public void printTextToConsole() {
System.out.println(text);
}
// Method to save the book's text to a file
public void saveTextToFile(String filePath) {
try (FileWriter writer = new FileWriter(filePath)) {
writer.write(text);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Alright, let's break down what's happening here. We have a Book
class that does a lot of things:
Storing Book Data: It holds the book’s title, author, and text.
Printing to Console: It has a method to print the book's text to the console.
Saving to a File: It has another method to save the book's text to a file.
Now, why is this a problem?
Mixing Responsibilities
Imagine you’re working on a project where you need to change how the book's data is saved to a file. Maybe you need to change the file format or the location where it's saved. Because the Book
class is handling this, you have to modify the Book
class itself. This means every time you want to change the saving mechanism, you risk breaking something else in the Book
class, like printing to the console or the book data storage.
- Harder to Maintain and Test
When a class has multiple responsibilities, it becomes harder to maintain and test. If you want to test the book’s data storage, you might inadvertently run into issues with the file-saving functionality or the console printing functionality. This makes debugging more difficult and increases the chances of introducing bugs.
So, how do we fix this?
To fix this, we should separate these responsibilities into different classes. Here's how we can refactor it:
public class Book {
private String title;
private String author;
private String text;
// Constructor
public Book(String title, String author, String text) {
this.title = title;
this.author = author;
this.text = text;
}
// Getters
public String getTitle() {
return title;
}
public String getAuthor() {
return author;
}
public String getText() {
return text;
}
}
public class BookPrinter {
public void printTextToConsole(String text) {
System.out.println(text);
}
}
public class BookSaver {
public void saveTextToFile(String text, String filePath) {
try (FileWriter writer = new FileWriter(filePath)) {
writer.write(text);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Now, we've got three classes:
Book: This class is only responsible for storing the book's data (title, author, and text).
BookPrinter: This class handles printing the book's text to the console.
BookSaver: This class deals with saving the book's text to a file.
By splitting these responsibilities, we make each class simpler and focus on a single task. This makes our code easier to understand, maintain, and test. If we need to change how the text is saved to a file, we only need to modify the BookSaver
class, leaving the other classes untouched. Similarly, if we want to change how the text is printed to the console, we just modify the BookPrinter
class. This way, our code is much more robust and less prone to bugs.
Conclusion
Uncle Bob often uses real-world analogies. One of his favorite examples is comparing software classes to journalistic principles. Just as a good news article answers "who, what, where, when, and why" in distinct sections, a well-designed class should address a single aspect of the system’s functionality.
Following SRP, and SOLID principles in general, ensures your codebase remains clean, flexible, and maintainable, saving you time and effort in the long run.
Subscribe to my newsletter
Read articles from Suhaimi Sulaiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
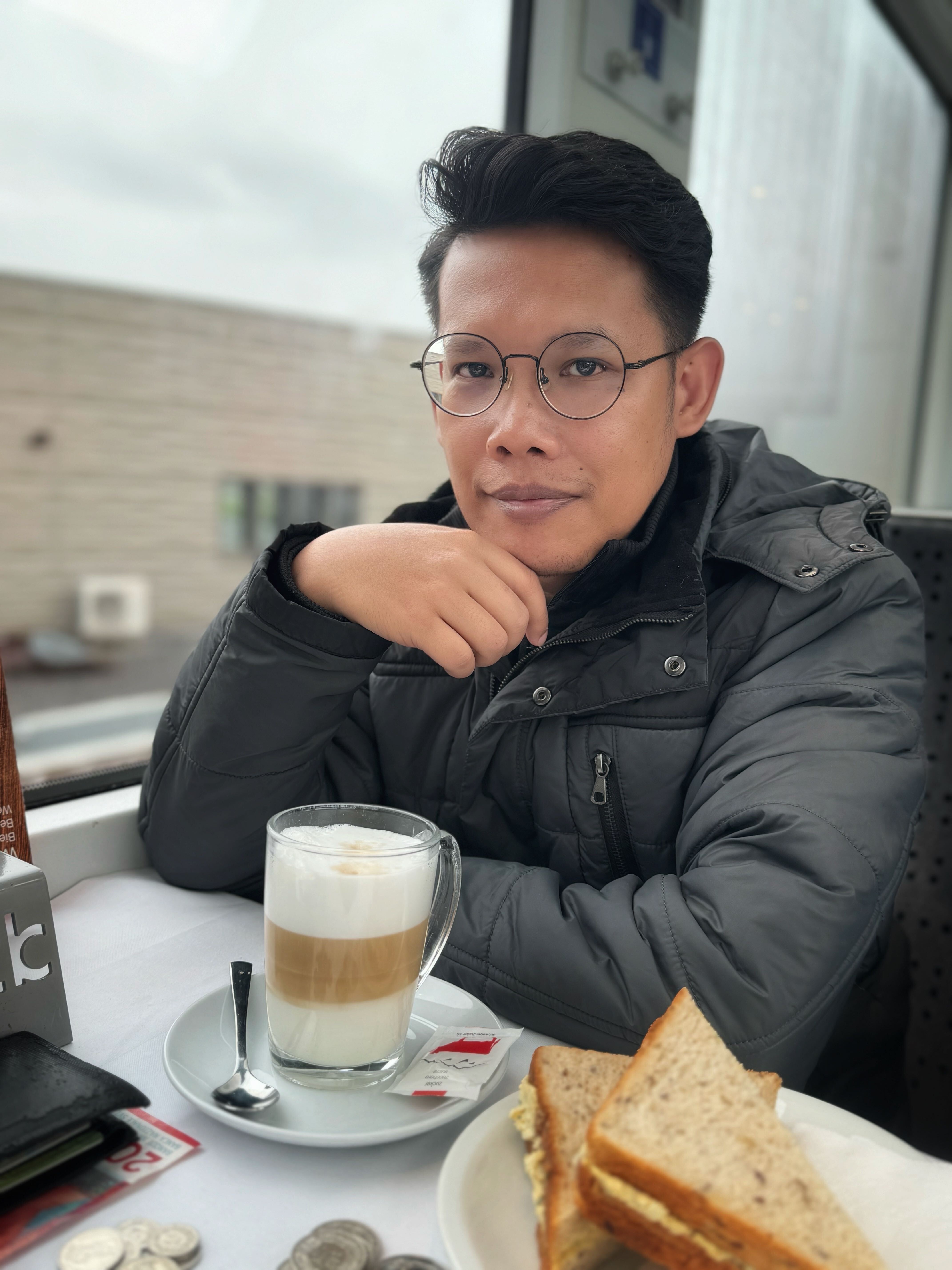
Suhaimi Sulaiman
Suhaimi Sulaiman
I am a Team Lead cum Backend Engineer at Setel Ventures Sdn Bhd.