Optimizing JavaScript Applications with RxJS: Observable Operators
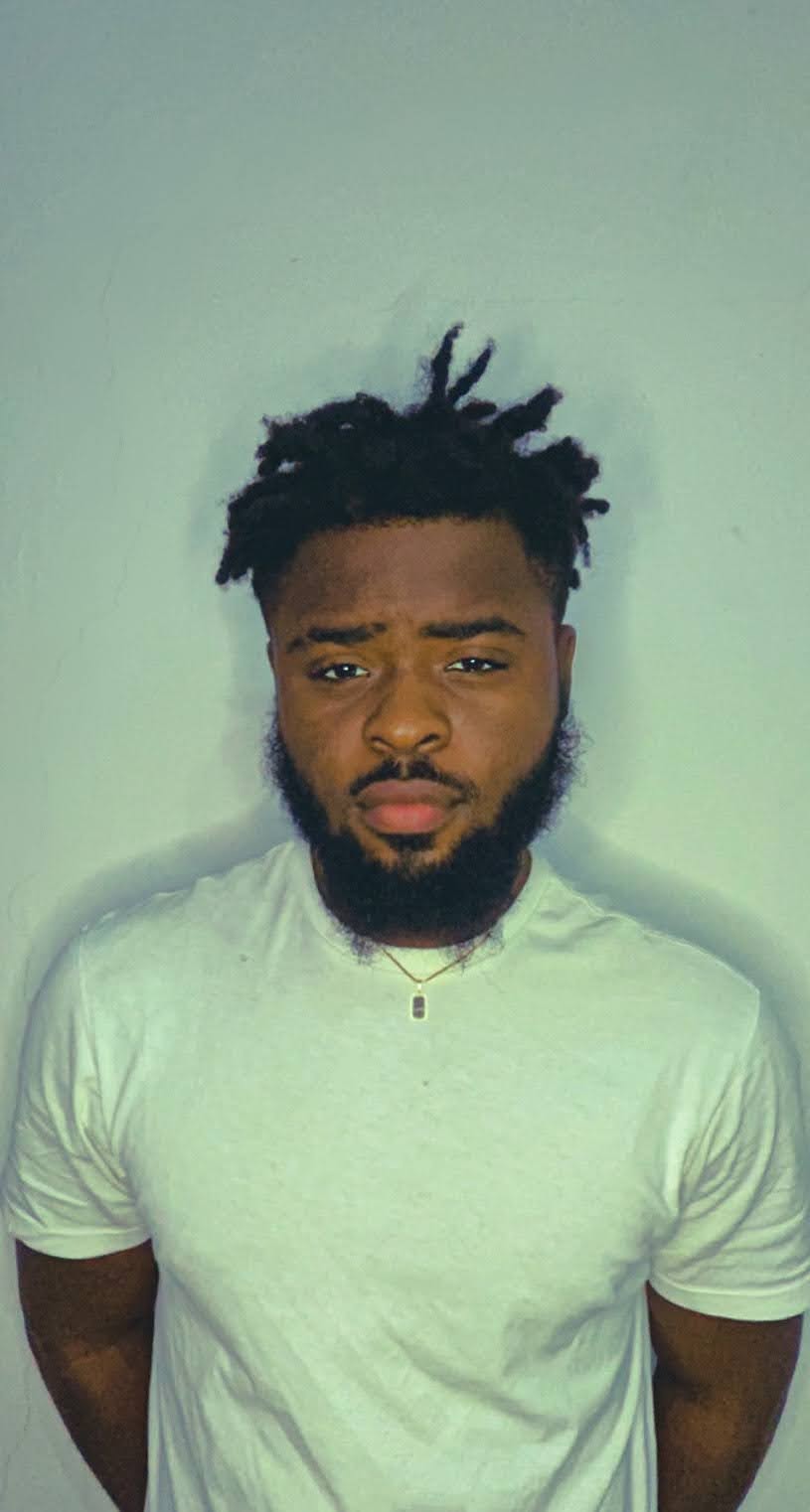
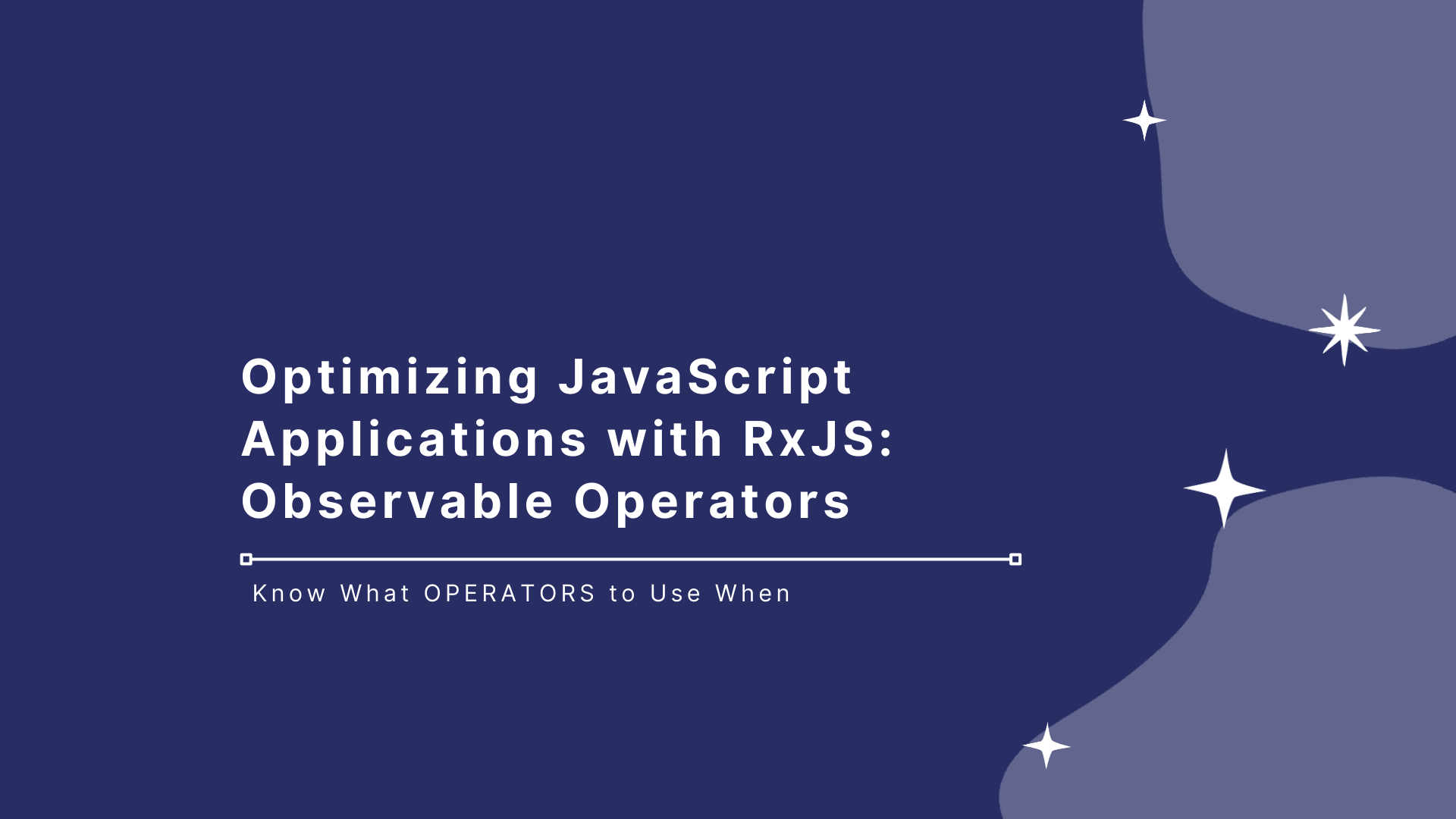
Reactive programming helps in managing asynchronous operations and data streams in JavaScript applications. RxJS (Reactive Extensions for JavaScript) is a library that brings the principles of reactive programming to JavaScript, it helps to create and manage complex asynchronous data flows. Observables, an entity of RxJS, are used to represent and handle these data streams. This guide will help you understand when to use Observables, the different types of Observables available in RxJS, and the functions to use in various scenarios, while also comparing them to typical JavaScript alternatives with code examples.
What is an Observable?
An Observable is a core primitive in RxJS that represents a stream of data that can be observed over time. Observables can emit data, complete, or error out. Subscribers can listen to these events and react accordingly.
When to Use Observables
Observables are particularly useful in the following scenarios:
Asynchronous Data Streams:
Use Case: When dealing with asynchronous data sources like HTTP requests, WebSockets, or any event-driven architecture.
Why Use Observables: Unlike Promises, Observables can handle multiple values over time (streams), Observables also provide operators for transforming and combining data streams. Promises are more suitable for single asynchronous operations that resolve or reject once.
Event Handling:
Use Case: When managing user interactions like clicks, mouse movements, or keyboard events.
Why Use Observables: Like Javascript's
addEventListener.
Observables can easily combine and transform multiple events and provide a declarative approach to handling events. Event listeners often require manual setup, making them more cumbersome for complex scenarios.
State Management:
Use Case: When managing state in complex applications, especially when state changes need to be propagated throughout the application,.
Why Use Observables: Observables can provide real-time updates and transformations of states.
Animation and timing:
Use Case: When working with animations or timed events.
JavaScript Alternative:
setTimeout
,setInterval
, or CSS animations.Why Use Observables: Observables provide a unified approach to handling time-based events, allowing for complex timing logic and composition with other streams, which is not easily achievable with
setTimeout
orsetInterval
.
Streams and Data Transformation:
Use Case: When transforming or combining data streams.
JavaScript Alternative: Array methods (e.g.,
map
,filter
,reduce
).Why Use Observables: Observables extend the concept of data transformations to asynchronous data streams, allowing for more complex and powerful transformations compared to array methods that work only with synchronous data.
Types of Observables in RxJS
RxJS provides several types of Observables, each suited for different use cases:
Cold Observables:
Characteristics: These Observables do not start emitting values until an observer subscribes to them.
Use Case: HTTP requests are often cold Observables because the request is made only when there is a subscriber.
Hot Observables:
Characteristics: These Observables start emitting values even if there are no subscribers.
Use Case: WebSocket connections or user input events.
Subject:
Characteristics: Subjects are types of Observable that allows multicasting to multiple observers.
Types of Subjects:
Subject: Basic Subject that acts as both an observer and an Observable.
BehaviorSubject: Emits the last value to new subscribers.
ReplaySubject: Emits a specified number of the most recent values to new subscribers.
AsyncSubject: Emits the last value only when the Observable completes.
Operators to Use in RxJS
RxJS provides a rich set of operators to create, transform, and combine Observables. Below are some key operators and functions categorized by their use cases:
Creating Observables
of: Creates an Observable from a sequence of values.
import { of } from 'rxjs'; const observable = of(1, 2, 3);
from: Creates an Observable from an array, promise, or iterable.
import { from } from 'rxjs'; const observable = from([1, 2, 3]);
fromEvent: Creates an Observable from DOM events.
import { fromEvent } from 'rxjs'; const observable = fromEvent(document, 'click');
JavaScript Alternative:
document.addEventListener('click', (event) => { console.log(event); });
Why Use
fromEvent
: Allows for event handling, easy composition, and transformation of events using RxJS operators.interval: Creates an Observable that emits values at specified intervals.
import { interval } from 'rxjs'; const observable = interval(1000);
JavaScript Alternative:
setInterval(() => { console.log('tick'); }, 1000);
Why Use
interval
: It provides a declarative approach to handling intervals and integrates with other RxJS operators for more complex scenarios.timer: Creates an Observable that emits a value after a delay and optionally continues emitting values at specified intervals.
import { timer } from 'rxjs'; const observable = timer(2000, 1000);
JavaScript Alternative:
setTimeout(() => { console.log('initial delay'); setInterval(() => { console.log('tick'); }, 1000); }, 2000);
Why Use
timer
: Abstract the functionalities ofsetTimeout
andsetInterval
in a more simple way.
Transforming Observables
map: Transforms the items emitted by an Observable by applying a function to each item.
import { map } from 'rxjs/operators'; observable.pipe(map(x => x * 2));
JavaScript Alternative:
const array = [1, 2, 3].map(x => x * 2);
filter: Filters items emitted by an observable by only emitting those that satisfy a specified predicate.
import { filter } from 'rxjs/operators'; observable.pipe(filter(x => x > 1));
JavaScript Alternative:
const array = [1, 2, 3].filter(x => x > 1);
Why use filter: Applies filtering to asynchronous data streams.
switchMap: Maps each value to an Observable and flattens all of these inner Observables using switch.
import { switchMap } from 'rxjs/operators'; observable.pipe(switchMap(x => anotherObservable));
Illustration from RxJs Documentation
Why use
switchMap
: Efficiently handles switching to new Observables, and canceling previous ones.
Combining Observables
merge: Combines multiple Observables into one by merging their emissions. This means that the resulting Observable will emit all the values from each of the input Observables as they occur
import { merge } from 'rxjs'; const merged = merge(observable1, observable2);
Why use
merge
: Provides a declarative and concise way to merge multiple streams.concat: Concatenates multiple Observables by emitting their values sequentially.
import { concat } from 'rxjs'; const concatenated = concat(observable1, observable2);
Why use
concat
: Simplifies sequential execution of multiple streams.The merge and concat operators in RxJS both combine multiple observables, but they do so in fundamentally different ways to suit different use cases. The merge operator subscribes to all input observables simultaneously. Emissions from any of the input observables can appear in the result without any guarantee of order. This is useful when you need to handle multiple streams of data concurrently, such as user interactions and system events, where the order of events is not a primary concern. Concat is the direct opposite, as it guarantees sequential order.
combineLatest: Combines multiple Observables to create an Observable whose values are calculated from the latest values of each of its input Observables.
import { combineLatest } from 'rxjs'; const combined = combineLatest([observable1, observable2]);
Error Handling
catchError: Catches errors on the source Observable and returns a new Observable or throws an error.
import { catchError } from 'rxjs/operators'; observable.pipe(catchError(error => of('Error!')));
retry: Retries the source Observable a specified number of times in case of error.
import { retry } from 'rxjs/operators'; observable.pipe(retry(3));
Utility Operators
tap: Transparently performs side effects for notifications from the source Observable.
import { tap } from 'rxjs/operators'; observable.pipe(tap(value => console.log(value)));
Why Use
tap
: Separates side effects from the main logic, keeping code cleaner and more maintainable.debounceTime: Emits a value from the source Observable only after a particular time span has passed without another source emission.
import { debounceTime } from 'rxjs/operators'; observable.pipe(debounceTime(300));
take: Emits only the first
n
values emitted by the source Observable.import { take } from 'rxjs/operators'; observable.pipe(take(5));
Why Use
take
: Simplifies limiting the number of emissions from a stream.
Conclusion
Observables in RxJS provide a simple and elegant way to handle asynchronous data streams, events, and complex data transformations in JavaScript applications. I compared Observables with typical JavaScript alternatives, highlighting their advantages, to make it clear when and why to choose Observables for certain scenarios.
Subscribe to my newsletter
Read articles from AJIBADE HAMMED directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
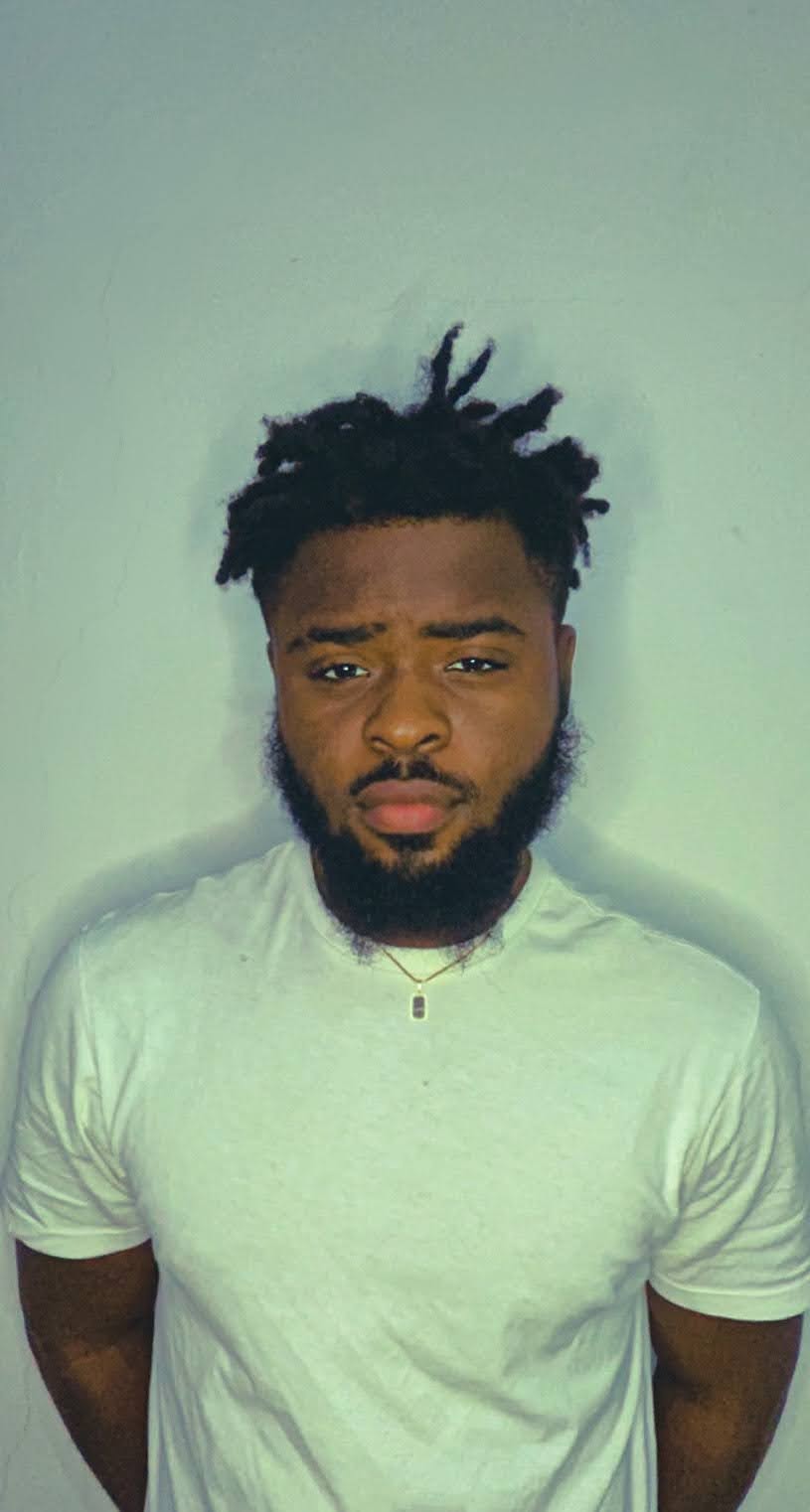
AJIBADE HAMMED
AJIBADE HAMMED
A professional software engineer with years of experience in an array of programming languages and frameworks. I appreciate tackling complicated problems using well-thought-out strategies and simple design solutions, as well as leveraging technology to positively touch the lives of people all over the world.