Unlocking the Power of Data Type Conversions in Python

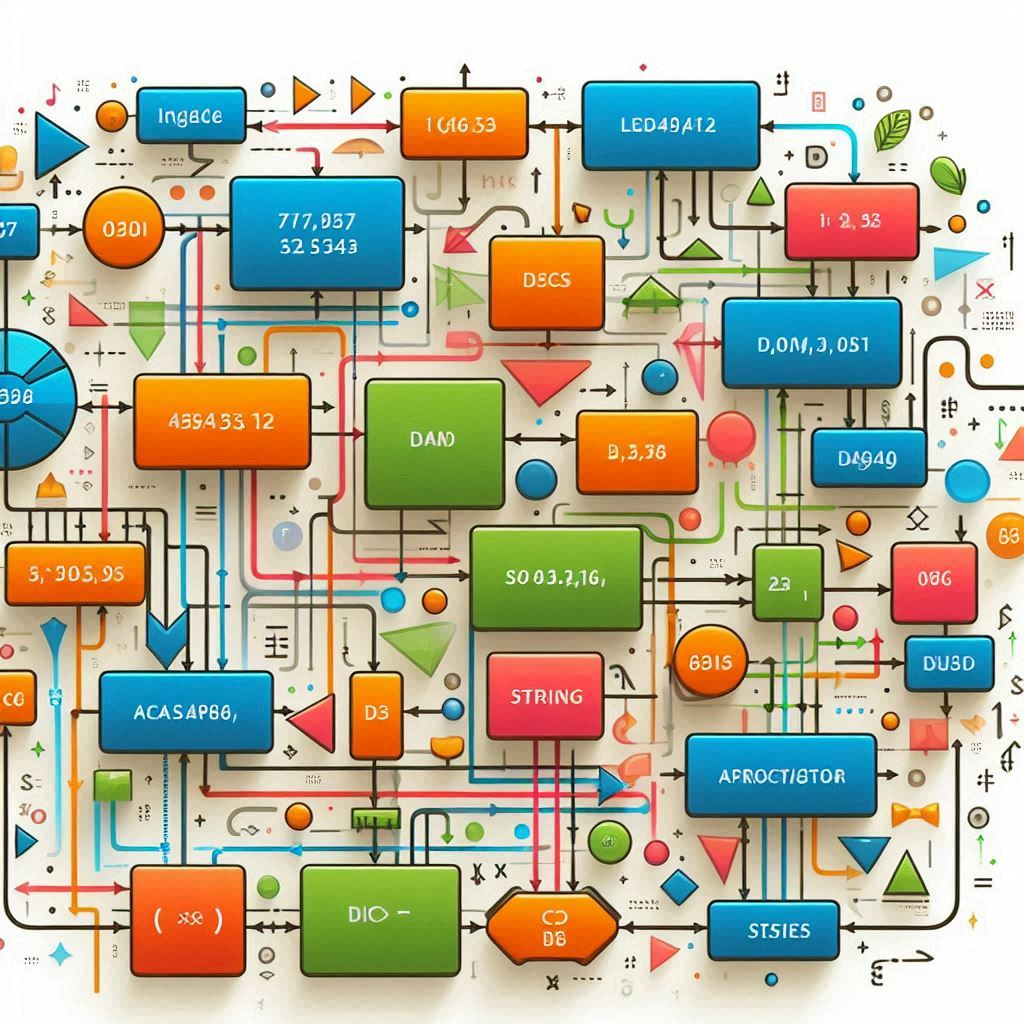
In Python, every data type created is treated as an object, offering immense flexibility in manipulating and converting data. Understanding how to convert between different data types is a fundamental skill for any Python programmer, enabling seamless data processing and transformation. Let's delve into some essential data type conversion techniques and explore their significance through practical examples.
Converting Binary to Decimal and Vice Versa:
Converting binary strings to decimal integers and vice versa is a common task in programming. To convert a binary string to decimal, use the int()
function with base 2. Conversely, to convert a decimal integer to binary, utilize the bin()
function. Remember to remove the leading '0b' from the binary representation using slicing.
binary = '11'
decimal = int(binary, 2) # Output: 3
binary = bin(decimal)[2:] # Output: 11
Converting Characters to Binary:
Converting characters to binary involves converting each character's Unicode representation to binary using the ord()
function. This is particularly useful for encoding text data into binary form.
char = 'A'
binary = bin(ord(char))[2:] # Output '1000001'
Converting Strings to Binary:
To convert an entire string to binary, iterate over each character in the string, convert it to binary, and concatenate the binary representations.
string = 'Hello'
binary_string = ' '.join(bin(ord(char))[2:] for char in string)
# Output: 1001000 1100101 1101100 1101100 1101111'
Converting Lists to Integers and Vice Versa:
Converting a list of integers into a single integer involves joining the integer elements of the list into a string and then converting it to an integer. Conversely, converting a string of space-separated integers into a list is achieved by splitting the string and mapping each element to an integer.
nums = [1, 2, 3]
num = int(''.join(map(str, nums))) #Output: 123
l = '12 9 61 5 14'
nums_list = list(map(int, l.split())) #Output: [12, 9, 61, 5, 14]
Converting Lists to Strings and Vice Versa:
To convert a list into a string, join the elements of the list into a string using the join()
method. Conversely, converting a string into a list can be done by splitting the string.
nums = [1, 2, 3]
str_nums = str(' '.join(map(str, nums))) #Output: "1 2 3"
Converting Lists to Dictionaries
1. Converting Two Lists into a Dictionary:
One common use case is converting two related lists into a dictionary, where one list contains keys and the other contains values. This can be achieved using the zip()
function combined with the dict()
constructor.
codekeys = ['name', 'age', 'city']
values = ['Alice', 28, 'New York']
dictionary = dict(zip(keys, values))
print(dictionary)
# Output: {'name': 'Alice', 'age': 28, 'city': 'New York'}
2. Converting a List of Tuples into a Dictionary:
If you have a list of tuples, where each tuple contains a key-value pair, you can directly convert it into a dictionary using the dict()
constructor.
list_of_tuples = [('name', 'Bob'), ('age', 25), ('city', 'Los Angeles')]
dictionary = dict(list_of_tuples)
print(dictionary)
# Output: {'name': 'Bob', 'age': 25, 'city': 'Los Angeles'}
Converting Dictionaries to Lists
1. Converting Dictionary Keys to a List:
To extract the keys from a dictionary and store them in a list, you can use the keys()
method.
dictionary = {'name': 'Alice', 'age': 28, 'city': 'New York'}
keys_list = list(dictionary.keys())
print(keys_list)
# Output: ['name', 'age', 'city']
2. Converting Dictionary Values to a List:
Similarly, to extract the values from a dictionary, use the values()
method.
dictionary = {'name': 'Alice', 'age': 28, 'city': 'New York'}
values_list = list(dictionary.values())
print(values_list)
# Output: ['Alice', 28, 'New York']
3. Converting Dictionary Items to a List of Tuples:
To convert the key-value pairs of a dictionary into a list of tuples, use the items()
method.
dictionary = {'name': 'Alice', 'age': 28, 'city': 'New York'}
items_list = list(dictionary.items())
print(items_list)
# Output: [('name', 'Alice'), ('age', 28), ('city', 'New York')]
Conclusion: Mastering data type conversions in Python unlocks a myriad of possibilities in data manipulation and processing. Whether it's converting between binary and decimal, encoding text data into binary form, or transforming lists into strings and vice versa, understanding these conversion techniques is essential for efficient programming. By harnessing the power of data type conversions, Python programmers can tackle a wide range of tasks with ease and efficiency, making their code more versatile and adaptable to diverse scenarios. So, dive into the world of data type conversions and elevate your Python programming skills to new heights!
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.